SMS and MMS Marketing Notifications with Java and Servlets
Time to read: 3 minutes
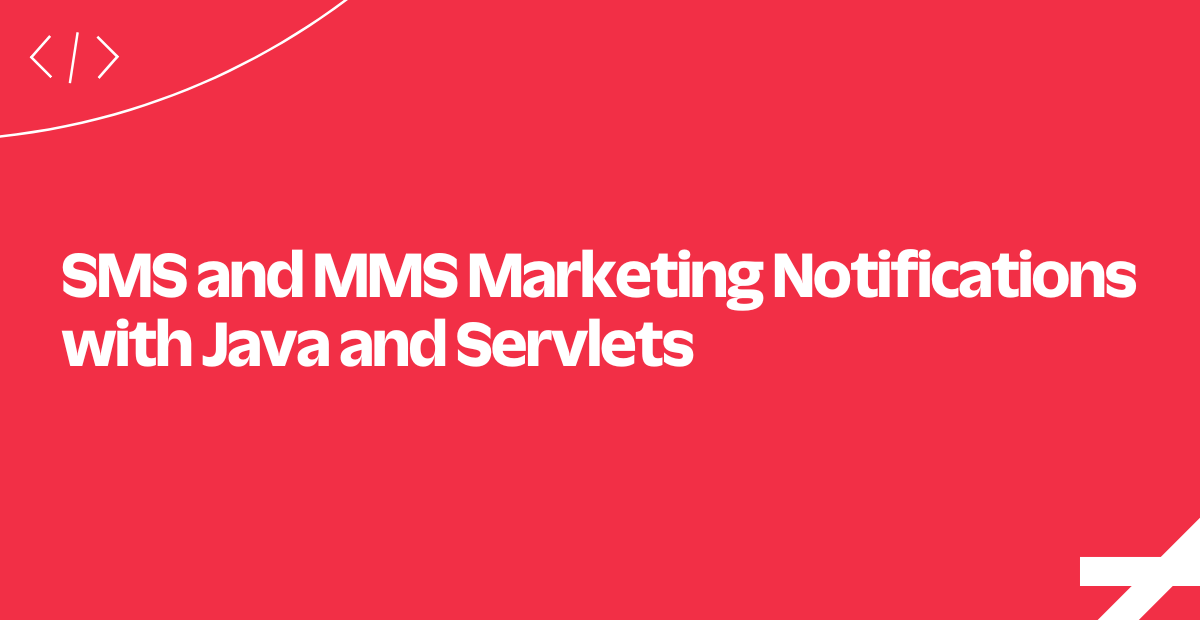
Ready to implement SMS and MMS marketing notifications with Java and Servlets?
Here's how it'll work at a high level:
- A possible customer sends an SMS to a Twilio phone number you advertise somewhere.
- Your application confirms that the user wants to receive SMS and MMS notifications from your company.
- An administrator or marketing campaign manager uses a web form to craft a message to push to all subscribers via SMS/MMS message.
Building Blocks
To get this done, you'll be working with the following tools:
- TwiML and the <Message> verb: We'll use TwiML to manage interactions initiated by the user via SMS.
- Messages Resource: We will use the REST API to broadcast messages out to all subscribers.
Let's get started!
The Subscriber Model
Before send out marketing notifications to a subscriber, we need to start with the perfect model:
phoneNumber
stores where to send the notifications.subscribed
stores if a subscriber is active (only an active subscriber will receive notifications).
Let's see how to handle incoming messages.
Handle Incoming Messages
This is the endpoint that will be called every time our application receives a message.
We begin by getting the user's phone number from the incoming Twilio request. Next, we try to find a Subscriber
model with that phone number.
If there's no subscriber with this phone number, we create one, save it, and respond with a friendly message asking our newest user to send an SMS with "add" as a command. This is done to confirm that they want to receive messages from us.
We've created a Subscriber
model to keep track of the people that want our messages. Now, let's look at the options we provide our users for opting in or out.
Managing User Subscriptions
We want to provide the user with two SMS commands to manage their subscription status: add
and remove
.
These commands will toggle a boolean flag for their Subscriber
record in the database and will determine whether they get our marketing messages. Because we want to respect our user's preferences, we don't opt them in automatically - rather, we have them confirm that they want to receive SMSes or MMSes from us.
To make this happen, we will need to update the controller logic which handles the incoming text message to do a couple things:
- If it is a
add
orremove
command, create/update aSubscription
with the right status in the database. - If it is a command we don't recognize, send a message explaining the two available commands.
Let's now take a look at how to send notifications.
Send Notifications Out
When a form is submitted, we first grab the message text and/or image URL. Second, we loop through all Subscribers and call the method send
on our Sender
domain object.
When the messages are on their way, render the index page with a success message and delight the marketing team.
Let's take a closer look at how to send SMS or MMS notifications.
Sending SMS or MMS Notifications
When the model object is loaded, it creates a Twilio REST API client that can be used to send SMS and MMS messages. The client requires your Twilio account credentials (an account SID and auth token), which can be found in the console:
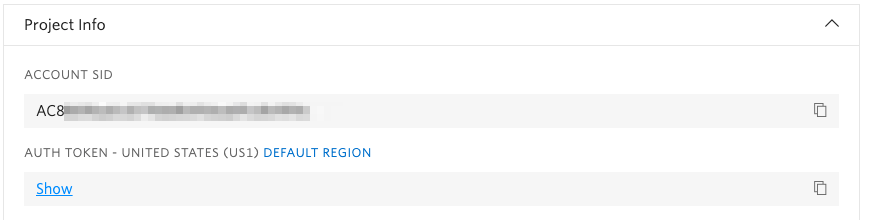
Next, all we need to do is create
a message using the MessageCreator
class. The Twilio Message API call creator requires To
, From
and Body
parameters. MediaUrl
is an optional parameter.
That's it! We've just implemented a an opt-in process and an administrative interface to run an SMS and MMS marketing campaign. Now all you need is killer content to share with your users via text or MMS.
Where to Next?
Love Java? So do we at Twilio! Here are two other excellent tutorials you should check out:
SMS notifications are a great way to alert humans quickly when important events happen. In this example, we'll show you how to send SMS notifications to a list of people when an error happens in a web application.
Don't let valuable insights slip away - survey your customers with Voice and SMS.
Did this help?
Thanks for checking out this tutorial... tweet @twilio to let us know how you did and what you're building!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.