Implement 2FA in Go Web Apps With Twilio Verify and Gin-Gonic
Time to read: 6 minutes
Implement 2fa in Go Web Apps With Twilio Verify and Gin-Gonic
Two-factor verification (2FA) is a security feature that protects your online accounts. For example, it could require you to enter a code sent to your phone or email, in addition to your username and password, when you log in. This way, even if someone knows your password they cannot access your account without the code.
In this tutorial, you will learn how to implement 2FA in your Go web applications using Twilio Verify and Gin-Gonic.
Prerequisites
To follow along with this tutorial, you will need the following:
- Go installed on your system
- A Twilio account (free or paid). If you are new to Twilio, click here to create a free account.
- A Twilio phone number that has SMS capabilities
- Familiarity with the Go programming language would be ideal
Two-factor verification in Go and its importance
2FA is important for web applications because in addition to helping prevent unauthorized access, it helps prevent data breaches and identity theft. By using 2FA, you can enhance the security of your users and your application, and comply with best practices and standards in the industry. 2FA also improves user trust and confidence in your service, as they know their accounts are better protected.
What is Gin-Gonic?
Gin-Gonic is a web framework for the Go programming language. Its powerful, streamlined architecture provides a lightweight HTTP router that is fast and has robust middleware compatibility. Gin-Gonic is a popular option for creating web applications and APIs in Go due to its efficiency, simplicity, and user-friendliness. Gin-Gonic allows developers to quickly and effectively construct scalable web services with minimal boilerplate code.
What is Twilio Verify?
Twilio Verify is a service that simplifies sending and verifying one-time passcodes (OTPs) for Multi-Factor Authentication (MFA). Twilio Verify can send OTPs via SMS, voice, email, or WhatsApp, and verify them with a simple API call. Twilio Verify also handles global compliance , routing, and formatting complexities.
Twilio Verify offers several compelling features that make it an excellent choice for developers looking to integrate MFA into their applications. Here are some of its standout features:
- Multi-Channel Verification: Twilio Verify supports various channels, including SMS, voice, and email, giving users flexibility in receiving their verification codes.
- Global Reach: With Twilio’s extensive network, you can reach users anywhere worldwide, ensuring your application is globally accessible.
- Simplified Compliance: Twilio Verify helps adhere to various compliance requirements, making it easier to manage the complexities of global communications laws.
