Alert System with Twillio SendGrid and Node.js
Time to read: 5 minutes
Alert system with Twilio SendGrid and Node.js
In the world of IoT, a common case is to monitor specific parameters of a system or device and send notifications and alerts in the case if the parameters are beyond predefined threshold limits.
Suppose you have to enhance an air quality monitoring system with a possibility to send email notifications in the case when the air temperature or concentration of 10 mm particles exceed the threshold values. This tutorial describes how it can be implemented with the usage of Twillio SendGrid platform and Node.js technology. The development process is described in context of using Linux OS. All these steps can be performed in other OS as well, with a minimal adjustment.
Requirements
- Node.js version 6, 8 or >=10
- A SendGrid account - if you’re new to Twilio/SendGrid create a free account
- A text editor or IDE - Visual Studio Code is used in this tutorial, but you can use whatever tool you are accustomed with.
Project set-up
First of all, you need to get your SendGrid API key. Provided having a SendGrid account, you can generate a new API key for use in your email applications. If you have Node and npm packages installed and running in your computer, you can create a new directory, say, alert-system, and run the following command inside it:
Running the npm init
command initiates a prompt to create a package.json file. As the prompt explains, you’ll walk through configuring the most basic settings of your Node.js application in this file.
For now, you can leave index.js as the entry point, along with a short description and your name as the author, and elect to use the default values offered by pressing the Enter key until you reach the end of the prompt. Then you’re asked to confirm your settings with a preview of your package.json file. Press Enter to confirm and return to the regular terminal prompt.
Then, you’ll need to install the SendGrid package:
This command puts all necessary dependencies inside the node_modules directory. The --save
flag saves the sendgrid/mail
package as a dependency for this project.
