Schedule surprise messages with Twilio SMS for a mystical date
Time to read:
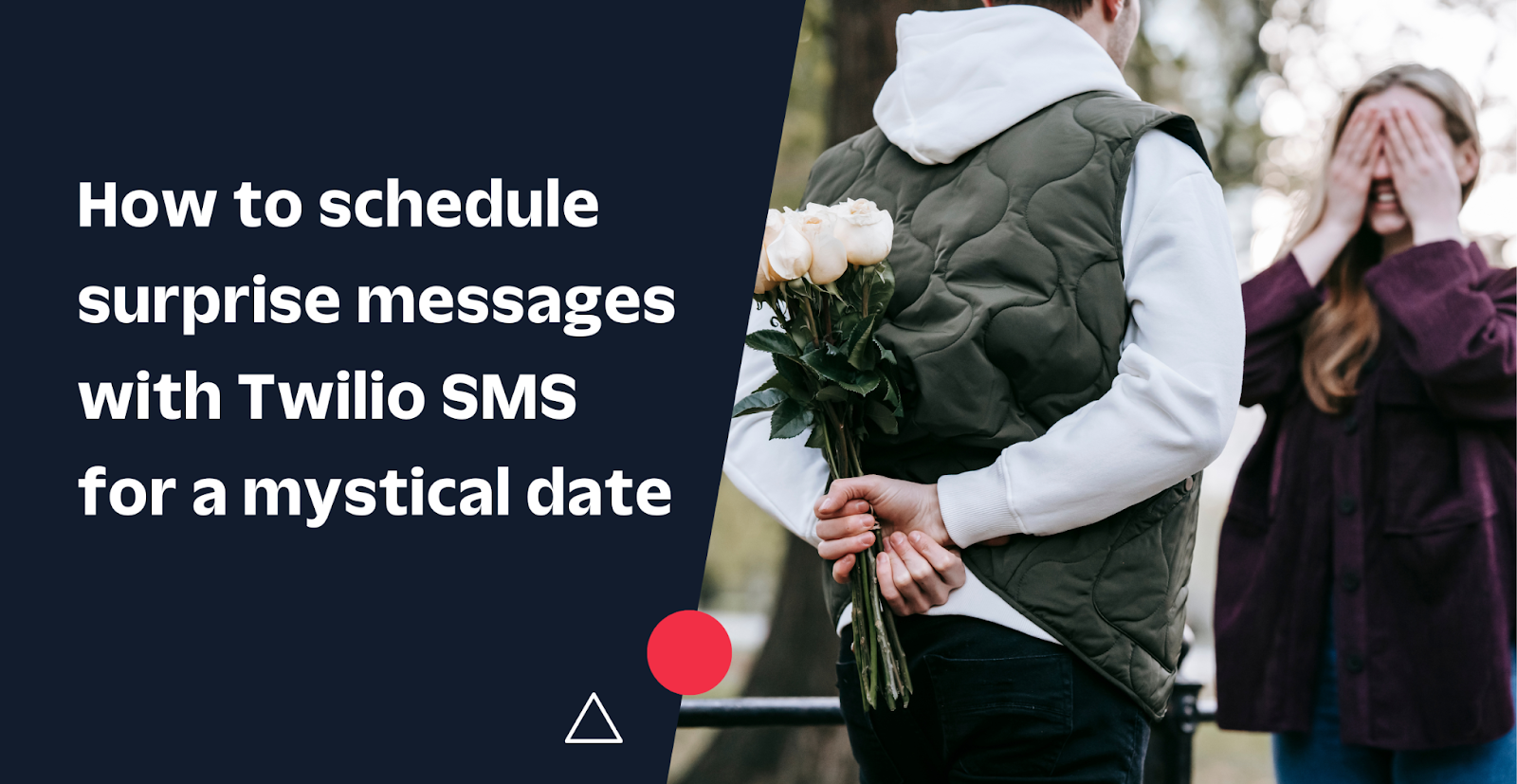
Imagine that you want to give a fantastic dinner to your loved ones a great New Year's Eve dinner, but you want to keep it a surprise for as long as possible. So you decide to start sending them different SMS messages with a series of clues on different days to maintain the "mystique" of this surprise.
In this article, you will create a console application to send scheduled SMS messages with Twilio that are sent at a specific time/date.
Prerequisites
You will need the following for your development environment:
- a .NET IDE (Visual Studio, VS Code with C# plugin, JetBrains Rider, or any editor of your choice)
- .NET 6 SDK (earlier and newer versions should work too)
- A Twilio account (try out Twilio for free)
You can find the source code of this tutorial in this GitHub repository.
Configure Twilio
Get your Twilio Credentials
To interact with Twilio, you will need an account with credits (it can be with trial credits for this tutorial), and you'll need to get your Twilio Account SID and Auth Token to use the Twilio SDK. Sign in to the Twilio Console and find your Account SID and Auth Token on the home page of your Twilio Account.
