How to Create A Text-To-Subscribe App in PHP Using Twilio and Laravel
Time to read: 5 minutes
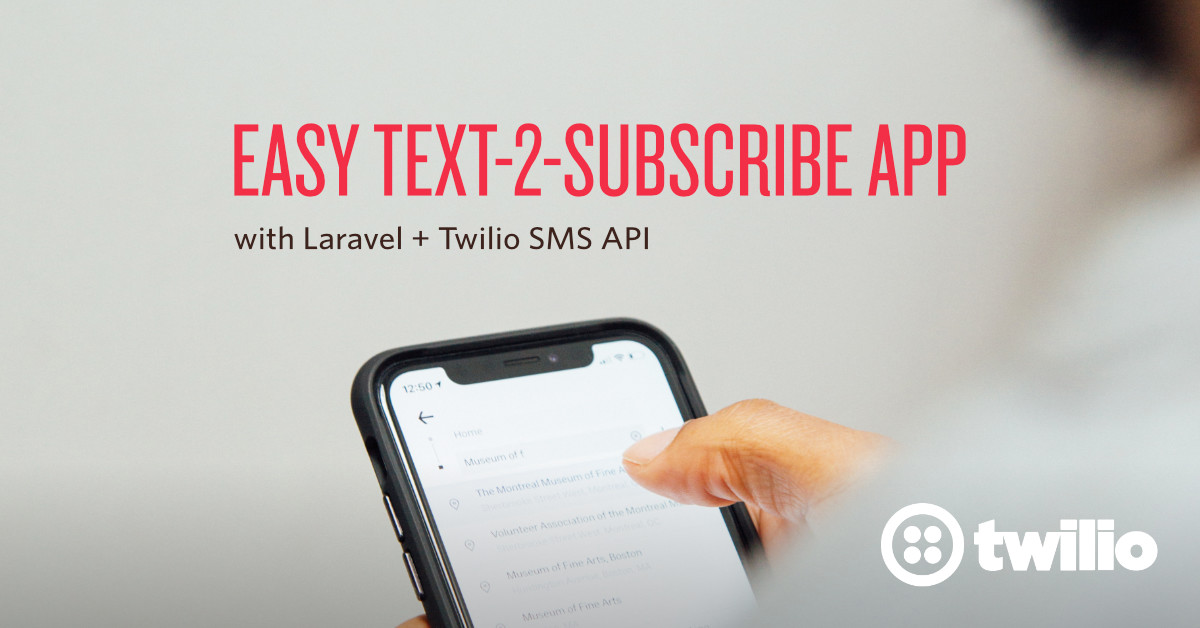
Introduction
The world has gone global and almost everyone has access to the internet every day. However, we shouldn’t leave out those without smartphones or regular PC’s. One way to cater to them is by creating SMS friendly applications, where even with the least data-capable phones, they can access our services. In this tutorial, we will use Laravel and the Twilio SMS API to make a text-to-subscribe app.
Perquisites
This tutorial assumes that the reader has a basic knowledge of PHP and Laravel. It also assumes that you have Composer set up and installed on your local system.
Set Up A Twilio Phone Number
To create a text-to-subscribe app, we need a phone number where our users can send text messages to. When the messages are received they will call our API to trigger the actions.
Login to your Twilio account if you already have one, or sign up for a new one. Once you are logged in, locate the # sign at the left side-bar of the site, and click on it. This will open a new page which shows a button that says “Get your first Twilio phone number”. Click the button and choose the selected number as shown below:
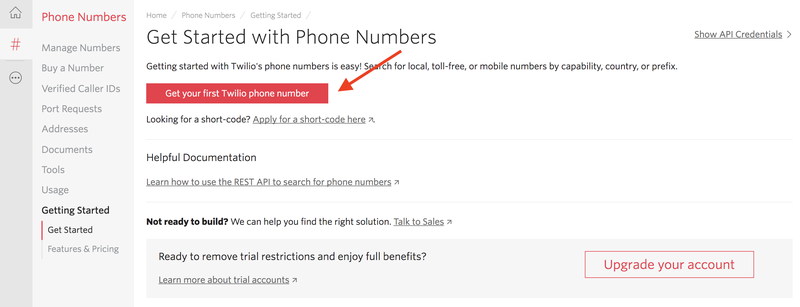
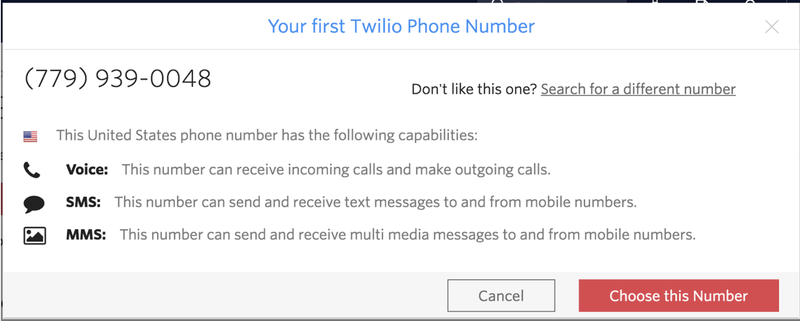
Extend The Inbuilt Laravel Registration Form To Accept A Phone Number
Now we have our phone number ready, let’s create a Laravel app. To do that, we run:
Now that we have our basic app set up, we need to create the auth scaffolding so we can edit it while extending the inbuilt Laravel authentication system. To do that, we run:
Once the command has completed, before we run our migrations, let’s edit our user's migration to have a new column called phone
. Open database/migrations/2014_10_12_000000_create_users_table.php file
and replace the code with:
If you look at the code above, you will notice the addition of this line $table->string(‘phone’);
.