To communicate with the SendGrid server, you need to inject the API key into your application. It is not recommended to hard-code the key into the application code due to possible security issues, so you need to pass it to the application in a secure way, e.g. via an environment variable. You can get details about setting environment variables here. In the development environment, you can, for example, create a set-up script, say sendgrid.env, containing the following command:
The script can be run with the source
command:
Then you use the key as follows:
In general, there are two approaches to implement a monitoring system: the push-data approach and pull-data approach. In the push-data case, you provide a possibility to push data into your system from an external source. In the pull-data case, your system pulls data from an external source itself. For the beginning, you can implement the push approach first. Then you would be able to add the pull functionality as well.
Air quality alert system – the data-push approach
To enable pushing data into your system, you expose a REST endpoint, which can be called by external data producers. It is possible to use the express
library for it:
Suppose an external service sends the measurement data in the following format:
For these input data, you can implement the data processing endpoint as follows.
It is good to put all configuration settings into the configuration script, e.g. config.js, to be used by all the application components:
where: <recipient email address>
is the destination email address you want to send the alert to; <your verified sender email address>
is the email address you registered as the verified sender address .
Next, you implement the main script, index.js, containing the basic application logic:
where the email-alert-system.js script encapsulates all business logic for sending emails with the usage of SendGrid service:
To start the application, you can use the following command:
If you see the following statement in the console:
then the application is ready to accept data requests. Now you can test it with the usage of a HTTP client such as Postman . You can send the following test data, for example:
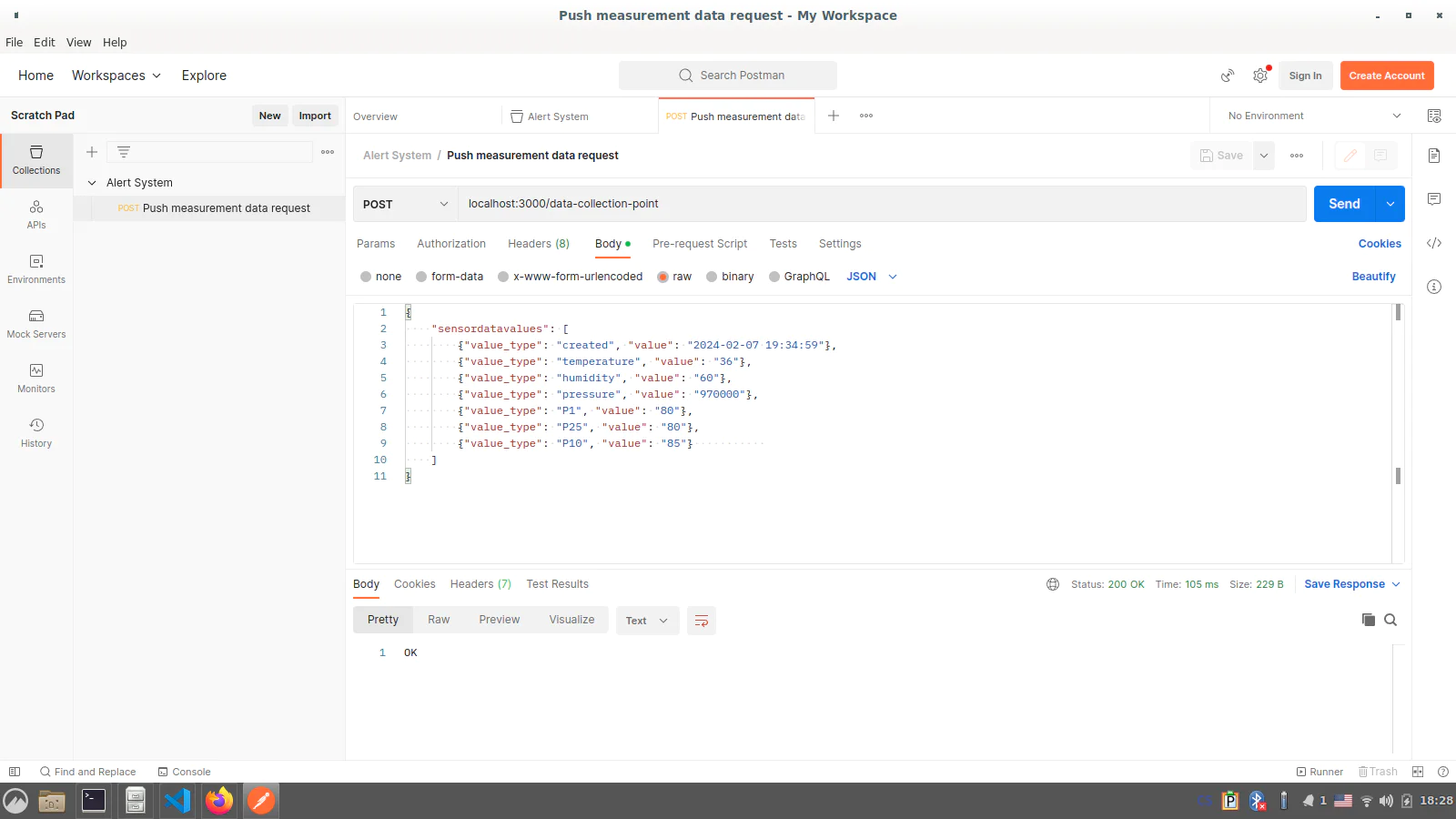
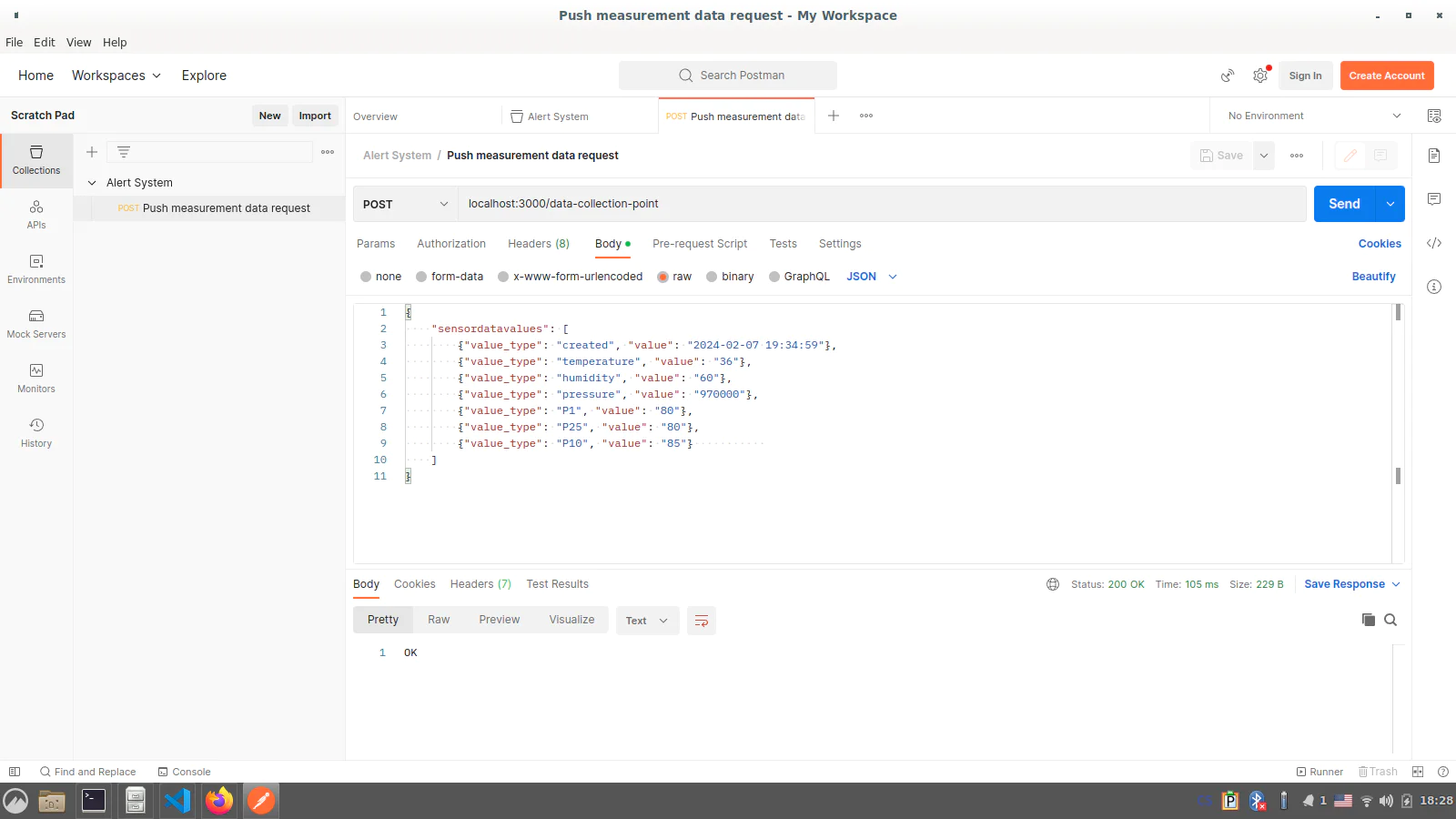
After sending the request, you should see something similar in the console:
If there are the corresponding new messages in your mailbox, it means that your system works correctly. Congratulations! Now you can enhance the system with data-pull staff.
Air quality alert system – the data-pull approach