Create the Go app
Retrieve your Twilio credentials
To get started, sign in to your Twilio Console , scroll to the bottom of the page, and you should see your Account SID and Auth token, as shown in the image below, in the Account Info panel. Copy these values and keep them in a safe space for now.
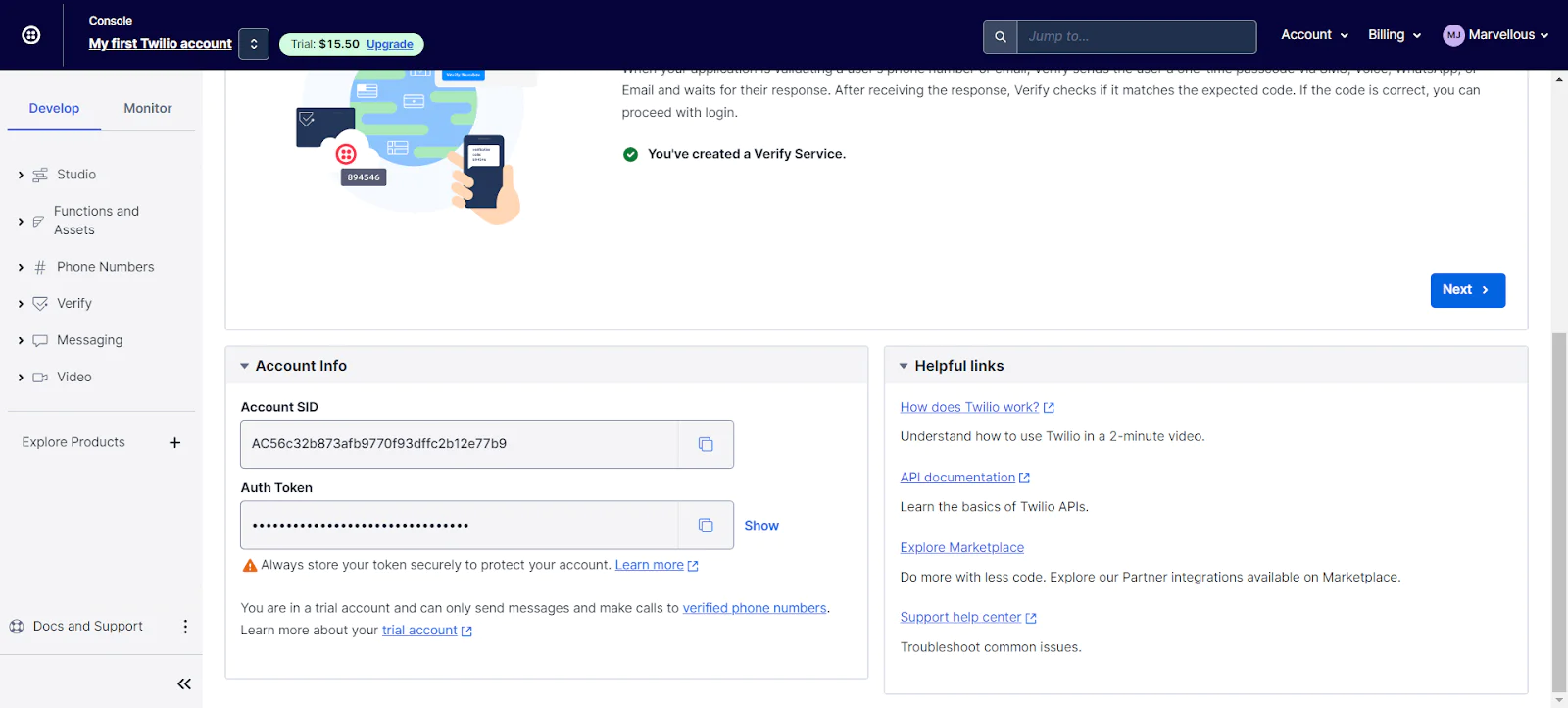
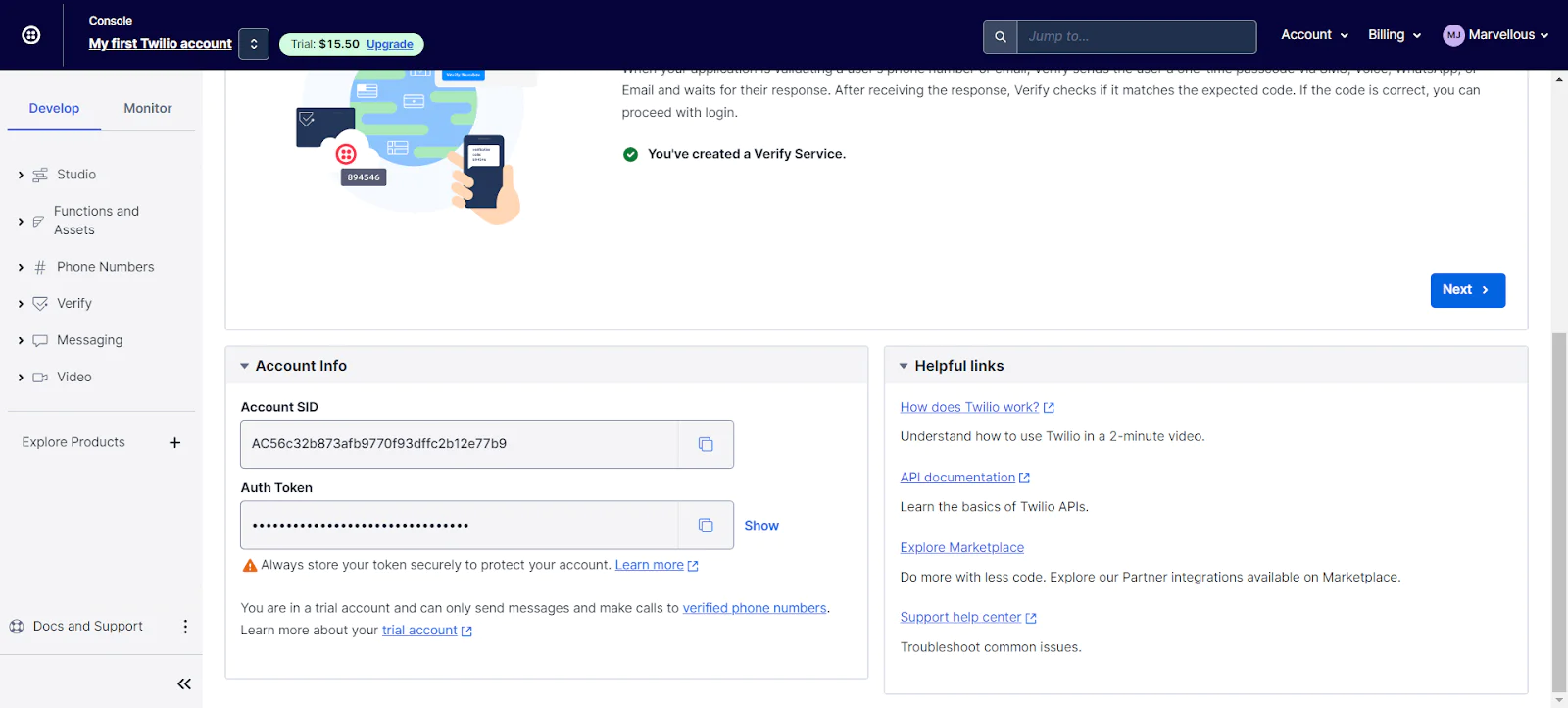
Next, navigate to the Explore Products > Verify (under User Authentication & Identity) > Services page, via the left-hand side menu bar of the Twilio Console.
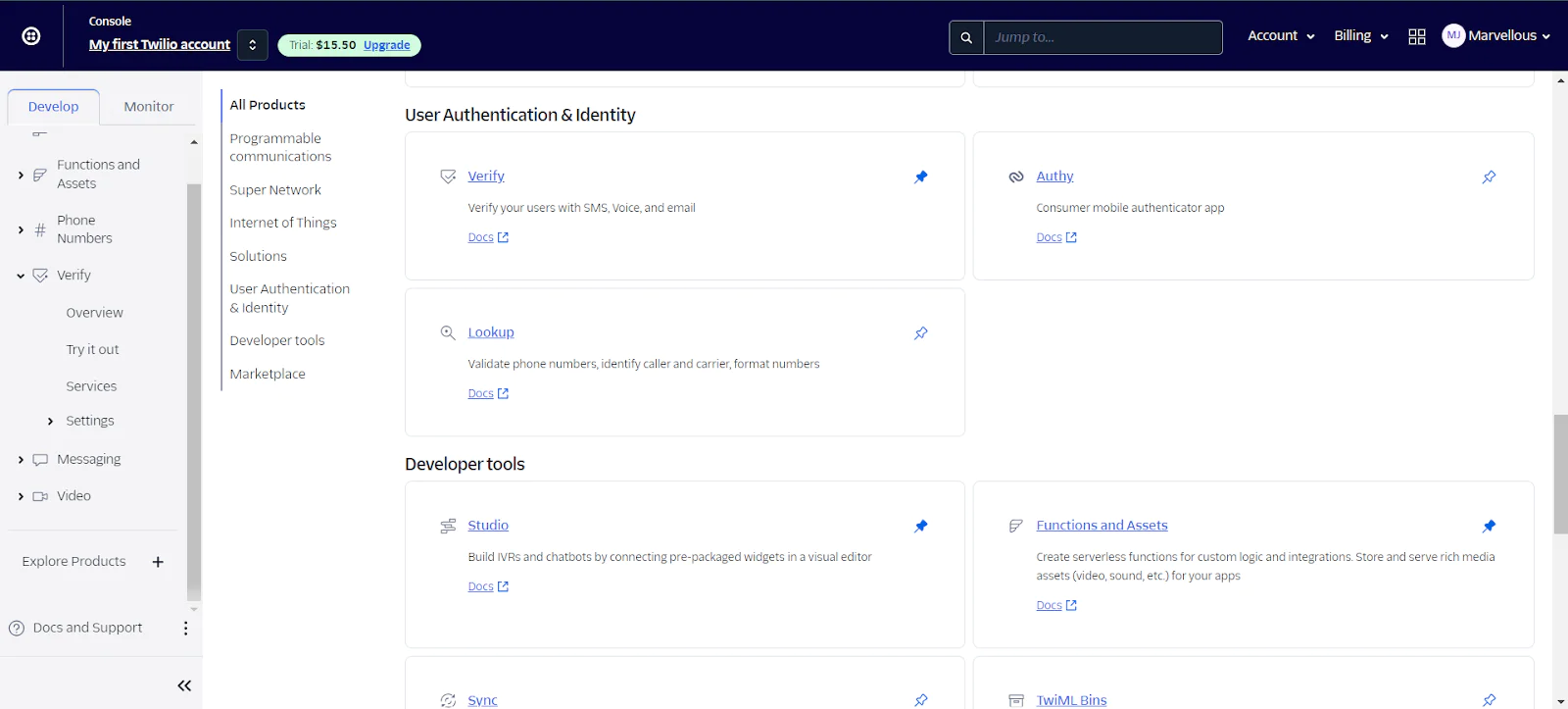
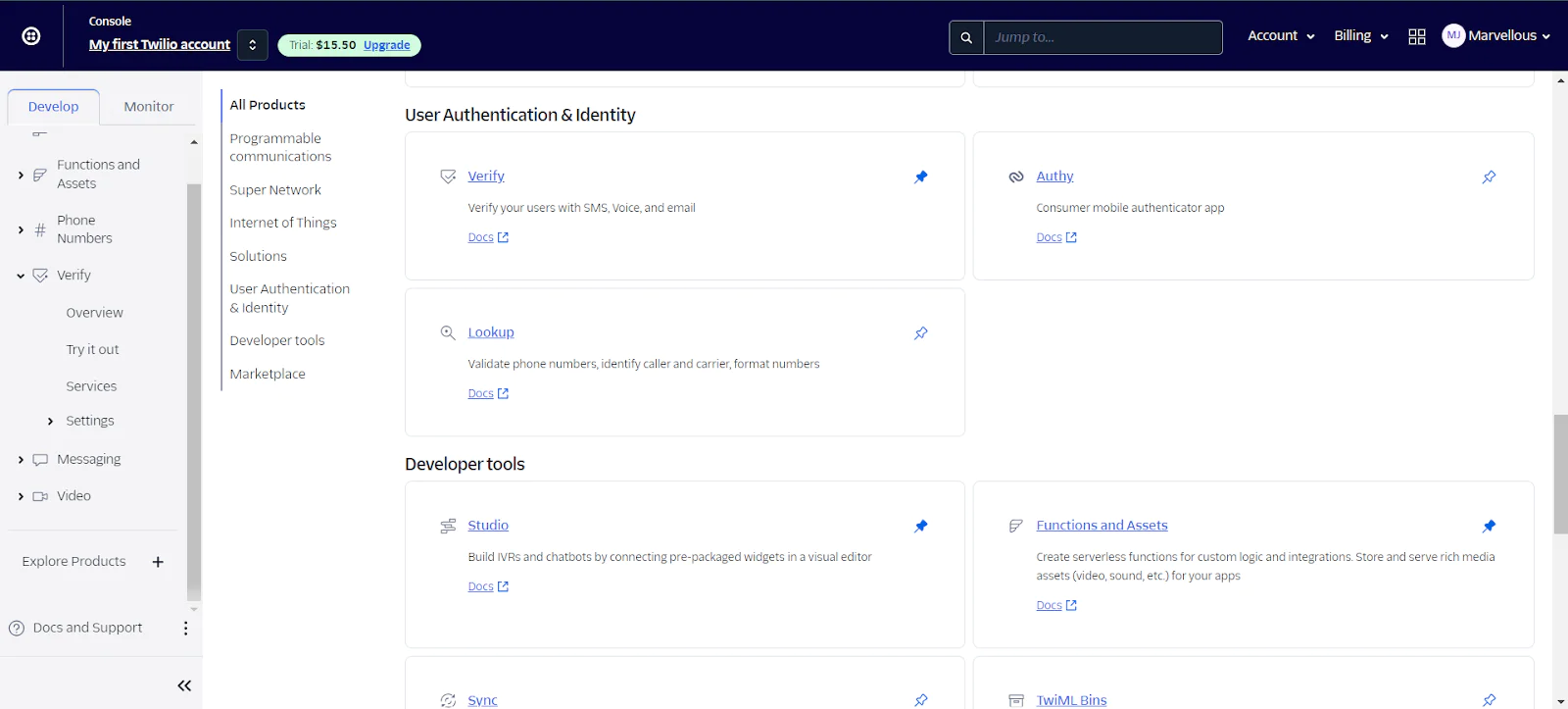
There, you should see a list of the Twilio Verify applications, if you’ve created any in the past. As a first-time user, a demo application is also created for you by default. Select this demo application (or create a new one) and then copy the Service SID and keep it somewhere as well.
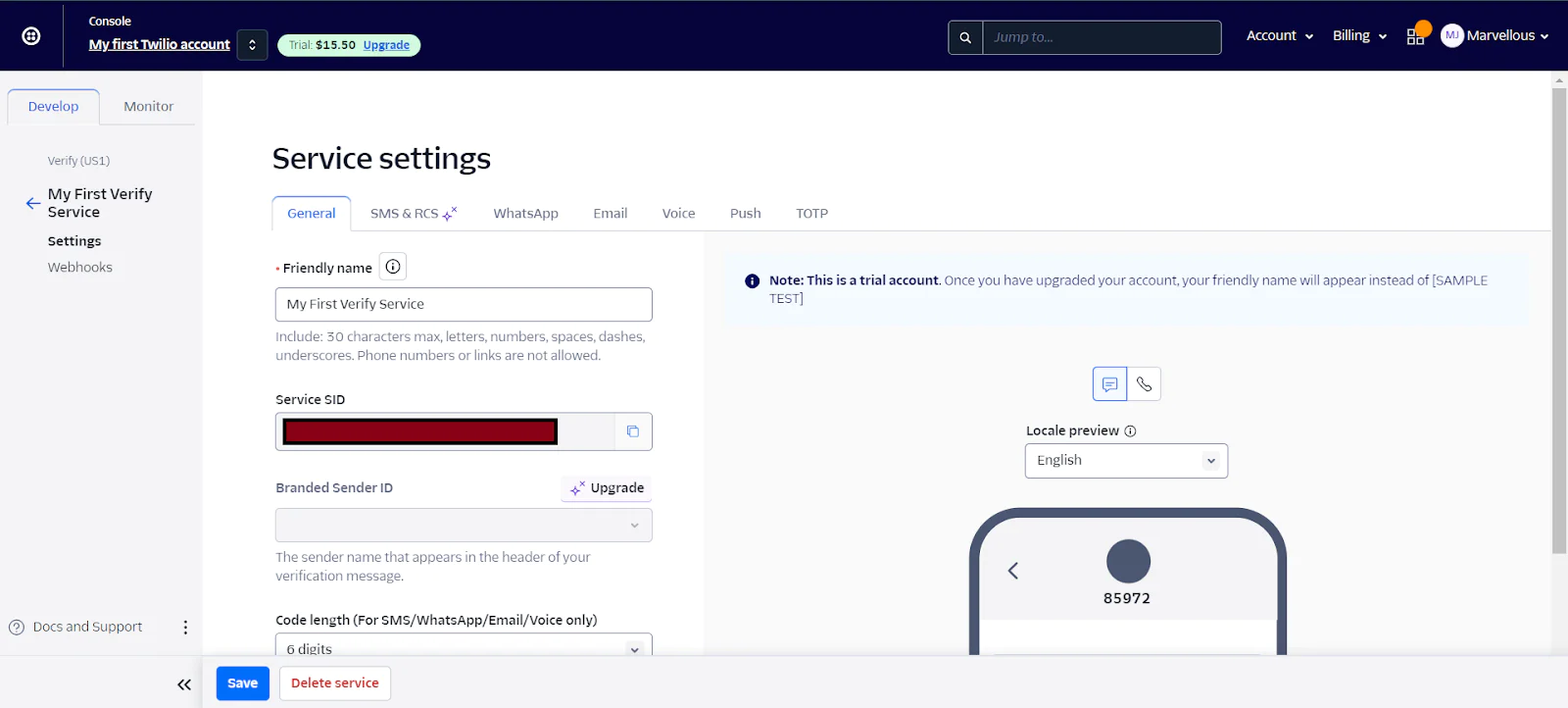
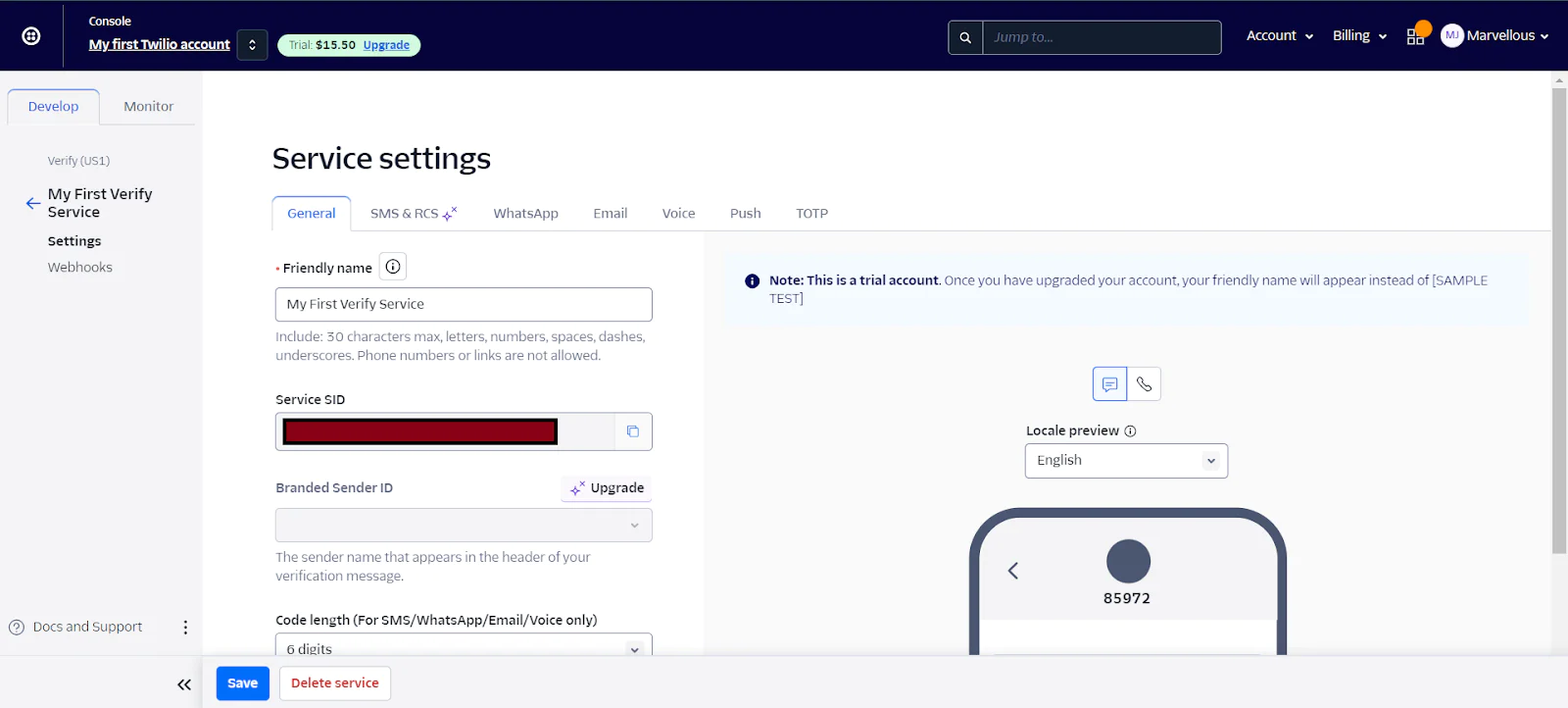
Implement 2FA with Twilio Verify and Gi-Gonic
Let's proceed with creating a new Go application, by running the following command:
This command sets up a new Go module for us. Next, we’ll need to install Twilio's Go Helper Library, gin-gonic, gorilla/sessions for session management, and godotenv to process environment variables, by running the following command.
Next, create a new .env file in your project root directory and update it with the Twilio credentials you retrieved in the previous step, as shown below.
With this basic setup complete, you can proceed with creating the application.
Create HTML templates
Let’s start with creating the static HTML pages for the app. In your project's root directory, create a new folder called templates and create three new files inside it:
- signin.html: to display a form for the user to complete the first level of authentication.
- enter-code.html: to display another form for the user to enter the OTP code sent to them.
- success.html : a success page that welcomes the user after they've completed the authentication process.
Inside the templates/signin.html page, paste the code below.
The code above creates a basic sign-in form that let’s users enter their username and password, and sends the form request to /signin
using the POST method.
Then, in enter-code.html paste the following code:
The code above is the markup for the page where users will enter the OTP code sent to them by Twilio Verify. In addition to the code verification form, we also receive a message from the server and display it on this page. This message is set to display a custom message indicating the phone number that the code was sent to.
