How to Make a Clicker Game with Java
Time to read: 4 minutes
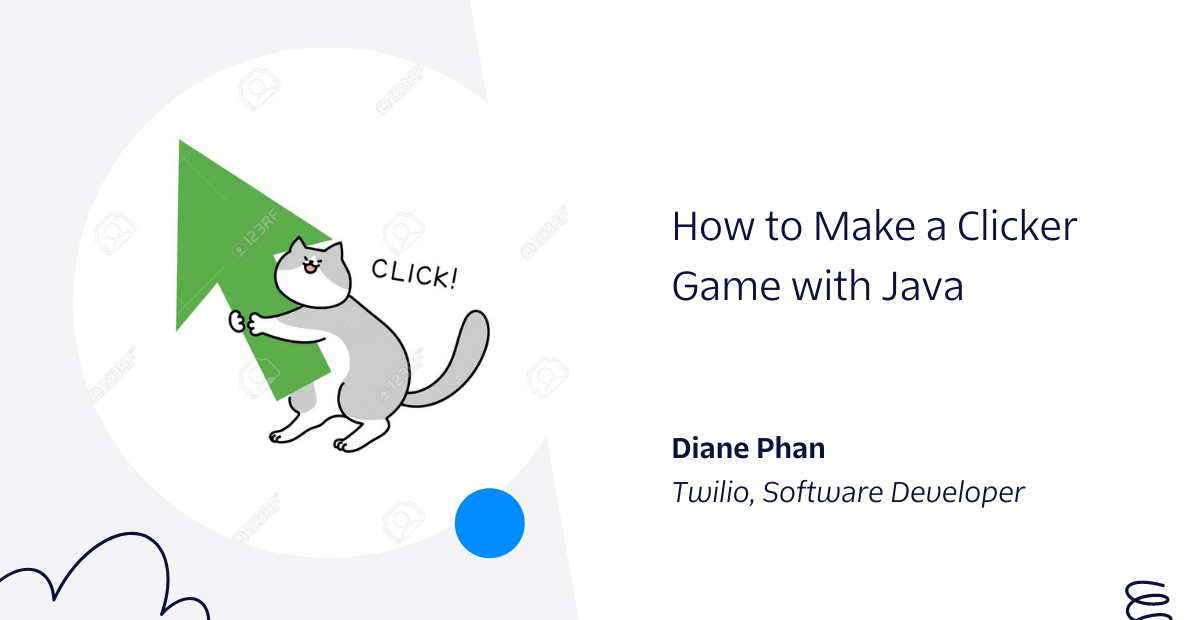
Making mini browser games can be a great way to learn how to code and understand how the internet works. It's also fun to just make your own version of pointless and addicting games such as Cookie Clicker.
In this article, you will learn how to make your own clicker game that runs on the Java Spring Boot application. The objective of the clicker game is to count the amount of clicks on a chosen image. In this particular example, we will allow the user to click on an image of a cat to give it some treats.
Tutorial requirements
- Some prior knowledge of Java or a willingness to learn.
- Some knowledge of JavaScript.
- Refer to this Twilio article to set up a Java Spring Boot application that can be hosted on the web.
Add the Thymeleaf dependency
Navigate to the pom.xml file to add the following dependency to the list of dependencies:
The Spring Boot Starter Thymeleaf is required to build web applications that serve HTML. This is essential for creating the web based clicker game.
Customize the web controller
After you’ve set up your application, navigate back to the WebController.java file and replace the contents with the following code:
The WebController
has a helloCat()
method that returns a string referencing the path name for the HTML template page that will be rendered.
Create the HTML page
The objective of this clicker game is to make a POST
request whenever the user clicks on the cat picture. Whenever the image is clicked, a counter should increment by one and display the counter dynamically. A text response in string format is then created to store the response value of how many times, or total
amount the image was clicked.
Go ahead and place a picture of a cat in the src/main/resources/static subfolder. The /static subfolder is a location for all static assets to be used and referenced throughout this project.
Find the src/main/resources/templates subfolder and create a new file named cat.html. This file will be rendered from the helloCat()
method in the web controller.
This will be a fun section for those who enjoy using JavaScript to make their websites interactive. This clicker game will be simple and only display an image that responds to user clicking events, but feel free to change it up as you wish.
