How to Send Emails in Rust With SendGrid
Time to read: 4 minutes
If you're coding in Node.js, Python, C#, PHP, Java, Go, or Ruby, then sending an email using SendGrid is pretty trivial. But what about Rust, one of the newest and most popular languages?
Sadly, there isn't a helper library available for it – yet. So, in this tutorial, I'm going to show you the essentials of sending an email using SendGrid's API with Rust.
Prerequisites
To follow along with this tutorial, you're going to need the following:
- Rust and Cargo, along with some prior experience with both
- Your preferred text editor or IDE. I recommend VIM or Visual Studio Code.
- A free SendGrid account. If you are new to SendGrid, click here to create a free account.
- An email address to receive the email
- An email address to send the email which has a verified identity
Set up a new Rust project
The next thing to do is to create a new Cargo package, to save some time and effort in writing the necessary code. To do that and change into the new package (directory), run the following commands.
Add the required Crates
Next, to avoid writing too much code, you're going to need a few crates (external dependencies); these are:
- dotenv: This crate merges variables defined in .env into the application’s environment variables provided by the operating system. Among other things, this ensures that secure credentials, such as your SendGrid API key, are kept out of code
- error-chain: This crate simplifies the use of Rust's error handling functionality
- http: This crate contains common HTTP types
- reqwest: This crate provides a convenient, higher-level HTTP Client
- serde and serde_json: These crates, collectively, serialise responses from the Twilio API into a series of structs, making them easier to work with, programmatically
To add them to the project, along with the required features, run the following commands.
Set the required environment variables
The next thing you need to do is to set 5 environment variables. These are a combination of your SendGrid API key, so that you can make authenticated calls to SendGrid's API, and a sender and recipient for the email; both full name and email address.
Create a new file in the project's top-level directory, named .env, and in that file add the following configuration.
Now, you need to create and retrieve a SendGrid API key, if you don't already have one. To do that, log in to your SendGrid account and under Settings > API Keys click Create API Key.
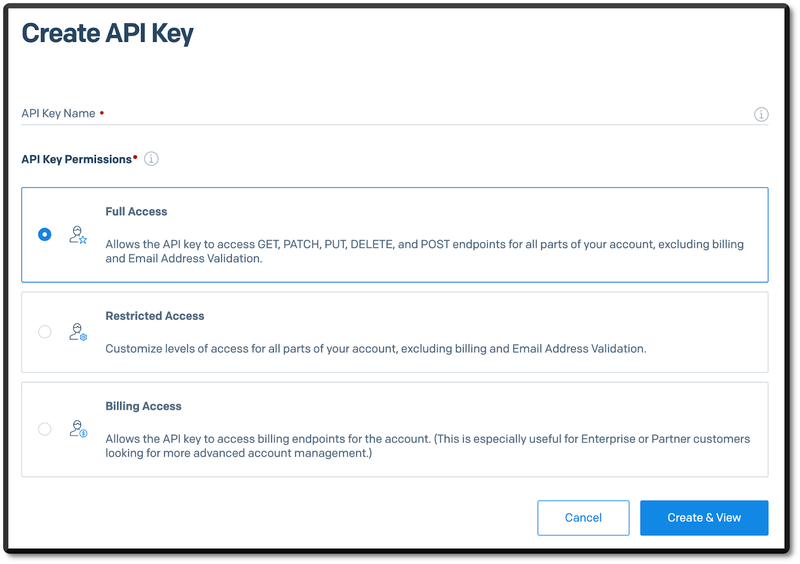
In the form that appears, enter a meaningful name for the key in the API Key Name field and click Create & View.
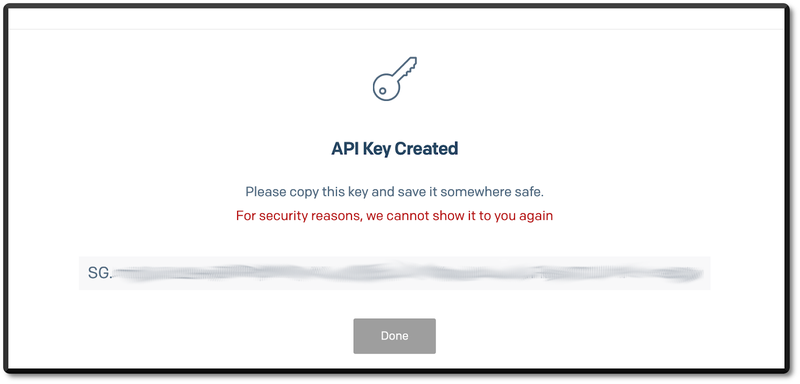
The SendGrid API key will now be visible. Copy and paste it into .env in place of the <<SENDGRID_API_KEY>>
placeholder.
