How to Use MongoDB in Laravel Lumen
Time to read:
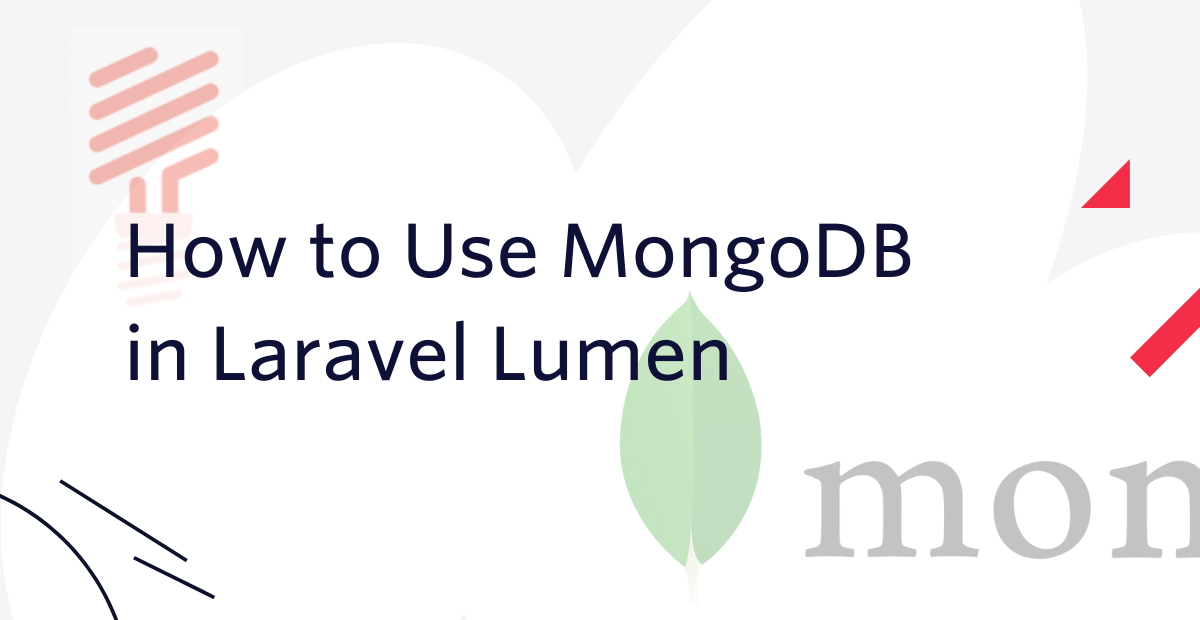
As consumers demand more access to apps and services, developers pursue new ways to make data structuring and querying more effective, in order to reduce response times.
This demand has inadvertently led to a migration from Structured Query Language (SQL) to Non-structured Query Language (NoSQL) for database interactions. For applications that require data to be stored in one place and accessed once without creating relationships, this leads to faster querying.
One of the most widely adopted versions of NoSQL is MongoDB, an open-source document-based database used for cross-platform document-oriented database programming.
In this tutorial, you will learn how to use MongoDB in a Laravel Lumen application, to store, edit, and read data.
Prerequisites
In order to complete this tutorial you will need access to the following:
- Basic knowledge of PHP
- Basic experience with Laravel and Lumen
- Basic experience with MongoDB
- PHP 8.0 or higher, with the mongo-db extension
- A MongoDB account
- curl
- Composer installed globally (and Git, which Composer requires)
MongoDB is a document-oriented, NoSQL database that does not rely on tables, supports transactional queries, and has a powerful search query system while using a simple and common query system, returning the data in JSON format to be consumed.
Using MongoDB with Lumen provides many advantages for data management such as document-oriented data storage, flexible document schemas, and powerful analytics.
Advantages of MongoDB with Lumen
Just in case you’re new to implementing MongoDB within Lumen, here is a more comprehensive look at the benefits:
- Document-Oriented Data Storage: Data will be stored as documents, removing the need for a relational database and providing data replications and high availability. Data is stored as collections and collections have an unlimited amount of documents that can contain diverse data types at scale.
- Easy Integration: For example, with the Laravel MongoDB package, created by Jens Segers, integrating and using MongoDB is relatively easy. The package supports provider configurations and proper documentation.
