How to send SMS Without a Phone Number using Alpha Sender and Java
Time to read:
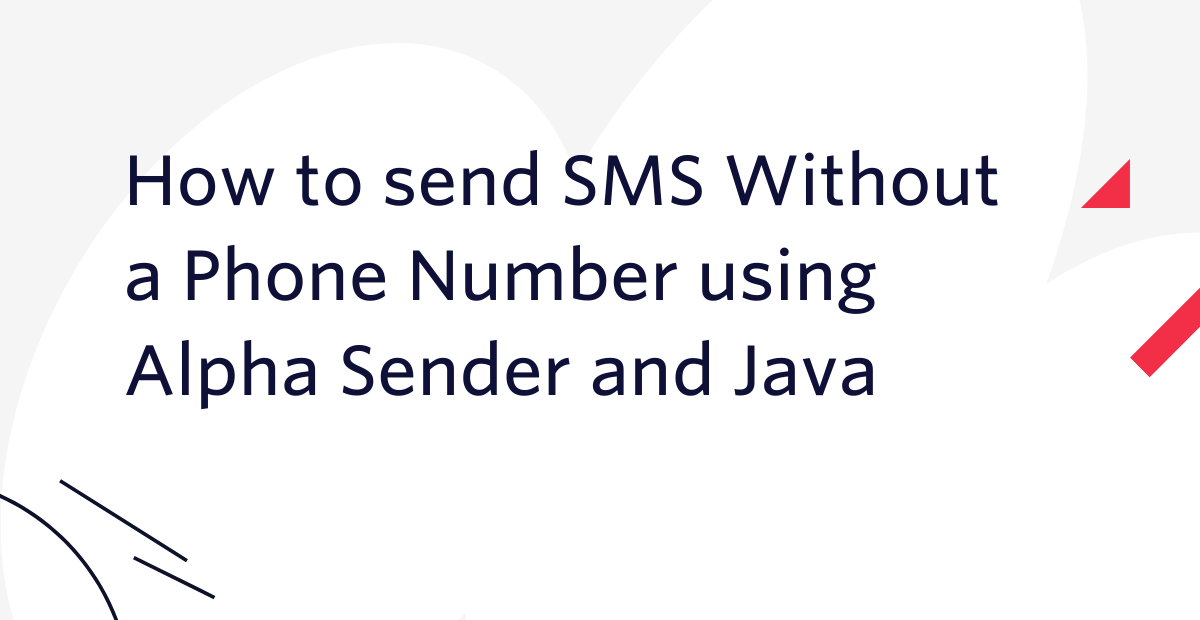
SMS is useful for two-way messaging – you can chat with your friends and engage with businesses. There are also a lot of use cases where one-way messages are handy, for example: notifications, alerts, verifications. For this kind of message you should consider using an Alphanumeric Sender ID.
Some things to know about using an Alpha Sender:
- A message identifies the sender by name, without the recipient needing to have a phone number in their address book already.
- A single Alpha Sender can be used across many countries without needing a dedicated phone number for each.
- It is not possible to reply to a message from an Alpha Sender. Pro: you don't need to handle incoming messages, Con: not suitable for use cases that need conversations.
An incoming message from an Alpha Sender called "MatthewTwlo" will look like this:
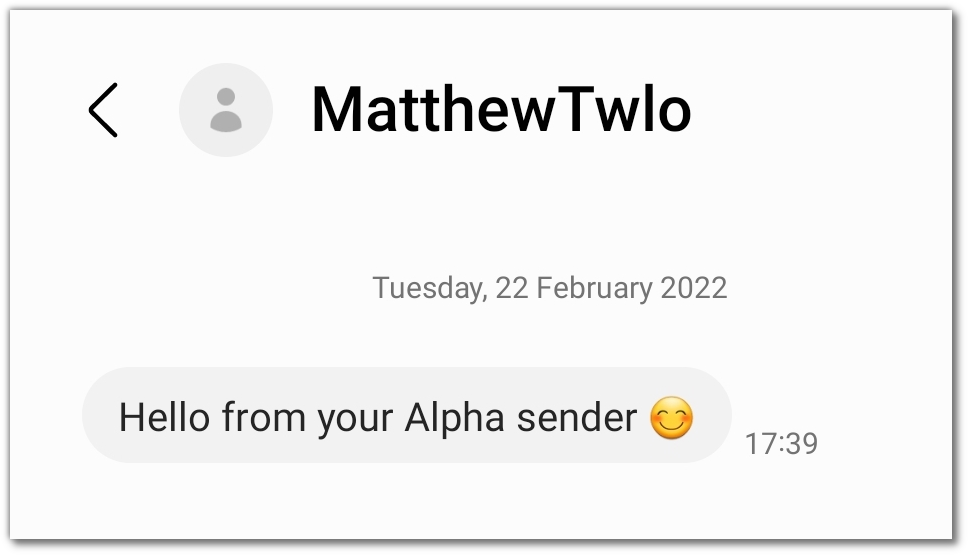
It isn't possible to send an SMS to an Alpha Sender, so my messaging app shows this message with no option to reply.
