Use Azure Functions and Twilio SMS to know: Will it Rain Today?
Time to read:
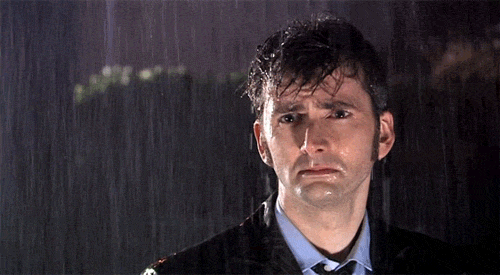
Will you need an umbrella today? Don't know? That's easy to fix by looking out the window or by using Azure Functions and its Twilio SMS output binding. In this post, I'll show you how with just a few lines of code you can have a text message arrive each morning which lets you know if it might rain that day.
You can build along with me by signing up for free Azure and free Twilio accounts.
Create an Azure Function
We'll start by creating a new Azure Function App in the Azure Portal.
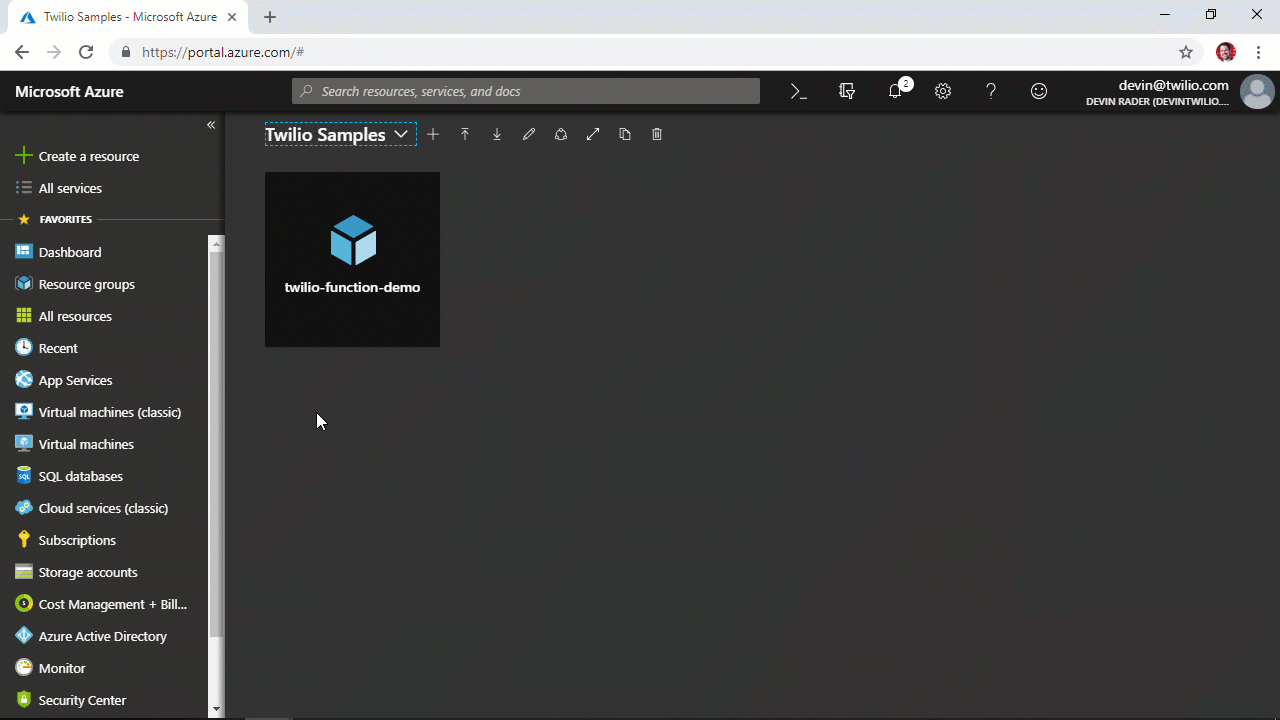
Once created continue configuring the Function App by adding environment variables to hold our Twilio credentials. Open the Applications Settings for the Function App.
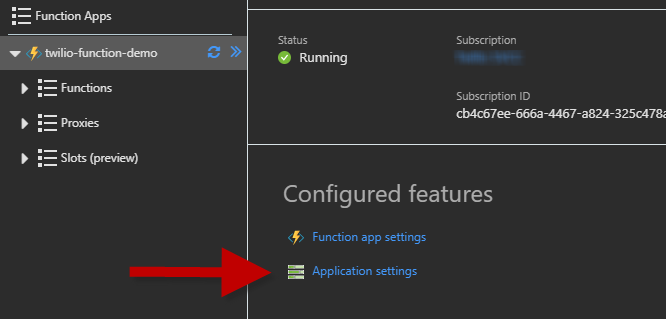
Add two new keys named TwilioAccountSid
and TwilioAuthToken
, setting their values to your Twilio AccountSid and AuthToken respectively. You can find your Twilio credentials in the Twilio Console.
