Automated Survey with C# and ASP.NET MVC
Time to read: 3 minutes
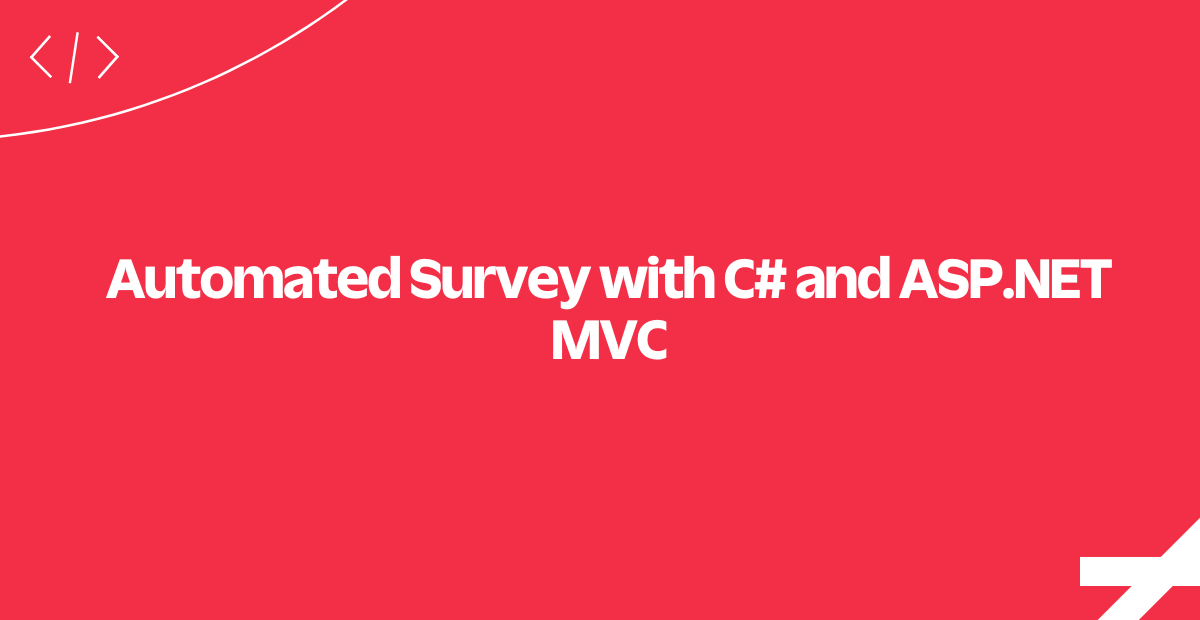
This ASP.NET MVC sample application demonstrates the use of the Twilio C# helper library and TwiML to deliver a survey to be completed via voice call.
In this tutorial, we'll walk you through the code necessary to power our automated survey. To run this sample app yourself, download the code and follow the instructions on GitHub.
Building an automated survey can seem like a daunting project to take on. With the help of the Twilio C# helper library, we will do this in a few simple steps! Click the button below to get started.
Configuring the Twilio Phone Number
To receive incoming calls, we need to setup our Twilio phone number.
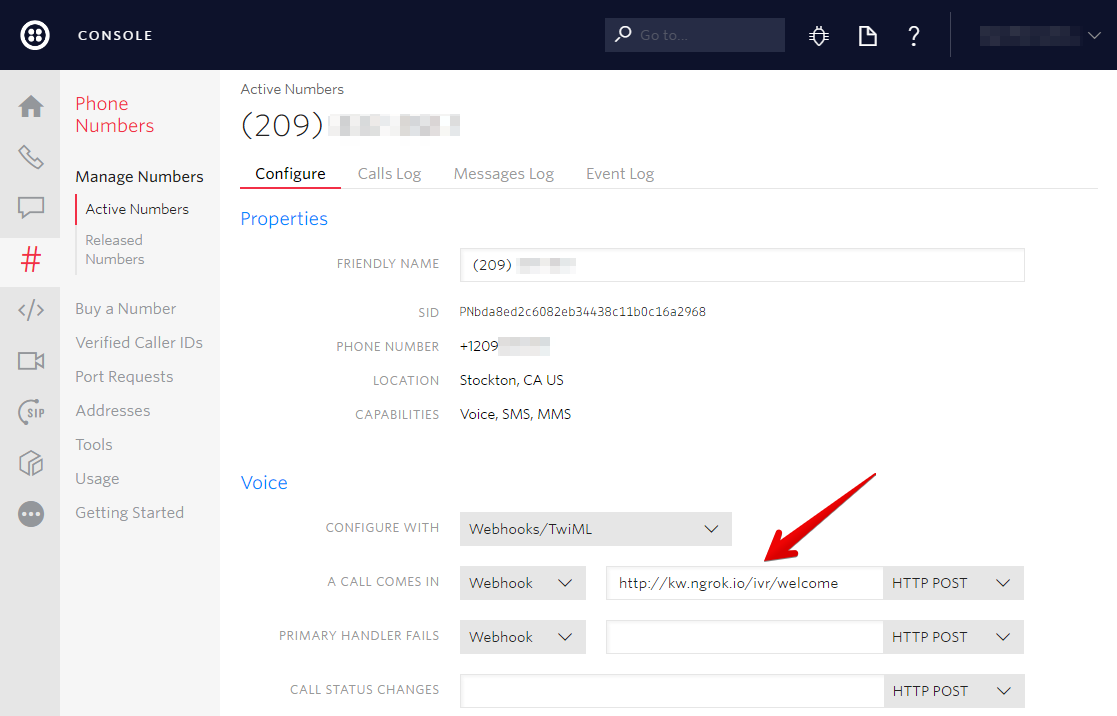
As you can see, the Twilio Console makes it easy to setup your webhooks for handling SMS messages and phone calls for your phone numbers. Setting up your Twilio phone number is a critical step for letting Twilio know where to forward messages and calls. If you don't already have a running server for this project, you can test your webhooks locally using ngrok.
Up next, we will dynamically create a survey from a series of questions stored in a database.
Creating a Survey
The questions are created using the Configuration.Seed
method. The advantage of this approach is that the data is populated when you run Update-Database
.
Now you know how to seed your database with the first survey. Next, we need to generate the TwiML for the initial response sent to the user.
Responding to Twilio's Initial Request
Whenever one of your Twilio phone numbers receives a call, Twilio will forward the request to the appropriate webhook.
For this application Twilio should be configured to make a GET request to the application’s surveys/connectcall
endpoint. Here the application finds the last created survey. Then, it uses the <Say>
verb to speak the welcome message, and the <Redirect>
verb to redirect to the first question in the survey.
Now you've seen how to handle incoming calls and generate the TwiML necessary to redirect the user to the first question. In the next section, we will see how to build a voice response and gather user input at the same time.
Asking the Caller a Question
At this point, Twilio has made a request for the first question. We’re using the Twilio C# helper library to generate a TwiML response.
After using the verb <Say>
to ask the user a question, we use either the verb <Gather>
or the verb <Record>
