Automating Twilio Integration Tests with Test Credentials
Time to read: 6 minutes
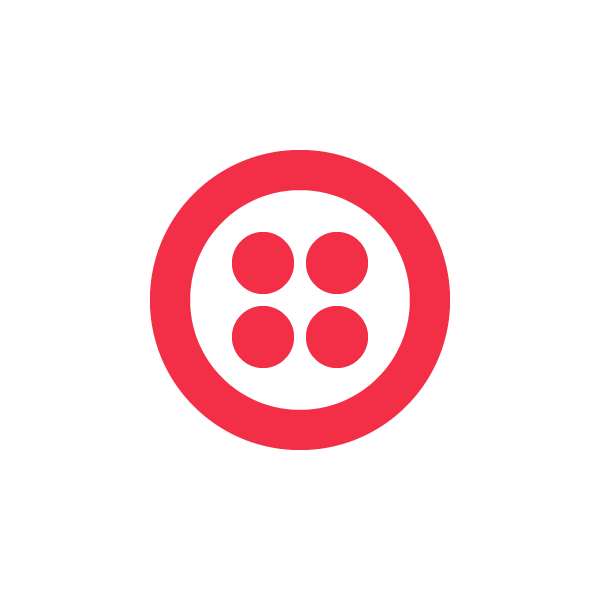
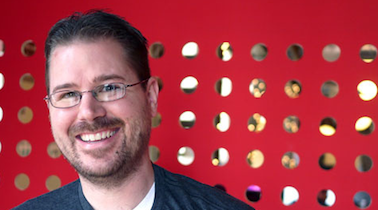
In this post I’ll show you how you can use Test Credentials to build automated integration tests for your application. I’ll be using the Visual Studio unit test framework for my samples, but the principles should apply to any unit test framework.
Exploring Test Credentials
Test Credentials is composed of two parts. First, Test Credentials provides you with a second Account Sid and Auth Token that you can use to authenticate requests made to the Twilio REST API. When you use these credentials, it tells Twilio to execute the request as a simulation, but Twilio will still validate all of the input as though you used your real credentials.
You can find your Test Credentials under the Dev Tools section of the Twilio dashboard.
The second part of Test Credentials are a set of special phone numbers that you use to get Twilio to return a specific error responses. Using these numbers allows you to test the parts of your application that handle the different error conditions that that Twilio might return when sending an SMS, initializing a phone call or buying a phone number.
Test Sending an SMS Message
Let’s start by setting up some tests that exercise sending an SMS message. To help demonstrate this I created a simple Message class that encapsulates an SMS message that I want to send via the Twilio API, as well as the logic needed to actually communicate with the Twilio API.
The code for the Message class is pretty straight forward and uses the Twilio C# helper library to make calls to the REST API. If you are interested in seeing the complete code for the class, head over to my page. In this post I’m going to focus on two parts of the class, the Send method and the Status property.
Normally a developer using the Message class in their application will see only a single Send method that takes no parameters. This is because the class looks in a config file for the credentials needed to authenticate against the Twilio API. However when running tests, I need to use the Twilio test credentials and I don’t want to keep those in that config file.
There are lots of different techniques for injecting values into a class, but in this case in order to allow my tests to inject the Test Credentials but keep them isolated inside of my test project, I’ve created an internal overload for Send that takes an Account Sid and Auth Token:
In order to let my tests see this internal method, I need to set the InternalsVisibleTo attribute.
Now that I can use the correct credentials in my tests, I can test sending a message.
The part of the Message class that I want to test for in my integration tests is the Status property, which is a read-only property that tells me what the current status of the Message is. The Status property is initially set to Unsent, but once I call the Send method the Message class will automatically change the value of the property based on the result of sending the message.
You can see the test I wrote to test the value of the Status property below:
The test asserts that the Status property will be equal to Success, indicating that the message was sent to Twilio successfully, and Twilio responded with a successful response. In this case I know that the Twilio request will succeed because the value of the TO_NUMBER variable is a valid 10 digit phone number which Twilio will validate properly.
Now let’s test a failure case. Let’s say that a customer provides a land line phone number, which obviously cannot receive a text message. In that case, when I try to send the Message, Twilio is going to return an error code and I want to make sure that my app handles that correctly. Below is a test that exercises that code path:
In this case I’m passing in a static string named NONMOBILE_FAIL_TO_NUMBER into the create method. The value of this variable is +15005550009, which is one of the special numbers that Twilio provides and tells Twilio to return a 21614 error, the error returned when you try to send a test message to a non-mobile number. For this test to pass, I assert that the value of the Status property will be Fail.
So far I’ve created tests that let me test sending different To numbers to the Twilio API, but what about different from numbers? Its not uncommon that you might want to let your users pass in a number to send the message from. Twilio has special numbers that let you test error conditions resulting from invalid From numbers.
The test below tries to send a message from a number that is not owned by your Twilio account:
Admittedly all of these tests are fairly simplistic. If I wanted to make the tests more comprehensive, one option would be to modify my Message class to expose additional error information like the Twilio error code and message and then change my tests to assert against those properties, in addition to the Status property.
Test Initiating an Outbound Call
Now let’s create a test that simulates initiating an outbound phone call via the Twilio REST API. Like sending an SMS message, in order to demonstrate initiating and outbound voice call, I set up a simple PhoneCall class which encapsulates the data and logic needed to make the request to the Twilio API to initiate an outbound phone call.
Just like the SMS tests, this test asserts on the Status property.
To simulate an error, I’ll pass in another special number provided by Test Credentials to the Twilio API, this time simulating a call to an international number that I do not have permissions to call.
Like the SMS tests in the previous section, I can also create tests to simulate valid and invalid From numbers.
Test Buying a Phone Number
Lastly, let’s look at how Test Credentials can help you simulate buying new phone numbers through the Twilio API. In order test this, I’ve create a mock Customer object which exposes a BuyNumber method. Calling this method will make a request to the Twilio API adding a new incoming phone number to my Twilio account, set the Customer classes PhoneNumber property to this new number then return a bool value indicating the success or failure in buying the number.
To create a test, I’m passing in my Test Credentials into an internal overload of the Customer constructor, and then asserting that both the method returned True and that the PhoneNumber property equals the expected number.
In the test above I am testing purchasing a specific phone number, but the Twilio API also allows me to purchase a new phone number by specifying just the area code that I want the number in. If I do that, Twilio will pick a random number in that area code for me.
However, there are some area codes, such as Manhattan’s 212 area code, where phone numbers are scarce and may not be available. Test Credentials lets me simulate trying to purchase a number from an area code with no available numbers by passing in a special area code:
In this case the AREA_CODE variable contains 533, which tells Twilio to simulate an attempt to purchase a number from an area code with none available.
Wrapping Up
Twilio’s Test Credentials are a handy way to create integration tests for your application, without having to worry about impacting your account. They are also a great way to ensure you’ve got those error cases covered in your app.
The complete code for this post can be found on my github page, and I welcome your questions and comments on this post. Reach out to me on twitter at @devinrader or by email at devin@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.