It’s getting easier to get sentimental about SMS with Twilio Add-ons
Time to read: 4 minutes
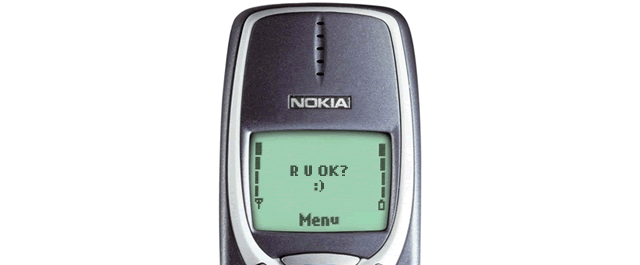
Twilio Add-ons is a marketplace for 3rd party services that you can use to add superpowers to your Twilio applications. I’m a huge fan of Add-ons because they handle tasks we would otherwise need custom code for in our applications. We can also streamline existing applications by replacing our code with an Add-on.
Last year I wrote about sentiment analysis of SMS messages using Twilio, Bluemix and AlchemyAPI. It didn’t take a lot of code to create the beginnings of a powerful service based on sentiment analysis of incoming SMS messages. But with code I often find less is more. At least, less code that I have to maintain is better in my mind. One of my favourite things is deleting code, so let’s see what happens if we take that original code and replace the explicit call to the AlchemyAPI with the IBM Watson Message Sentiment Add-on.
Tools
We’re going to be building on top of the original Ruby application that I wrote.
- It runs on IBM’s Bluemix platform which you can use with a free trial
- You will also need a Twilio account, you can sign up for a free Twilio account too
- To get the application deployed and running, I recommend reading through the previous post.
If you’ve got all that, then let’s see how we can improve this project.
Switch on the Add-on
First we need to switch on the Add-on for our account. Open up the Add-ons section of the Twilio console and find the IBM Watson Message Sentiment Add-on (or click here to go straight to it). Click the big red install button, read and agree to IBM’s terms of service and you’re nearly done. Just check the box that enables the Add-on for incoming SMS messages, save the form and you will start receiving sentiment analysis with every text.
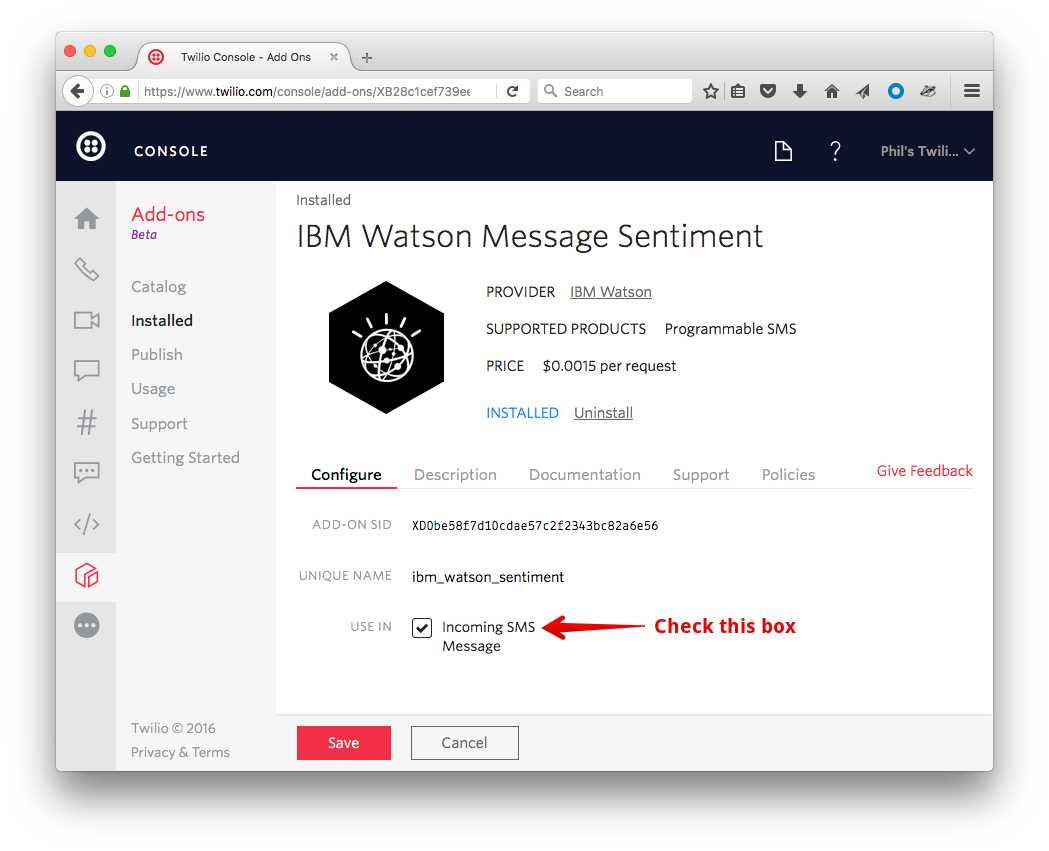
Please note that this will pass all incoming messages to your account through this service and charge you $0.0015 per request. If you don’t plan to use this after this blog post remember to turn off the Add-on.
