Generating Lyrics in the Style of your Favorite Artist with Python, OpenAI's GPT-3 and Twilio SMS
Time to read: 4 minutes
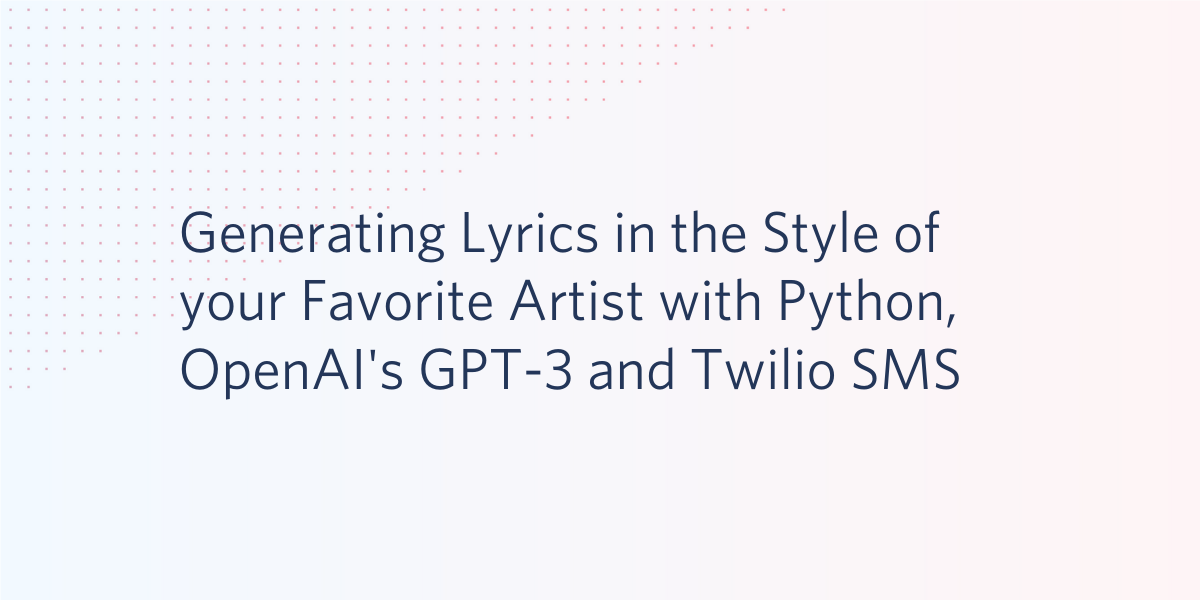
With concerts canceled and many artists being unable to release new music, people around the world are missing their favorite bands. What if you could fill that void by bringing any band or artist's lyrical style home with Python code to generate new songs?
Try texting the name of your favorite artist to +1 (315) 65-LYRICS (+1 315 659-7427) or +44 7401 193427 if you're in the UK to get lyrics in their style, and continue reading to find out how to program this yourself!
