How to Send a vCard using PHP and SMS
Time to read: 4 minutes
If you need to share a contact's details with a friend, the last thing that you want to do is to copy their details manually, one by one, from your contacts app into an SMS. And your friend's not going to enjoy copying them, one-by-one, from the SMS into a new contact on their phone either.
The same goes for sharing contacts from PHP applications. Copying details from a website into an SMS and sending it is very tedious.
Gladly, no one needs to, as contacts can be exchanged using vCards!
If you're not familiar with them, vCards are, effectively, an electronic form of a business card. They can contain all of the contact details (or properties) that you'd likely expect to see, such as first and last names, nicknames, photos, anniversaries, roles – and so much more.
In this tutorial, I'm going to show you how to create one with PHP and then send it to a contact in a Twilio-powered SMS.
Prerequisites
To follow along with this tutorial, you will need the following:
- Composer installed globally
- PHP version 8.1 or above
- A Twilio account (free or paid). If you are new to Twilio, click here to create a free account
- A mobile/cell phone that can receive SMS
- ngrok and a free ngrok account
- Your preferred text editor or IDE (I recommend PhpStorm)
Create the project directory structure
The first thing to do is to create the project's directory structure. So, where you store your projects, run the following commands to create the project directory and change in to it.
If you're using Microsoft Windows, run the following commands instead.
The project directory contains a directory named public, where the sole PHP file will be created. In that directory, is another directory called assets. This directory is where the vCard will be written to, when created.
Install the required dependencies
Now, you need to install the 3 required dependencies; these are:
- sabre/vobject: This package simplifies creating vCard files.
- Twilio's PHP Helper Library: This package simplifies interacting with Twilio's APIs, making it pretty trivial to send an SMS.
- PHP dotenv: This package helps us keep environment variables and other secure credentials out of code, yet still be simple enough to work with.
To install them, run the following command.
Set the required environment variables
Now, you have to set several environment variables. These are:
- Your Twilio Account SID and Auth Token: required to make authenticated requests to Twilio's APIs.
- Your Twilio phone number: so that the correct account can send the SMS.
- The ngrok Forwarding URL: This is the public-facing URL of the PHP application. It needs to be publicly available so Twilio can retrieve the generated vCard.
- Your phone number: So that the application knows which phone number to send the SMS to.
These will be stored in a new file, in the top-level project directory, named .env. Create the file with your preferred text editor or IDE and then paste the configuration, below, into the file.
Paste your phone number in place of the placeholder for YOUR_NUMBER
.
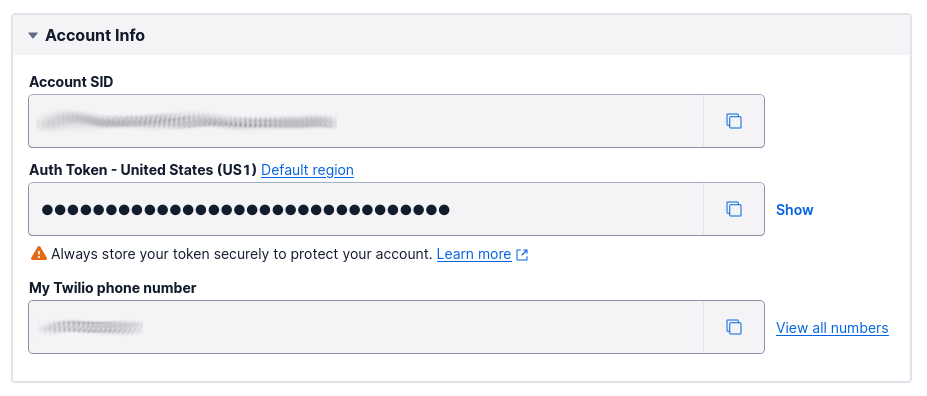
Then, open the Twilio Console in your preferred browser, and from the dashboard's Account Info panel, copy your Account SID, Auth Token, and Twilio phone number and paste them into .env in place of the placeholders for TWILIO_ACCOUNT_SID
, TWILIO_AUTH_TOKEN
, and TWILIO_NUMBER
respectively.
The NGROK_URL
placeholder will be replaced toward the end of the tutorial.
Write the PHP code
Now, it's time to write the code. In public, create a new file named index.php. Then, paste the code below into it.
The code starts off by loading the environment variables defined in .env into PHP's $_ENV
and $_SERVER
superglobals. The call to $dotenv->required()
ensures that the environment variables listed in the array passed to the call are defined and have a value.
After that, a new VCard
object ($vcard
) is initialised. The vCard specification lists quite a number of properties, but the code only sets five:
FN
: The contact's full nameORG
: The name of the organisation that the contact works forTEL
: The contact's telephone numberTITLE
: The contact's title or identifierURL
: A URLBDAY
: The contact's birthday
Then, using PHP's file_put_contents() function, a vCard file is created from $vcard
in public/assets, named twilio.vcf. Then, a Twilio Client object ($twilio
) is initialised with your Twilio Account SID and Auth Token. After that, the call to $twilio->messages->create()
sends an SMS to the number stored in $_ENV['TWILIO_NUMBER']
. During this process, Twilio will retrieve the vCard file from the PHP application and attach it to the message.
If you're curious about what the vCard file looks like, open it in your text editor or IDE, where you should see something similar to the following.
Test the application
With the code written, it's time to test that the application works. To do that, first start the application, by running the command below.
Then, start ngrok running to provide a publicly facing URL to the application, by running the following command.
From the terminal output, copy the Forwarding URL and paste it into .env in place of the placeholder for NGROK_URL
.