What you'll need to send Alpha Sender SMS
- You will need an upgraded Twilio account.
- The code at the end of this post uses Java, so to follow that section you will need to set up your Java dev environment.
How to set up an Alpha Sender
The recommended way to configure sending from an Alpha Sender is to set up a Messaging Service. It is possible to send Alpha Sender messages without a Messaging Service but we won't cover that here. Setting up a Messaging Service only takes a minute and will be more flexible in the future. Create the Messaging Service from the Twilio console menus:
Messaging > Services > "Create Messaging Service"
Create and configure a Messaging Service
Step 1
Give your Messaging Service a name:
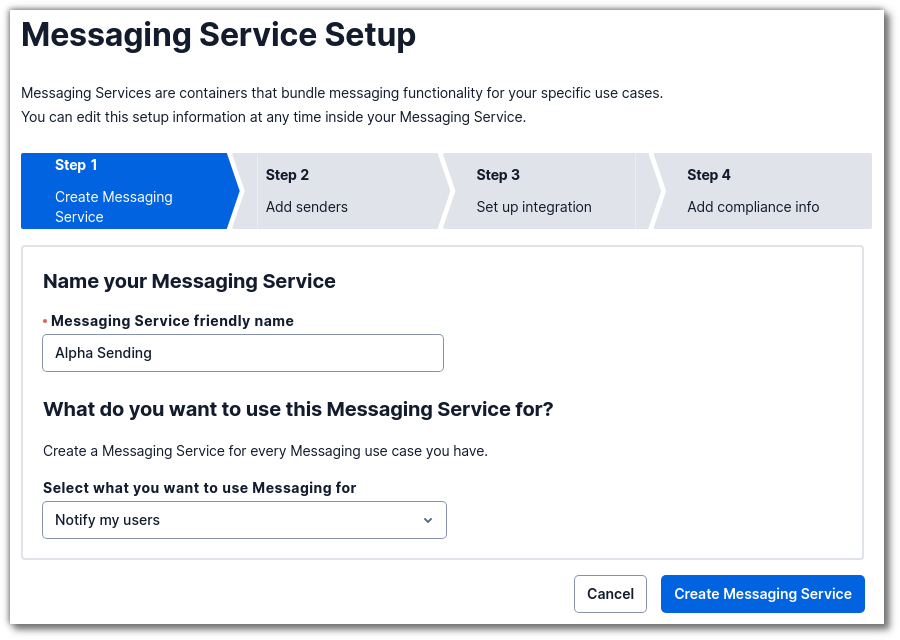
Step 2
Add a sender to your new Messaging Service, selecting "Alpha Sender" as the Sender Type:
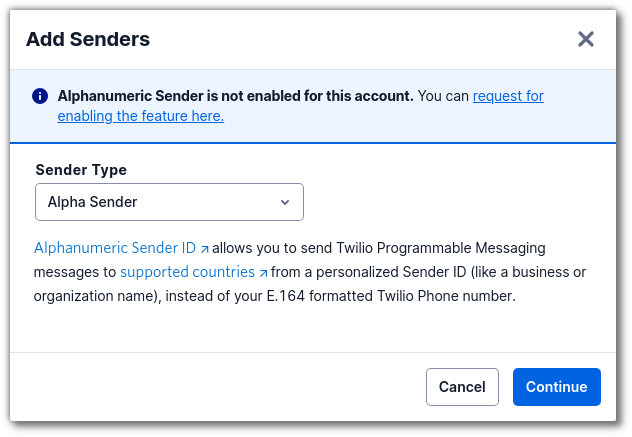
On this dialog are links to Alphanumeric Sender ID documentation and details of international support for Alpha Senders.