Time to remove some code
Now that the work to gather the sentiment analysis from Watson is being done by Twilio we’re not going to need the AlchemyAPI gem any more. Remove it from the Gemfile
.
And stop requiring it in helloWorld.rb
.
We no longer need to pick up our AlchemyAPI credentials from Bluemix’s VCAP_SERVICES
. Nor do we need to initialise the AlchemyAPI wrapper with an API key.
Now we get to our /messages
endpoint. This is the path that Twilio will use to POST SMS messages to as they arrive. Instead of receiving the message and sending the body off to the AlchemyAPI we will be getting the sentiment analysis along with the message.
Using Twilio Add-ons we get an extra parameter delivered with our message, AddOns
. It contains a JSON string which contains all the information Twilio has gathered based on the Add-ons you have activated in your account. We need to parse the JSON, find out if Twilio was successful in retrieving the results from the Add-ons and then reach into the results and extract the data we are interested in.
When using the IBM Watson sentiment analysis Add-on the results live under the ibm_watson_sentiment
key. You can see more about this in the Add-on documentation, but the format is:
To replicate our previous feature, we need to retrieve the type
from within the docSentiment
field.
The rest of the request is the same. Well, there’s actually some error handling here that I missed the first time around. We check if the Add-on returned successfully by ensuring the status is “successful”, in the case that we didn’t have a successful result we want to return the generic response that isn’t based on sentiment. So we add the else
to that conditional like so:
And that’s it! Push your work up to Bluemix, wait for the deploy to complete and send yourself a positive or negative SMS.
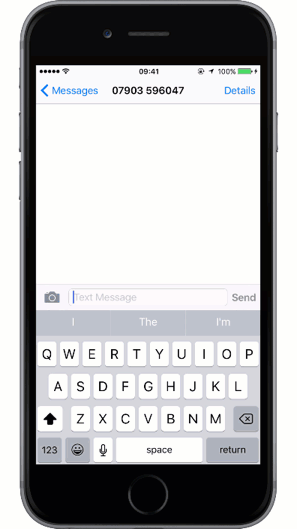
You’ll see you have the same results, but with one less gem dependency, one fewer HTTP request in our code and 3 less lines of code total.
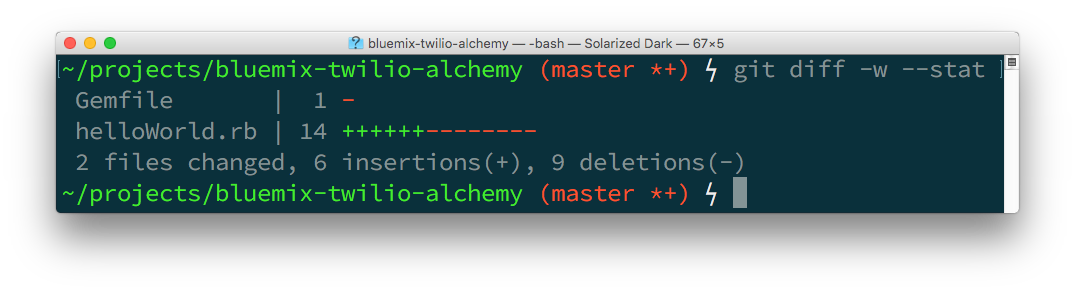
Twilio Add-ons do the work so that you don’t have to
We’ve seen today that including an Add-on can save you time, effort and code. Using Twilio Add-ons for a feature like sentiment analysis means your application can be more powerful and more intelligent without you having to write a whole bunch of code or learn a new API or platform. In our simple example we removed a dependency and an HTTP request and required less lines of code to achieve the same result. Check out the code now.
There’s more to Add-ons than just sentiment analysis too.
- If you wanted to go deeper into the analysis, check out the IBM Watson Message Insights Add-on which extracts semantic metadata from content so that you can understand what your users are saying.
- You can also analyse the phone number itself with Add-ons like the Whitepages Pro Phone Intelligence Add-on that can help you identify fake sign ups, find out what type of line a phone number is connected to and whether it is pre-paid or not.
- Or find out your caller’s name and other details with Next Caller’s Advanced Caller ID Add-on
Check out all the available Add-ons and find out the super-powers your application could gain. Maybe you’ll get to delete some code too!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.