How to use custom HTTP clients with Twilio in Java
Time to read: 2 minutes
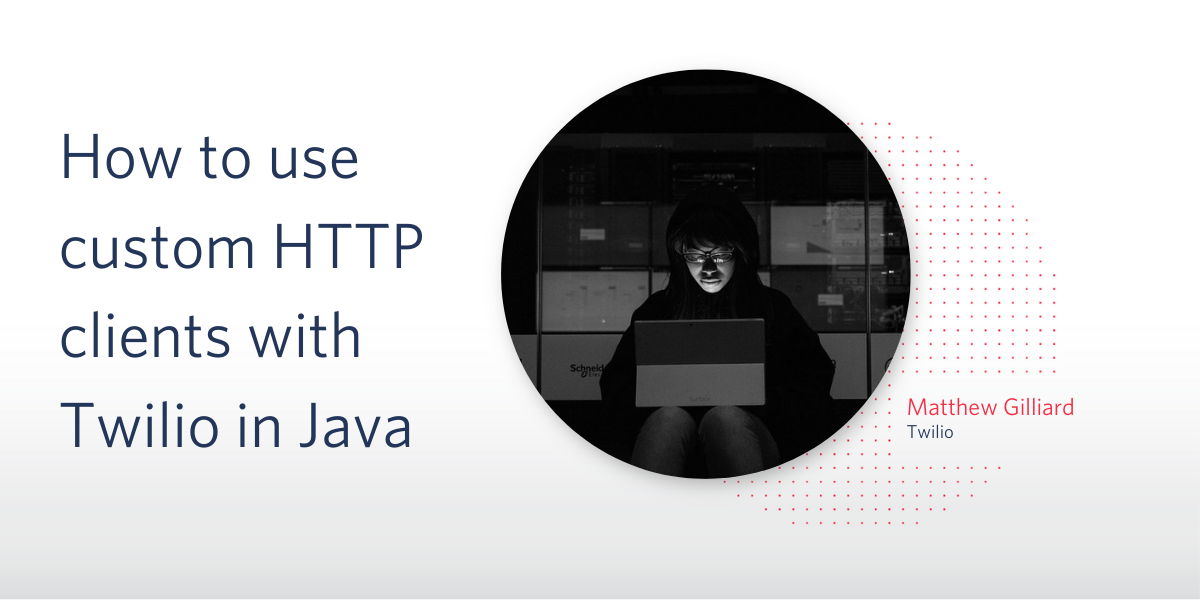
[Header image adapted from this image, CC BY 2.0 from Microsoft NYC]
When building a Java application that uses Twilio's API most developers will have code that runs at startup, initializing the Twilio helper library like this:
This sets the account credentials as static fields in the Twilio
class. Effectively they are now global settings for the application, and when your code calls the API these will be used automatically, and the helper library creates an HTTP client for you. This means that you don't have to repeat your credentials every time you call a method that uses the Twilio API:
This is convenient, however there are cases where you want more control over the HTTP client. This might be to configure an HTTP proxy, or to provide custom credentials if you are using multiple Twilio accounts or Projects in the same codebase.
To enable this, all the methods which call the Twilio API are overloaded to take an instance of TwilioRestClient
. If you pass a TwilioRestClient
each time you call any of the create/read/update/delete methods, you will no longer need to call Twilio.init()
, you can customize your HTTP clients and use as many different instances of them as you need.
In this post I'll show how to do that for a couple of common cases:
- Using multiple sets of Twilio credentials
- Calling the Twilio API through an HTTP proxy
The code samples are all in a repo on GitHub, too.
Using custom HTTP clients to work with multiple sets of credentials
If you're using several Twilio accounts or Projects in one application, you shouldn’t configure your Account SID and Auth Token globally. Instead, you can use the builder pattern provided by TwilioRestClient
to create as many clients as you need. Here's an example where I've been working on two apps ("Cake" and "Pie") using different Twilio Projects with different credentials and phone numbers:
By passing in a TwilioRestClient
client to each call to .create
I can control which Project is used (and billed) for each message. To make sure I keep my two sets of credentials safe, the Secrets
class loads them from environment variables.
Using a custom HTTP client with an HTTP proxy
Twilio's API lives on the internet, and many folks on corporate networks will therefore need to configure an HTTP proxy to access it. Again this is possible with a custom TwilioRestClient
, although in this case we'll need to write some code of our own. There are a few layers to this, so let's unpack them:
- A
TwilioRestClient
builder can take a custom instance ofcom.twilio.http.HttpClient
. (This would be a good place to inject a mock for testing your calls to the Twilio API) - The default implementation of Twilio's
HttpClient
is calledNetworkHttpClient
. NetworkHttpClient
can be initialised with an Apache HttpComponentsHttpClientBuilder
.HttpClientBuilder
can be configured to use a proxy.
To create an HttpClient
instance, this code is sufficient:
Then, use that method to create a proxied client and add it to your code like this:
In Summary
Using the defaults for configuring your Twilio credentials and creating an HTTP client is convenient, but there are definitely times when a bit more control is needed. I've shown a couple of typical cases, and if you need more control the Apache HttpClientBuilder docs are comprehensive.
If you're building with Twilio, I'd love to hear about it. Let me know what you're up to - I can't wait to see what you build:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.