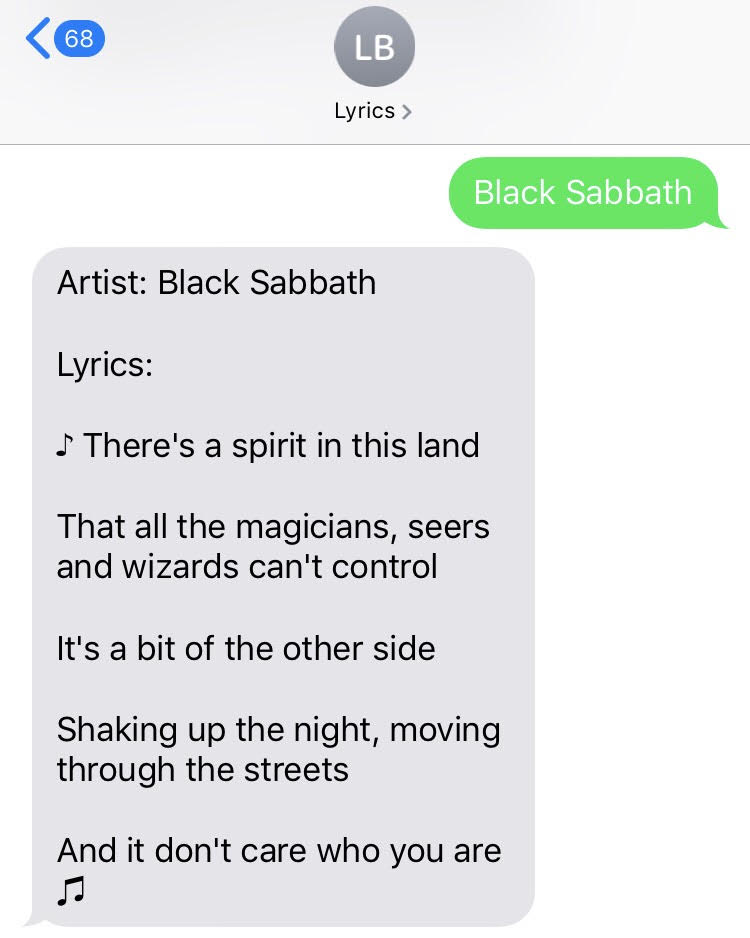
OpenAI's new GPT-3 (Generative Pre-trained Transformer 3) model was trained on a massive corpus of text making it incredibly powerful. This can be used to generate song lyrics in the style of any artist with surprisingly little input text given to it as a prompt.
Let's walk through how to create a text-message powered bot to generate song lyrics in Python using Twilio Programmable Messaging and OpenAI's API for GPT-3.
Before moving on, you'll need the following:
- Python 3.6 or newer. If your operating system does not provide a Python interpreter, you can go to python.org to download an installer.
- A virtual environment enabled before installing any Python libraries.
- A Twilio account and a Twilio phone number, which you can buy here.
- An OpenAI API key. Request beta access here.
- ngrok to give us a publicly accessible URL to our code
Introduction to GPT-3
GPT-3 (Generative Pre-trained Transformer 3) is a highly advanced language model trained on a giant amount of text. In spite of its internal complexity, it is surprisingly simple to operate: you feed it some text, and the model generates some more, following a similar style and structure.
We can see how versatile it is by giving it a tiny amount of input. Simply providing text with an artist name in a format like you'd see on a lyrics website can potentially generate lyrics in the style of that artist. For example, here's what it generates with the input "Artist: Michael Jackson\n\nLyrics:\n":