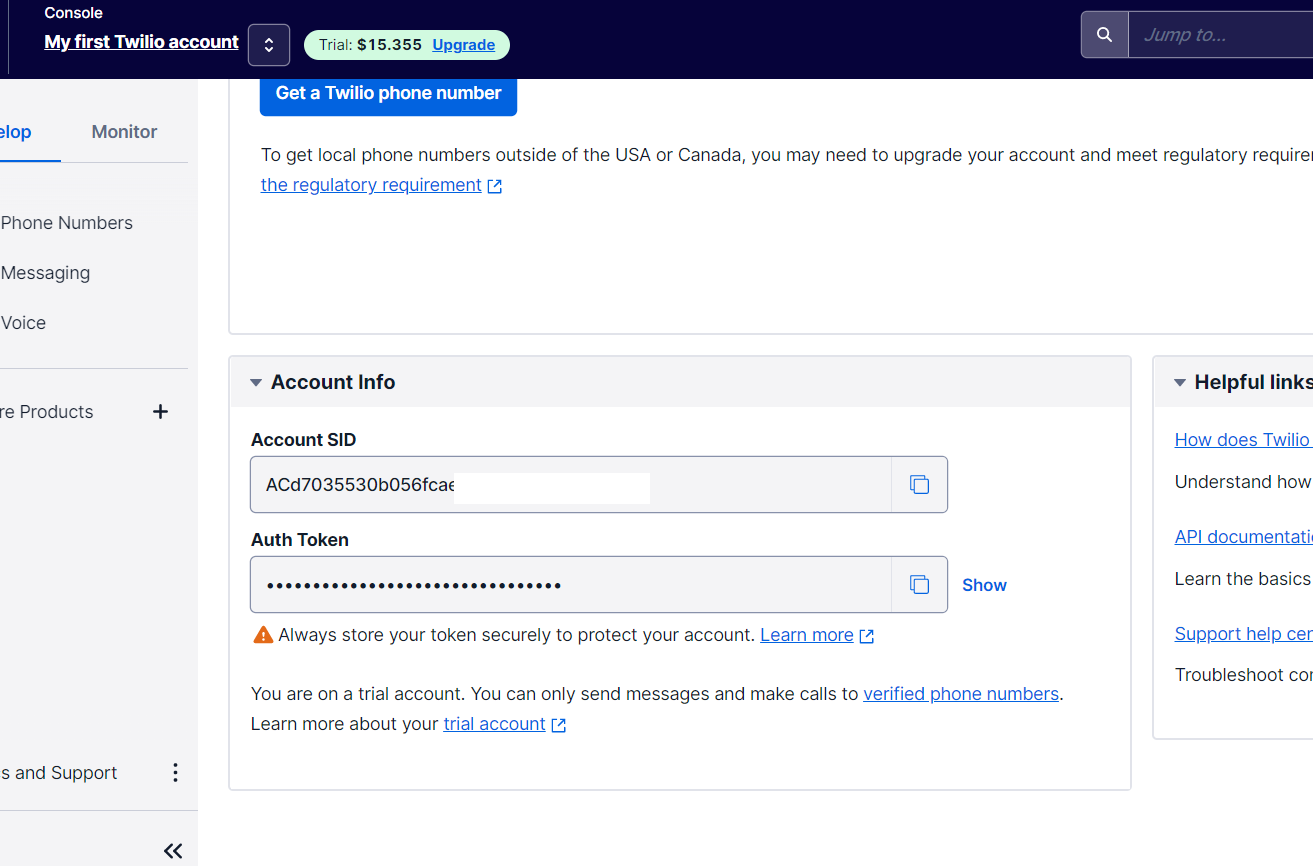
Copy them in a safe place because you will use them later.
Buy a number
You'll need to have a Twilio Phone Number to send SMS messages.
In order to buy one, in the Twilio Console, you need to go to the left side menu, select the "Phone Numbers" option, then "Manage" to finally click "Buy a number".
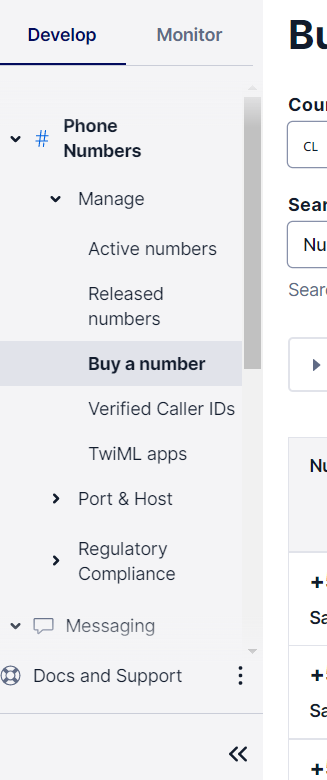
In the filters on the displayed screen, select a country that has the SMS option available, for example, the USA.
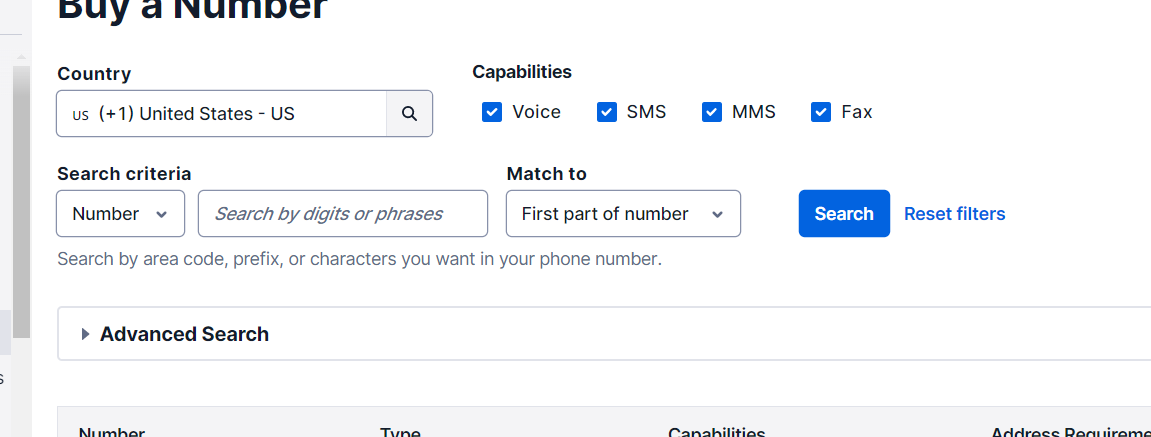
Select one of the available numbers by clicking on the "Buy" option.
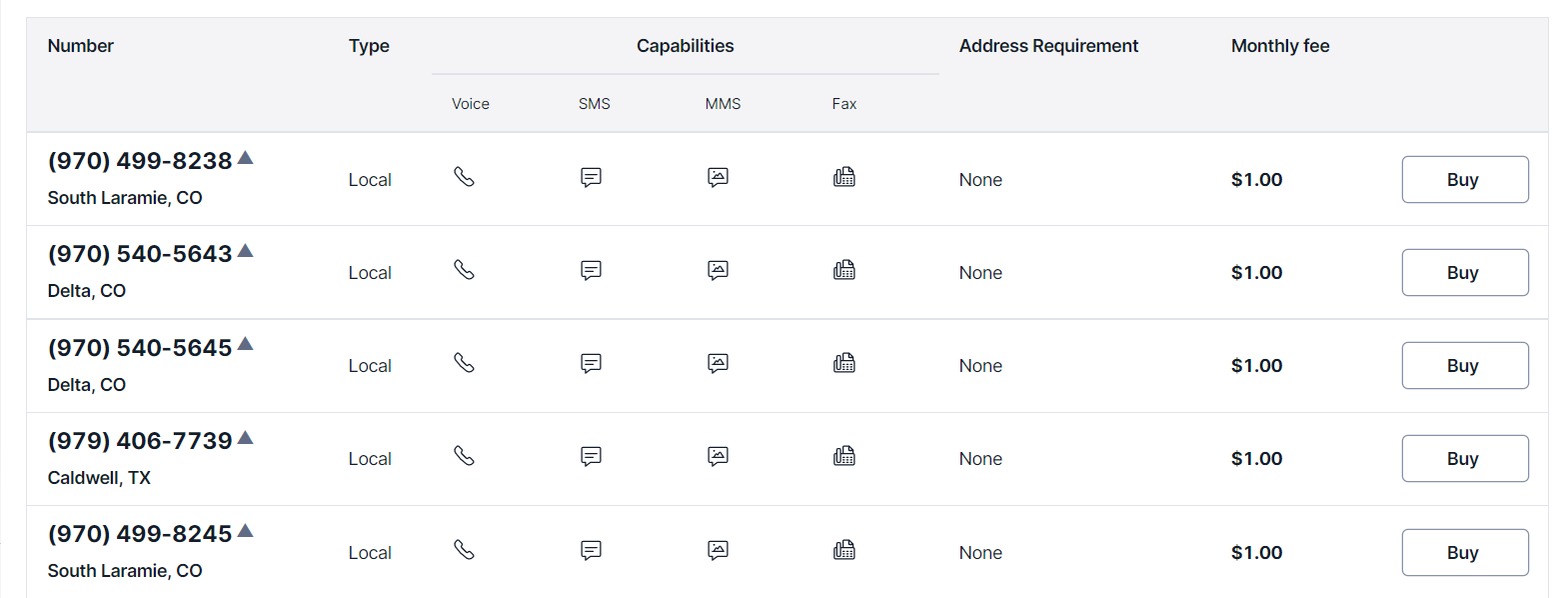
A screen will be displayed indicating the properties of your phone number before buying it. Here, you can verify that the ability to send SMS is available. Click on "Buy" and then click "Buy <phone number>".