Next, we need to update the controller, models, and views to recognize the new phone field. Open app/Http/Controllers/Auth/RegisterController.php
and replace with:
In the code above, we will notice two new additions:
- The first addition is the phone field we have added in the validator-rules, which sets the phone field to be unique and also required.
- The second addition is in the array passed to the
User::create
call where we add the phone field to the data to be stored while creating a new user.
Now that our controller knows of this new field, we are ready to add it to the fillable array in our model, or else, it will be ignored. Open the /app/User.php
file and add the phone field to the fillable array, so that it reads as follows:
For the final stage of extending this field, we have to add an input to collect and display errors from the field in the register view field. Open resources/views/auth/register.blade.php
and add the following block to the form:
Implement A Callback API To Interact With Your Twilio Phone Number
Now that we have set up our app to receive phone numbers on registration, it is time to set up a callback to interact with our Twilio number. First, let’s create a new model and controller to handle subscriptions. To do that, let’s run:
The above command will generate a model, controller and migration file for subscriptions. Open the newly generated migration and replace it with the following code:
The migration above is simple. Here, we create a table that holds the user_id
which is the id of the client and the subscription status which is a boolean. Next, we run migrations so we can have all the tables migrated.
NOTE: Before you run migrations, you must have updated the DB driver and connection details in your .env
file.
Add the following route to our routes/api.php
file:
In this route, we called the index
method of our SubscriptionsContoller
class, which we will create now. Open the app/Http/Controllers/SubscriptionsController.php
, and add the following use statement:
We want to use the Log class above to see what the content Twilio sends to us will look like. Next, create a new index()
method as seen below:
To get the Log from Twilio, we need to set our development API as our callback URL. To do that, we need to expose our API to the public and then set it as our callback URL in Twilio settings. We will expose our API to the public by installing and using Ngrok. You can install Ngrok by following the instructions here. Once installation is complete, we fire it up by directing DNS traffic to the port Laravel is running from:
Next, we add the generated link to our callback by going here, clicking on the number we want to use, and then updating the webhook with our new link so it looks like this:
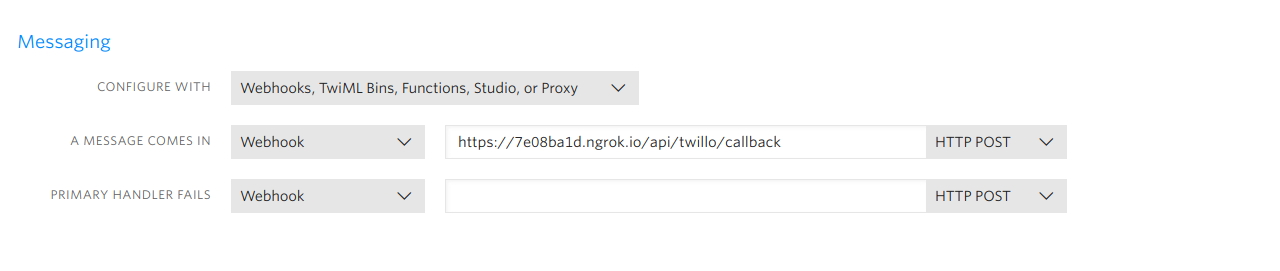
Adding Verified Caller IDs So We Can Test Our App
An existing non-Twilio phone number, like the number to a wireless phone or a landline in your home or office, must be validated on your Twilio project before it can be used for an outbound call or SMS testing. A free trial account is available, should you need it for testing.
- Login to your account at www.twilio.com/console.
- Click Phone Numbers #
- Click Verified Caller IDs.
- Click the red + sign icon
- +, or Verify a number.
- Enter the desired phone number to verify, and then click Call Me.
NOTE: Click Text you instead to receive a text message for verification.
