Create an OpenCart SMS Extension to Notify Store Owners of New Orders
Time to read:
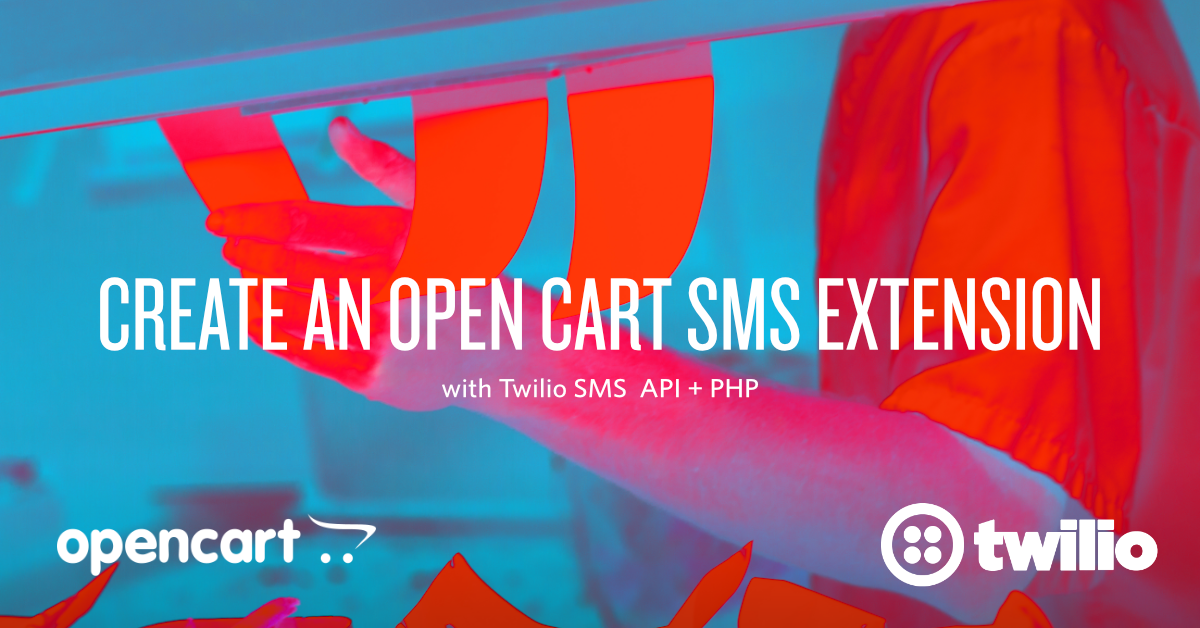
In this tutorial, we'll explore how to use the Twilio SMS API to create an OpenCart module that sends an SMS when a customer places an order, registers, or has their order history modified.
OpenCart is a free open source e-commerce platform for online merchants. It provides a professional and reliable foundation from which to build a successful online store. This foundation appeals to a wide variety of users; ranging from seasoned web developers looking for a user-friendly interface to use, to shop owners just launching their business online for the first time.
Tutorial Requirements
- PHP development environment using lamp (for Linux users), xampp (for windows users) e.t.c.
- Global installation of Composer
- Twilio Account
- OpenCart Installation
OpenCart requirement and Installation
OpenCart requirements and installation can be found in the OpenCart official documentation.
Understand the OpenCart folder structure
The OpenCart is one of the great examples of MVC framework as it provides separate folders for controller, model, view and language. In order to start creating your first OpenCart module, you must know the basic fundamentals of OpenCart. You can learn the basic fundamentals of OpenCart by visiting the OpenCart documentation. For creating a module in OpenCart, you have to follow a directory structure. You can learn about the directory structure here. So, in order to start module development in OpenCart, you have to create a file in the module folder of each controller, language, and view. You don’t need to create a model file in module development as your values of the module will be stored in a table named setting
. The queries for saving the values in the setting table are written in the admin->model->setting->setting.php
file.
Create a new OpenCart Twilio SMS custom module
Twilio Messaging API
The Twilio Messaging API makes it easy to send and receive SMS and MMS messages as well as query meta-data about text messages such as delivery status, associated media, and leverage tools like Copilot to manage your messages globally at scale. In this tutorial, we will only be using the SMS API for our OpenCart module. We will first learn the module development in OpenCart 3.2.0 (which at the time of writing is the latest).