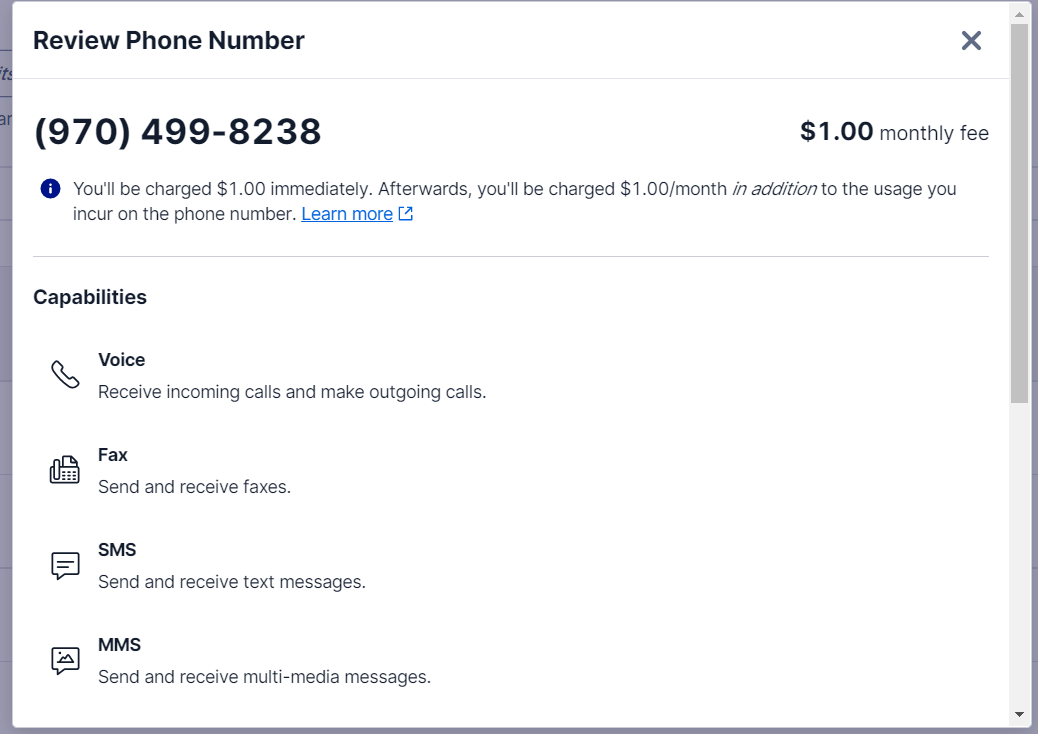
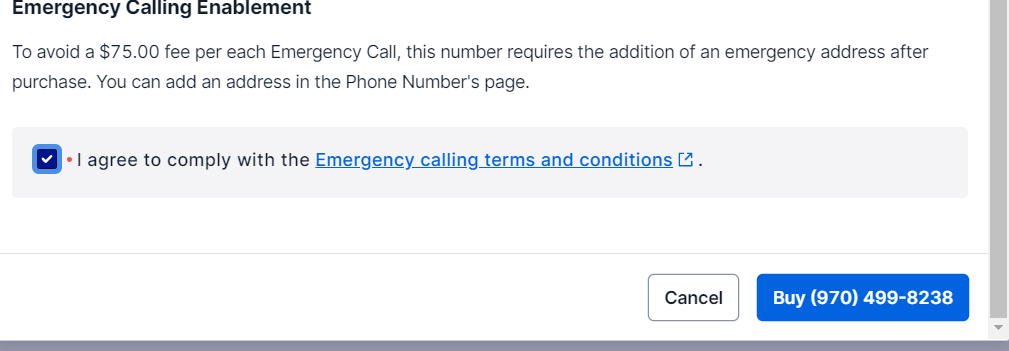
Finally, a screen will be displayed with the confirmation of your new phone number available to use.
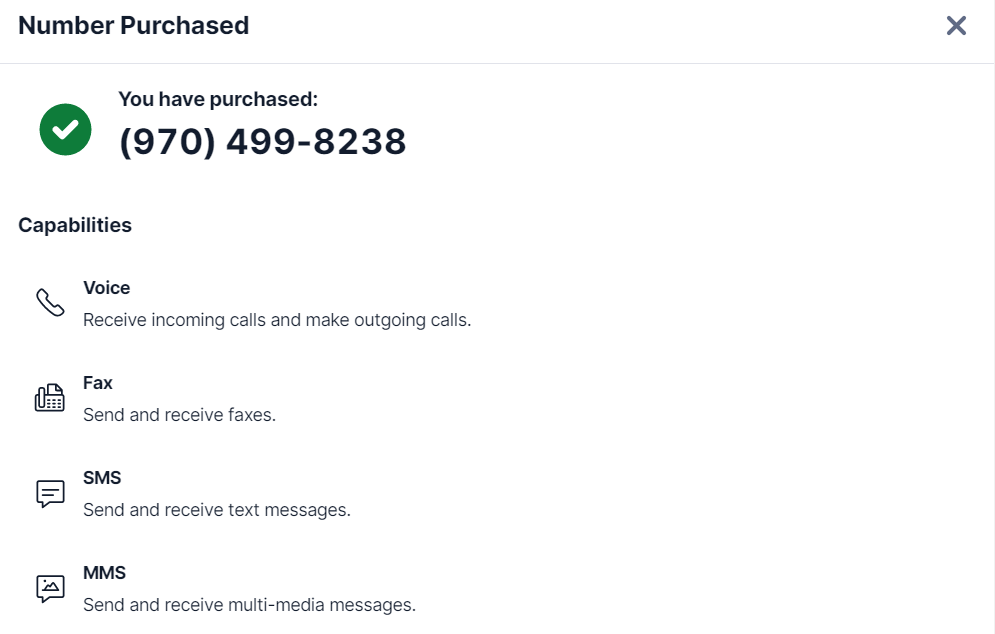
Create a Messaging Service to schedule messages
Next, you will need to create a Messaging Service where you can associate your phone number with the service and then use the service to schedule messages.