Finally, in success.html, paste the code below.
This code displays the user's username along with a friendly welcome message.
Now, let’s proceed with registering the template directory as well as creating the necessary routes with Gin-Gonic. To simplify things, we’ll be mocking an array of users instead of using a database.
Create static users and routes
To proceed, create a new main.go file in your project root directory and paste the following code inside it.
The code above imports the necessary packages. It then defines the required Twilio credentials and creates two static users to mock a database. Here, ensure that the phone number added for one of the users is the same as your verified Twilio phone number, if you're using a trial account.
Next, we register the template directory we created earlier, and render the signin.html template for requests to the default (/
) route. Furthermore, we also register a new POST /signin
route, which is triggered when users submit the initial sign-in form. Handled by a new function named sendVerificationCode()
, it checks if they are one of the users defined earlier, stores necessary details in session, and sends them an OTP code.
Following the route definition, let's define the sendVerificationCode()
by pasting the code below at the end of main.go:
This code uses Twilio's Go Helper Library package to send the OTP to the user's phone number via SMS.
With this setup complete, once users sign in successfully, they should be redirected to enter-code.html to enter their OTP code.
Verify the 2FA code and redirect the user to the welcome route
The code entered by the user in the form in enter-code.html is sent to the verify-code
route. So, we need to define this route as well. Update your previous routes definition in the main()
function in main.go to include the two below.
The code above handles POST requests sent to /verify-code
and GET requests sent to /success
. For the /success
endpoint, it retrieves the signed-in user’s username and assigns it to the success.html template, displaying a custom welcome message with their username.
For /verify-code
