ETA Notifications with PHP and Laravel
Time to read:
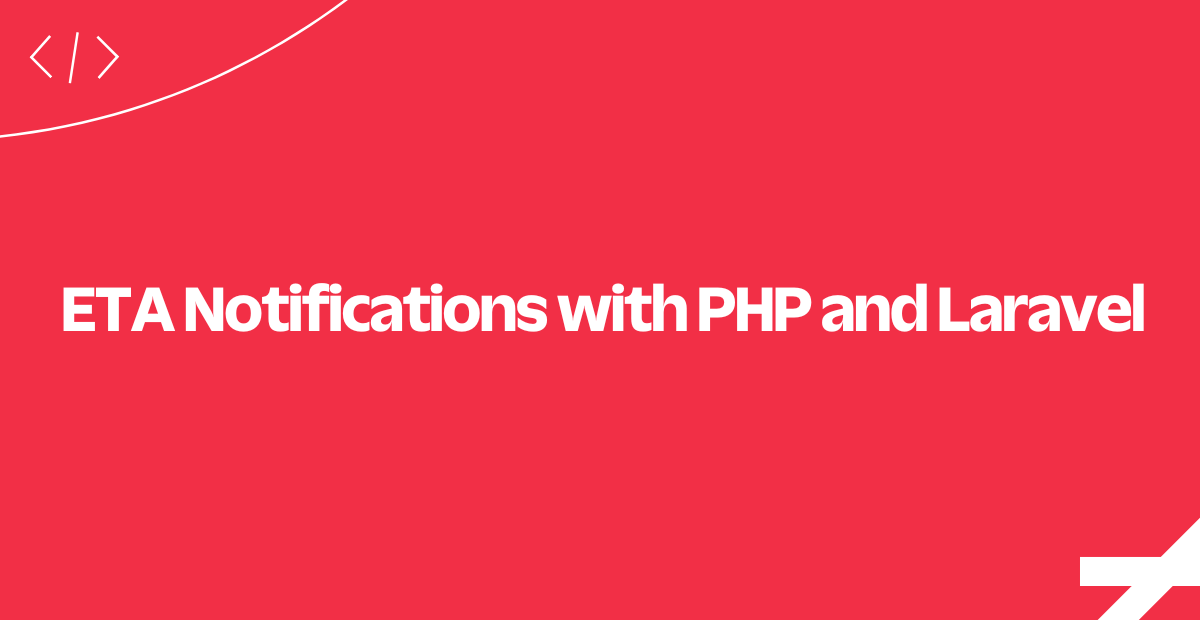
Companies like Uber, TaskRabbit, and Instacart have built an entire industry around the fact that we, the customers, like to order things instantly, wherever we are.
The key to these services working is notifying customers instantly when things change. Customers appreciate being in the loop on order status, and (of course) like to know when something changes.
In this tutorial, we'll build a notification system for a fake on-demand laundy service Laundr.io with PHP and Laravel.
Let's get started!
Trigger the Notifications
The delivery person's screen will have two buttons to update orders which are wired to the appropriate routes:
- Delivery person picks up laundry to be delivered (
/pickup
) - Delivery person is arriving at the customer's house (
/deliver
)
The buttons will suffice for our demo app. In a production app you would likely use GPS to trigger deliver
, but the concept is the same.
Now let's look at how we set up the PHP Twilio REST Client.
Setting Up the Twilio REST Client
Here we create an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize the client with our Twilio account credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
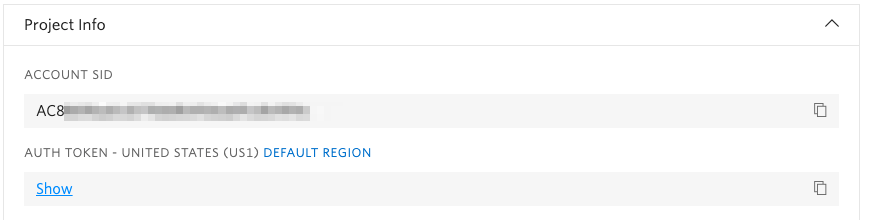
Next up, let's look at what we do with incoming notification triggers.
Handle Notification Triggers
In OrderController
we extract the phone number stored in each order, and then simply send an SMS message with an appropriate message body. Easy!
Next, let's look closer at how we send the SMS itself.
Send a SMS (or MMS) To the Customer
This code shows how we send the SMS with the Twilio PHP Client.
Better shown than said? We agree - you can add an image of the order with the optional mediaUrl
:
'mediaUrl' => 'http://lorempixel.com/image_output/fashion-q-c-640-480-1.jpg'
In addition to the required parameters (and optional mediaUrl
), we can pass a statusCallback
url to let us know if the message was delivered.
The message delivery status updates are interesting. Let's zoom in on those next.
Handling a Twilio Status Callback
Twilio will make a post request to this controller each time our message status changes to one of the following: queued
, failed
, sent
, delivered
, or undelivered
.
We then update this notification_status
on the Order
and your own business logic would take it from there. This is an excellent place to add logic that would resend the message in the case of a message failure, or send out an automated survey when there is a successful delivery to the customer.
That's a wrap (a fold?)!
We've just implemented an on-demand notification service that alerts our customers when their laundry order is arriving. Let's take a look at other features that are easy to add with Twilio.
Where to next?
We've got a lot of great PHP content here on the Docs site but we've selected just a couple you might like to visit next:
Increase your rate of response by automating the workflows that are key to your business. In this tutorial, learn how to build a ready-for-scale automated SMS workflow for a vacation rental company.
Protect your users' privacy by anonymously connecting them with Twilio Voice and SMS. Learn how to create disposable phone numbers on-demand so two users can communicate without exchanging personal information.
Did this help?
Thanks for checking this tutorial out! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.