Implementing Programmable Chat with Laravel PHP and Vue.js
Time to read: 4 minutes
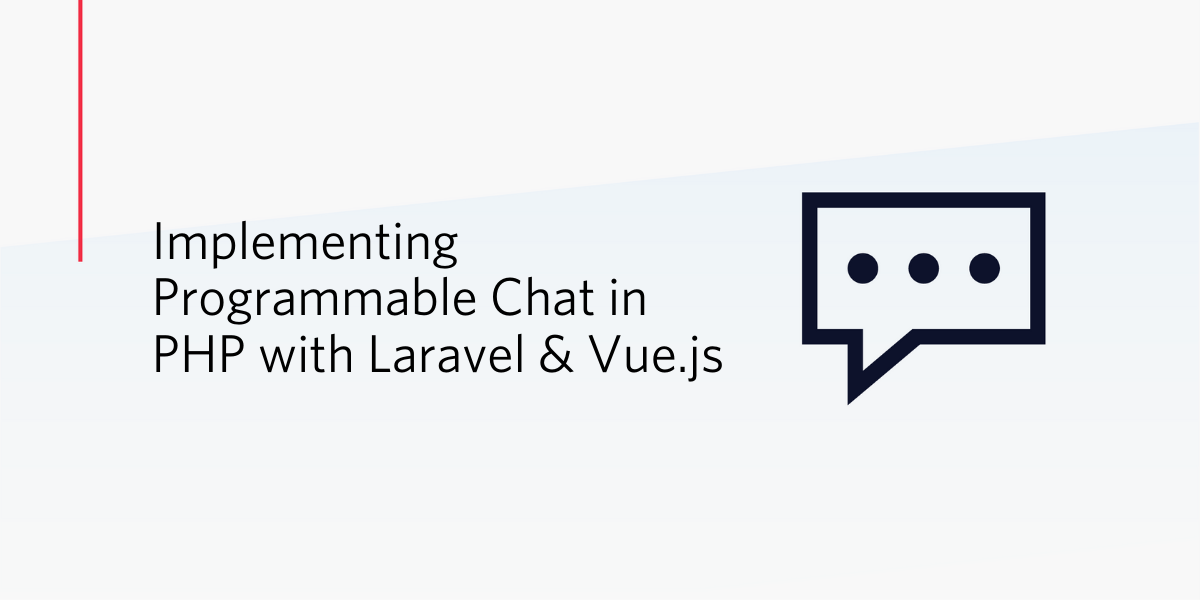
This tutorial will teach you how to use Twilio's real-time, Programmable Chat technology. You will be able to see this in action between two or more browser sessions. At the end of this tutorial, you would have developed a chat system between users.
Installing dependencies and Requirements
Here is a list of dependencies you need to install, and steps needed to be taken before you can complete this tutorial.
- PHP 7.1+
- Composer
- Npm
- Laravel
- A Twilio Account
If you have Laravel installed on your machine, you can proceed to creating a new project by running any of the commands below:
or using the composer create-project command
After this process , we will need to install the Twilio package in the project directory with Composer using the commands below:
NOTE: Your version of vue-template-compiler might be a mismatch for your current version of Vue. If so, re-install with the correct version such as npm install vue-template-compiler@2.5.16 --save-dev
When you have created your Twilio account, make sure you set up the Programmable Chat service and record the Chat SID and Chat Secret.
Your Twilio account comes with some important keys that will be needed in the course of this tutorial. You should save them in your .env
file.
After all of these configurations have been completed, a controller needs to be created called TwilioChatController
. It will house the basic code for token generation. Run the following command to create the controller.
We now need to make our controller aware of some Twilio classes needed for functionality and setup the constructor with some properties. Add the following code to TwilioChatController.php
:
Creating the view
We need to create a view that would house the Vue.js Component we will be interacting with. Create a blade file called chat.blade.php
in the resources/views
folder. Add the following code:
NOTE: We have a twilio-chat
component that houses our Vue.js code. You should go ahead and create TwilioChat.vue
in the resources/js/components
directory.
Inside a Vue.js Component
The view component consists of a template, some styling, and the JavaScript code used to interact with Twilio’s APIs. Let's get into it.
The template basically consists of the HTML to house our "chat box and channels". Add the following code to resources/js/components/TwilioChat.vue
:
Next, we will add the chat box, which contains a message container and input boxes for the username and the chat message. Replace the line of code <!-- Add the chat box code here -->
with the following code:
Observe that we have some data attributes and functions:
- tc.messagesArray
: an array which holds the messages in the current channel.
- showMessages
: a boolean value which signifies we can fetch messages for the current channel.
- userNotJoined
: a boolean which signifies whether or not the user has joined or connected to a channel.
- connectClientWithUsername()
: A function triggered when the enter key is pressed. This function fetches a token and registers the member with the Twilio chat service.
- handleInputTextKeypress()
: A function which handles the action of sending messages.
Add the following code to the bottom of the file. It will provide the functionality that we defined in the <template>
above.
Let's look at the methods we defined in the HTML above and include them within the methods
object of the <script>
:
The connectClientWithUsername()
function fetches a token assigned to the user through the fetchAccessToken()
method.
Creating the routes
In web.php
(located in the routes
directory), we will add the following route to fetch the token:
In our TwilioChatController, the getToken
method looks like the following code. Add it to the file located in app/Http/Controllers
.
Next, replace the root route /
with the following:
Back to the Vue Component
Once the token is retrieved, connectMessagingClient()
connects the user to the Twilio Chat client. Then loadChannelList()
gets all the channels assigned to this client.
Add the following code to methods()
in TwilioChat.vue
:
Joining a channel
The General Channel is the default channel that would automatically be created in this process. We will add the method needed to join the general channel with the following code:
If the general channel is not found, it creates it. If it is found, it sets up the channel by fetching the messages unique to the channel though the setupChannel()
function.
Add setupChannel()
to the methods
as follows:
The setupChannel()
method calls a variety of methods, but we can focus on the joinChannel()
for now. Through this method, the member joins the channel (general or a personally created channel).
Creating a channel
We can now add the channel section to our Vue template which will list all channels available to the client. In the <template>
, replace <!-- Add the channel code here -->
with the code the following code:
Now add the Vue function handleNewChannelInputKeypress
to create a channel:
Switching channels
Based on the list of channels you have available, you can switch to any channel of choice using the selectChannel
method. Add it now:
You can also delete the current channel with the following code :
Using a web browser of your choice, visit http://127.0.01:8000
. Fill in the form and submit.
Finally open up the application in two windows or browsers. You can enter a username and send a message on one of the windows or browsers. Check the other instance and also interact with it.
Conclusion
You could extend the application built in the tutorial by displaying a list of users. Now that you have completed this tutorial, you know how to:
- Add Twilio’s Programmable Chat to your Application
- Expose actions between chat users
Favour Oriabure
Email: favoriabs@gmail.com
Twitter: https://twitter.com/favoriabs
Github: https://github.com/favoriabs
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.