Convert Bitcoin to Local Currency using PHP
Time to read: 4 minutes
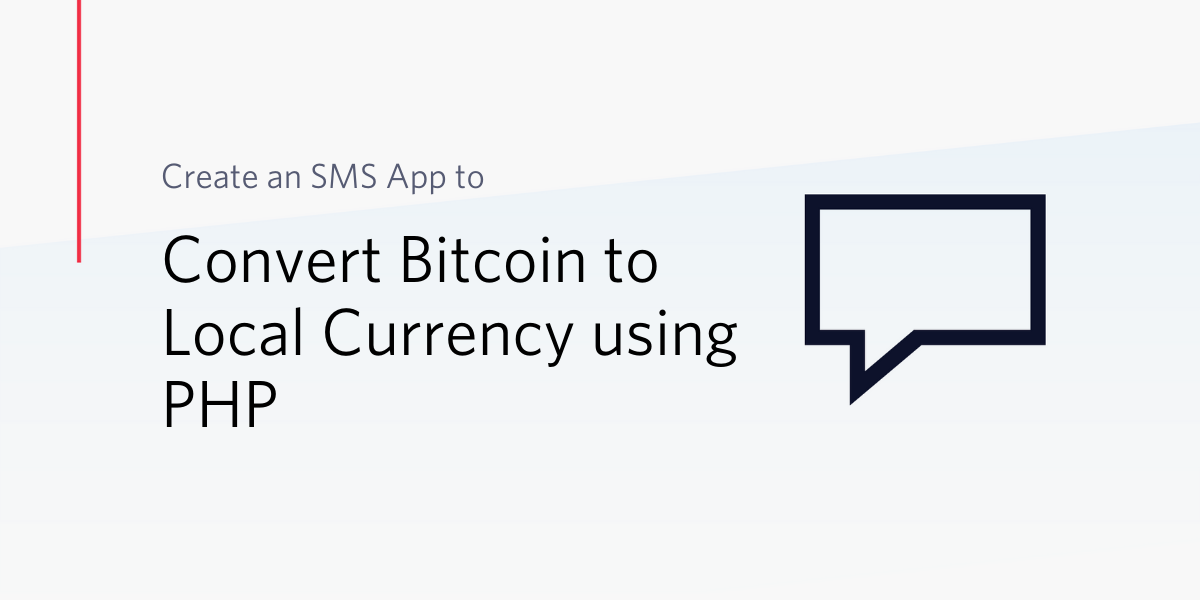
There are different methods of converting bitcoins to local currency and vice versa such as blockchain exchange sites and wallet apps, Today, this tutorial will walk you through creating your simple Bitcoin converter using SMS and PHP, allowing you to check current market rates on the go!
Technical Requirements
To complete this tutorial, you will need the following dependencies globally installed on your computer:
-
PHP 7 development environment
- Global installation of Composer
- Twilio Account
- Cryptocompare API
- Global installation of ngrok
Set Up Your Development Environment
To kick start our project, we will need to create a project directory for it. You may use cryptoconverter
as this is what I will be using. Create the following files in the project folder:
- .env
- webhook.php
- functions.php
Next, we need to set up our .env
file.
NOTE: The .env
file is a hidden file used to store secret and private keys on our servers. It is not accessible by the browser, so we can store our API keys or credentials in it and access it from anywhere within our app on the server-side.
To allow the support of .env
configs in our app, we need to install the dotenv package using Composer. We will also need to install the Guzzle package in order to easily make HTTPS requests from within our app. Run this command in your terminal inside of the project directory:
There are many libraries that enable us to access the Twilio API more efficiently than using the traditional cURL requests. For this project, we will install the Twilio PHP SDK. Run the following command in your terminal in the project directory.
Setup Twilio SMS Credentials & Phone Number
If you have not already done so, take a few minutes and head to your Twilio account to create a new number. We need to get the following information from the Twilio console to allow us to send and receive SMS from within our project:
- Twilio Account SID
- Twilio Auth Token
- A Phone Number
Once we have these items, we can add them to our .env
file.
By now you should have all of the necessary details to connect your app to Twilio. Now let’s have a short math class!
Understanding How the Conversion Works
To convert Bitcoin (BTC) to local currency and vice versa, we need to first understand how it works so we can write a function to automate this process and then enable SMS conversions.
Let’s take a look at the following example. To convert from USD to BTC, we need to get the USD to BTC global ticker price from the blockchain. At the time of writing this article, the conversion rate is $7111.57 USD.
Assuming we’re converting $10 USD, we will follow the following formula; $10 USD /$7111.57 USD, which will equal 0.0014062 BTC.
To convert BTC back to USD, just run the previous formula in reverse by multiplying.
E.g 0.0014062 BTC to USD will equal $10 because 0.0014061 x $7111.57 is 10.
Create a Function to Convert Bitcoin to Local Currency
Let’s create a simple helper function that processes the user’s SMS command e.g convert 1 BTC to USD
or convert 40 USD to BTC
.
Add the following code to your functions.php
file. This function will parse the user’s SMS command into a much more readable format that we can use for the conversion.