Suppose, the sensor layer exposes an endpoint providing current readings of the air condition parameters. To simulate such a service, you can use the `json-server` tool. This is a package for creating web services from JSON data or JavaScript code. It can be installed as a common node package:
where 0.17.4 is the latest stable version of json-server at the moment of writing.
Flag --save-dev
indicates that this package is used during development and will not be part of the alert system application.
To use the json-server
tool, you need to prepare test data as a JavaScript file, e.g. test-sensor-data.js:
Then you start the mock service with the following command:
You need to add the mock sensor host connection parameters to your configuration settings:
where sensorHost.samplingInterval
is the frequency of retrieving the measurement data.
Now you can use the http
package in the index.js script to send requests to the sensor layer endpoint for pulling the data:
If you start the application now, you can see in the console something like the following output:
and, correspondingly, a new message in the mailbox. After the time interval defined in the configuration (sensorHost.samplingInterval
) , you should see a similar output added in the console and the next message in the mailbox. If it is the case, it means that your pull-data stuff works correctly!
Troubleshooting and additional notes
If you see the following output in the console after running the application:
This means that the environment variable SENDGRID_API_KEY
is not set. You can set it, for example, by running the sendgrid.env script: source ./sendgrid.env
.
Conclusion
In this tutorial, you created an alert service to send email notifications under conditions defined by the business logic. This application can be used as a prototype for real IoT projects as well as in other commercial and industrial systems. Used technologies allow easily adjusting the application behavior according to specific needs for each particular use case. SendGrid service provides a lot of possibilities for customization of the email sending process, like dynamical templates, sending multiple emails with address tags, to name a few. You can check SendGrid documents for details.
Sergiy Pylypets is a Full Stack Developer and Data Engineer in Nový Dům, Central Bohemia, Czechia, reach out on LinkedIn to connect.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.