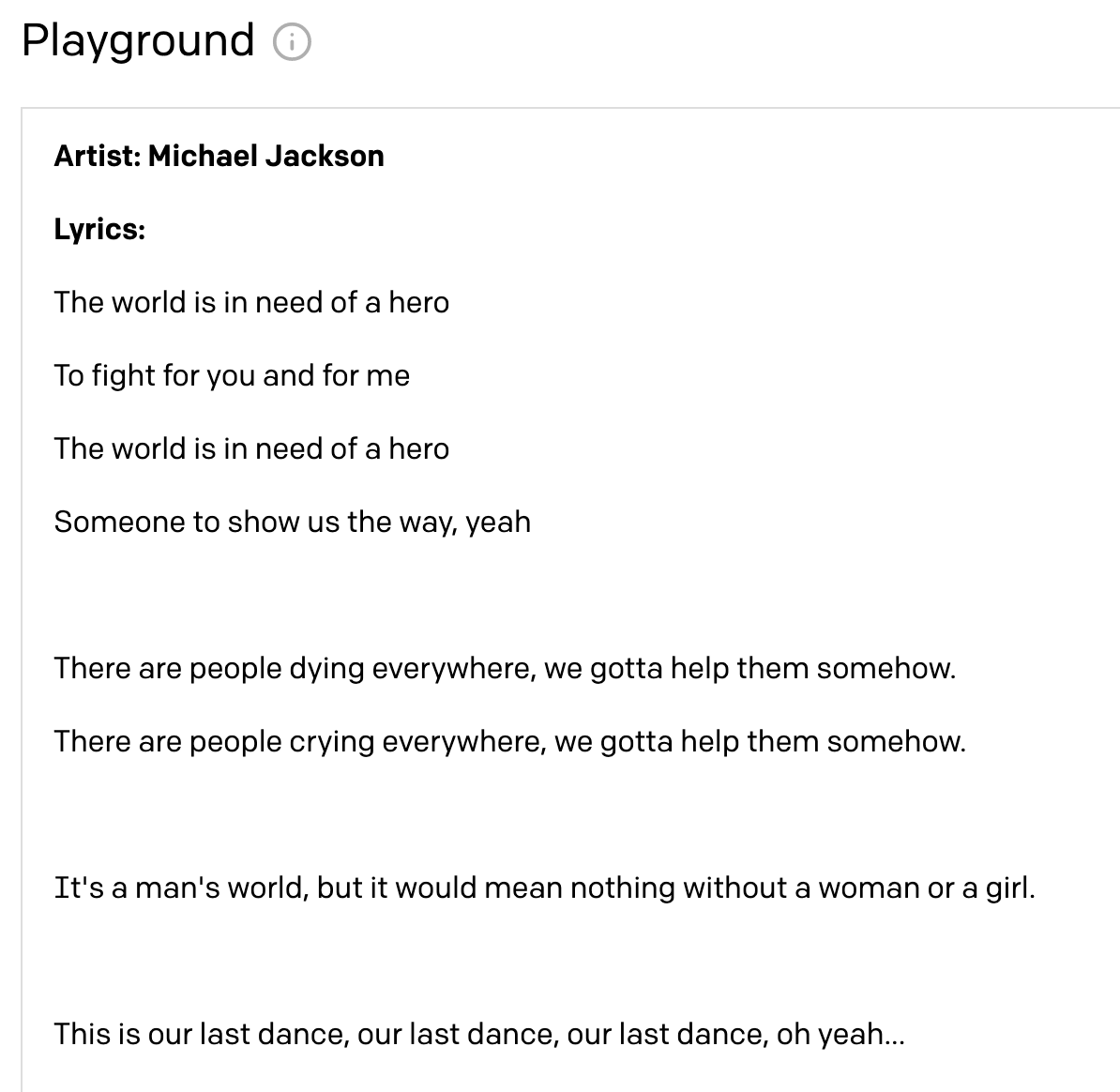
As mentioned above, this project requires an API key from OpenAI. At the time I’m writing this, the only way to obtain one is by being accepted into their private beta program. You can apply on their site.
Once you have an OpenAI account, you can use the Playground to play around with GPT-3 by typing in text and having it generate more text. The Playground also has a cool feature that allows you to grab some Python code you can run, using OpenAI's Python library, for whatever you used the Playground for.
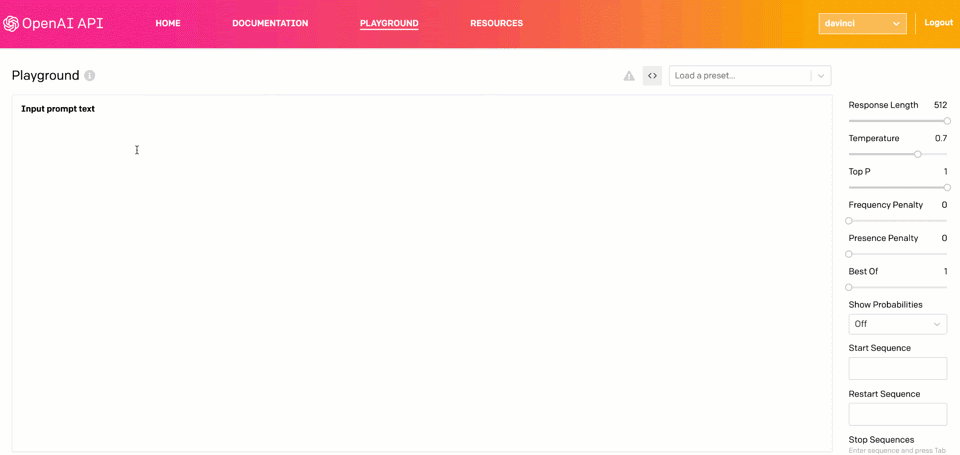
Working with GPT-3 in Python using the OpenAI helper library
To test this out yourself, you'll have to install the OpenAI Python module. You can do this with the following command, using pip:
Now create an environment variable for your OpenAI API key, which you can find as the "secret key" at the top of the Developer Quickstart.:
We will access this in our Python code. To try this out, open a Python shell and run the following code, most of which I took directly from the Playground:
To show that this even works with relatively obscure music, this code uses the longer name of one of my favorite heavy metal bands Slough Feg, but you can try it with any artist that you love. Run this code to see what happens. Sometimes it takes a few tries before coming up with a really good one, but sometimes you get gems.