Copy and paste the following code to the cat.html file:
Be sure to replace <FILE_NAME_FOR_CAT_IMAGE>
with the path name for the image you uploaded in the src/main/resources/static subfolder.
The image is given an id
so that it can be retrieved from the Document Object Model (DOM). The catpic
image is retrieved from the document
in the JavaScript portion so that an onclick
event function can be attached to the image.
Notice that total
is used to store the totalCount
element from the DOM, similar to the catpic
. This element is represented on the DOM as the <span>
text element and will be manipulated in JavaScript. This POST
request will be discussed further in the Controller later.
Here's an example of the expected HTML page at the end of the article:
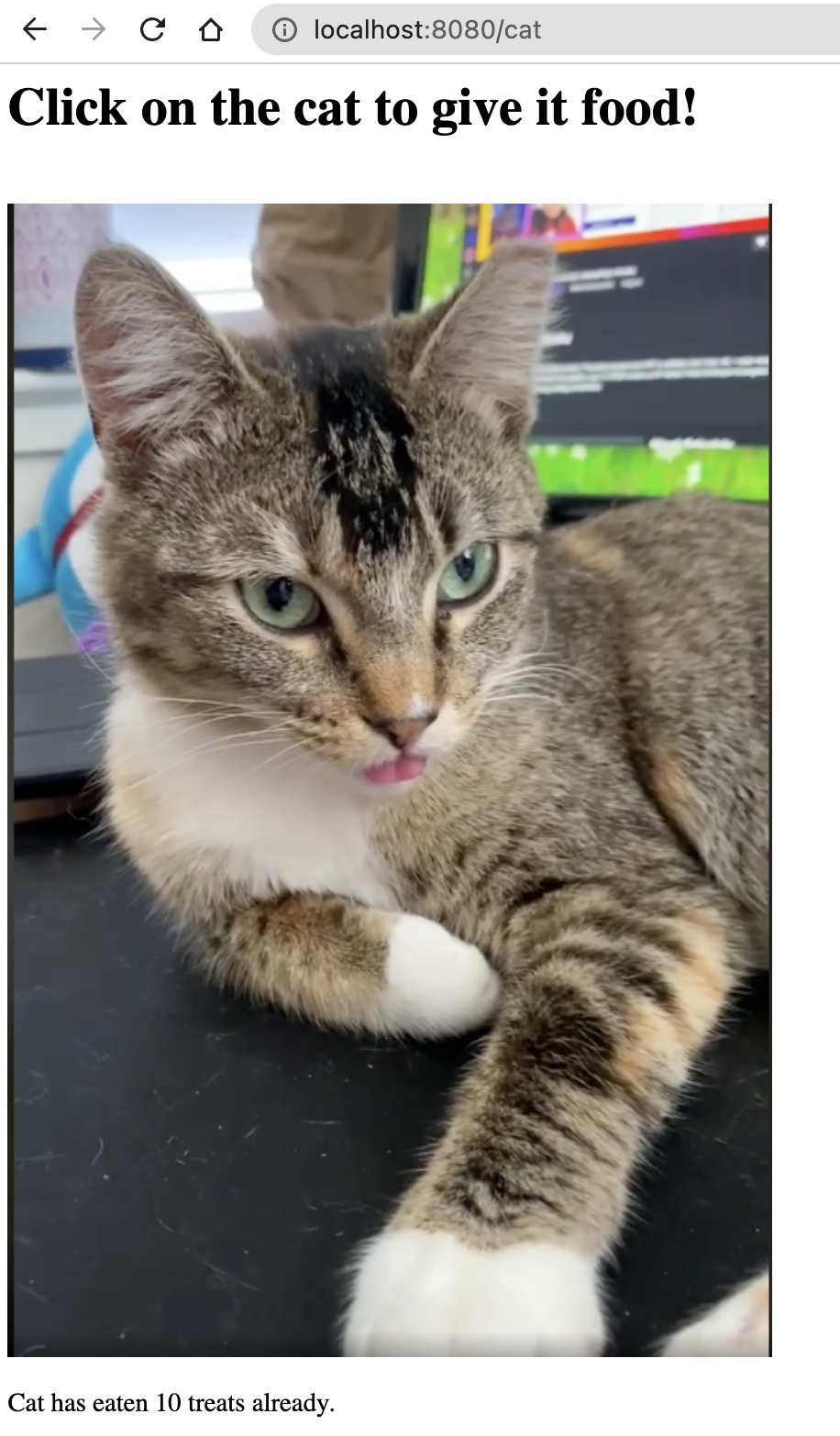
An optional addition to this clicker game is to have the document body display a total count once the page is freshly loaded for the user, such as when the URL is shared to another user or opened in a new tab. This requires the game application to make a GET
request and retrieve the last recorded counter before the user increments it by clicking.
