ETA Notifications with Ruby and Rails
Time to read:
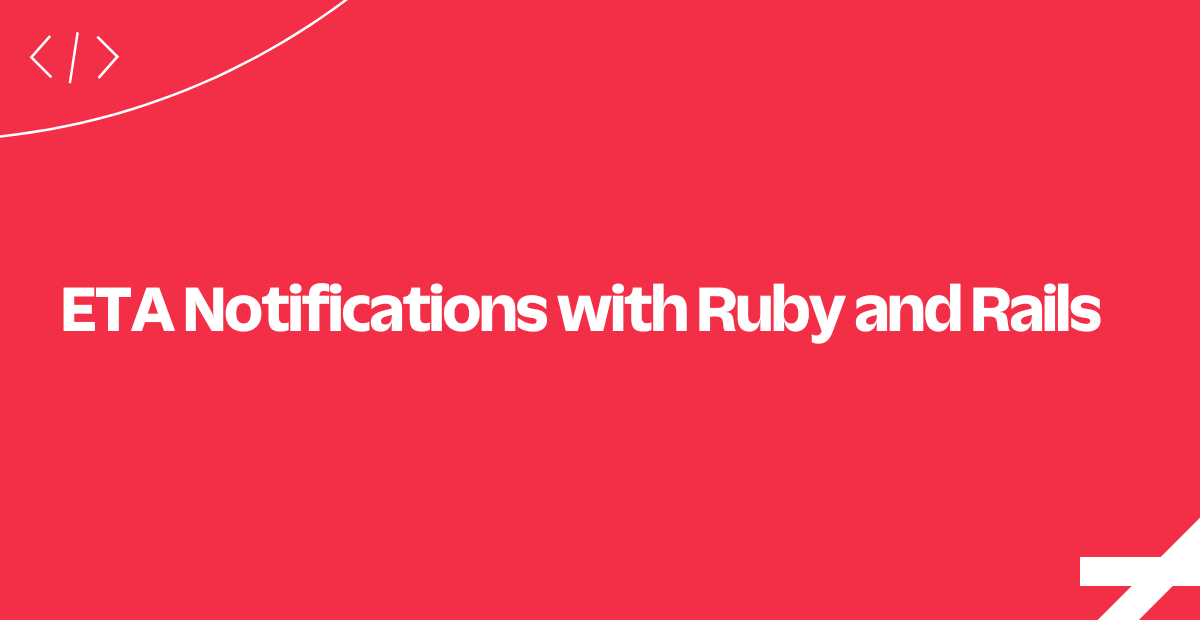
Companies like Uber, TaskRabbit, and Instacart have built an entire industry around the fact that we, the customers, like to order things instantly, wherever we are. The key to those services working? Notifying customers when things change.
In this tutorial, we'll build a notification system for a fake on-demand laundry service Laundr.io using Ruby on Rails.
Let's get started! Click the below button to begin.
Trigger the Notifications
There are two cases we'd like to handle:
- Delivery person picks up laundry to be delivered (
/initial_notifications
) - Delivery person is arriving at the customer's house (
/delivery_notifications
)
In a production app we would probably trigger the second notification when the delivery person was physically near the customer, using GPS.
(In this case we'll just use a button.)
Let's look at using the Ruby Twilio REST API Client to actually send out the notifications.
Set up the Twilio REST Client
Here we create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
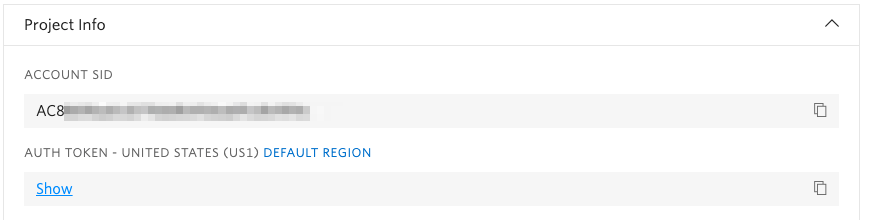
Next up: how we handle notification triggers.
Handle a Notification Trigger
This code handles the HTTP POST
requests triggered by the delivery person.
It uses our MessageSender
class to send an SMS message to the customer's phone number, which we have registered in our database. Simple!
Next, let's look closer at how we push out the SMSes.
Send an SMS (or MMS)
Here we demonstrate how we actually send the SMS.
Picture worth 1,000 words? We can add a picture of the laundry by adding:
media_url: "http://lorempixel.com/image_output/fashion-q-c-640-480-1.jpg"
In addition to the required parameters (and the optional media), we can pass a status_callback
url to let us know if the message was delivered.
Message status updates are interesting - let's look there next.
Handle a Callback from Twilio
Twilio will make a POST
request to this controller each time our message status changes to one of the following: queued
, failed
, sent
, delivered
, or undelivered
.
We then update this notification_status
on the Order
and let the business logic take over. This is a great place to add logic that would resend the message if it failed or send out an automated survey soon after a customer receives his or her clothes.
That's all, folks! We've just implemented an on-demand notification service that alerts our customers when their order is picked up or arriving.
Now let's look at other features that you might want to add to your application.
Where to next?
We've got lots of Ruby and Rails content here on the Docs site. Sadly, we wanted to cut it down - here are just two other excellent tutorials you might enjoy:
Workflow Automation with Ruby and Rails
Increase your rate of response by automating the workflows that are key to your business. In this tutorial, learn how to build a ready-for-scale automated SMS workflow for a vacation rental company.
Masked Phone Numbers with Ruby and Rails
Protect your users' privacy by anonymously connecting them with Twilio Voice and SMS. Learn how to create disposable phone numbers on-demand so two users can communicate without exchanging personal information.
Did this help?
Thanks for checking this tutorial out! Let us know what you've built - or what you're building - on Twitter.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.