Instant Lead Alerts with Node.js and Express
Time to read: 2 minutes
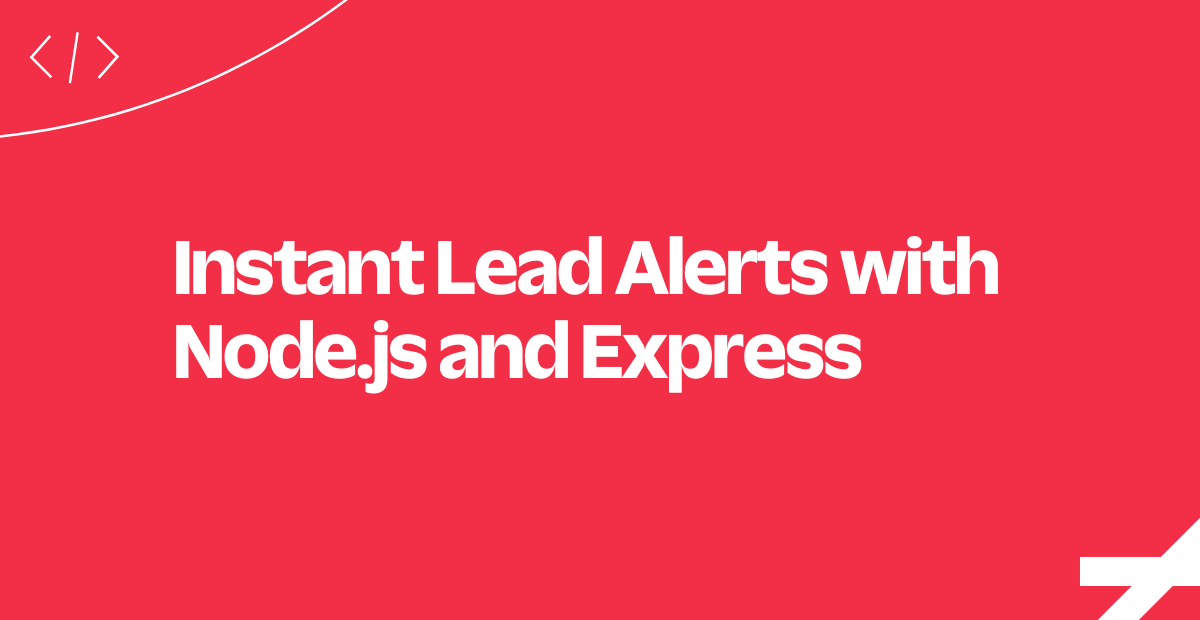
You probably already have landing pages or product detail views which you're using to generate some excellent leads for your business. Would you like to let the sales team know when you've got a new qualified lead?
In this tutorial, we'll use Twilio Programmable SMS in a Node.js and Express application to send a message when a new lead is found.
In this example, we'll be implementing instant lead alerts for a fictional real estate agency.
We'll create a landing page for a new house on the market and notify a real estate agent the moment a potential customer requests information.
Populate Landing Page Data
To display a landing page for our house we first need some information we'd like to display for the user.
For demonstration purposes we've hard-coded an object containing the information we need.
Next, let's look at how our landing page will be rendered.
Render the Landing Page
In our Jade template we insert data about the house.
We'll also include a form in the sidebar which will let a user enter their contact information when they request more information.
Now that our Landing Page is ready, let's setup our Twilio Client to send messages to the sales team whenever a new lead comes in.
Creating a Twilio REST API Client
Now we need to create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
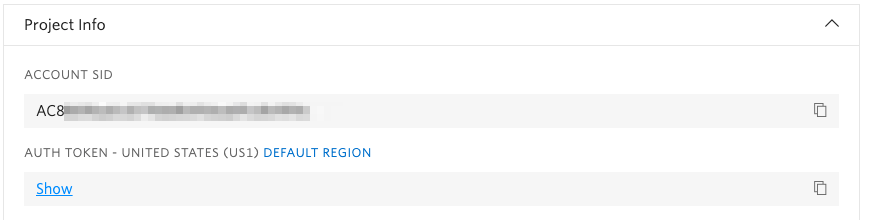
Our Twilio Client is now ready to send messages. Next, let's see how we will handle an incoming lead.
Handling the POST Request
This code handles the HTTP POST
request issued by our landing page.
Here we use the Twilio REST API Client to send an SMS message to the real estate agent's phone number, which is stored in an environment variable. We include the lead's name, phone number, and inquiry directly in the body of the text message sent to the agent.
Now the agent has all the information they need to follow up on the lead.
That's a wrap! We've just implemented an application to instantly route leads to salespeople using text messages.
Itching to build more? We'll look at some other use cases in the next pane.
Where to Next?
Node and Twilio - a great combination. Here are a couple other times we teamed them up for great results:
Learn to implement appointment reminders on your web app with Twilio, and avoid some of those no-shows.
Two-Factor Authentication with Authy
Learn to implement two-factor authentication (2FA) on your web app with Twilio-powered Authy.
Did this help?
Thanks for checking out this tutorial! Tweet @twilio to let us know what you're building.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.