A Simple Way to Receive an SMS with Ruby, Sinatra, and Twilio
Time to read:
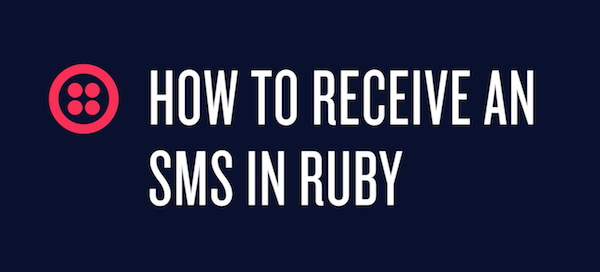
Here’s all the code you need to receive an SMS message and to send a reply using Ruby, Sinatra, and Twilio:
If you’d like some explanation on how that code works, watch this short video, or just keep reading.
When someone texts your Twilio number, Twilio makes an HTTP request to your app with details about the SMS passed in the request parameters. In this post, we’ll use Sinatra to handle Twilio’s request, parse the parameters, and to send a response.
First we install Sinatra from the console:
We create a file called app.rb and require Sinatra:
Then we create a route called message to accept a POST request.
Details about the inbound SMS are passed via the request parameters. If you want to see all of them, you could drop a puts params
in here and watch the console when the text comes in. In our case, we’ll just grab the number the message was sent from and the body of the message.
(A common mistake here is to forget to capitalize the keys, so be careful there.)
Great, so we’ve accepted an inbound SMS and pulled information from it. But how do we send a reply? After all, it’s rude when someone texts you to not text them back.
When Twilio makes that HTTP request, it expects an HTTP response in the form of TwiML, a simple set of XML tags that relay instructions back to Twilio.
So we set the Content Type to return XML.
Then we implicitly return a string that contains our TwiML <Response> to tell Twilio to reply with a <Message> that includes the number it was sent from and message body from the inbound SMS.
We save our file, then start our Sinatra app from the console.
This app needs a publicly accessible URL, so you’ll either need to deploy it to the cloud or use a tool like ngrok to open a tunnel to your local development environment.
Ngrok will provide you with a url that points to your localhost.
Set up Twilio
Sign up for a free Twilio account if you don’t have one.
Buy a phone number, then click Setup Number. Scroll to the Messaging section and find the line that says “A Message Comes In.”
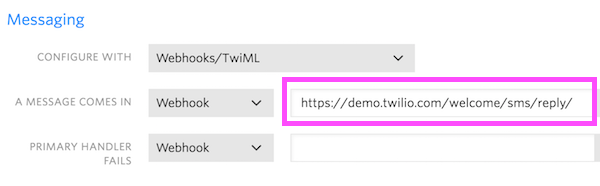
Fill in the full path to your file (i.e., https://yourserver.com/message.php) and click Save.
Now, send an SMS to your shiny new phone number number and revel in the customized response that comes back your way.
Next Steps
If you’d like to learn more about how to use Twilio and Ruby together, check out :
And if you’d like to chat more about this, drop me a line at gb@twilio.com.
Happy Hacking!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.