Improving PHP Code Quality with PHP CodeSniffer
Time to read: 3 minutes
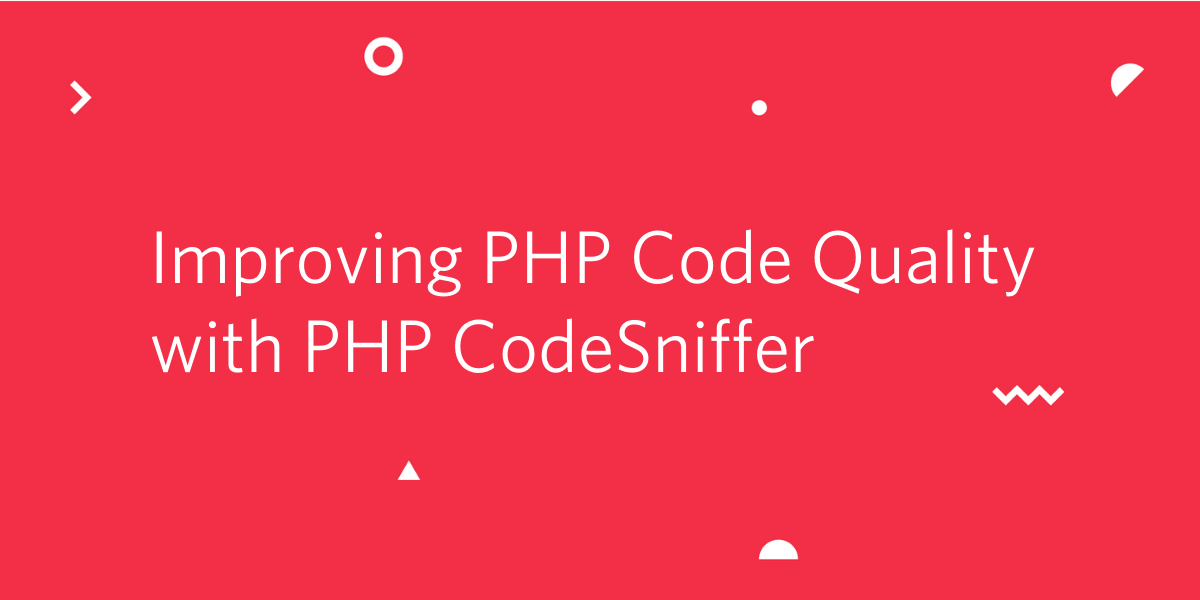
As a project grows, it becomes necessary to maintain and enforce some defined standards for the code. Such standards help achieve uniformity, readability, and maintainability, irrespective of the engineer that wrote the code. For a PHP project, one of the best ways to enforce such standards is PHP_CodeSniffer (or PHPCS for short).
PHPCS is a tool that helps detect violations of pre-defined coding standards. It also includes an additional tool that can automatically correct those violations.
Prerequisites
To complete this tutorial you will only need Composer globally installed.
About the project
PHPCS is framework-agnostic, but for simplicity, we will be using it with a simple Laravel project. The project, called “Twilio Greeter”, displays a list of common greetings depending on the language chosen by the user. You can view the complete source code here.
The project files that are of interest to us are:
PROJECT_ROOT/app/Http/Controllers/WelcomeController.php
: Our main controller has anindex
method which retrieves the preferred language from theGET
parameter and sends the data to the view for rendering.PROJECT_ROOT/resources/views/welcome.blade.php
: Displays the list passed into it from the WelcomeController.PROJECT_ROOT/routes/web.php
: Our project routes file
You can learn more about how the above files work and Laravel in general from the official Laravel documentation.
Setting up the project
Clone our greeter app and change into the project directory with:
Next, run
to install the project dependencies and
to start the server. Our application is now accessible at http://localhost:8000
.
Installing PHPCS
Since it’s desirable to run the PHPCS CLI as a stand-alone command, we will install it globally with the following command:
You can check your installation with:
NOTE: If you get an error similar to “command not found”, ensure you place the Composer bin folder in your PATH (usually $HOME/.config/composer/vendor/bin
for Linux and $HOME/.composer/vendor/bin
for MacOS).
Our Preferences
Our application works fine after cloning, but the coding style could use some improvements. PHPCS supports lots of coding standards out of the box such as PSR12, PSR2, PEAR, and Zend. You can also create your own rules if they are all found wanting.
For our use case, we want some rules such as:
- Visibility of all properties/methods must be explicitly declared (PHP defaults to public visibility if none is provided).
- Opening and closing braces for methods should be on a separate line.
- Function calls that span multiple lines should have only one argument per line e.g
Fortunately for us, our preferences are conventional and have been covered by the PSR12 standard, so we will just go ahead and use the PSR12 as our base configuration.
Configuring PHPCS for our Project
To start, let’s create a new file called phpcs.xml
in our project root directory with:
and add the following:
The code above has been commented to provide some explanation, but you can look up the PHPCS wiki to see the different options you can provide.
Running our first test
At this point, our project is ready and we can test for violations now. In the root directory, run:
If all went well, you should see an output similar to:
Fixing as we go.
The report above included the files and the line number in which we went against the rules. For instance, we need to specify the method visibility on line 8 and move the opening brace on line 18 to a new line.
A quick way out would be to run:
in the project directory and PHPCS will automatically try to fix the violations marked with [x]
, but let’s attempt to fix them ourselves.
From our report, the only file we need to clean up is app/Http/Controllers/WelcomeController.php
so open it and make the following corrections. First, we add a blank line between line 1 and 2 so it looks like the following:
Then, we specify the visibility for the showGreetings
method and move the opening brace to a new line, so replace:
with:
Finally, we mark our greetings method as private
(since we don’t need to call it outside of the WelcomeController class). We’ll also move it’s opening brace to a new line too as follows. Replace:
With:
NOTE: The clean version of the project is on the tidied
branch and you can view it by checking out the tidied branch like so:
Now, run phpcs again and our errors should have disappeared.
Conclusion
Code guidelines help to reduce the cognitive load required to work in a project with multiple developers, and PHPCodeSniffer does a good job of ensuring adherence to pre-set standards. If you’re looking to build your own custom rules, the existing rulesets are a good place to start.
I hope you enjoyed the post and if there are any issues or questions, please feel free to reach out to me via Email or on Github.
Additional Reading
To further your education, check out the related topics:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.