How to send SMS using NodeJS and Azure Functions
Time to read: 5 minutes
How to send SMS using NodeJS and Azure Functions
Want to add SMS capabilities to your web application without managing servers? In this blog post, I will show you how to easily send SMS messages using Node.js and Azure Functions. Functions as a Service (FaaS), like Azure Functions, offer a serverless approach to development. This means you don't need to worry about maintaining complex infrastructure – just write your code and let the platform handle the rest. Serverless solutions offer advantages like scalability, cost-effectiveness, and ease of deployment.
Let's dive into building the Azure Function project using the CLI and learn how to send SMS messages in a snap!
Prerequisites
Before we can move forward, make sure you have the following.
- A Twilio Account: You can sign up here
- An Azure Account: Register at Azure to open an account.
- Azure CLI (locally or Cloud Shell via Azure portal)
- Azure Functions Core tools
Developing Azure Function locally
Today, we will create our own SMS sending service using Azure Functions and Twilio. Azure Functions is a serverless offering that allows us to deploy our code to the cloud without worrying about the underlying infrastructure.
Let’s start with creating the Azure Function project locally on our machine. You will need the Azure Functions Core tools installed. Once you have it installed, run the following command in your terminal.
This command creates a JavaScript project that uses the desired programming model version.
Now, let's create a function. To add a function to our project, run the func new
command using the --template
option to select your trigger template. The following example creates an HTTP trigger named SMSTrigger:
Now you should see a new directory within your MyTwilioProject directory, called SMSTrigger. This directory has two files that were generated: index.js
and function.json
.
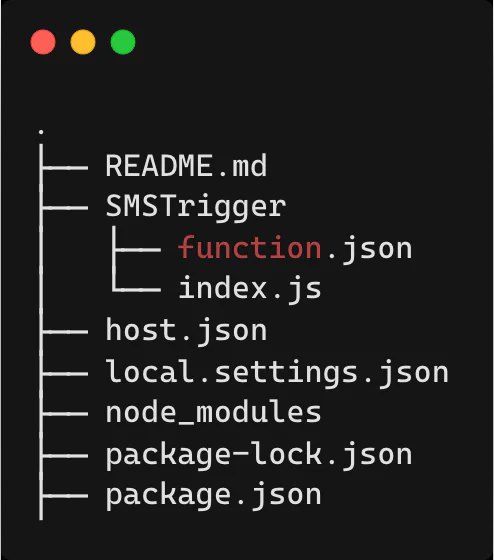
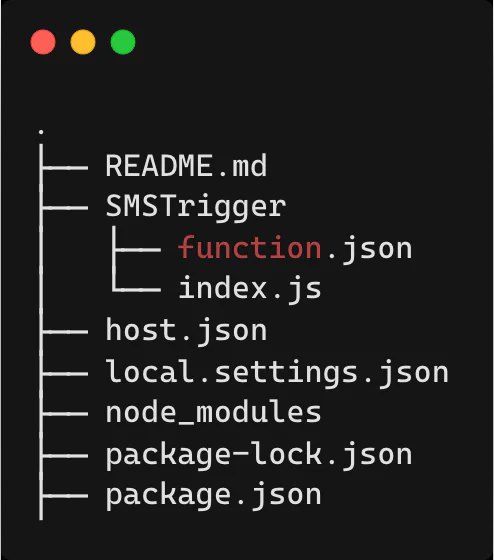
Writing the Azure Function
In order to use Twilio within our function, we will need to install the npm package:
Now let’s edit the index.js
in our SMSTrigger
directory so that it can use Twilio library to send an SMS.
Also, for the purposes of this demo, let’s set the Azure Function authentication level to anonymous so that we can trigger the function via HTTP with no key (Authorization).
Edit the function.json
in the SMSTrigger
directory, set the "authLevel": "anonymous".
With our JavaScript code in place, we are left with setting up the credentials for our Twilio library. We will be using Account SID and AUTH token from our Twilio account.
Configuring your Twilio Account
Once you are logged in to the Twilio Console, scroll down to find your Account SID and Auth Token. Copy these values, as we will save these as Environment Variables within our Azure Function.
