Asynchronous JavaScript: Tools, Techniques, and Case Studies
Time to read: 3 minutes
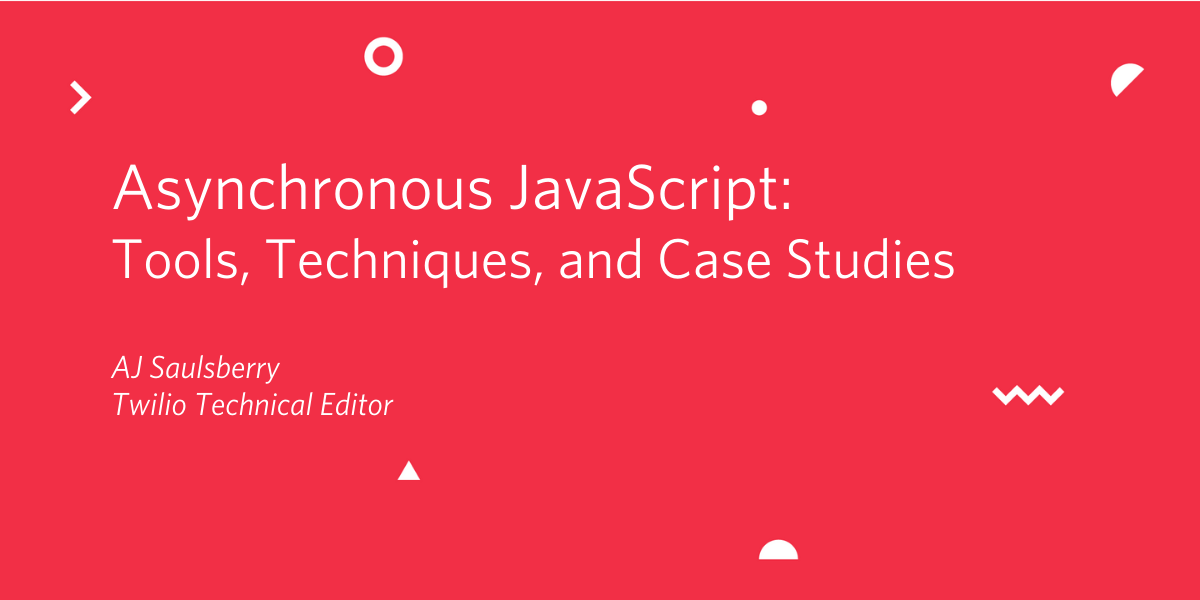
There are a number of asynchronous tools in JavaScript, and there are also asynchronous tools in popular libraries that extend JavaScript. And there are many uses for these technologies.
You can learn about the fundamental asynchronous JavaScript tools in posts here on the Twilio blog: There is a post devoted to each technology, and they’re summarized in the post:
Asynchronous JavaScript: A Comprehensive Guide
When you’re learning any new programming tool or technique it’s helpful to have examples of how to use the code in realistic situations, so in addition to covering the fundamentals we have a series of posts showing practical demonstrations of how to use asynchronous code.
Confirming SMS Message Delivery with RxJS Observables, Node.js, and Twilio Programmable SMS
You’ll learn a couple of important things from this post: 1) how to create an Observable wrapper around an API request so your code gets a notification when the status of the request changes and 2) how to send SMS messages from Node.js with Twilio Programmable SMS. Together, these techniques enable you to verify the status of an SMS message in real time. To help you visualize the sequence of events the post includes sequence and interaction diagrams. It’s a fun project to try, and it gives you practical experience with RxJS Observables with code you can reuse in your projects.
COVID-19 Diversions: Tracking the ISS with Real-Time Event Notifications Using Node.js, RxJS Observables, and Twilio Programmable SMS
Here’s another fun programming exercise: getting automatic notifications when the International Space Station passes overhead. Sure, there’s an app for that, but it’s cool to build it yourself. You have more than enough experience installing apps; this post will give you more experience with Node.js, RxJS Observables, and Twilio Programmable SMS. This post gets into deeper detail on how you can use Observables to handle sequences of events and how to work with collections of Observables. You’ll also gain experience with Observable transformation operators, the file system, and REST APIs.
Using RxJS Observables With JavaScript Async and Await
Sometimes a simple demonstration is the easiest to understand and put to use on your own. This post shows you how to get data from an unreliable REST API and report the status of the attempt and the results. You’ll see how to use RxHR, one of the most widely used libraries for Node.js, to interact with a REST API. You’ll also learn how to convert RxJS Observables into JavaScript Promises so you can take advantage of async…await
to handle long running and sometimes failing actions like API calls. Best of all, you’ll see how you can do all this in 26 lines of code.
But wait; there’s more
These posts are closely related to the Asynchronous JavaScript series, but there are many other cool and interesting JavaScript tutorials available here on the Twilo blog. To peruse them, use this link to the blog’s convenient JavaScript tag.
Additional resources
Twilio account – If you sign up for a free Twilio account using this link you’ll receive an additional $10 credit when you upgrade. It will come in handy when you do some of these tutorials.
TwilioQuest – Defeat the forces of legacy systems by completing JavaScript missions in this role-playing game inspired by the 16-bit golden age. Learning can be fun!
If you’re looking for reference documentation on the topics covered in the posts mentioned here, check out the following canonical sources:
JavaScript | MDN – Part of the Mozilla Developer Network’s web docs, this is an essential source for JavaScript reference information and tutorials.
Draft ECMA-262 – Want to know what’s coming next in JavaScript? This is the official draft specification for the next version. Although called JavaScript, the language is officially ECMAScript.
Node.js Docs – Node.js provides a runtime environment for JavaScript so you can run JavaScript programs from your operating system’s console prompt. That’s pretty useful, so it’s a good idea to know something about it. If you want to be au currant, you might want to check out Deno, a new critter on the RTE landscape.
V8 – The JavaScript runtime environment used in most web browsers, including Chrome and Edge, is Google’s V8. In addition to ECMAScript, it also implements WebAssembly and runs on many flavors of operating system. It’s written in C++ and can be embedded in C++ applications for those who aren’t afraid to write unmanaged code.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.