In The Twilio Console click on "Messaging" (if you don't see it, click on "Explore Products", which will display the list with the available products, and there you will see "Messaging"). Then, click on "Services". Next, on the displayed page, click the "Create Messaging Service" button to create your service.
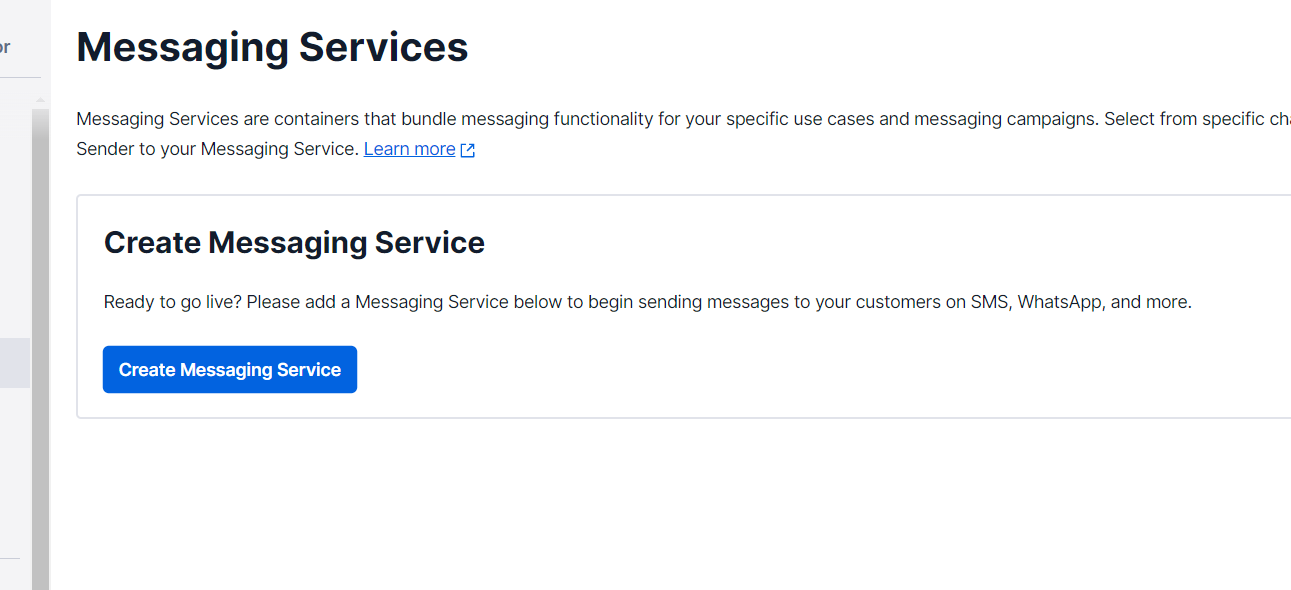
Enter a name that identifies what you will do with your service, select what you are going to use your service for in the available list, and finally click on "Create Messaging Service".
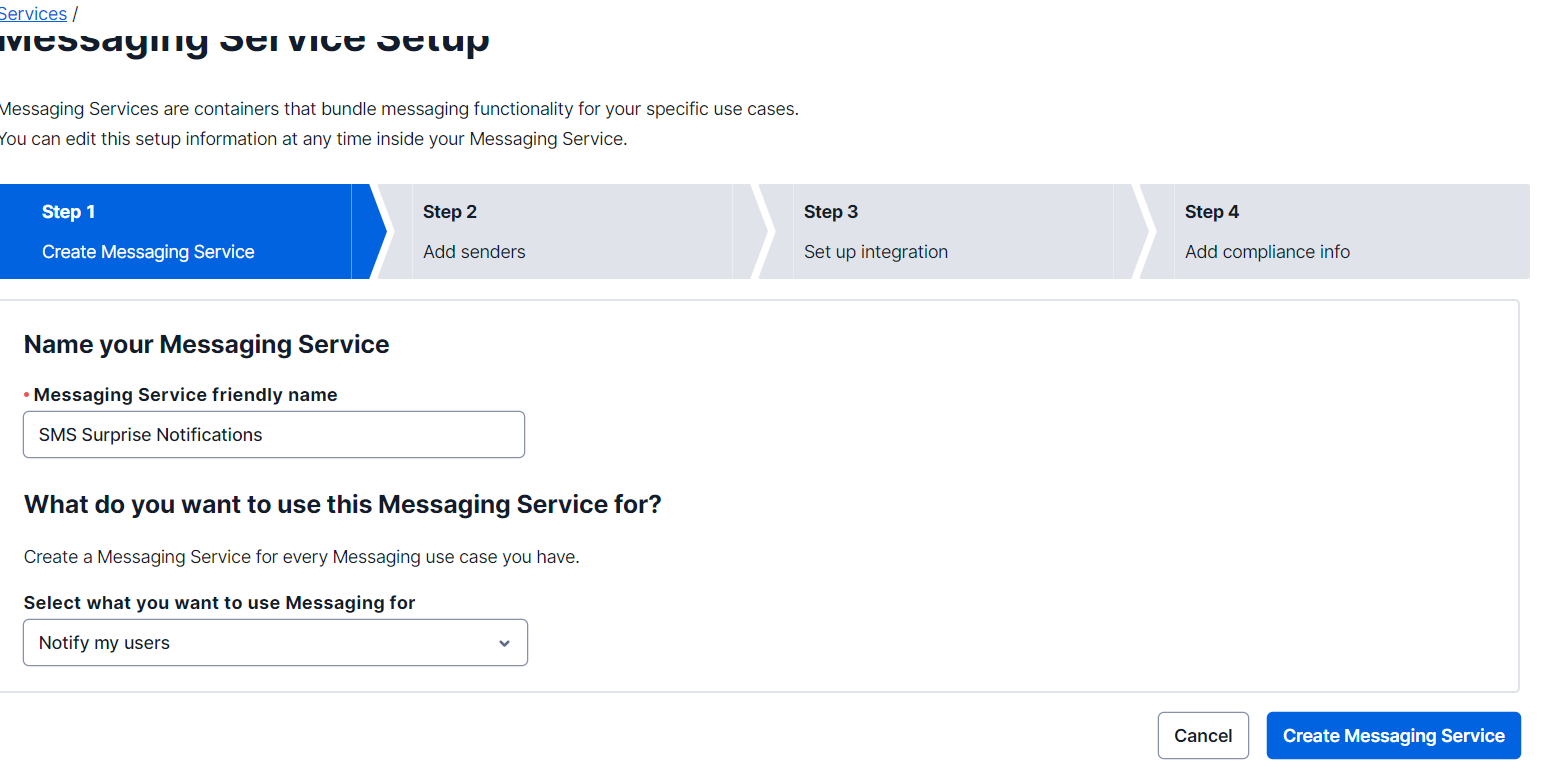
Your service has been created. Now it is time to configure a sender, that is, a phone number from which you will send the messages.