Now that we have verified our phone number, we can send any text to the phone number which we created via Twilio from our verified number, and the post value would be logged. If we check our Laravel log, we will see a structure similar to this:
Implement The Twilio SDK To Send Messages Back To The Subscribing Number
Now that we know the structure of the incoming message data. let’s implement the actual subscribing and responding via SMS. To get started, add the Twilio SDK to your Laravel project by running the following:
Next, replace the content of app/Http/Controllers/SubscriptionsController.php
with:
What is going on in the code above? First, we require the Twilio SDK. Then we set it up using our account sid
and the secret key
. (To know where to get your own credentials, please read here.) Next, we capture the message, sender, and the phone number to which it was sent (our Twilio number). We then see if the user is already registered or not. When the user isn’t registered, we send them a message asking the user to register.
In the event that the user is registered, we check for which command the user has sent and trigger the corresponding function. When the command isn’t one we observe, we send the user the list of commands we accept.
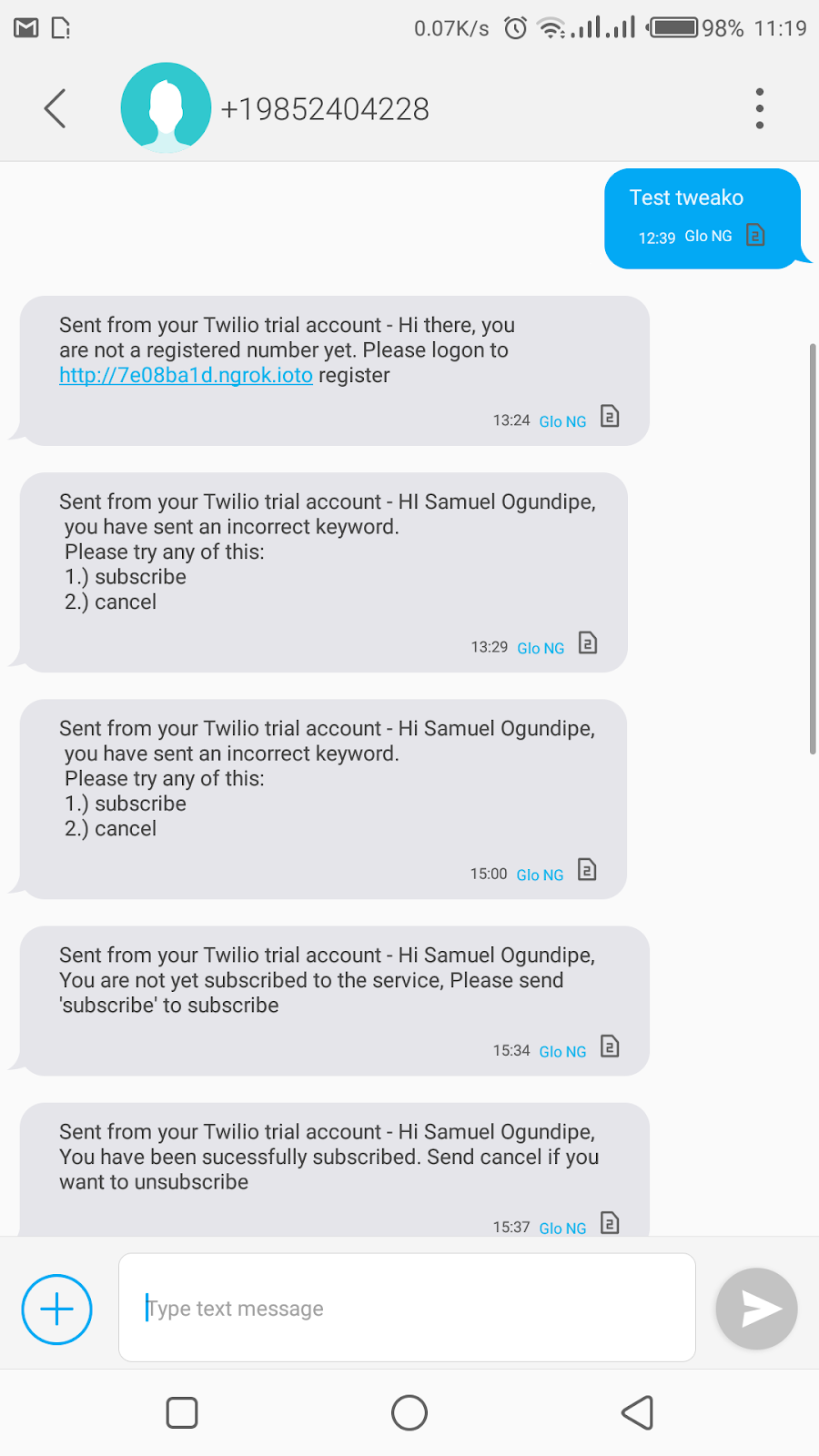
Conclusion
Now you have set up a simple text to subscribe app! You can see how easy it is to repeat this formula and set up more interesting applications in less time. You could extend the current application by accepting registrations via the text to subscribe method, which makes your app even better. The code base to this lesson is available in a publicly hosted GitHub Repository. Please experiment with it and let me know
Ogundipe Samuel. Software Engineer and Technical writer. I would love to connect with you on my Website, Github and Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.