, it checks the user’s session data to see if they’re a valid user, and calls verifyCodeWithTwilio()
to verify the code which they provide. So, next, add the verifyCodeWithTwilio()
function, below to main.go:
With all of these updates, your main.go file should be the same as the entire content in this GitHub Gist. The entire code for this tutorial is also hosted here.
Test the app
Now, let’s start the application with the following command:
Open http://localhost:8080 in your preferred browser and you should see the sign-in page as shown below.
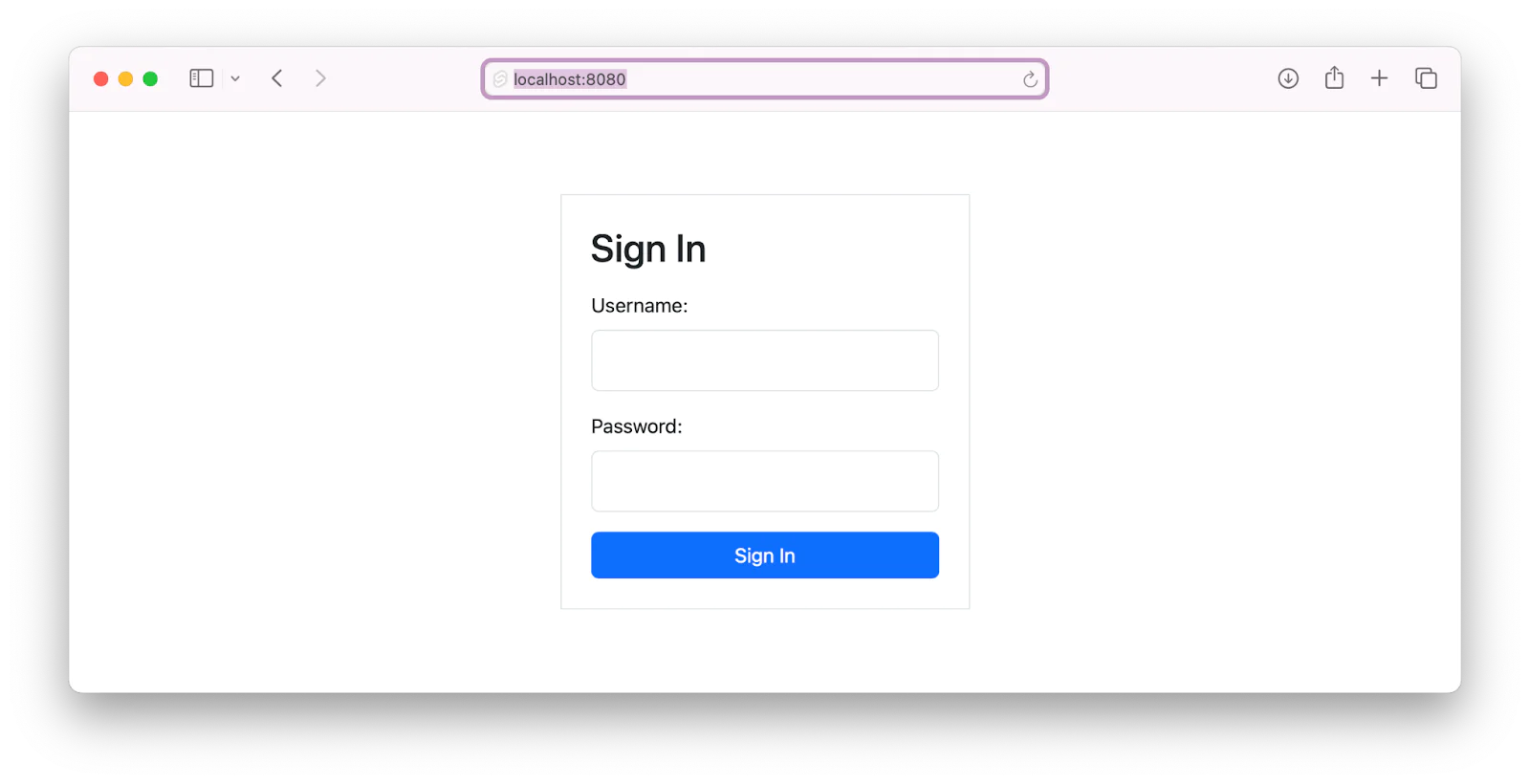
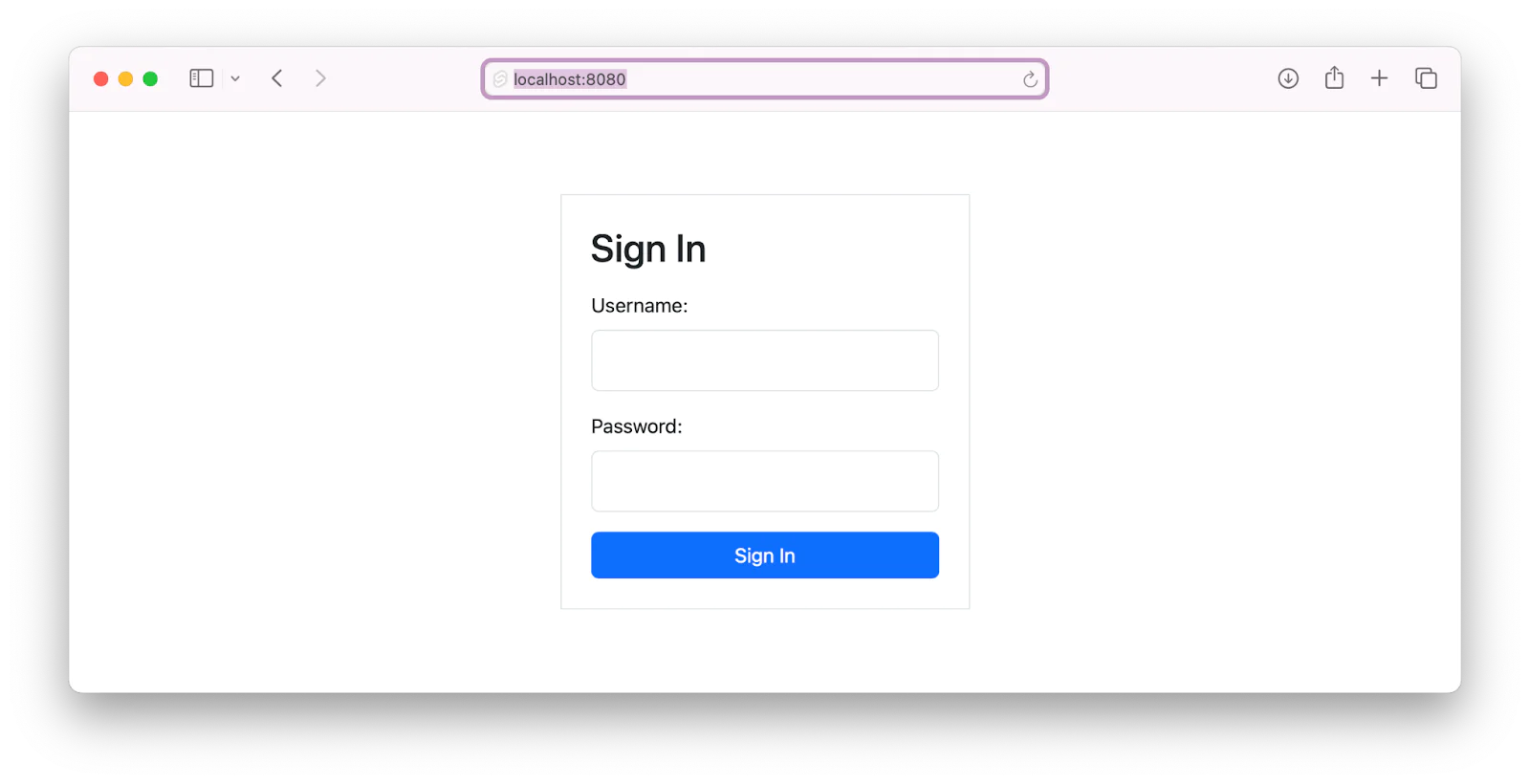
Enter a valid username and password, choosing from the ones we defined earlier, click Sign in, and an OTP should be sent to the mobile number you added for the user. You will also be redirected to a page to enter the OTP as shown below.
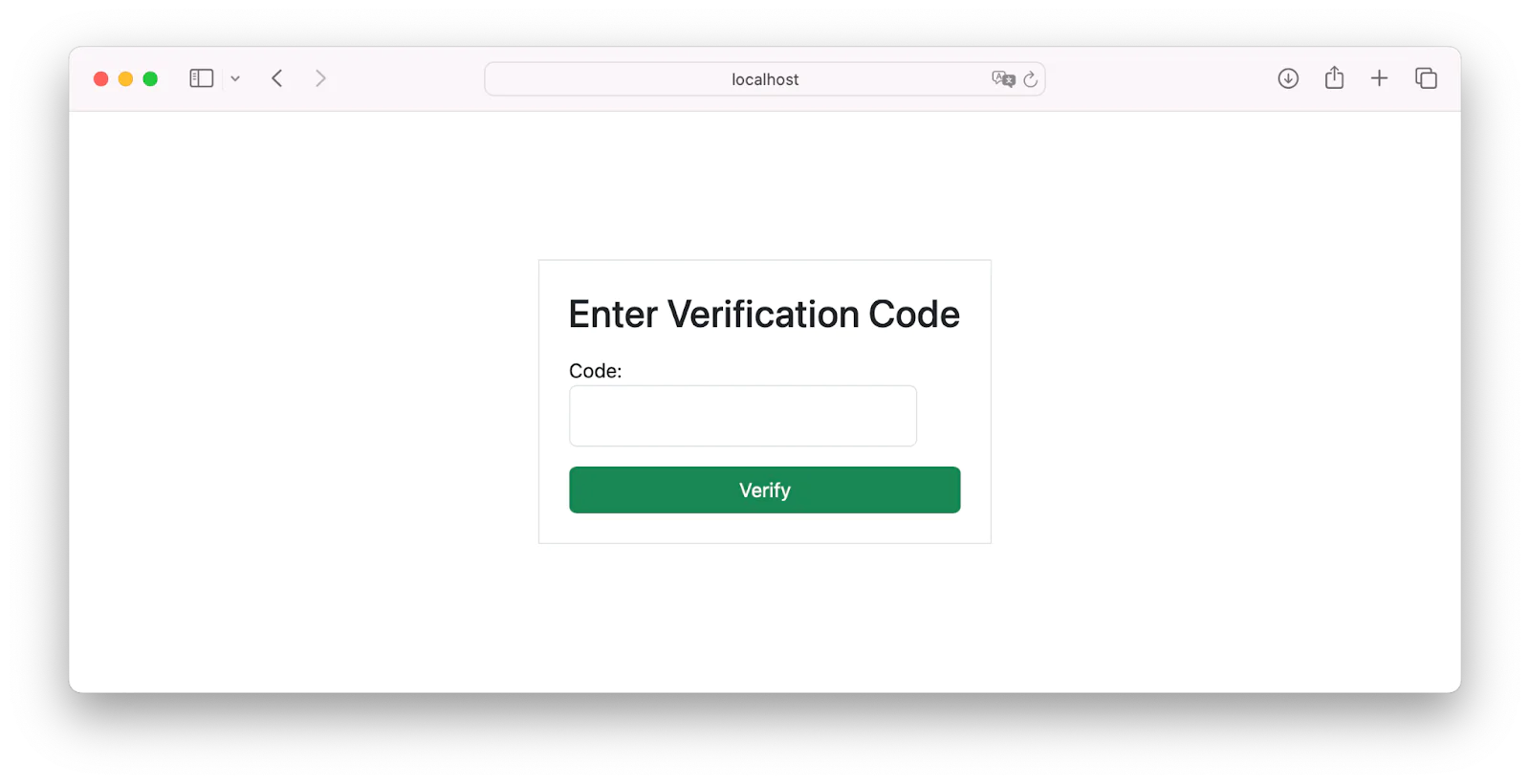
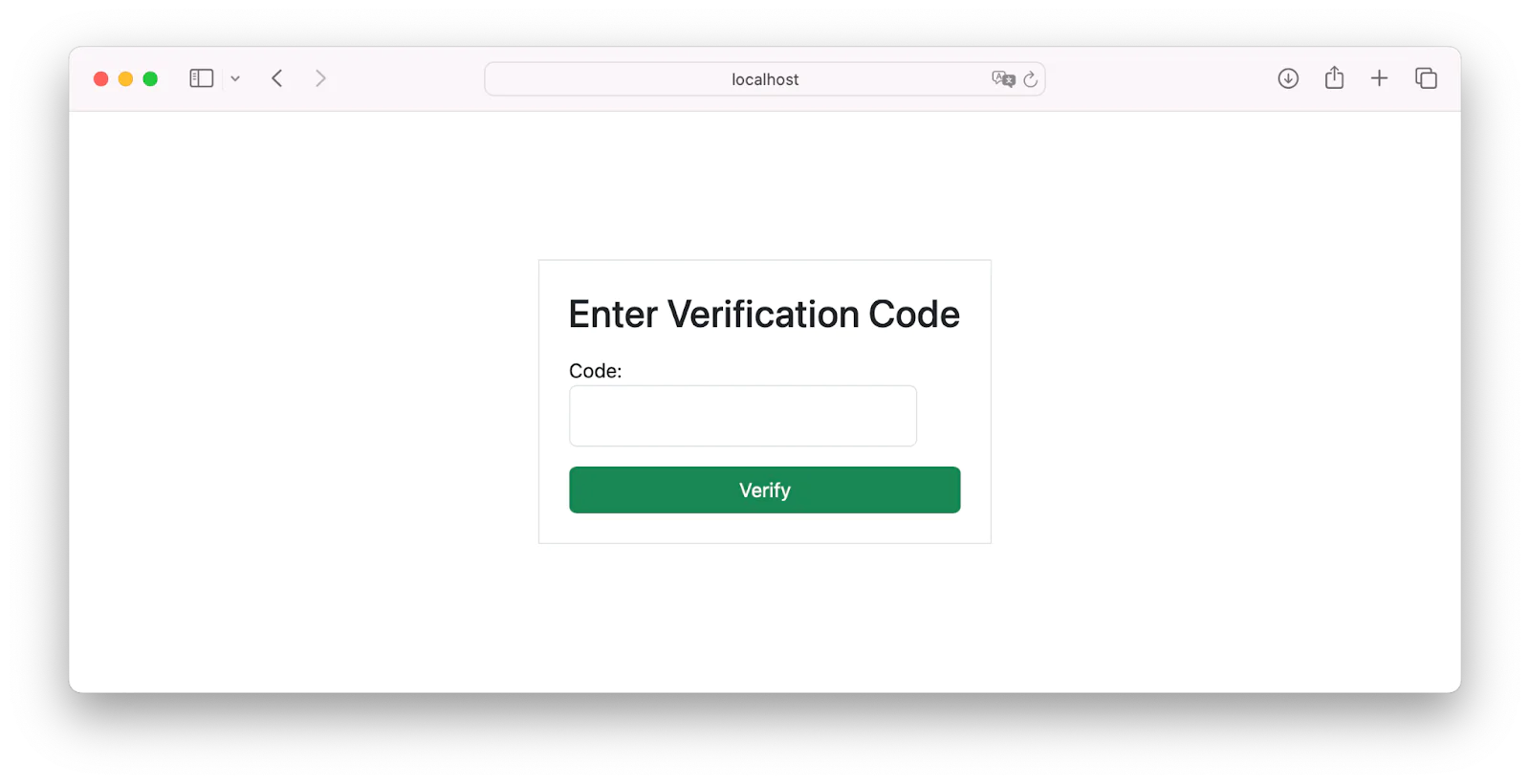
Enter the OTP sent to your mobile number and click Verify. You should then be redirected to the success page.
