Migrate from Lookup V1 to Lookup V2
Time to read: 4 minutes
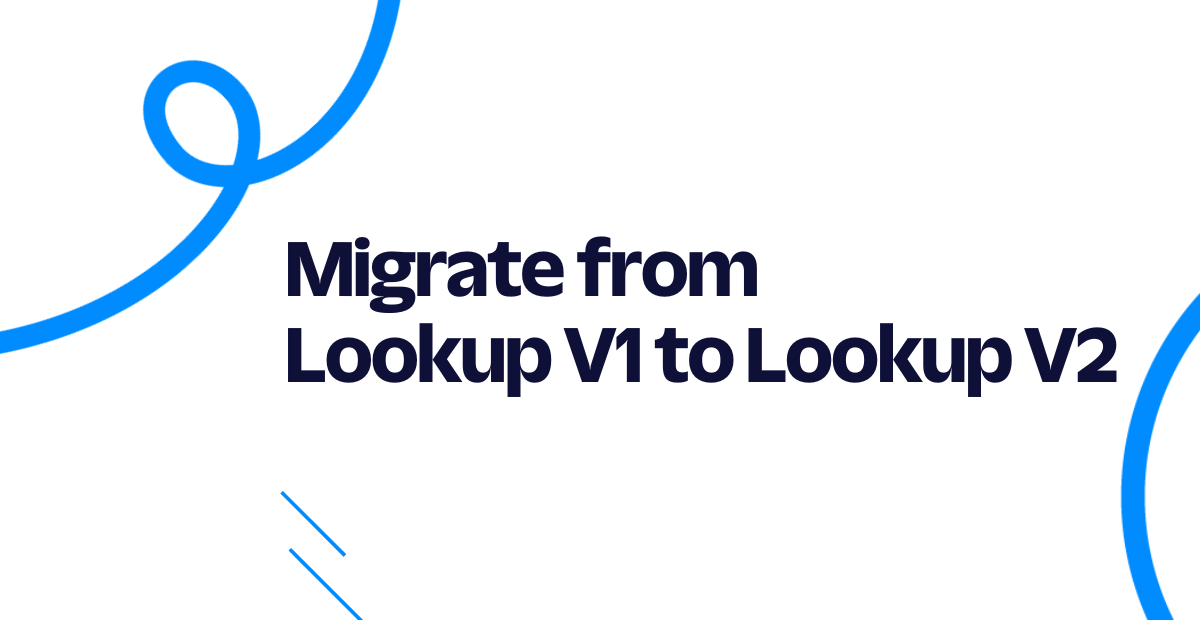
Twilio's Lookup API has helped customers build customer onboarding experiences that seamlessly improve deliverability, mitigate risk, and produce a better user experience. We're excited to expand the Lookup API capabilities with new features and expanded geographic coverage with Version 2 of the API, now in Public Beta.
With continued support for formatting and validation, line type and carrier detection, and caller name information, V2 includes the following enhancements:
🌏 Global support for line type detection, including VoIP 🎉
☎️ 12 line type response options (expanded from 3) including: fixedVoip
, nonFixedVoip
, landline
, mobile
, personal
, tollFree
, premium
, sharedCost
, uan
, voicemail
, pager
, and unknown
.
✅ Improved phone number validation error handling, with error messages including INVALID_COUNTRY_CODE
, TOO_SHORT
, and TOO_LONG
.
🆕 New data packages like SIM swap and call forwarding detection.
This blog post will provide the technical requirements to migrate from Lookup V1 to V2.
Migration requirements for phone number Lookup V2
The new API uses a different API URL (or helper library method call) and will have additional response fields dependent on the data package you request.
API differences between Lookup V1 and V2
V2 Base URL
The new base URL (and associated helper libraries) use v2 instead of v1 so 'https://lookups.twilio.com/v1/PhoneNumbers/+15108675310' becomes 'https://lookups.twilio.com/v2/PhoneNumbers/+15108675310'.
Type becomes Fields
To request additional data, use the Fields
parameter in V2. Possible values include:
line_type_intelligence
(replacescarrier
from V1)caller_name
sim_swap
call_forwarding
live_activity
Request multiple Fields
by comma-separating field names.