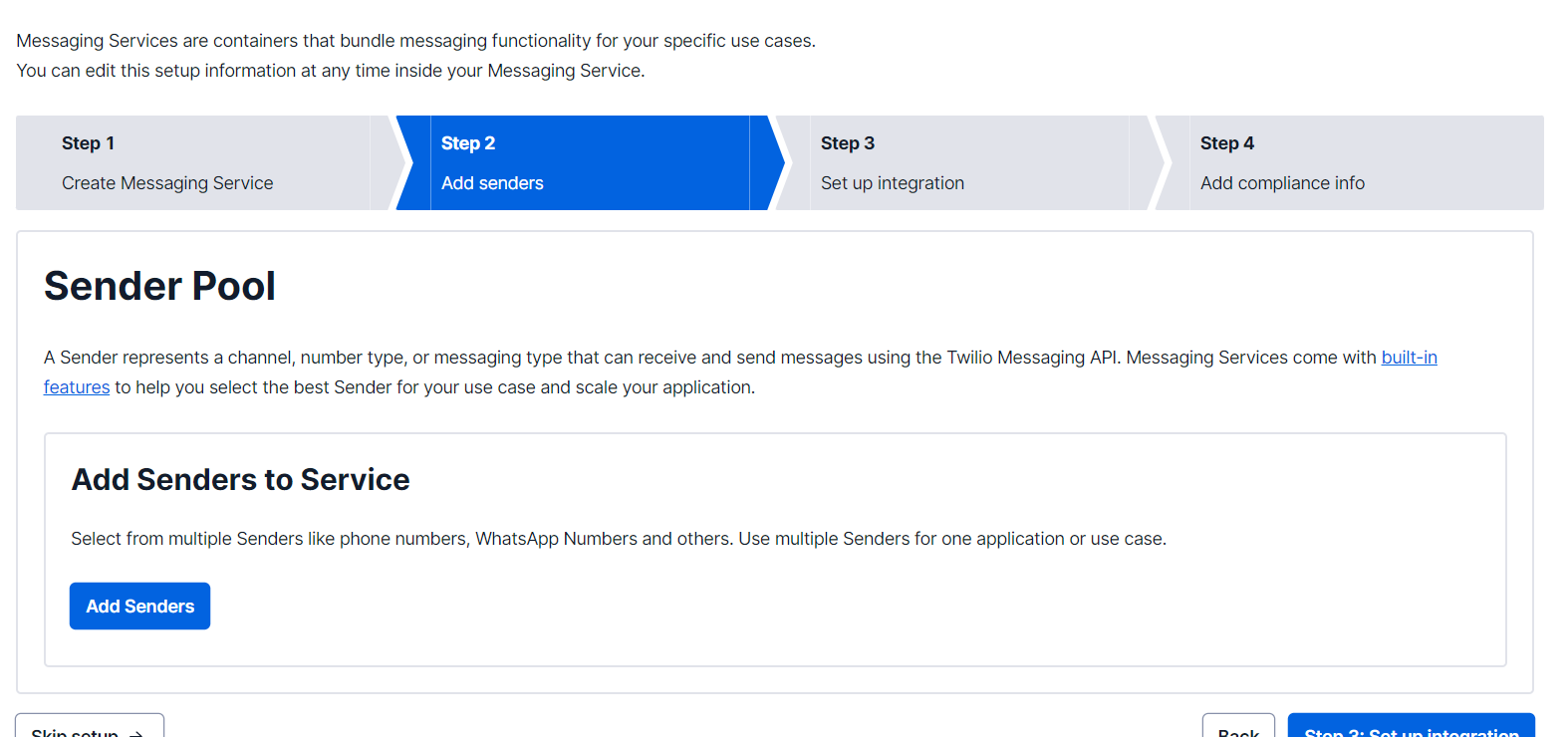
Click on the "Add Senders" button and in the modal, select the "Phone Number" option in the "Sender Type" dropdown, and finally click on "Continue".
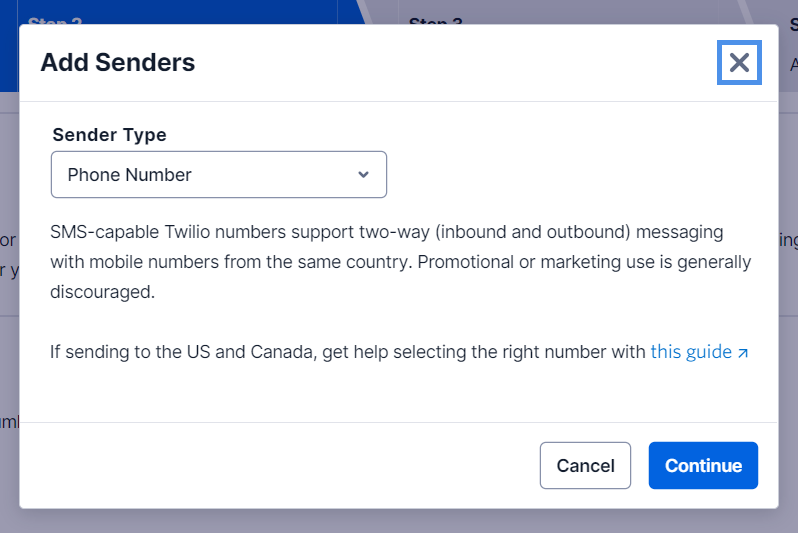
You will see the list of phone numbers available in your account (the one you just bought). Select the checkbox for that number, which appears in the first column, and then click "Add Phone Numbers".