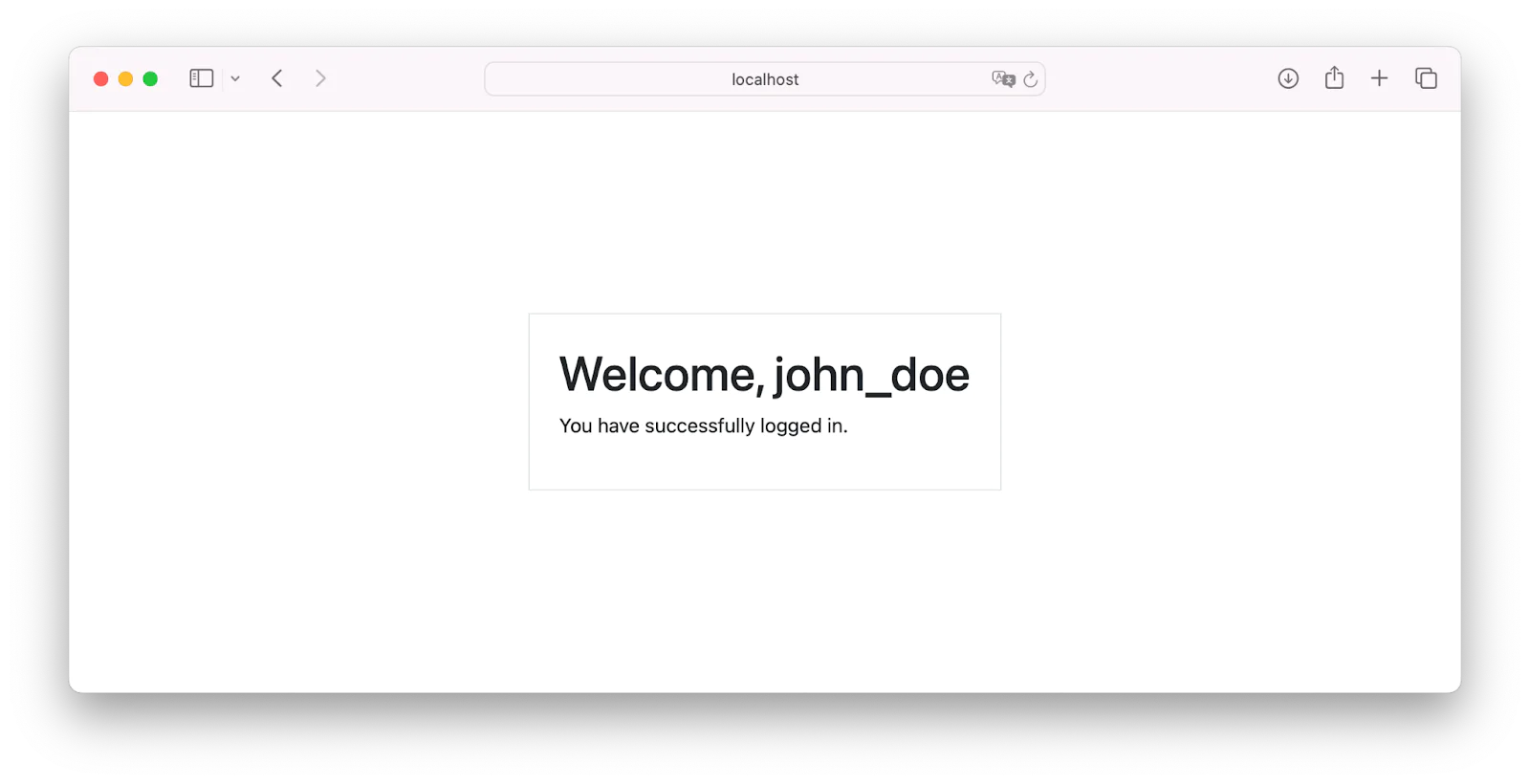
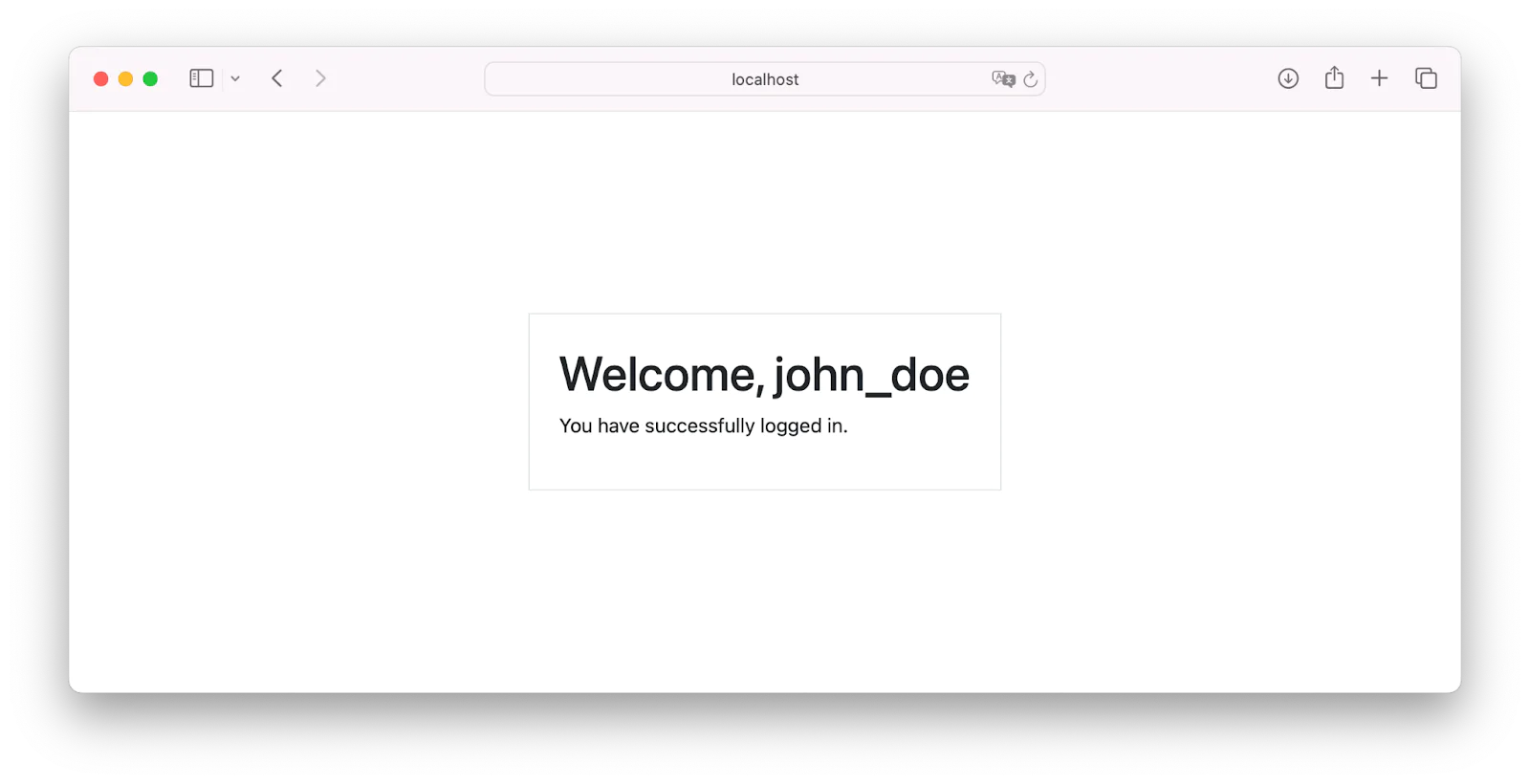
Conclusion
In this article, we looked at how to add two-factor authentication to Go apps using the Gin-Gonic framework and Twilio Verify. By adding a second layer of verification, we recognized how important two-factor authentication (2FA) is to improving online application security.
We also learned how to use the Gin-Gonic framework to integrate Twilio's Verify Service into our Go application, which sped up the development process.
I'm Jesuleye Marvellous Oreoluwa, a software engineer who is passionate about teaching technology and enjoys making music in my own time.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.