Five Common Twilio StackOverflow Questions in Node.js, Ruby and PHP.
Time to read:
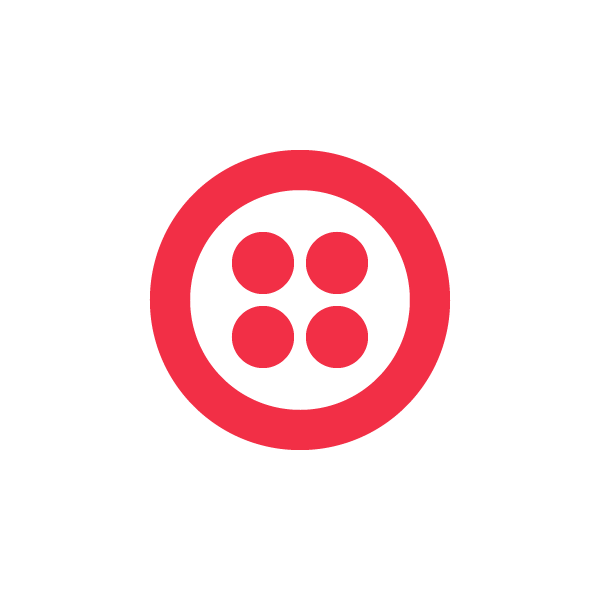
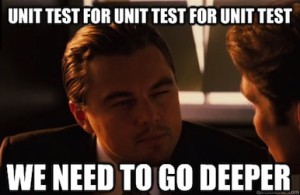
Question 1: How to use status_callback.
This is a great question and something that is important to grasp at a fundamental level. Let’s use a made-up example to hit this one home. Imagine you’re a developer in the North Pole building an app for Santa Claus to verify the information he has on file for every child on his list. The app you built does the following:
- Calls the phone number on file for each family
- If the phone is answered: routes the call to a live elf to verify the information
- If the line is busy or no-answer it updates the status of the number and adds it to a queue to call later
One way you could implement this would be to use a status_callback url when you initiate the phone call:
Now when the phone call is complete Twilio will request the status_callback url with some parameters, including CallStatus which will tell you whether the call was answered or not. Presto! You’ve just made Santa’s job so much easier, which means he has even more time to eat cookies and drink hot cocoa.
Question 2: How to Play a sound after calling a number
The hacker who asked this question was trying to play a sound after a call was initiated but their TwiML was not behaving as expected. Let’s look at the code and then we’ll debug it together.
This is a case where the TwiML interpreter does exactly what it’s told, and in a specific order that we call “control flow”. In this case the call was initiated and the control flow starts with which expects a phone call to complete before it continues with the TwiML instructions.
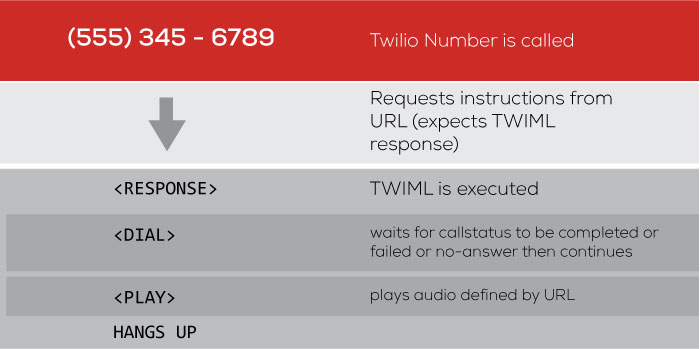
Obviously this is not what the developer wanted. Instead he should be initiating the call via the REST API, with the url that will return the following TwiML
Here is what the REST API code in the app would look like:
It’s helpful to remember that the TwiML interpreter follows the control flow and unless a verb is nested within another it will wait until the previous verb is executed to continue.
Question 3: How do you add natural pauses in Twiml?
One of my favorite Twilio features is the verb . Whenever you need a Twilio number to convert text to speech you simply use the verb in your TwiML. Here is an example of how one might use :
For text that is going to be processed by Twilio’s speech engine there are some useful tips at the bottom of the Twilio Say Docs.
- Remember that the interpreter reads digits which have no space between them as multi-digit numbers. For example: ‘12345’ will be spoken as “twelve thousand three hundred forty-five.” Whereas ‘1 2 3 4 5’ will be spoken as “one two three four five.”