Build the REST controller
As mentioned in the previous section, a few HTTP requests are necessary for the flow of this clicker game. This file is essential for serving data and REST ednpoints.
Create a file named CatRestController.java and copy and paste the following code:
The @RestController
annotation marks the request handler required to build and provide the RESTful web service during runtime.
The counter
variable is initialized at the beginning, which means that the total point cache resets once the application process is killed. If you want to expand on this project and save all the point increments overtime, consider adding a database to the project later on.
Since the POST
request is required to handle all increments of image clicks, a @PostMapping
annotation is created for a function clicker()
that returns a string with the updated click value. The return value is a concatenated string message sharing how much the "cat has eaten" and shows the updated clicker counter.
For the optional GET
request portion that displays when the page is loaded, a @GetMapping
annotation is created instead to define the returnClick()
function. The return value is similar and returns a string message along with the latest counter value.
An example for that optional portion is seen in the GIF below:
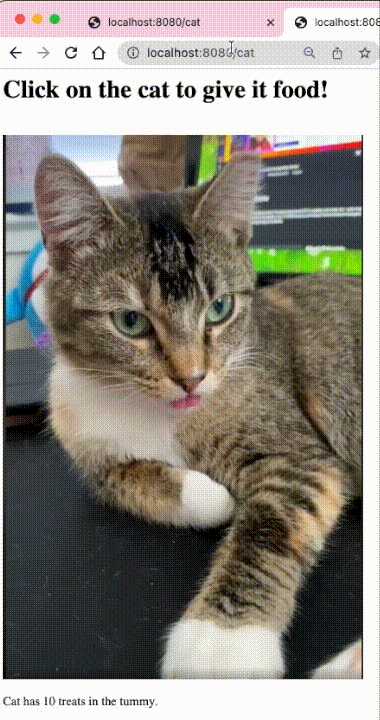
Notice how the counter started off with 10 treats already.
Play the clicker game
It's time to test out the game and give treats to the cat! Here is the GitHub repository for the full code.
If you need a reminder on how to run a Spring Boot application, refer to this article.
Let's give this cute cat some treats!
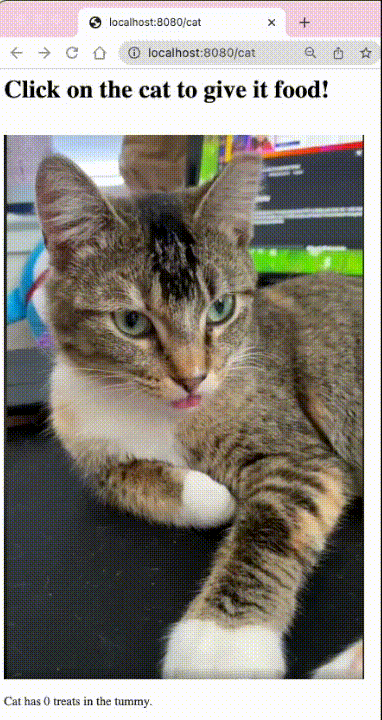
You can have some more fun by customizing your cursor for the web application so it looks like your cursor is a real treat.
What's next for building Java Spring Boot projects?
Congratulations on building your first clicker game!
Challenge yourself and expand on this project by storing all of the clicks in a database.
Explore some other neat Java Spring Boot projects.
- Organize a lunch order with Twilio SMS.
- Learn how to send an SMS with a click of a button.
- Make the button send a WhatsApp message using Java
Let me know what cat picture you used and how your game looks by reaching out to me over email.
Diane Phan is a software developer on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.