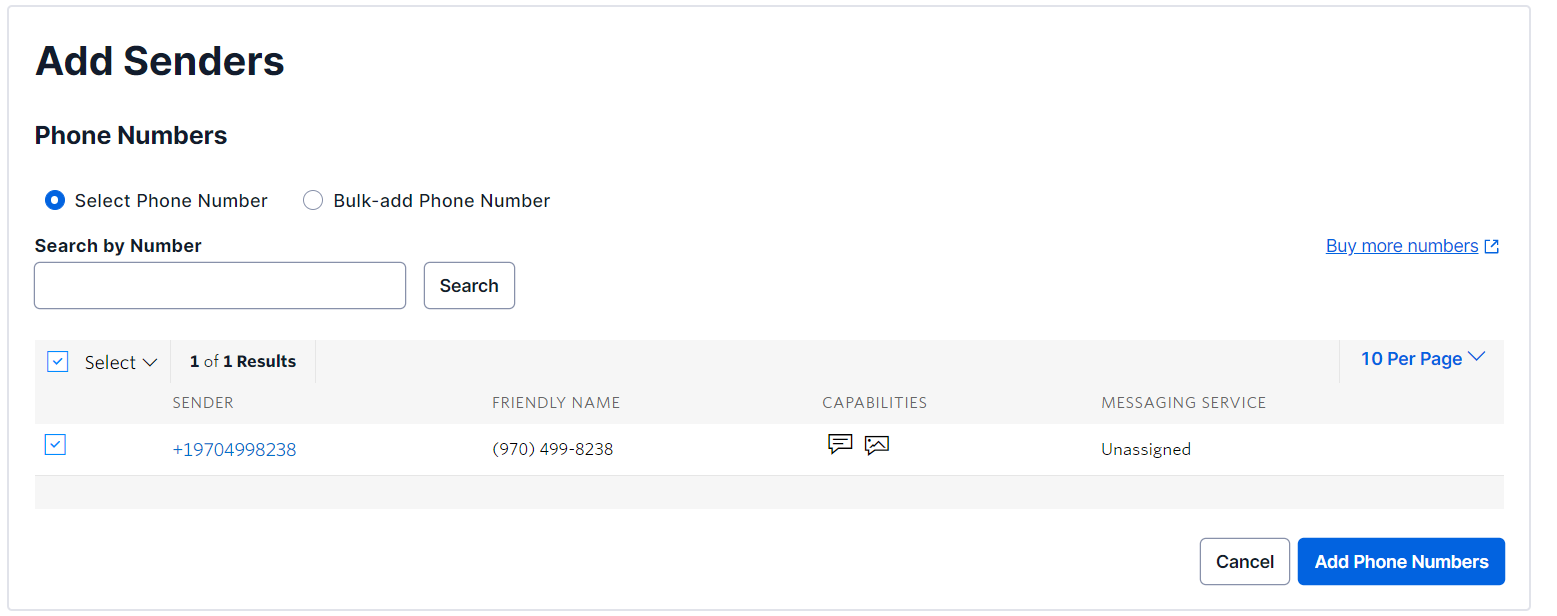
Then, click "Set up integration", where you can configure what to do with incoming messages on your senders and the maximum time your messages can be queued before not being sent (like a timeout).
Select the "Drop the message" option since you won't be doing anything with incoming messages in this project.
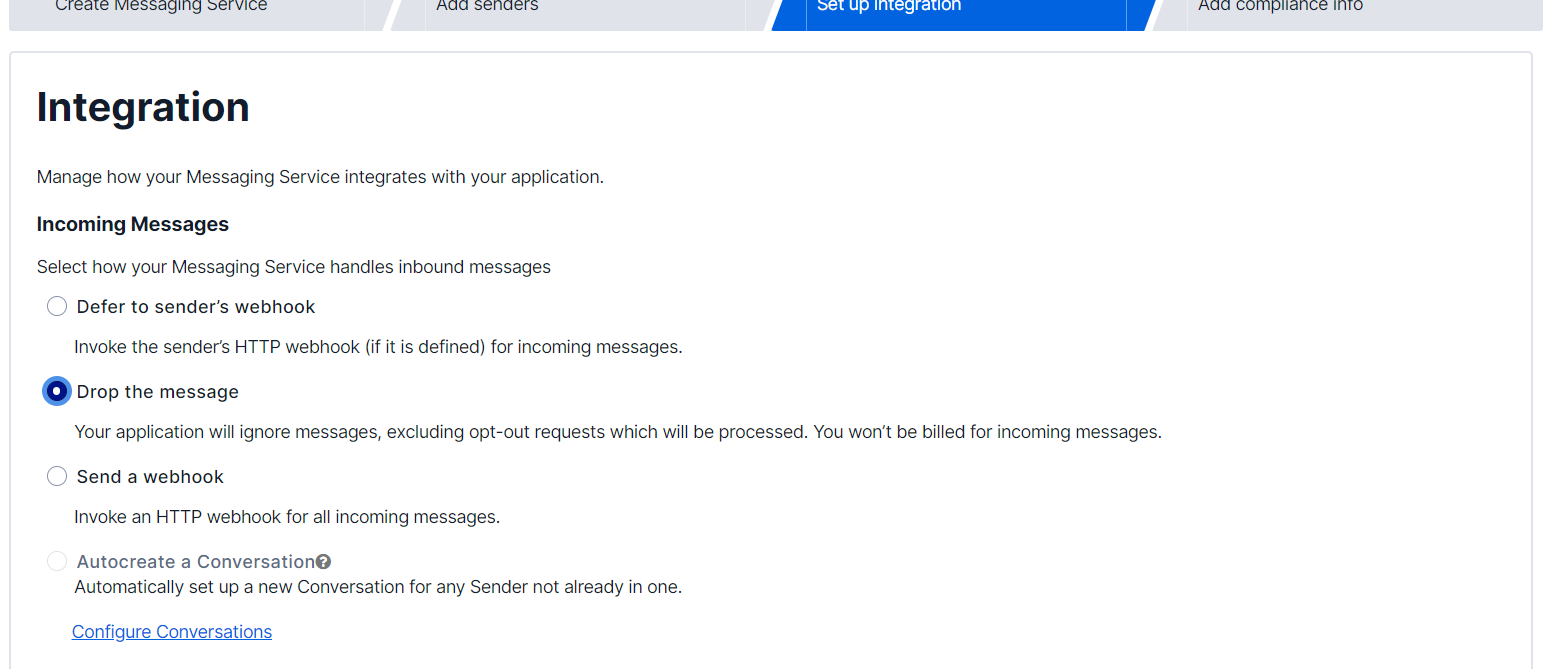
Then click the "Step 4: Add compliance info" button.