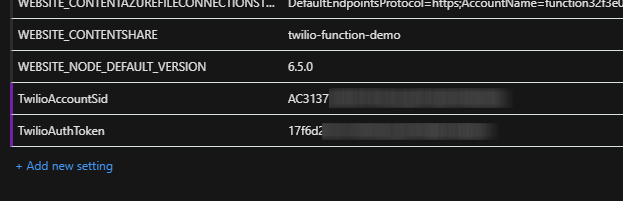
Save the Application Settings and then create a new Function using the Timer template.
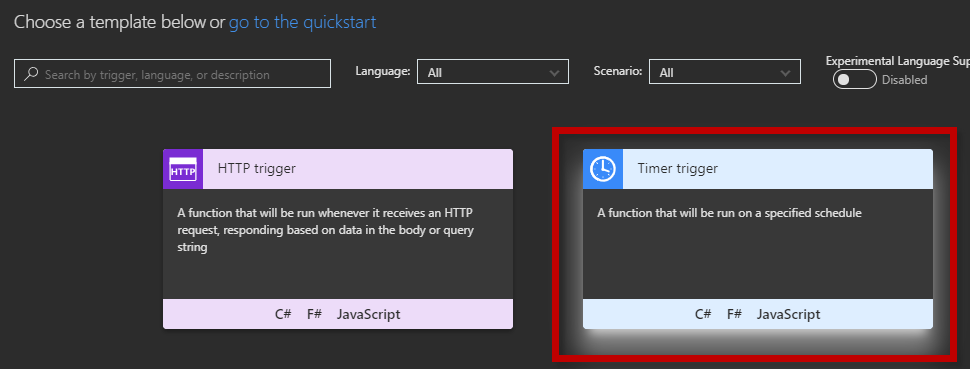
Use a cron expression to configure the timer to run once per day.
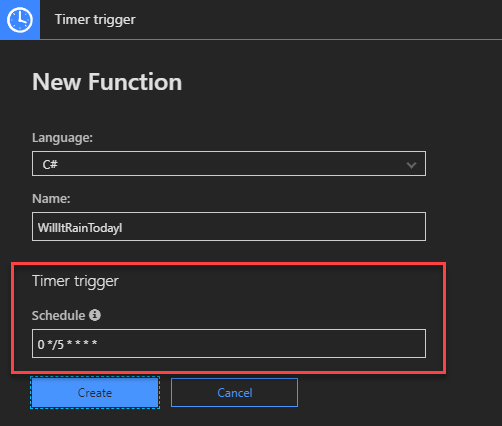
With the Function created, open the Integrate tab for the Function and add Twilio SMS as a new Output:
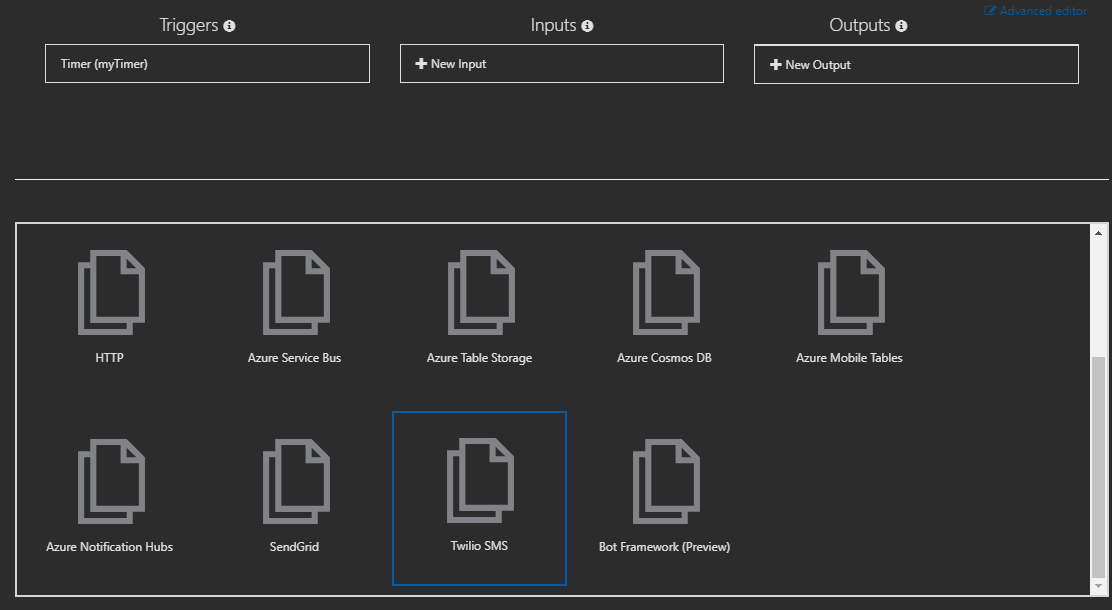
Configure the Output settings with the TwilioAccountSid
and TwilioAuthToken
environment variable keys we created earlier, a Twilio phone number to send From, and your own phone number to send To.
