Developer Fundamentals: Parameters
Time to read:
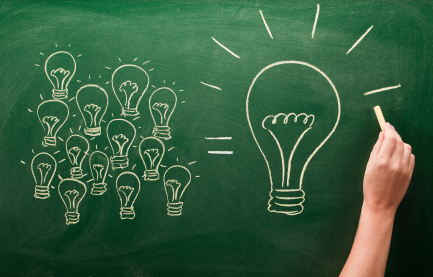
Parameters
What are parameters?
All of the data that you use in a given program comes from somewhere. Sometimes it’s obvious that you’ve assigned a given value to a specific variable or constant within the [scope] of the code you’re looking at, but other times a program will make use of variables or constants where the values have been assigned somewhere else and passed along to the code you’re looking at.
Parameters refer to variables or constants that a program needs to have passed in from somewhere else rather than being defined or looked up within said code. The values passed into those parameters are generally called arguments.
<ex>
How are they used?
To see an example, create a file calledprogram.php and paste in the following code:
If you run this program by executing the following command in your terminal, the values for the parameters it needs will look like a series of values following the file to be executed:
In this example, the output of this program would be "A whole bowl of fruit, and you choose 3 red apples." This is useful, but you might notice that the assignment of value to parameters by $argv
is entirely based on the order the values are given. If instead you executed:
Your output would now read "A whole bowl of fruit, and you choose apples 3 red."
If you wanted to be able to provide more context for whatever human might use this program in future, you might instead redesign your code to use flagged parameters:
To run this update code, you’ll need to change your command to include to the named options the code is now expecting:
As demonstrated in both of the above examples, when programmatically calling a function that has parameters, you follow the function name with parentheses containing a comma-separated list of the values (or variables containing those values) for the parameters in the order they’re declared:
When creating a function or command, you specify any required or optional parameters to be provided when calling that function.
It’s perfectly possible to declare a function or command which doesn’t use any parameters or which only uses optional parameters, but if a function or command has any required parameters, it will not even attempt to execute if values for those parameters aren’t provided when the function or command is called.
What can make parameters tricky?
Many large code projects, and even relatively simple coding frameworks, often fulfill parameter requirements through inheritance, where the value is generated or defined somewhere else in the codebase.
While this can make complex operations easy to utilize, it can also make troubleshooting particularly challenging if anything interferes with that inheritance such as a function being called from an unexpected location or an inherited value being overwritten in a way that makes it no longer match what the inheriting function or command expects.
Debugging and troubleshooting functions with parameters
If you’re troubleshooting a function or command that takes in parameters but is giving you unexpected behavior, a great step to figuring out what’s going on is to add print commands for the values of parameters so you can make sure they match your expectations.
Fun bonus fact:
In functional programming, the concept of recursion is where you run the same function repeatedly using the output value from the previous run for the parameter input in subsequent runs:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.