100% Protection from SMS pumping fraud
with Verify
Verify is a fully managed API for multichannel user verification. And it now includes guaranteed protection from SMS pumping fraud with Fraud Guard. Terms apply.
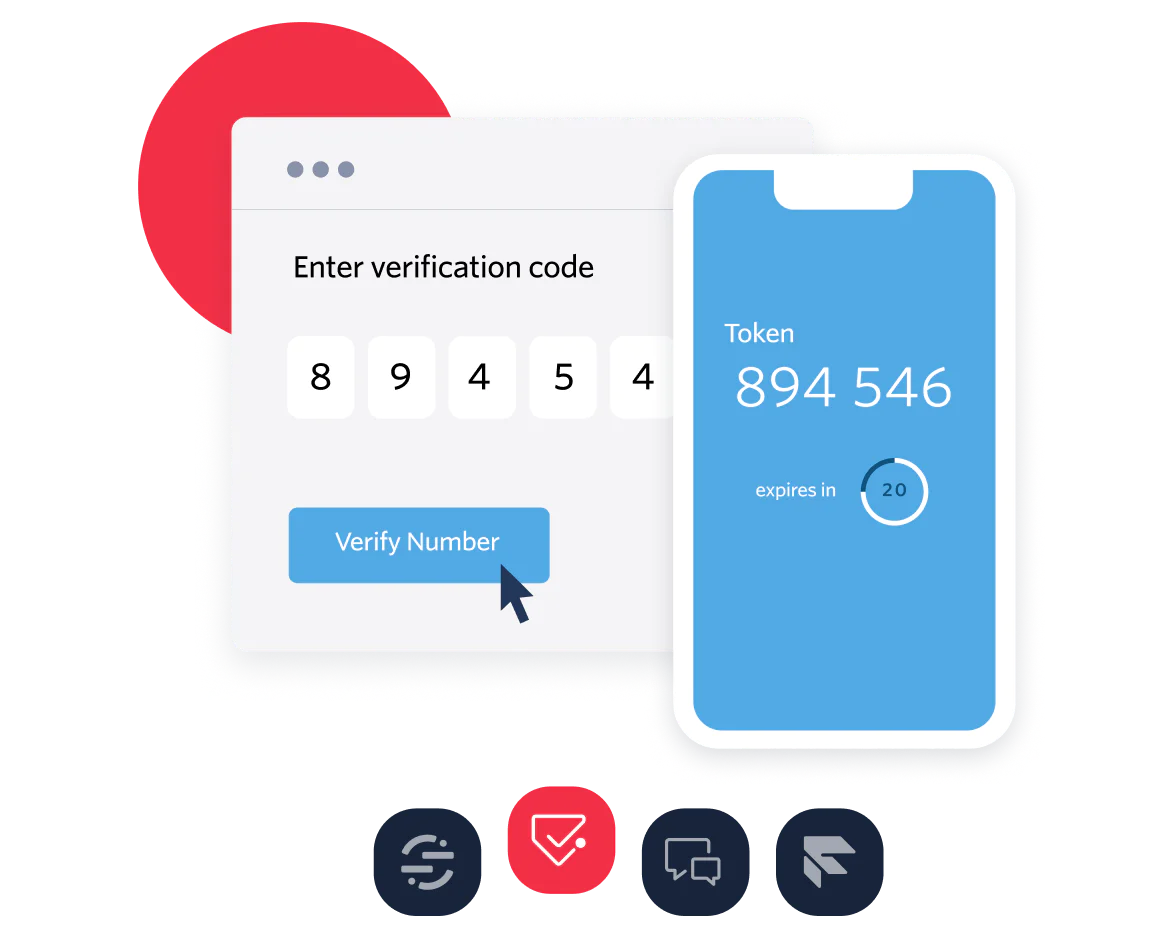
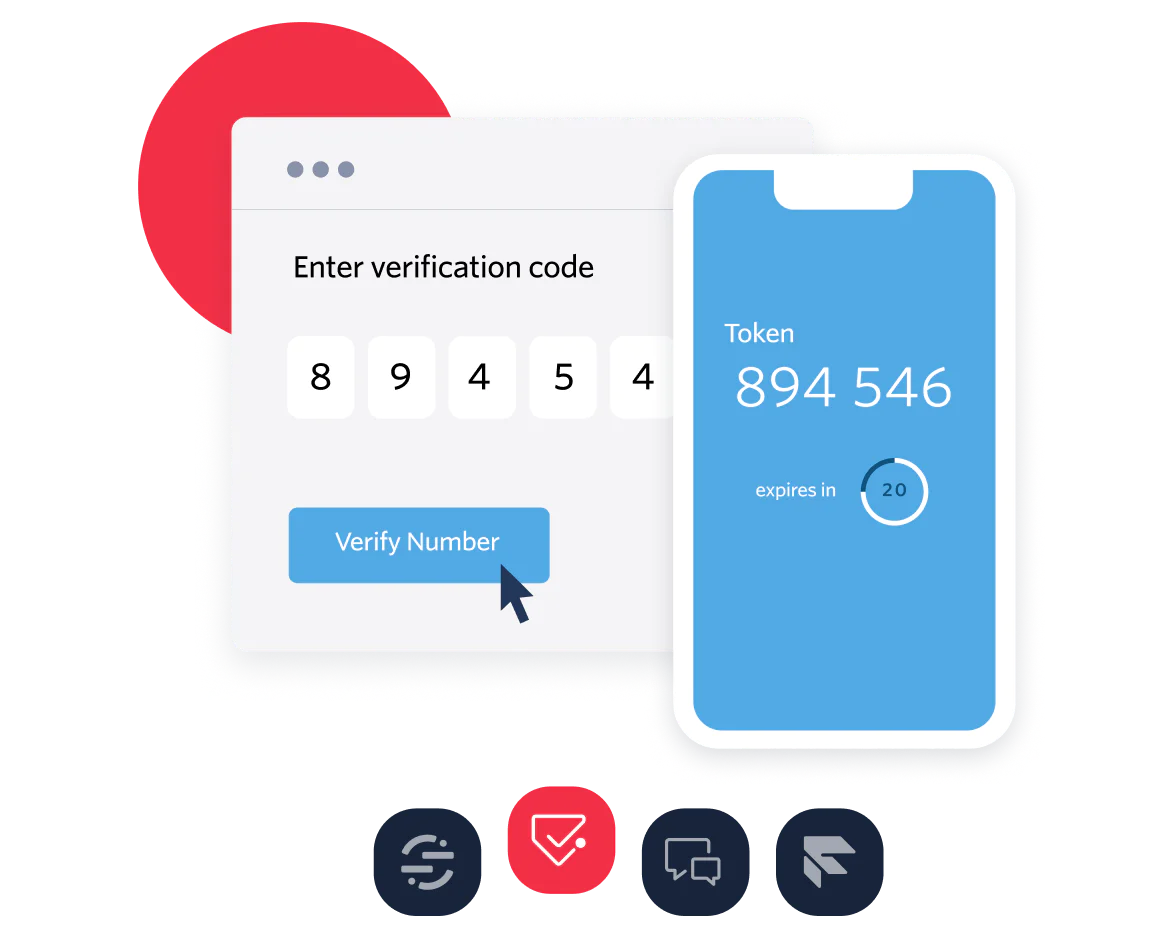
Verify is a fully managed API for multichannel user verification. And it now includes guaranteed protection from SMS pumping fraud with Fraud Guard. Terms apply.
Verify is a turnkey API for user verification. Add two-factor authentication across channels like SMS, email, WhatsApp, and TOTP or implement frictionless verification with Silent Network Authentication (SNA).
Quickly integrate a one-time password authentication (OTP) solution that handles your connectivity, channels, code generation, fraud monitoring, and prevention.
Trusted user verification with a delightful user experience.
Global user verification is hard—Verify makes it easy.
Fraud Guard has already saved customers over $55.8 million by blocking over 489 million* fraud attempts. With first-to-market innovation, Fraud Guard offers a 100% guarantee against SMS pumping fraud.
Phone number management
Carrier-approved templates
Actionable insight
Silent Network Authentication
PII-less, HIPAA-certified, SOC 2 Type 2
Push authentication
Route optimization
Rate limiting
Multiple delivery channels
Global reach
Integrate Verify into your app in as little as one sprint with its developer-first APIs and client libraries for a quick and confident deployment.
Bring your ideal solution to life with technology partners and top-tier consulting partners like Deloitte Digital, Perficient, and more. View partners
A simple verification solution to cut operational costs and increase OTP conversions.
Sign up for a free trial to get started. Then move to a pay-as-you go plan where you only pay for each successful verification.
* since June '22 up to 11 July '24
** Curve results with Twilio
*** Twilio 2023 internal data