Then, run the command below, after replacing the placeholder with the ngrok Forwarding URL.
You should see the following output in the terminal.
Shortly after that, you should receive an SMS with the vCard attached, similar to the screenshot below (allowing for my German mobile).
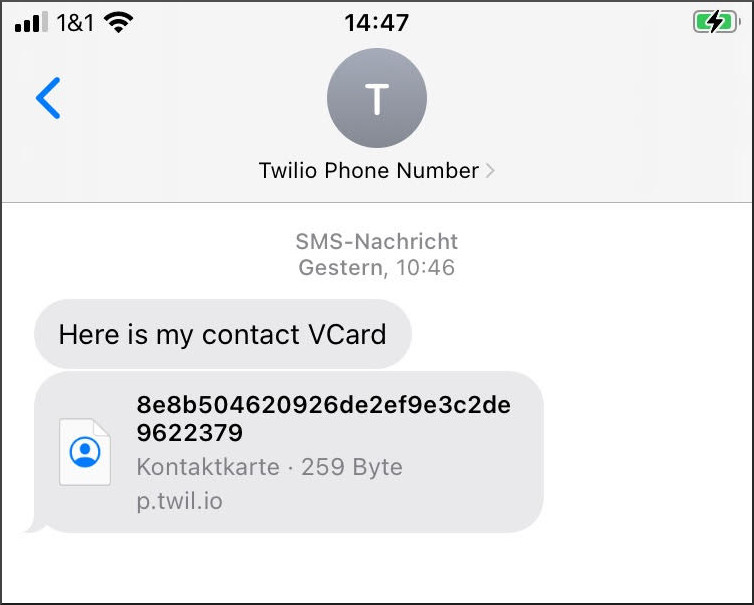
After you click on the vCard, it will download and open in your phone's contact application, similar to the screenshot below.
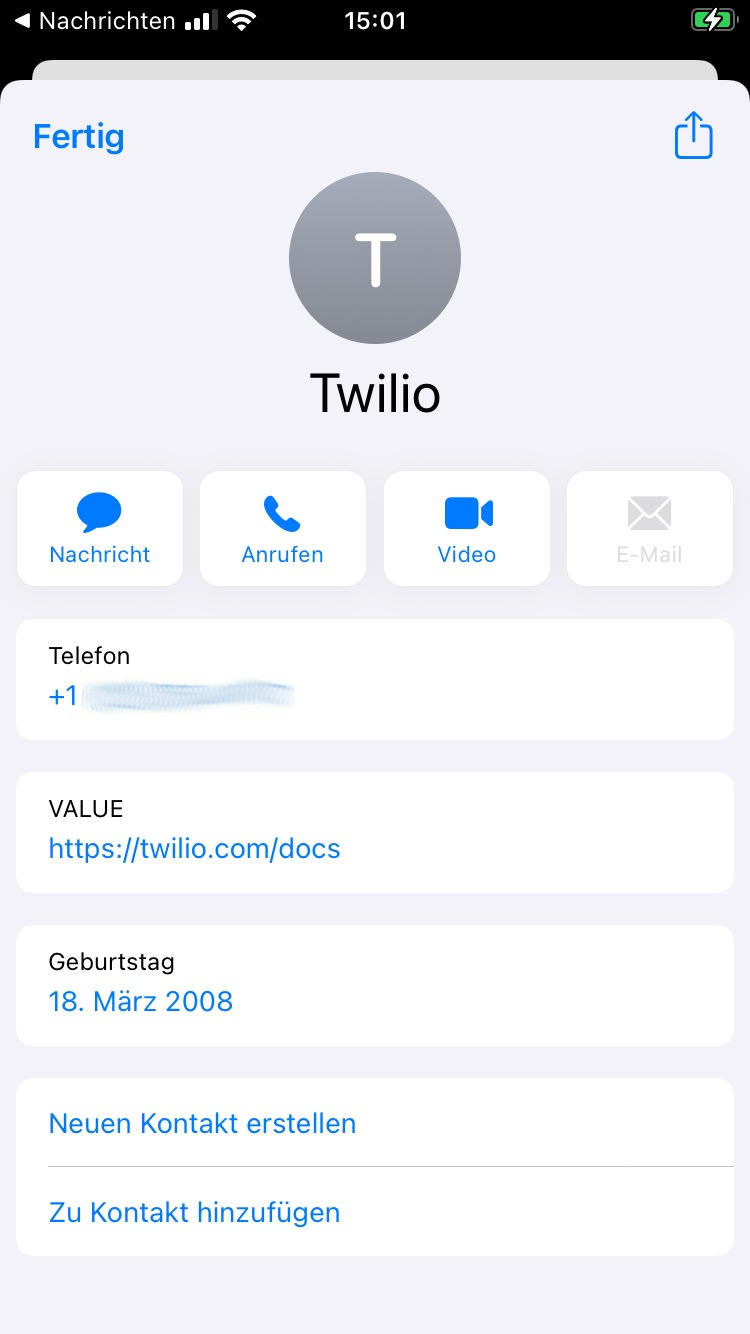
That's how to send a vCard with Twilio SMS and PHP
Now, if your users need to send contacts to other people, they can now send a vCard quickly and easily. No longer do they need to copy and paste details into an SMS or an email.
Do you see yourself using them in your PHP applications? Let me know on Twitter. I can't wait to see what you build!
You can find the entire code for this tutorial in the accompanying GitHub repository if you're interested.
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Docker Essentials. You can find him at msetter[at]twilio.com. He's also available on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.