- Punctuation such as commas and periods will be interpreted as natural pauses by the speech engine.
- If you want to insert a long pause, try using the verb. should be placed outside tags, not nested inside them.
Fortunately the speech engine approaches natural speaking pauses as closely as possible when you use punctuation appropriately. But if you need to add fine tuning remember commas insert about a 125ms pause and ellipses (“…”) insert a 500ms pause.
Question 4: Having Trouble with Gather recognizing inputs
This developer was having problems with their Twilio number not responding to inputs that was expecting. The code looked fine and in fact the problem was with the phone he was testing with. One of our support crew Trenton states in a response “ works by listening to the DTMF sounds that your phone or device produces. …VoIP phones can be installed with a non-standard DTMF tones as the default, which Twilio will have difficulty recognizing. VoIP phones might also be using pretty strong compression which can interfere with DTMF.”
Therefore, it’s important when testing Gather to make sure your phone is using the DTMF standard “Touch-Tone” signals. If you find yourself having troubles try using a different phone.
Question 5: How to test a twilio application?
StackOverflow Link
This is definitely one of the more popular questions we here at Twilio. Which is why Twilio has created all sorts of tools for testing every side of your application. In the case of the developer who asked this specific question he wanted to test Inbound calls to his Twilio endpoint, essentially verifying that the TwiML was executed and the phone call was recorded. So we’ll go over how to do this in a bit. But testing your Twilio app can mean all sorts of things. What if you want to test your REST API calls? What if you need to test how your application handles all of the possible API responses from Twilio? Luckily there are solutions for all of these situations!
Testing your TwiML
First let’s address the tests that this specific developer was trying to do. Essentially he wanted to test that his TwiML was executing properly. The solution as it’s posted is mostly right, with a couple exceptions. The best route here for automating a test is to write a script that will make the call, check that the call was completed, check that the recording was recorded and finally check the transcription to verify it is correct. Luckily the Twilio API allows us to do all of these things pretty easily. Here is an overview of what our test should do:
- Initiate Call to TwiML url: http://foo.com/test_call
- some sound to test that is working
- Check that recording was made
- Check that transcription is correct
So let’s look at some code (this script requires you own two Twilio numbers). First let’s write some Ruby to make these phone calls for us:
To run this script for lots of numbers you could just wrap it in a loop that iterates through your current IncomingPhoneNumbers.
Then on http://foo.com/test_call will have TwiML that looks like this:
Now on our other Twilio number (the :to number) we’ll point to a url that will host the following TwiML:
Luckily gives us super easy ways to verify that everything went swimmingly. First we passed an action url. When the recording is complete the Twilio will send a request to the action url with some parameters including RecordingDuration. Since we know our recording is 5 seconds we can check that the Recording Duration is at least 5 seconds, which will tell us the recording is working. Next by defining a transcribeCallback url we can check the transcription. When Twilio makes the request to transcribeCallback it passes a few parameters including TranscriptionText which we expect to contain “This is a simple recording to test with.”
Great now we can fire off a bunch of calls with the sample audio and make sure all of our Twilio numbers are behaving correctly!
But what if we want to test all of our REST API bits and pieces? That’s where Test Credentials come into play!
Test Credentials
For those of you who are restful testers (say that five times fast) Twilio gives you test credentials so you can exercise parts of the REST API without charging your account. You can find your credentials in the Developer Tools section of your Dashboard.
Test Credentials are great for all sorts of reasons. For one you can test that your app is all set up to send Messages and make Calls without getting charged or mucking up your production data. But more importantly you can test all of those error responses and handle edge cases long before they become issues. For example if you wanted to test error handling when you attempt to send an SMS to a non-mobile number you can just use the magic number: 1(500)555-0009. Here is how that would look in node.js:
Currently you can use test credentials to:
- Send Messages
- Make Phone Calls
- Buy Phone Numbers
Follow these links to read more about buying a phone number and using magic numbers.
Lastly, for our Ruby users out there, there is a very cool testing kit built by Jack Nichols called the Twilio Testing Toolkit (TTT). Impressively, TTT allows you to simulate incoming Twilio requests to your app methods, all from a simple gem install. You can read more about it here.
So my friends, that about does it. May the wind be at your back and may these solutions help you build something awesome. And of course, if you think these Twilio Stackoverflow questions aren’t the right ones or you would like to improve upon them send me an email. Onward fellow doers! See you on the stack.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.