to collect an answer, depending on what type of question your survey will ask.
Next, we will explore how to gather diverse types of information such as numbers and voice recordings.
Ask different types of questions
If we want a number or boolean (yes/no) response from the user, we use the <Gather>
verb. For a free-form speech response, we use <Record>
to collect an answer.
Both TwiML tags have an action
attribute and a method
attribute. Twilio will use both attributes to make another HTTP request to our application with the user's answer.
More specifically, the action attribute will be set to POST to an action method named answers/create
which will be used to save the user's answer.
Now you can generate the TwiML response that will ask your question, as well as set the correct method to gather input that will best serve your analysis. However, we still need to store all of the information gathered in the various steps of the survey for use later on.
Record the caller’s response
When the callers have finished entering their responses, Twilio will make a request to this controller with all the call parameters we will need. For this sample application, we will store either the RecordingUrl
for free-form voice responses, or parse Digits
for number and boolean responses. We'll also track the CallSid
so we can track answers for a particular survey response.
Most surveys include more than a single question so we must now redirect the user to the next question in this survey until the survey is complete. Once the survey is complete, you'll probably want an easy way to look at the survey results.
Display the survey results
For this route we simply query the database and display the information using a Razor template.
You can access this page in the application at /surveys/results
.
As you saw, building an application using the Twilio C# helper library was very straightforward. We hope you found this sample application useful.
Where to Next?
If you are a C# developer working with Twilio, you might want to check out other tutorials:
Employee Directory with C# and ASP.NET MVC
Use Twilio to accept SMS messages and turn them into queries against a SQL database.
IVR: Phone Tree with C# and ASP.NET MVC
IVRs (interactive voice responses) are automated phone systems that can facilitate communication between callers and businesses.
Did this help?
Thanks for checking this tutorial out! If you have any feedback to share with us, we'd love to hear it. Reach out to us on Twitter and let us know what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.