Employee Directory with Java and Servlets
Time to read:
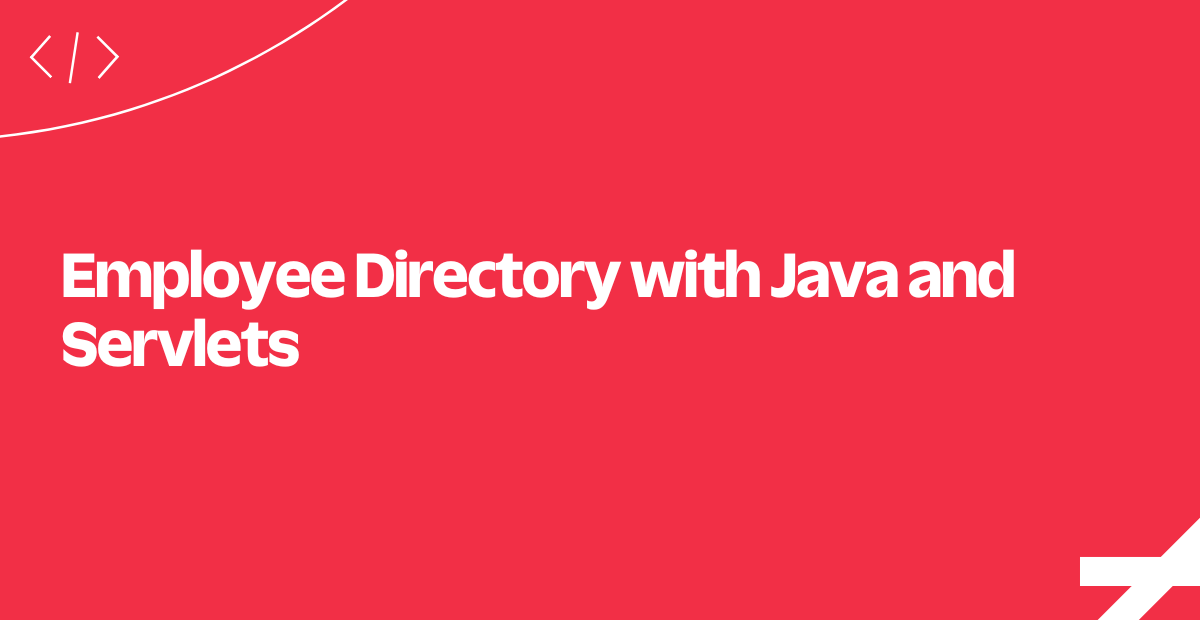
Learn how to implement an employee directory that you can query using SMS. Request information from anyone at your company just by sending a text message to a Twilio Number
Here is how it works at a high level:
- The user sends a SMS with an Employee's name to the Twilio number.
- The user receives information for the requested Employee.
Handle Twilio's SMS Request
When your Twilio Number receives an SMS, Twilio will make a POST request to /directory/search
asking for TwiML instructions.
Once the application identifies one of the 3 possible scenarios (single partial match, multiple partial match or no match), it will send a TwiML response to Twilio. This response will instruct Twilio to send an SMS Message back to the user.
Let's take a closer look at each one of the scenarios.
Find a Single Employee Match
This is the simplest scenario. We query our database expecting to find an employee whose name is whole or partially like the one specified in the SMS sent to our Twilio number. If a single match is found, a message containing this employee's information is built and sent back to Twilio as TwiML instructions.
If multiple matches are found we'll try to do a multiple partial match. That is our next possible scenario.
Find Multiple Matches
Now we'll try to get a partial match that returns more than one result. We need to store a List of BasicNameValuePair
containing indexed suggestions so the user can reply with a number that references one of the suggestions in order to get all the employee's information. We'll use Twilio Cookies to store the suggestions.
Now you know how to attempt to find employee matches. Next, we will see how to handle the case where a match can't be found.
What if no Employee?
If none of the previous scenarios occur, it means that there is no employee in the database that matches the user's query. In that case, a response will be sent to the user explaining that their query has no results.
We've built the search capability for our server. Next, we'll see how we use cookies to cache search suggestions.
Store Suggestions With Cookies
When a user gets multiple matches by searching the employee's directory, we reply with multiple indexed suggestions. We need to store these suggestions, so next time the user sends an SMS we know this is not a query for a new employee, but a selection of one of the suggestions.
We'll use Twilio Cookies to store suggestions. They will allow you to keep track of an SMS conversation between multiple numbers and your Twilio-powered application.
That's it! We have just implemented an employee directory using Java with servlets. Now you can get your employee's information by texting a Twilio number.
Where to next?
If you're a Java developer working with Twilio, you might also enjoy these tutorials:
Learn how to use Twilio Client to make browser-to-phone and browser-to-browser calls with ease.
Learn how to implement ETA Notifications using Java with servlets and Twilio.
Did this help?
Thanks for checking out this tutorial! If you have any feedback to share with us, we'd love to hear it. Tweet @twilio to let us know what you think!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.