Next set the actual Sender ID. This will appear in recipients’ messaging apps, as seen in the example in the beginning.
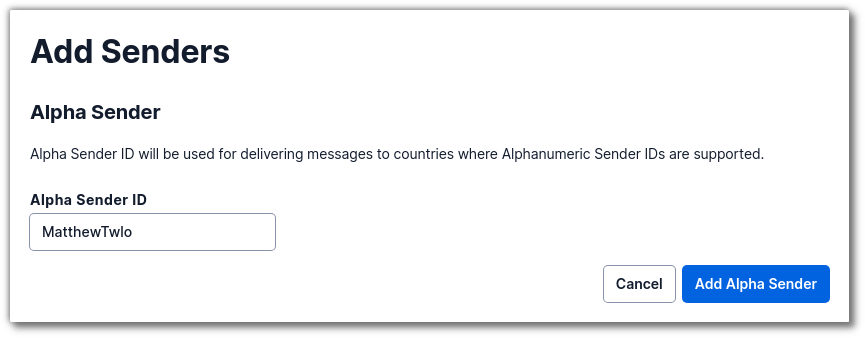
You are limited to 11 alphanumeric characters for the Sender ID.
Click on “Setup Integration”
Step 3
Set up integration: This relates to how incoming messages are handled by the Messaging Service, but Alpha Senders can't receive messages so you can click through to step 4 straight away.
Step 4
If you are sending Application-to-Person SMS in the USA you will be subject to A2P10DLC rules. I won't cover that here, but if it applies to you then follow this link to find out more and register. Click on the Complete Messaging Service Setup button.
Finish the setup
Click Complete Messaging Service Setup to finish setting up your Messaging Service and Alpha Sender.
You will be prompted to test from the console. (You can also get to this by navigating the console menus: Messaging > Try it out > Send an SMS) You may see a warning message that no phone numbers are configured for this messaging service which you can safely ignore - after all, when using an Alpha Sender, not having a phone number is expected.
There will be a curl command that you can Copy/Paste to your terminal. Put your Auth Token in. You'll receive an SMS to your phone from your Alpha Sender ID. Notice that you can't reply.
Send SMS from Alpha Senders in Java Code
Now you have set up everything you need in your Twilio account, it's time to write some code. If you want to skip ahead, the full project is available on GitHub.
Create a new Maven project
Create a new Maven project in your IDE. One quick way to do this is to use your IDE's New Project wizard. Once you have a Maven project set up, you're ready to start coding.
Use a recent version of Java
By default Maven may assume you are using an old version of Java. Update the project to the latest version with the following snippet of XML in pom.xml
if it's not there already:
The Twilio helper library requires at least Java 8, and 11 is the most widely used at the moment.
Add the Twilio Helper Library to your project
Add a dependency on the Twilio helper library by adding the following to the <dependencies>
section of your pom.xml
. Create the top-level <dependencies>
section if it's not there with the following lines:
These changes should leave your pom.xml
looking like this.
Write a little Java
Create a new class called AlphaSender
in src/main/java
. Paste the following content:
This code relies on 4 environment variables:
TWILIO_ACCOUNT_SID
Find this on your Twilio Console.TWILIO_AUTH_TOKEN
Find this on your Twilio Console.DESTINATION_NUMBER
The phone number you want to send to. Use E.164 format.MSG_SVC_SID
The ID of your Messaging Service. You will find this on the Twilio console page where you created the Messaging Service above.
Once you have saved the code and set up the environment variables, you can run this class directly from your IDE. You may see warnings about unconfigured logging libraries, which you can ignore for this example (in a larger app you should configure logging properly). The phone you put as DESTINATION_NUMBER
will buzz. Congratulations, you've used an Alpha Sender to send SMS!
Wrap up
If you made it this far, congratulations! You've sent an SMS from an Alpha Sender - but what's next is up to you. I'd love to hear how you're using Twilio and Java, get in touch with me to talk about what you're building or ask me anything.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.