Now that we can process user’s commands, we need to create a function that does the conversion. Update the functions.php
file with the following code:
We also need to update our .env
file with the crypto exchange price source’s URL with the following:
The final functions.php
file should look like the one below:
Create a Twilio Webhook
We will need to create a Twilio webhook in order to receive SMS conversion requests from users. Twilio has a webhook feature that sends a response to an endpoint whenever your Twilio phone number receives a message.
In the webhook.php
file, copy and paste the following code to create our own webhook that receives conversion requests
We will now use ngrok to make this webhook accessible through a public URL from our localhost.
From the terminal, run the command:
On a new terminal, run the command:
Your terminal window should look like this:
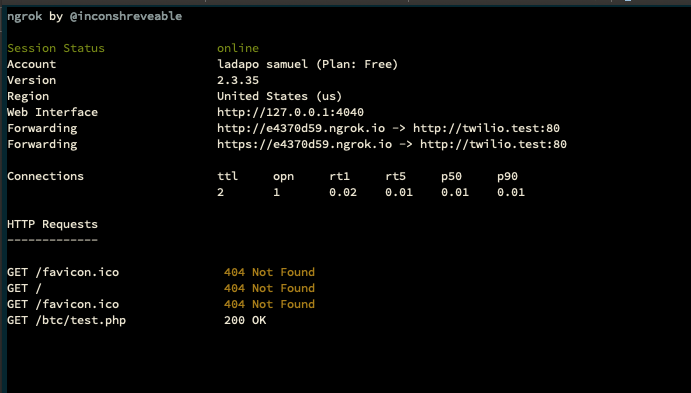
The Forwarding URL combined with the webhook.php
endpoint will be used as the webhook URL. For example, mine is:
The final step is hooking our Twilio number to the webhook we created. Navigate to the active numbers on your dashboard and click on the number you would like to use.
In the messaging section, there is an input box labeled “A MESSAGE COMES IN”. Replace the URL with our webhook URL and click “Save”!
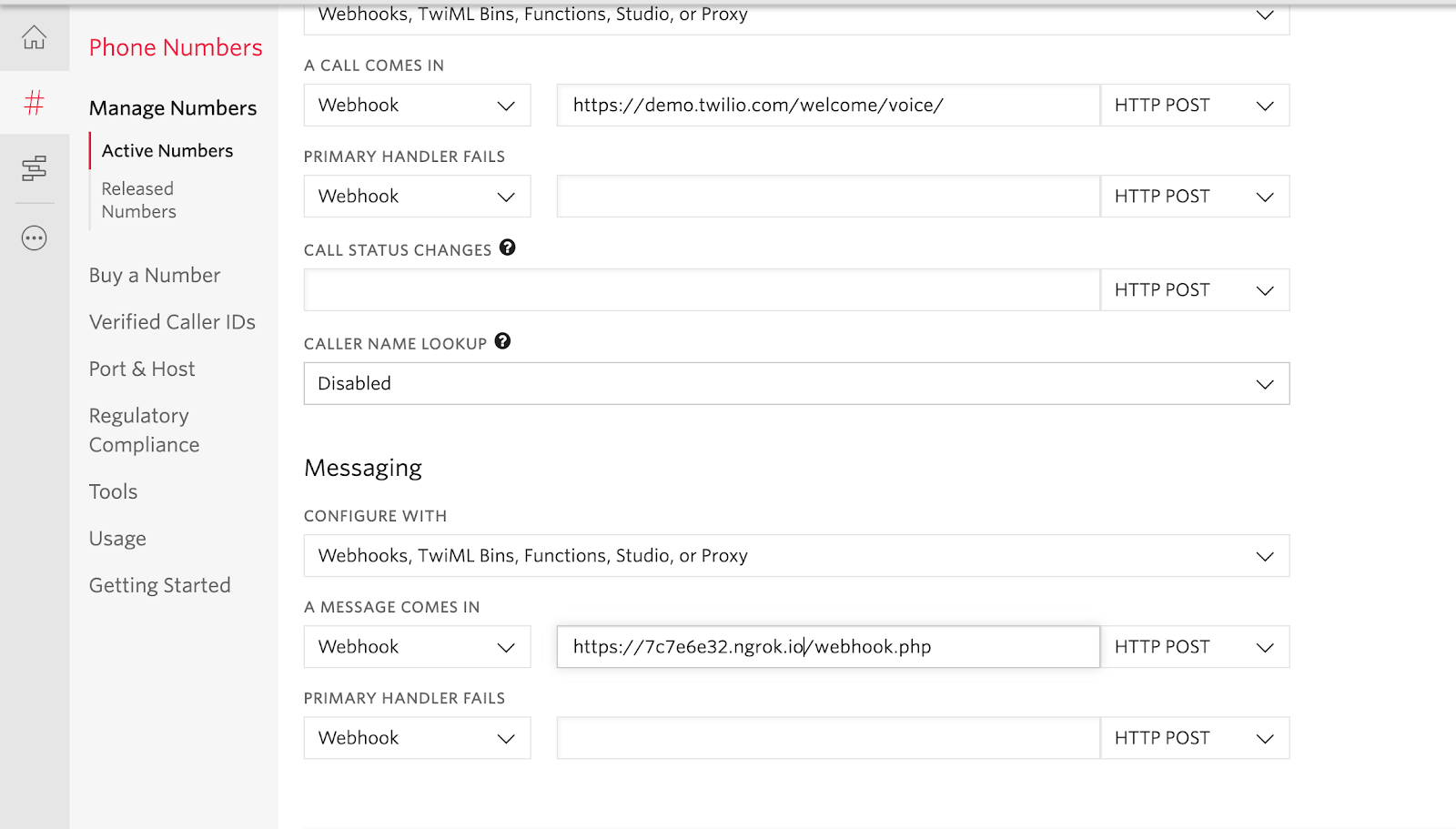
Testing Our App
Let’s test the application! Send convert 1 BTC to USD
to your Twilio number to receive the converted results.