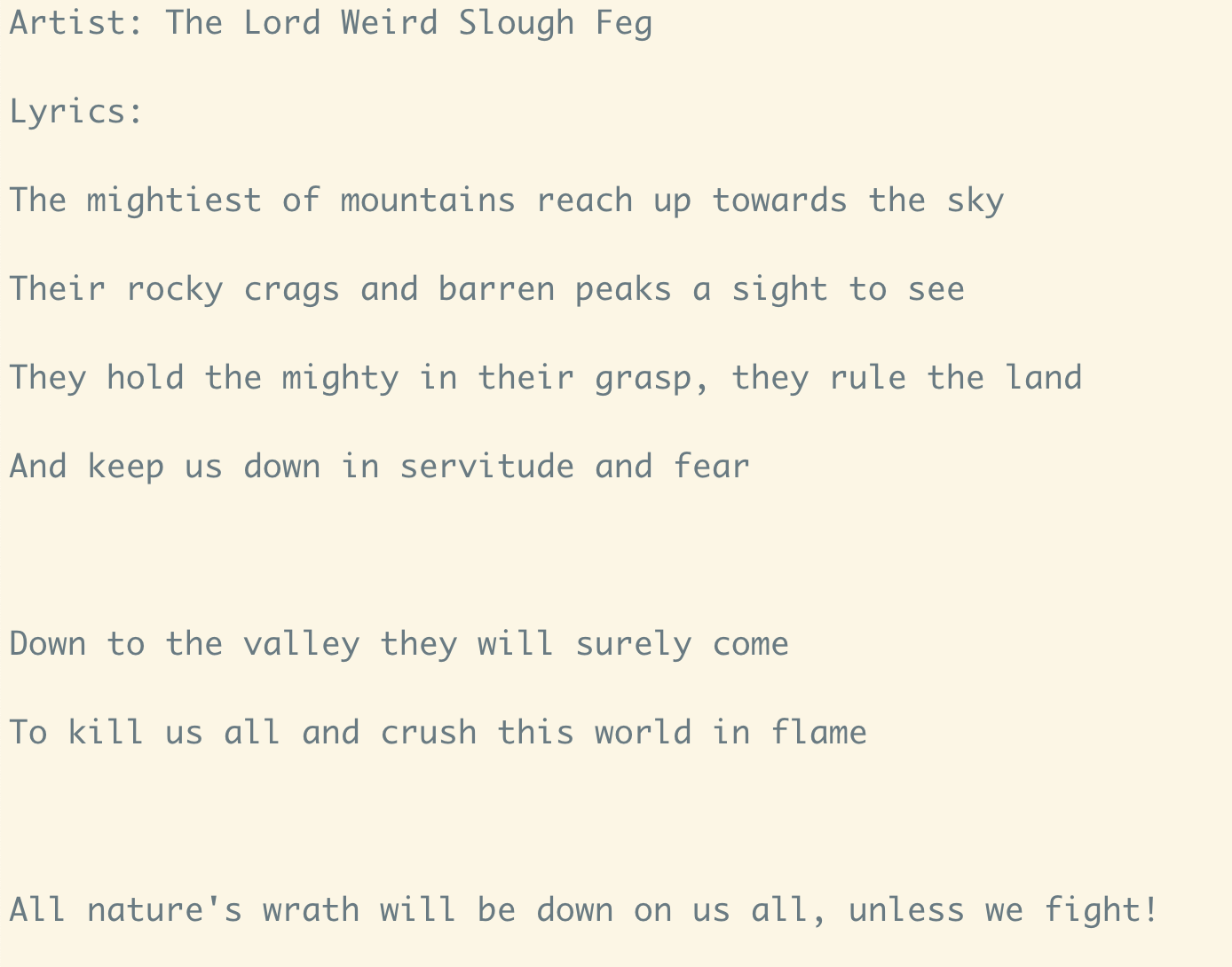
Configuring a Twilio phone number
Before being able to respond to messages, you’ll need a Twilio phone number. You can buy a phone number here.