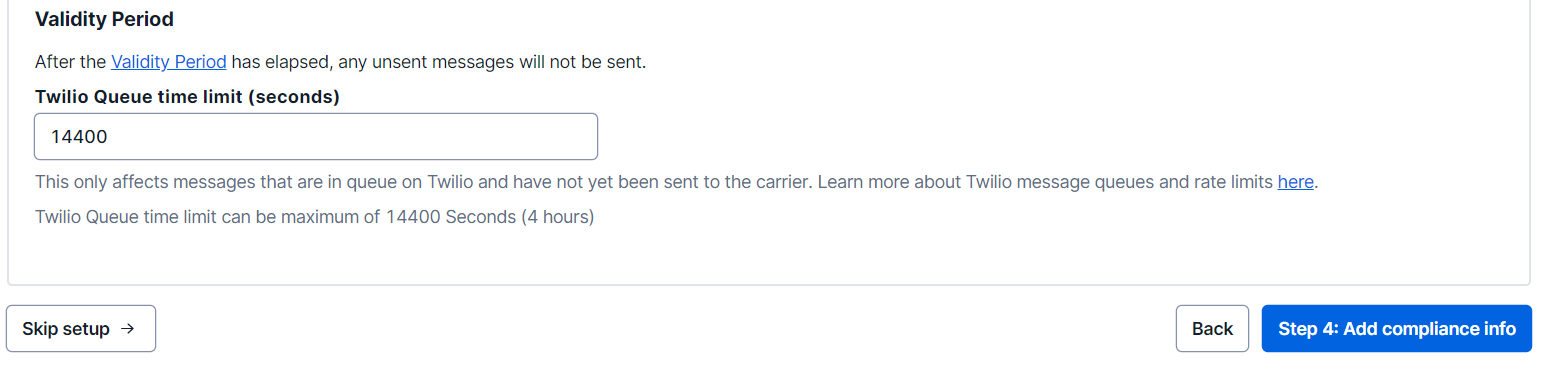
In this form, you should configure topics such as your business profile, among others, to ensure that your usage of messaging is compliant.
In this project, you will skip this step and click "Complete Messaging Service Setup" to finish the process.

After finishing the process, a modal screen will be displayed with the confirmation of this process. Click on the "View my new Messaging Service" button.
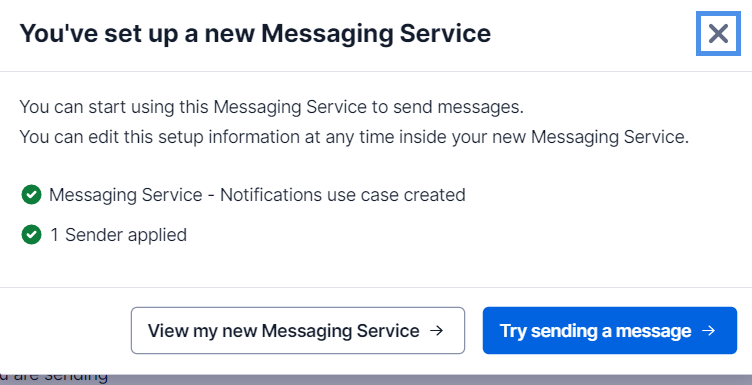
On the screen of your new service, you will see the "Messaging Service SID", which you should copy, since you will use it in your code to send the SMS messages.
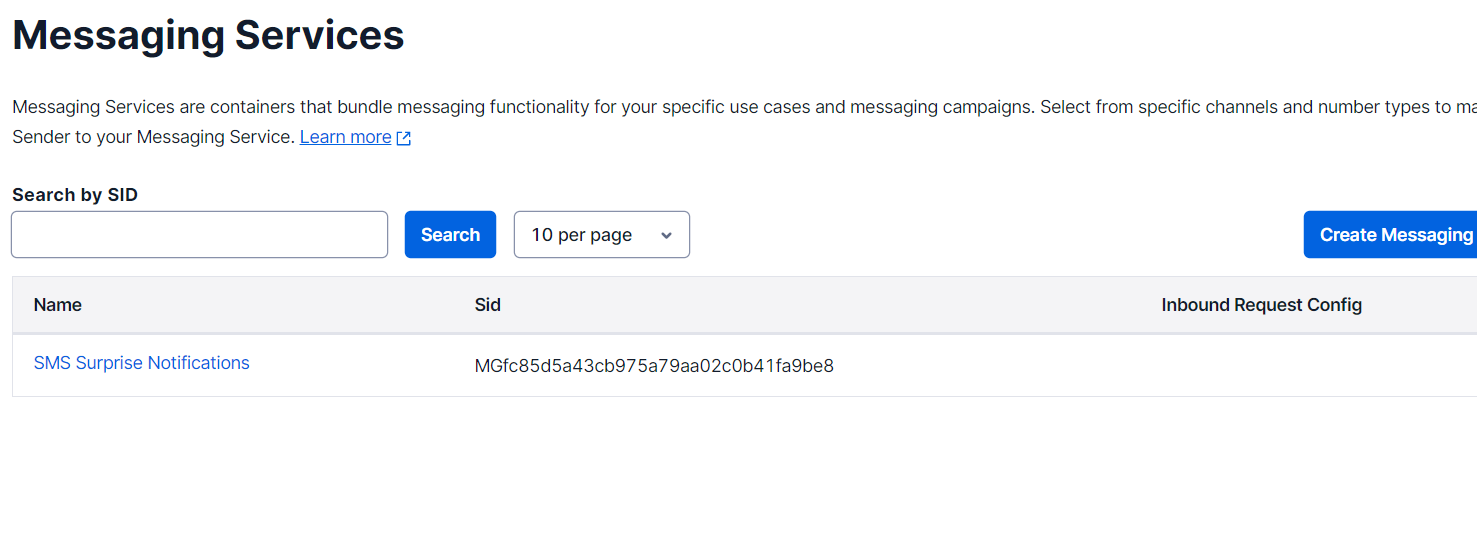
Alright, now that you've set up your Twilio Phone Number and Messaging Service for your project, it's time to set up a file hosting service and your .NET project.
