Employee Directory with Ruby and Rails
Time to read:
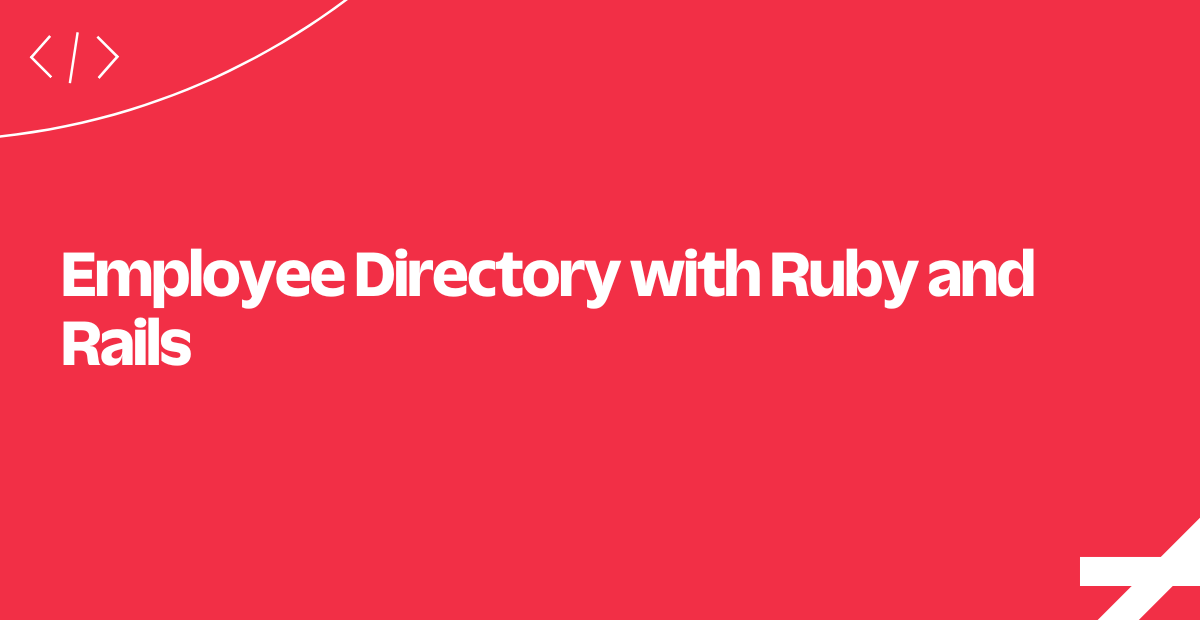
Learn how to implement an employee directory that you can query using SMS. Request information from anyone at your company just by sending a text message to a Twilio Number
Here is how it works at a high level:
- The user sends a SMS with an Employee's name to the Twilio number.
- The user receives information for the requested Employee.
Handle Twilio's SMS Request
When your Twilio Number receives an SMS, Twilio will make a POST request to /directory/search
asking for TwiML instructions.
Once the application identifies one of the 3 possible scenarios (single partial match, multiple partial match or no match), it will send a TwiML response to Twilio. This response will instruct Twilio to send an SMS Message back to the user.
Let's take a closer look at each one of the scenarios.
Find an Exact Employee Match
This is the simplest scenario. We query our database expecting to find an employee whose first and last name are exactly like the ones we specified in the SMS that was sent to our Twilio number. If a match is found, a message containing this employee's information is built and sent to Twilio as TwiML instructions.
If no match is found we'll try to do a single partial match. That is our next possible scenario.
Find a Single Partial Employee Match
If no employee's exact match is found on the database we'll try to find a close match using Fuzzily Gem. In this scenario we'll verify that only 1 partial match is obtained. When a single partial match is found, the user will receive a suggestion containing it. The user will be given the opportunity to reply with the word yes
to this SMS. If that were the case, the user will receive the information for the employee that was chosen.
We'll show you how the suggestions are stored for the SMS conversation using Twilio Cookies a few steps later.
Now that we know how to find individual employees, lets see how we can get multiple results.
Find Multiple Partial Employee Matches
At this point we have already tried to use the user's query as an exact employee match and a single partial match. Now we'll try to get a partial match that returns more than one result. Just like in the previous scenario, we'll use Twilio Cookies to store suggestions. Then only difference here is that we need to store a Hash containing index suggestions so that the user can reply with a number that references one of the suggestions in order to get all the employee's information.
The last scenario is simple. If none of the previous scenarios occur, it means that there is no employee in the database that matches the user's query. In that case, a reply will be sent to the user explaining that their query doesn't match any of the employees found on the database.
Now that we have full search functionality lets see how we can optimize it by caching search suggestions in the browser's cookies.
Storing Suggestions With Cookies
When a user gets a partial match by searching the employee directory, we reply with one or more suggestions. We need to store this suggestions, so the next time the user sends an SMS we know this is not a query for a new employee, but a selection of one of the suggestions.
We'll use Twilio Cookies to store suggestions. They will allow you to keep track of an SMS conversation between multiple numbers and your Twilio powered application.
That's it! We have just implemented employee directory using Ruby on Rails. Now you can get your employee's information by texting a Twilio number.
Where to next?
If you're a Ruby developer working with Twilio, you might also enjoy these tutorials:
Ruby Quickstart for Programmable Voice
Learn how to use Twilio Client to make browser-to-phone and browser-to-browser calls with ease.
ETA Notifications with Ruby and Rails
Learn how to implement ETA Notifications using Ruby on Rails and Twilio.
Did this help?
Thanks for checking out this tutorial! If you have any feedback to share with us, we'd love to hear it. Tweet @twilio to let us know what you think!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.