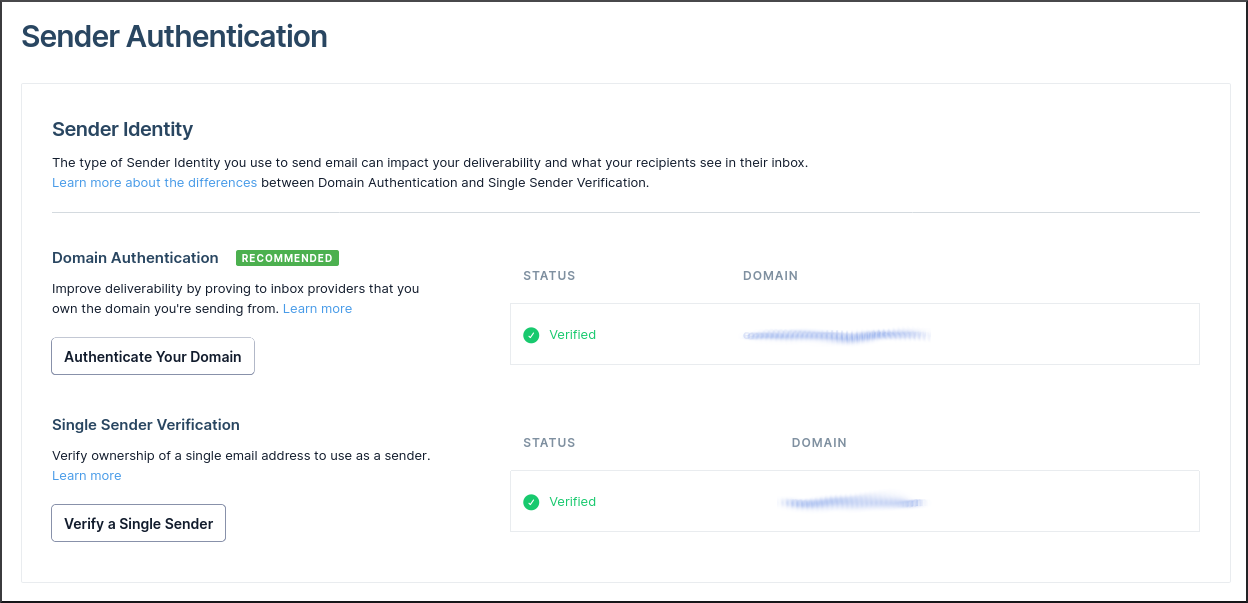
Then, in the left-hand side navigation menu, click Settings > Sender Authentication. There, copy one of the email addresses from the Single Sender Verification section, and paste it into .env in place of the placeholder for SENDER_EMAIL
.
Then, replace the placeholder for SENDER_NAME
with the sender's full name. After that, replace the RECIPIENT_NAME
and RECIPIENT_EMAIL
placeholders with the name and address of the email's recipient.
Write the Rust code
Now, let's write the Rust code! Replace the existing contents of src/main.rs with the code below.
The code starts by defining a small Struct, User
, to simplistically model an email sender and recipient. Then, the main()
function is defined. It starts off by:
- Loading all of the environment variables defined in .env;
- Initialising the API key (
api_key
) to authenticate against SendGrid's API, and the email's sender (sender
) and recipient (recipient
), from the five environment variables, defined earlier in .env.
Following that, using Serde JSON's json macro, the request's body (body
) is defined. It stores the email's sender, recipient, subject, and the plain text and HTML versions of the email's body.
Then, a new Reqwest Client object (client
) is defined, which handles making the call to SendGrid's API to send the email. On that object, it tells it to make a POST request to https://api.sendgrid.com/v3/mail/send, with a JSON body using the contents of body
.
The request uses the bearer_auth() method to set a Bearer token to successfully authenticate against SendGrid's API, and sets the response's accepted content-type to application/json
.
The request is then sent, with the response being stored in response
. The response's status code is checked to see if it was an HTTP 200 (OK), 201 (Created), or 202 (Accepted). If so, the user is notified that the email was successfully queued to be sent by SendGrid.
If the status code was not one of these three values (such as an HTTP 400 (Bad Request) or 404 (Not Found)) the response's body is printed to the terminal, as it contains the details about why the email was not accepted to be sent.