- Fast Queries: MongoDB fetches primary keys, indexes, and collections in a matter of milliseconds.
- Cloud-Based: MongoDB is fully cloud-based and updates are made in real-time to the database. This ensures the security of your data and redundancy with backups.
Install Lumen
To begin, you will install Lumen into a specified folder, before configuring MongoDB. Open your terminal/command line and run the following code to create a new Lumen project:
Change into the newly created project folder lumen-mongodb
, and start the application on port :8000 by running the commands below.
Next, generate an application key using the maxsky/lumen-app-key-generator package. Run the following command to install the package:
Next, add the command into your kernel file in app/Console/Kernel.php.
Now, open up your terminal and run the following command to generate a new application key:
The key will now be available near the top of .env.
Install and setup MongoDB
After installing and setting up Lumen, you’ll need to do three things:
- Add the MongoDB package to the application
- Create a new MongoDB cluster
- Link the cluster to the application
Let’s start with adding the MongoDB package to the application. To do that, run the following code in the application's top-level directory:
Next, register the Laravel MongoDB Provider to support the newly installed package by adding the following code to bootstrap/app.php, underneath // $app->withFacades();
.
Then, uncomment the following line.
Configure the MongoDB database
After the installation is complete, create a new directory named config, and in that directory a new file named database.php. This new file supports the MongoDB connection and makes it the default database for the application. This code will update your .env environment variables to determine where your MongoDB cluster is located.
The DB_URI
environment variable, in the preceding example, is generated by MongoDB when you generate a new cluster. To retrieve it, follow the instructions in this article which shows you how to install your MongoDB client, obtain your MongoDB connection string (URI), and connect to your MongoDB instance.
After the cluster has been generated, add the following snippet to the bottom of .env and replace the placeholder <MONGODB_URI>
with the retrieved MongoDB URI.
Interact with the MongoDB Database
With the installation process out of the way, next up, you have to create an application model that will target a collection in MongoDB and fetch the relevant data from it. After which, a new controller will be generated to fetch data from the MongoDB database.
Creating a new model allows us to connect with MongoDB and interact with a specific collection in the database. Upon connection, we can then specify the columns that can be filled, specify id in some scenarios, and so much more.
Create the model
Create a new file, named Blog.php, in the app/Models folder and add the following code to it:
After that, add the following use
statement to the top of the file:
In the previous code block, the private variable $connection
stores the database connection. This is important because, by default, Lumen uses MySQL as its default database.
Next, the variable $collection
specifies the name of the MongoDB database collection to query. When a request is made through a route, picked up by the controller, and sent to the model, the requested data is fetched from the specified $collection
.
Create a controller
To interact with the model created, a controller needs to be created and functions added to it. Upon completion, this controller will be able to fetch, update, create, and delete data in the specified MongoDB collection.
To do this, create a new file in the app/Http/Controllers directory, and name it BlogController.php. Then, in the newly created file, add the index()
function as described in the next section. This function will retrieve all the blog items from the collection and return them in JSON format.
After that, add the following use
statements to the top of the file:
Create the route
The next step is to create a route that will accept the API request and return all the blog items from the collection as a response.
To do that, at the end of routes/web.php create a new route that targets the index()
function in the blogs controller, by adding the following code.
Test the application
To test, we will be using curl on our terminal to make a get request to the all
route. This request will interact with the specified controller to get data from the collection, use the model, return a response that contains the data in a JSON format, and display it in the terminal.
To do that, run the following code in your terminal:
You should get a response similar to the following JSON example:
That's how to use MongoDB in Laravel Lumen
In this tutorial, you briefly learned what MongoDB is and its advantages for developing large-scale applications; including fast data queries and document-based data storage.
Then you created a new Laravel Lumen application and integrated MongoDB into it so it could interact with clusters and collections. Lastly, you learned how to fetch rendered data in JSON format in response to an API query.
I hope this article gave you a broad introduction on how to use MongoDB in your next Lumen project. If you want to clone the code used in this article and look further into it, you can use this GitHub repository.
Kenneth Ekandem is a full-stack developer from Nigeria currently in the blockchain space, but interested in learning everything computer science has to offer. He'd love to go to space one day and also own his own vlogging channel to teach the next generation of programmers.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.