New response fields
The response JSON is the same with the addition of the following fields:
V2 new response field | Description |
---|---|
calling_country_code | International dialing prefix of the phone number defined in the E.164 standard. Examples include '1' for the US or '57' for Colombia. |
valid | Boolean that indicates if the phone number is in a valid range that can be freely assigned by a carrier to a user. |
validation_errors | Contains reasons why a phone number is invalid. Possible values: TOO_SHORT, TOO_LONG, INVALID_BUT_POSSIBLE*, INVALID_COUNTRY_CODE, INVALID_LENGTH, NOT_A_NUMBER. |
Other | New packages only available in V2 like 'sim_swap' and 'call_forwarding' (others may be added at a later time) |
See V2 API documentation for more resource details and code samples in multiple languages.
Line Type Intelligence is the new Carrier Lookup
Lookup V2 Line Type Intelligence (LTI) is an improvement on the V1 version of carrier lookup with more line type options and global support for VoIP detection (VoIP detection was US only in V1).
LTI (v2) has 12 possible line types (e.g. mobile
, nonFixedVoip
) compared to carrier (v1) which only had 3 possible line types. LTI continues to return carrier information (e.g. Verizon, O2) when available.
Here is a comparison of the V1 carrier lookup vs V2 LTI lookup in JavaScript.
Carrier (V1):
Line Type Intelligence (V2):
See V2 code samples in more languages in the documentation.
Caller Name Lookup: V1 to V2
The request is very similar in V2 but for API consistency, the V2 caller_name
parameter uses snake case.
V1:
V2:
Error handling
In V1, an invalid phone number like +123
would return an HTTP 404 response.
In V2, an invalid phone number like +123
will return an HTTP 200 with valid=false
and validation errors like TOO_SHORT
. This is useful to propagate error messages back to an end user for potential typos or gain insight into the type of invalid numbers in your database.
Possible validation errors include: TOO_SHORT, TOO_LONG, INVALID_BUT_POSSIBLE*, INVALID_COUNTRY_CODE, INVALID_LENGTH, NOT_A_NUMBER.
This change applies to all requests regardless of requested fields
.
Best practices
Use Lookup at new user sign up
Instead of calling Lookup before every time you send a one-time passcode, trigger an API request when you collect a new phone number like at sign up or during a phone number change. This will help you prevent typos and potential fraud.
Learn more about best practices for phone number validation during new user registration.
Use Lookup to audit your database
Many customers perform periodic (e.g. quarterly or annually) database clean up. You can use Lookup to determine if numbers are still valid or still the expected line type. This will help prevent sending messages to landline numbers.
FAQ
What is the difference between Carrier Lookup and Line Type Intelligence?
V1 carrier lookup returns 3 line types: mobile
, landline
, and voip
. VoIP detection only works for US numbers with carrier lookup.
V2 Line Type Intelligence returns 12 different line types and the information is available globally. Available line types for LTI include:
Type | Description |
---|---|
landline | The phone number is a landline number; generally not capable of receiving SMS messages. |
mobile | The phone number is a mobile number; generally capable of receiving SMS messages. |
fixedVoip | A virtual phone number associated with a physical device (e.g., Comcast or Vonage). |
nonFixedVoip | A virtual phone number that can be obtained on the internet without the need for a physical device (e.g., Google Voice or Enflick). |
personal | A phone number designated for personal use. |
tollFree | A toll-free phone number, which is one where calls are free for the calling party. |
premium | A premium rate phone number, which typically charges higher than normal rates for special services. |
sharedCost | A shared cost phone number, which is one where the charge is partially paid by the calling party and charges higher than normal rates. |
uan | A universal access number, which is a national number which can route incoming calls to different destinations. |
voicemail | A phone number associated with a voicemail service. |
pager | A phone number associated with a pager device. |
unknown | A valid phone number, but the line type could not be determined. |
What is Lookup's pricing?
See twilio.com/lookup/pricing for updated pricing information.
Get started with Lookup V2
If you're ready to start building, consider adding Lookup filter VoIP numbers before initial phone verification or filter landlines before an SMS campaign.
If you still have questions or encounter any issues with your migration, please reach out. I can't wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.