Test that the application works
With all of the code and configuration in place and Crates installed, it's time to check that the code works. To do that, run the following command in the top-level directory of the project.
All being well, you should see the crates being installed followed by Email sent!
printed to the terminal. A little while later, the email should arrive in your inbox, which you can see below.
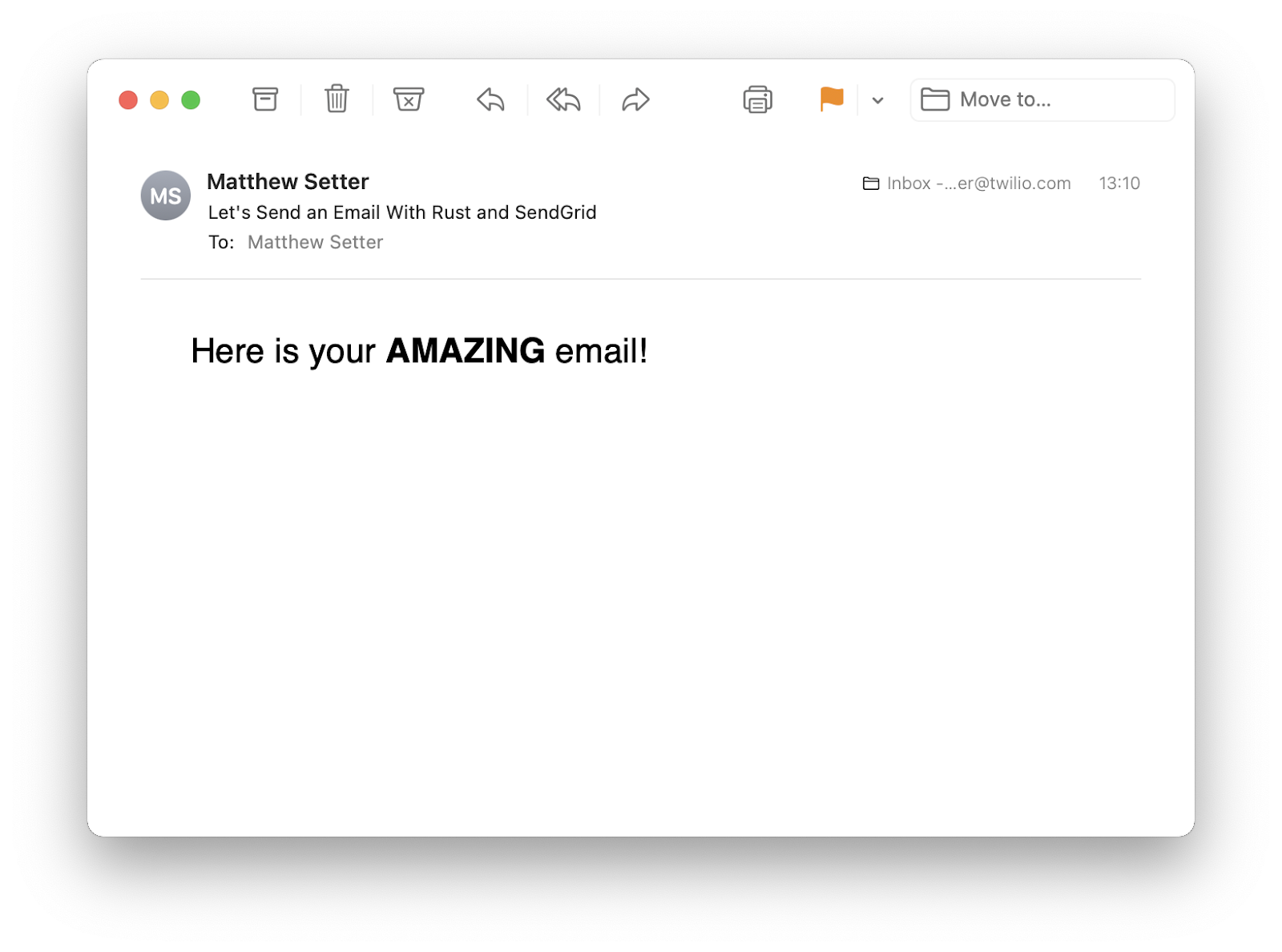
That's how to send emails in Rust with SendGrid
There's not a lot to it, but I thought that, as an avid Rust user, it was worth stepping through all the same. How would you have implemented the solution? Share your thoughts with me on LinkedIn.
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. You can find him at msetter[at]twilio.com. He's also on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.