We're going to create a web application using the Flask framework that will need to be visible from the Internet in order for Twilio to send requests to it. We will use ngrok for this, which you’ll need to install if you don’t have it. In your terminal run the following command:
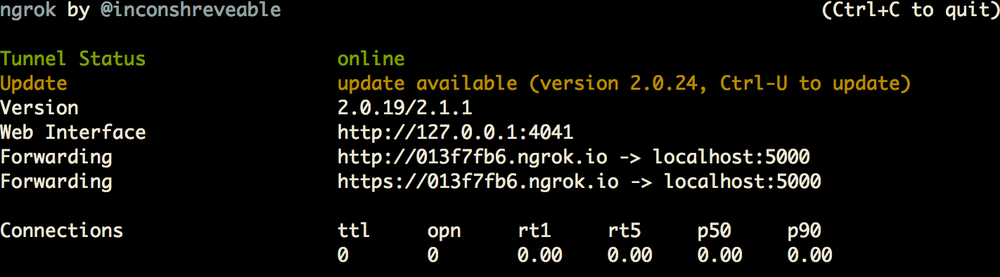
This provides us with a publicly accessible URL to the Flask app. Configure your phone number as seen in this image so that when a text message is received, Twilio will send a POST request to the /sms
route on the app we are going to build, which will sit behind your ngrok URL:
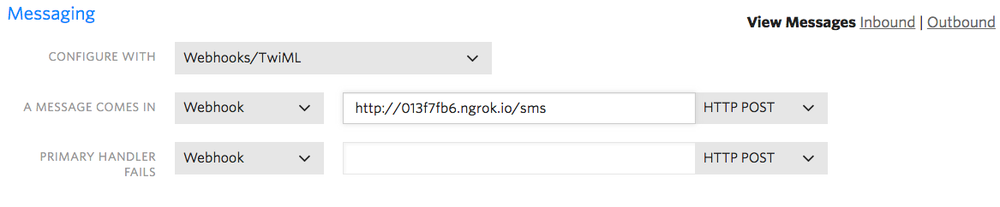
With this taken care of, we can actually build the Flask app to respond to text messages with some AI-generated songs!
Responding to text messages in Python with Flask
You have a Twilio number and are able to have the OpenAPI generate songs. It's time to combine the two.
Before moving on, open a new terminal tab or window and install the Twilio and Flask Python libraries with the following command:
Create a file called app.py
and add the following code to it for our Flask application:
This Flask app contains one route, /sms
, which will receive a POST
request from Twilio whenever a text message is sent to our phone number, as configured before with the ngrok URL.
Save your code, and run it with the following command:
Because we have ngrok running on port 5000, the default port for Flask, you can now text it and receive some lyrics.