First, we will create all the folders and files needed for this module using the given tree hierarchy below into a folder upload:
Create an admin interface to store the Twilio SMS Account credentials
To create the admin interface to store the Twilio SMS account credential three (3) files will be modified:
upload/admin/controller/extension/module/twilio_sms.php
controls the model, language and how to parse all the data to be used in the view file. In this file, you will also learn many other things about OpenCart such as how to load language, model, views and how to make their use of them in the view file. Add the following code to twilio_sms.php
.
upload/admin/language/en-gb/extension/module/twilio_sms.php
will assign the languages to the indices of an array. Please keep in mind that $_
is the name of the array. Add the following code to this file.
upload/admin/view/template/extension/module/twilio_sms.twig
contains the view portion of the admin module graphical user interface where the Twilio SMS account details such as your Twilio Account SID, Account Auth Token, phone number and also the status of the module will be inputted, saved, and edited. In this module you can install/uninstall and also enable or disable the module from the admin view section. Add the following code to the file.
NOTE: The view file is written using PHP twig templating engine as this is what is supported by the OpenCart version used in this tutorial.
Setup Twilio SMS
Create SMS template files
The purpose of creating this template is to replace the built-in email template that will not fit in the SMS screen and contain unnecessary fields not needed for SMS. These are custom SMS template view files we will be using to send SMS for orders placed by customers and when the status of an order changes.
NOTE: OpenCart already has some templates for sending email. Some of these templates will also be used for sending SMS since they fit in well while two of the templates will be written our self. The files below are the templates we will be writing.
Create the following files with the specified code.
upload/catalog/view/theme/default/template/mail/order_add_sms.twig
Include Twilio PHP SDK to OpenCart using Composer
To include Twilio’s PHP SDK in OpenCart we will be using composer for the installation. Run the command below in the root directory of your OpenCart project where the composer.json
is located.
After it has finished installing, go to \OpenCart_cart\upload\system\storage\vendor
to confirm the Twilio SDK installed properly by ensuring that the vendor
folder contains a twilio
folder like what we have below.
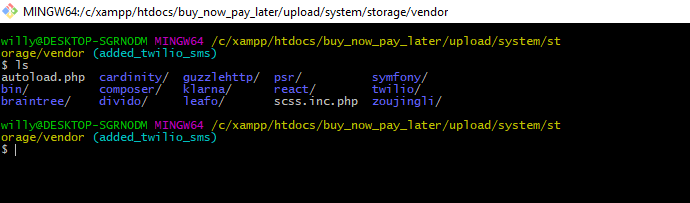
Understand how ocmod and OpenCart core modification system works
OpenCart Modification System
Modification System is one of the default functionalities of OpenCart allowing you to edit system functionality without directly editing core files. This feature works with creating an XML file. You can read further here for better understanding.
Implement an actions to send SMS to the customer using ocmod to modify OpenCart core
This section of this tutorial includes the virtual modification of OpenCart files with the respective functions to send, import Twilio SMS code, and taking care of users who registered their phone number with the country code and local number without the country code this way the module will be able to validate phone number before sending messages to the number.
Create a file named install.xml in the root of the module and fill it with the content of the file below or just download the file to your system at twilio-sms.ocmod->upload->install.xml.md
NOTE: The naming is very important in ocmod as this is what is going to be used for making this module installable from the open cart admin. To understand how creating a module in OpenCart works, look into the link in the previous step of this tutorial.
Now we should have something like this:
Package Your Code Into an Installable Module
To make this module installable, zip the module (including the upload folder and the install.xml file together) and name it anything you want. In my case, I named mine twilio-SMS.ocmod.zip
.
NOTE: Make sure the zip file name has an extension of ocmod.zip to avoid error when installing it from the admin end.
We should have something like this:
NOTE: To install this module from the OpenCart instance admin, all you need is the zip file (twilio-sms.ocmod.zip).
This module is written to work with Nigeria phone recipient number. To make this work with your country open the file install.xml
in the root of the SMS module and change all occurrence of +234 to your own country code then zip the module again, then you can install and it will work with your country.
Remember to enter a verified phone number if you are using a trial account, otherwise enter any number you’d like to receive the notifications from.