Create a Console Project
You will need to create a console project using the .NET CLI. Open a terminal and run the following commands to create a folder that will contain your project:
Next, create the project with the command below:
Add the API Key in the project
To add the Twilio Credentials to your project, you will use user secrets since it is a good option for local development that is easy to manage, more secure, and for good development practices.
To set user secrets for your project, run the following commands at the command line inside your project's root folder:
Replace <Twilio Account SID>
with the Twilio Account SID, <Twilio Auth Token>
with the Auth Token, and <Twilio Messaging Service SID>
with the Messaging Service SID you copied earlier.
To load user secrets, you must add the Microsoft.Extensions.Configuration.UserSecrets NuGet package. Add the package using the following command:
Install the Twilio library
Now, install the Twilio SDK to be able to send SMS messages.
Configure the project
Now, in the Program.cs file, replace all existing code with the following:
The code above imports all the libraries you'll use in the project.
Next, you are going to add the code that loads the configuration from your user secrets, where you stored the Twilio credentials.
Schedule SMS messages
Next, you have to initialize the Twilio SDK with your credentials (Account SID and Auth Token), create the messages, and schedule them.
In particular, you are going to create 3 messages, one notifying your partner that there will be a surprise tomorrow, another indicating that at 6 pm they must be in a specific place, and finally, one at 11:59 pm telling them that it was an incredible day.
Replace <to number>
with your personal phone number for testing.
Now, run your console project to send your SMS messages:
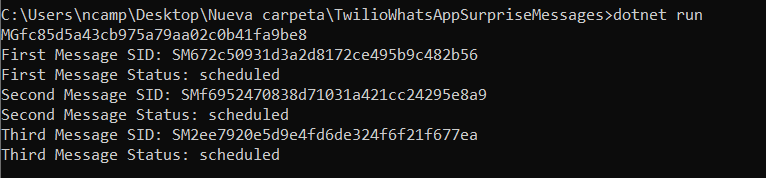
16 minutes later, you should receive three SMS messages similar to the following:
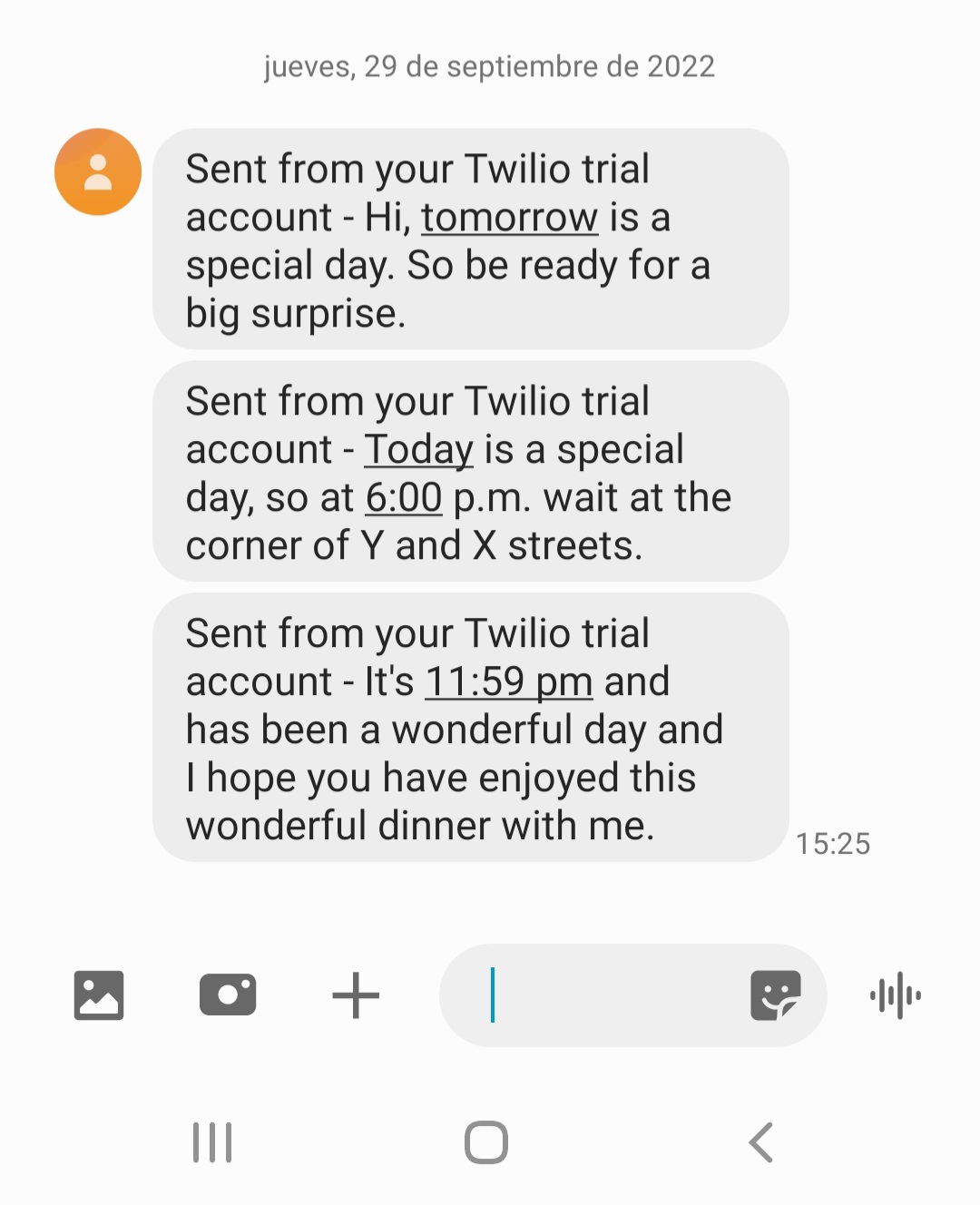
You will have started and ended with surprise messages for your partner, making a special day thanks to scheduled messages through Twilio.
Additional resources
Check out the following resources for more information on the topics and tools presented in this tutorial:
Twilio SDK for C# and .NET – You can explain all the options provided by the Twilio C# SDK.
How to Send Scheduled SMS with C# .NET and Twilio Programmable Messaging – This tutorial shows you how to schedule and cancel scheduled SMS.
Source Code to this tutorial on GitHub - You can find the source code for this project at this GitHub repository. Use it to compare solutions if you run into any issues.
Néstor Campos is a software engineer, tech founder, and Microsoft Most Value Professional (MVP), working on different types of projects, especially with Web applications.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.