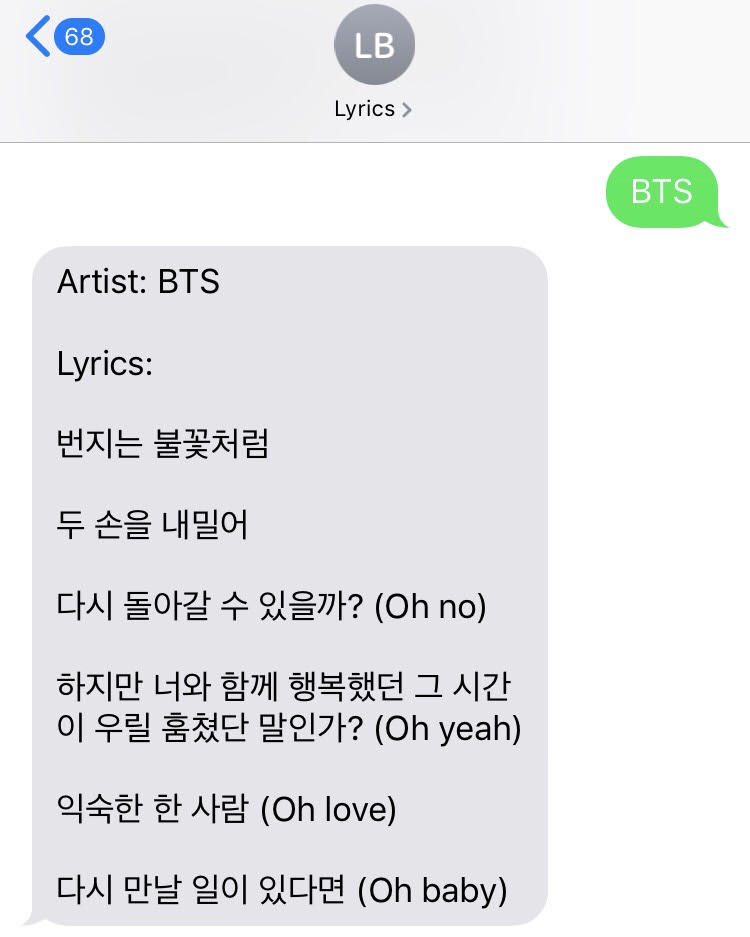
And sometimes after enough tries you may even get an instrumental, which is par for the course for certain bands.
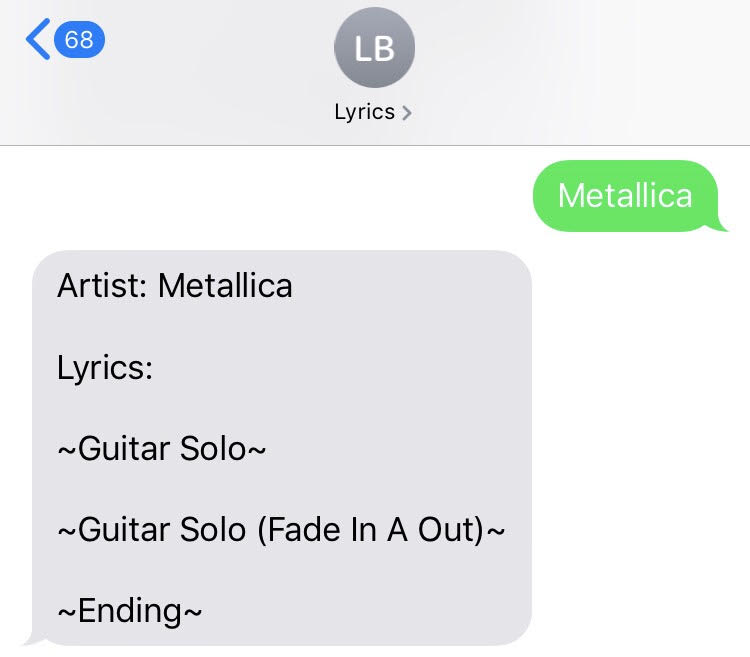
Moving on
Hopefully you've had fun generating new songs with code in the style of your favorite artists. In terms of technical changes you can also experiment with generating more tokens, and making up for the added time that takes by using Redis Queue to send a text message asynchronously when generation is finished, rather than risking a timeout by responding to Twilio's HTTP request right away with TwiML.
If you want to do more with Twilio and GPT-3, check out this tutorial on how to generate Dragon Ball fan fiction. You can also follow some other tutorials to narrow down which music genre you want to generate lyrics in, if you're into metal or punk music.
Feel free to reach out if you have any questions or comments or just want to show off any songs you generate.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.