How to do phone verification in Go with Twilio Verify
Time to read:
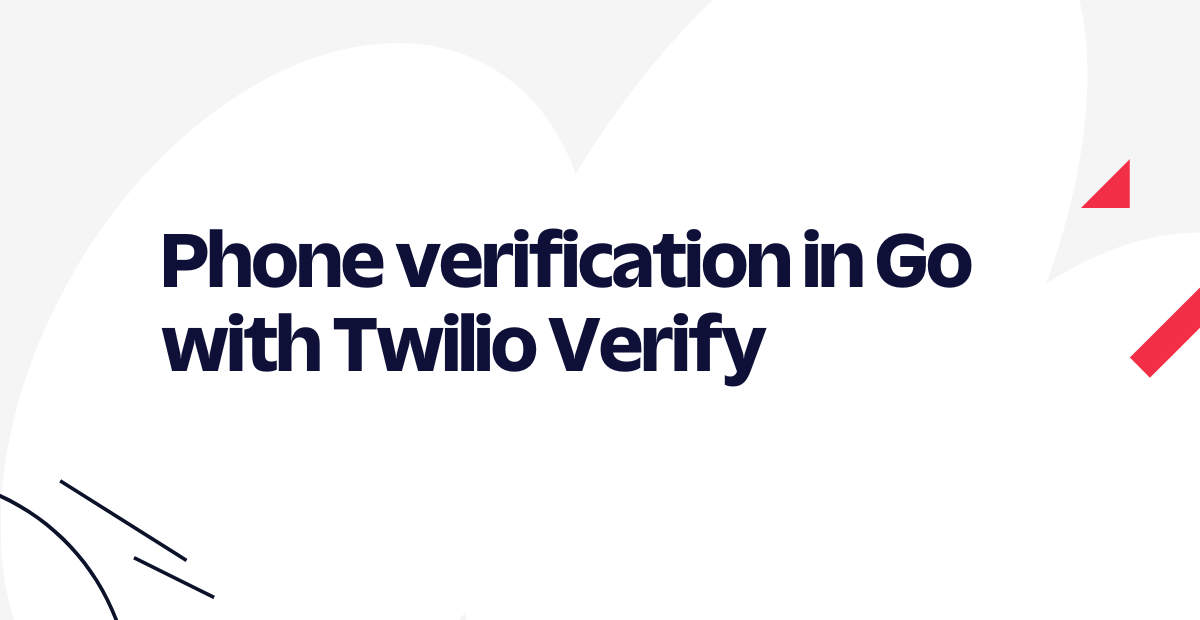
Phone verification is an essential part of the user onboarding process: whenever you collect new contact methods from users you should make sure those are valid. You can also use a similar workflow to authenticate users on an ongoing basis with a one-time passcode (OTP) sent to the user's phone. This is a user-friendly way to do either primary or two-factor authentication (2FA).
This blog post will show you how to send an SMS OTP in Go using Twilio's Verify API. Best of all, once you implement the code you can send an OTP via WhatsApp, voice calls, and emails with one parameter change.
Prerequisites for sending an SMS OTP
To follow along with this blog post you'll need:
- A Twilio Account. Sign up or sign in.
- A Twilio Verify Service. Create a Verify Service in the console.
- Go. Follow instructions in the documentation to download and install on your machine. Verify your install by running
go version
in your terminal. I'm on version go1.18.4.
Create a new Golang project to send one-time passcodes
Using your command prompt, navigate to your home directory. For new Go installations run:
Linux and Mac:
Windows:
Create a new folder for your verification project and change into the project folder by running the following commands; I named mine verify-go
:
Initialize a new module by running the following command. You can name it whatever you want, but I went with verify
:
Download the Twilio Go Helper Library:
Finally, create a new file where you can start writing code! I named mine verify.go
. Add the following code to set up the imports and instantiate the Twilio client:
Set your TWILIO_ACCOUNT_SID
, TWILIO_AUTH_TOKEN
, and VERIFY_SERVICE_SID
as environment variables using the respective details from your Twilio account. You can find these in the Twilio Console.
Send the OTP
Next, add the following function to the bottom of verify.go. This code will send the OTP by calling the Verification endpoint. For this example, "sms" is hard coded as the channel but you could make this dynamic to accept "call", "whatsapp" or "email"! Learn more about channel options in the documentation.
Check the OTP
Next add the checkOtp()
function below to the end of verify.go, which checks the OTP that is sent to the user. The function uses the command prompt and Go's fmt.Scanln
to accept user input as a simple interface. In production you would build an OTP input modal or page in your site interface to accept the input.
This code waits for user input to get the passcode which calls the Verification Check endpoint via the CreateVerificationCheck()
function. The code then checks to make sure that the status is approved
. If the user provides an incorrect code, the status will remain "pending".
Run the code to test the verification lifecycle
Finally, add a main()
function at the end of verify.go to bring everything together. Replace your phone number in E.164 format to test it out.
Back in the command prompt run go run .
to execute the code. You should see output like this:
Next steps for account security
Now that you've sent an SMS OTP, try changing the Channel
parameter to send the OTP via WhatsApp, a voice call, or email! If you want to go beyond OTPs, check out the Verify APIs for Authenticator Apps like Authy or Push Authentication.
For more account security best practices, check out:
- Best practices for phone number validation during new user enrollment
- Best practices for managing retry logic with SMS 2FA
- How to filter out VoIP numbers before sending an SMS OTP
I can't wait to see what you build and secure!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.