Testing
To test, open up your website on the browser and navigate to the admin area (website/admin).
- From the Admin interface, install the module we just created and refresh the modification for our changes to take place
- From the Admin interface, fill in your Twilio account credentials
- From the customer shopping interface,
- make an order and confirm an SMS is sent
- register a customer and confirm an SMS is sent
- approve a customer and confirm an SMS is sent
- reject a customer and confirm an SMS is sent
- modify the order history and confirm an SMS is sent
Testing Result
I have carried out my own testing and the results are below:
SMS result
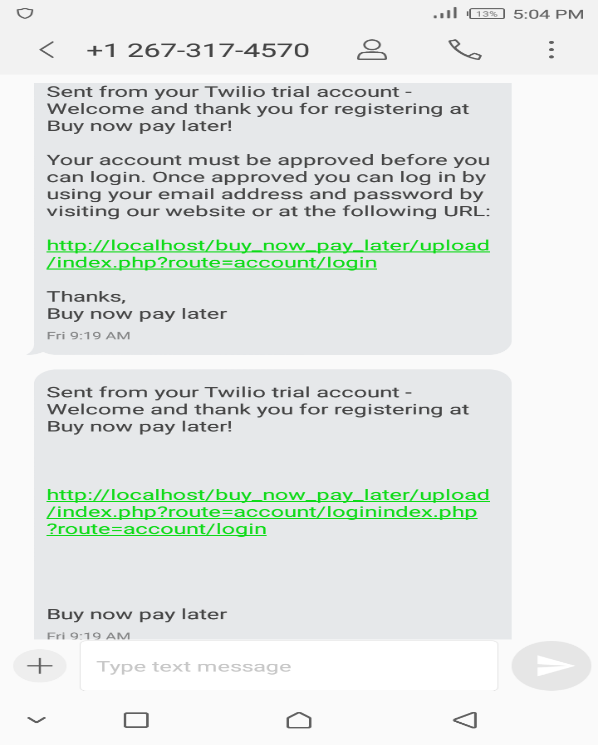
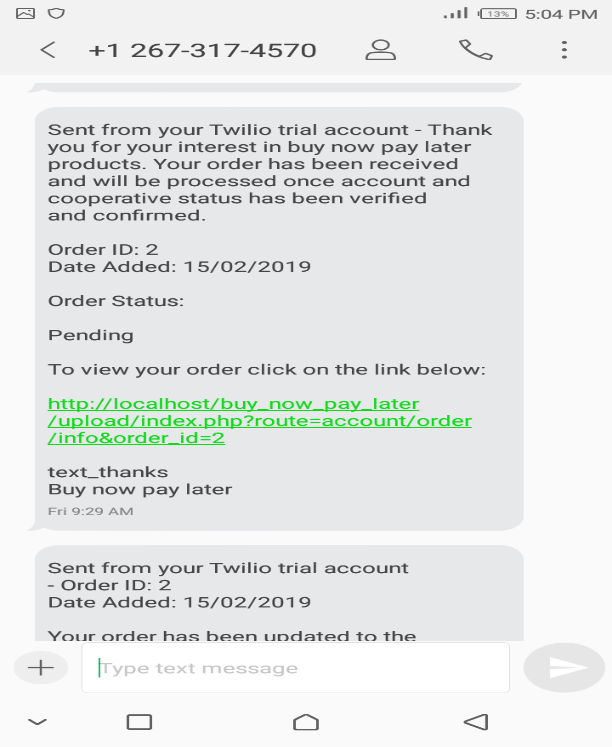
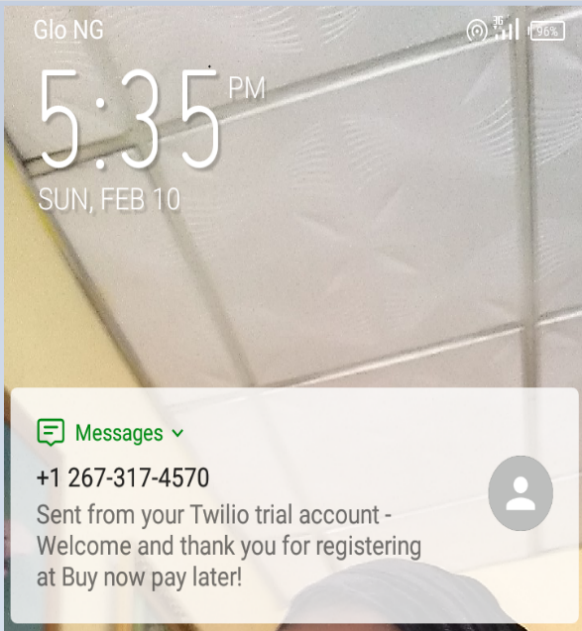
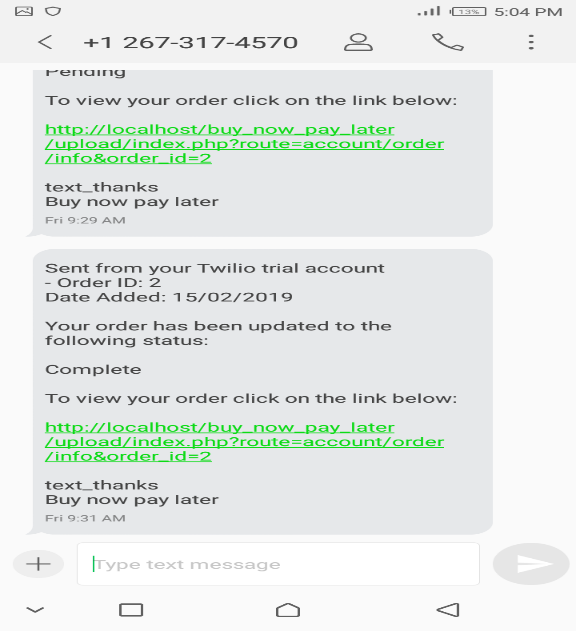
Potential Issues
SMS region permission:
Unable to create record: Permission to send an SMS has not been enabled for the region indicated by the 'To' number.

Solution:
What you need to do is go to your Twilio console, to the Geographic permissions (https://www.twilio.com/console/SMS/settings/geo-permissions) for SMS section and ensure you have the permission checked for sending to the country number you bought or the one you chose for your trial account. For me, mine is "US".
Permission error page:
Get permission error page when the Twilio credential module page is opened.
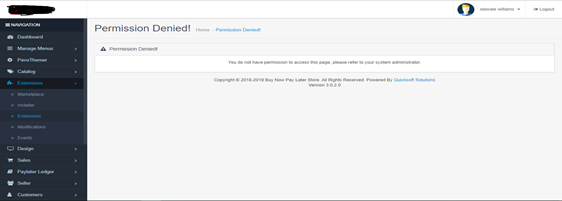
Solution:
Goto System->Users->User Groups
in the admin to grant access and modify permission to the user group as shown below.
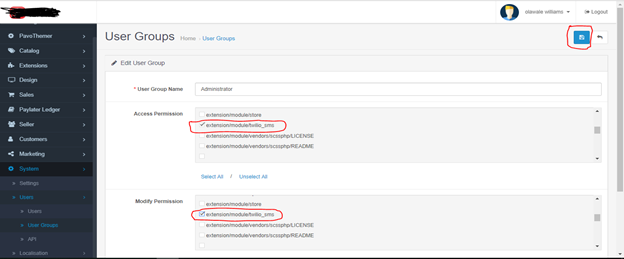
Conclusion
Now that you have completed this tutorial, you know how to:
- Create a custom open cart module
- Include the Twilio SDK in a new OpenCart module
- Add Twilio’s Programmable SMS to your OpenCart Store
Congratulations! You have just set up an OpenCart SMS module that sends corresponding SMS notification using Twilio programmable SMS API. Twilio programmable SMS API can be used for a lot more than we just did. You could work on building a customer service chatbot that not only alerts customers but also receives customer replies via text messages to address customers complaints, and also provides a timely response and leverage tools like Copilot to manage your messages globally at scale. I look forward to hearing about your projects.
The complete code can be found on Github.
NOTE: To use the code directly from my GitHub repo, you have to run the composer command (composer require twilio/sdk
) in the root folder of your OpenCart installation where the composer.json
for Twilio object to be available globally within the OpenCart installation and also don’t forget to verify your phone number for your trial account.
You can reach me via:
Email: olawalewilliams9438@gmail.com
Twitter: @sirwilly94
Github: williams9438
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.