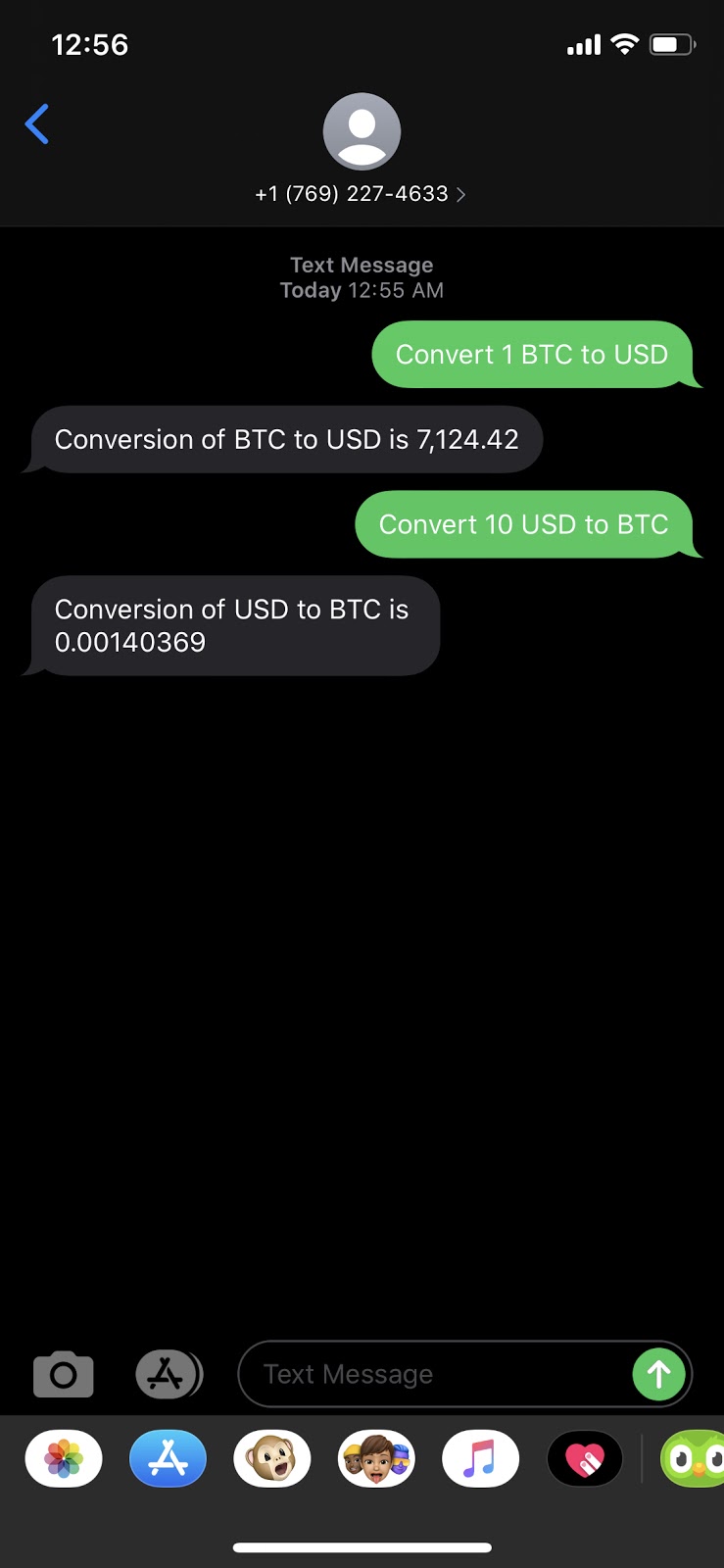
Conclusion
Good job! Now we can convert BTC to USD and vice versa using SMS. You can extend the project to allow multiple currencies such as Ethereum and bitcoin cash to enable a robust conversion system using SMS.
Bonus
Github Repo: https://github.com/ladaposamuel/bitcoin-converter-sms
Ladapo Samuel
- Email: ladaposamuel@gmail.com
- Twitter: @samuel2dotun
- Github: ladaposamuel
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.