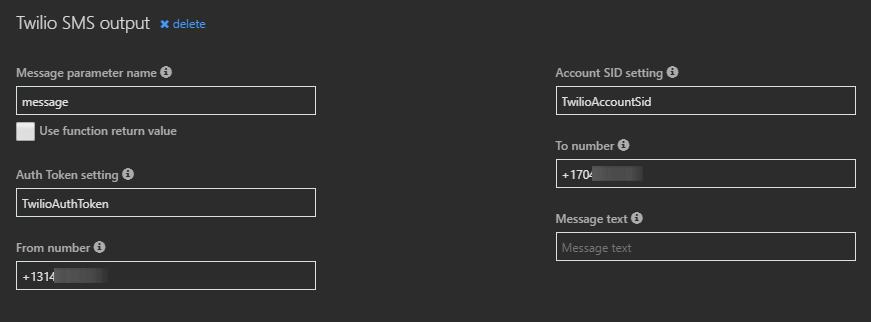
Return to the Function code and add references for the Twilio library:
In the Run function, add a new out
method parameter of type SMSMessage
named message
. Next, within the method create a new instance of the SMSMessage
type and set its Body
property to Will it rain today?
.
Test the Function and you should get a text message asking you if it will rain.
Next, we need to answer the question we just asked: Will it rain today? To do that we'll scrape the website willitrainin.com, grabbing the simple Yes or No value it displays on its city-specific forecast pages and appending that to the text message.
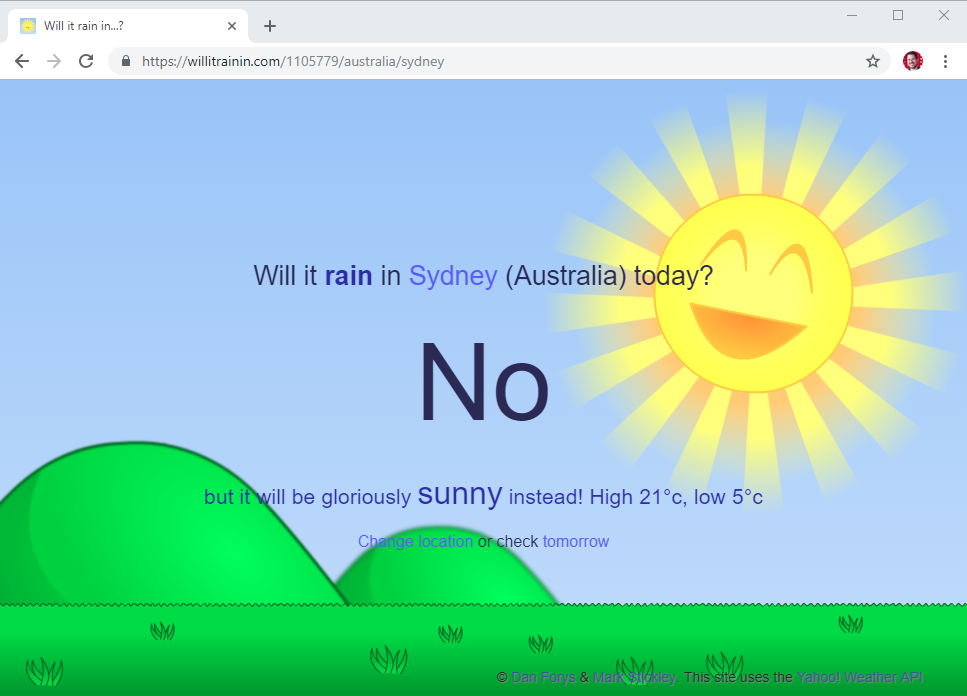
Add a new method called WillItRainIn
in run.csx
and use an instance of HttpClient
to fetch the Will It Rain In page for your local area:
We'll parse the Yes or No text from the HTML received by the HttpClient using the HtmlAgilityPack library.
Add the HtmlAgilityPack to the Function by creating a project.json
file and adding the libraries NuGet package reference as a dependency.
Save the file and Azure will automatically install the package for you.
Add a using
statement for the library in the run.csx
file.
Load the HTML into an HtmlDocument
and then use the SelectSingleNode
method to search for a <p> tag marked with the result
class. Put that tag's content into the result
variable.
Now we'll add a call to the WillitRainIn
method to our main Run method.
Because we're using HttpClient's asynchronous methods, we've marked the WillItRainIn
method itself as async
. That means in order to use it in our Run method we need to modify it to also be async.
Add the async keyword to the Run method signature and change the default return value from void
to Task
. Additionally, because async methods cannot include out parameters in their method signatures, we have to change the message
parameter to be a reference parameter (by removing the out
keyword) wrapped with an IAsyncCollector
interface.
public async static Task Run(TimerInfo myTimer, IAsyncCollector<SMSMessage> message, TraceWriter log)
Add the async call to the WillItRainIn
method. Create a new SMSMessage, set its Body property and then pass it to the IAsyncCollector.
Run the function again and now the text message should contain both the question and the answer. And now with just a few lines of code, you can know every day if you'll need to grab your umbrella! Let me know what you're building on Azure Functions - drop me a line on Twitter @devinrader or send an email to devin@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.