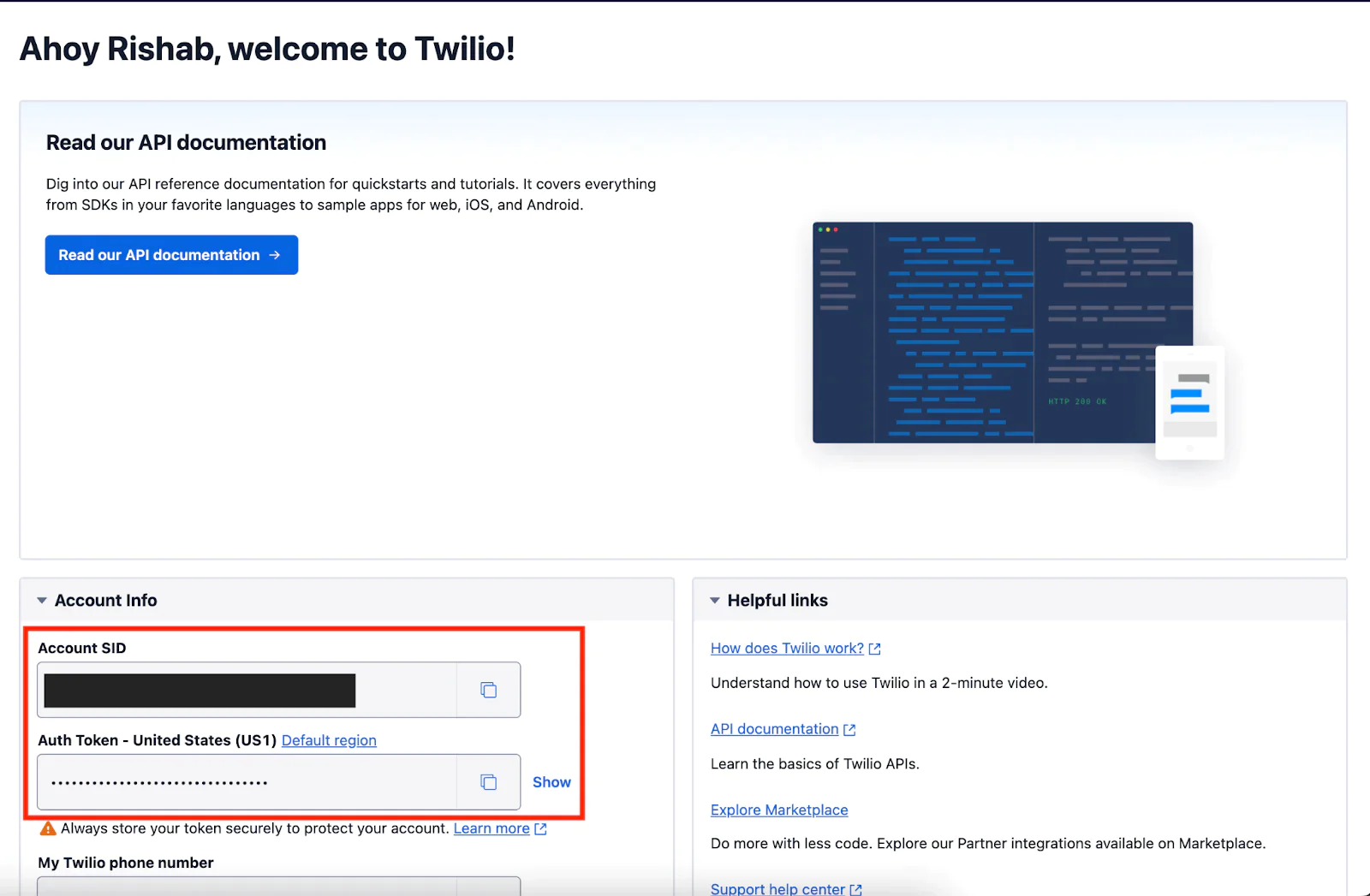
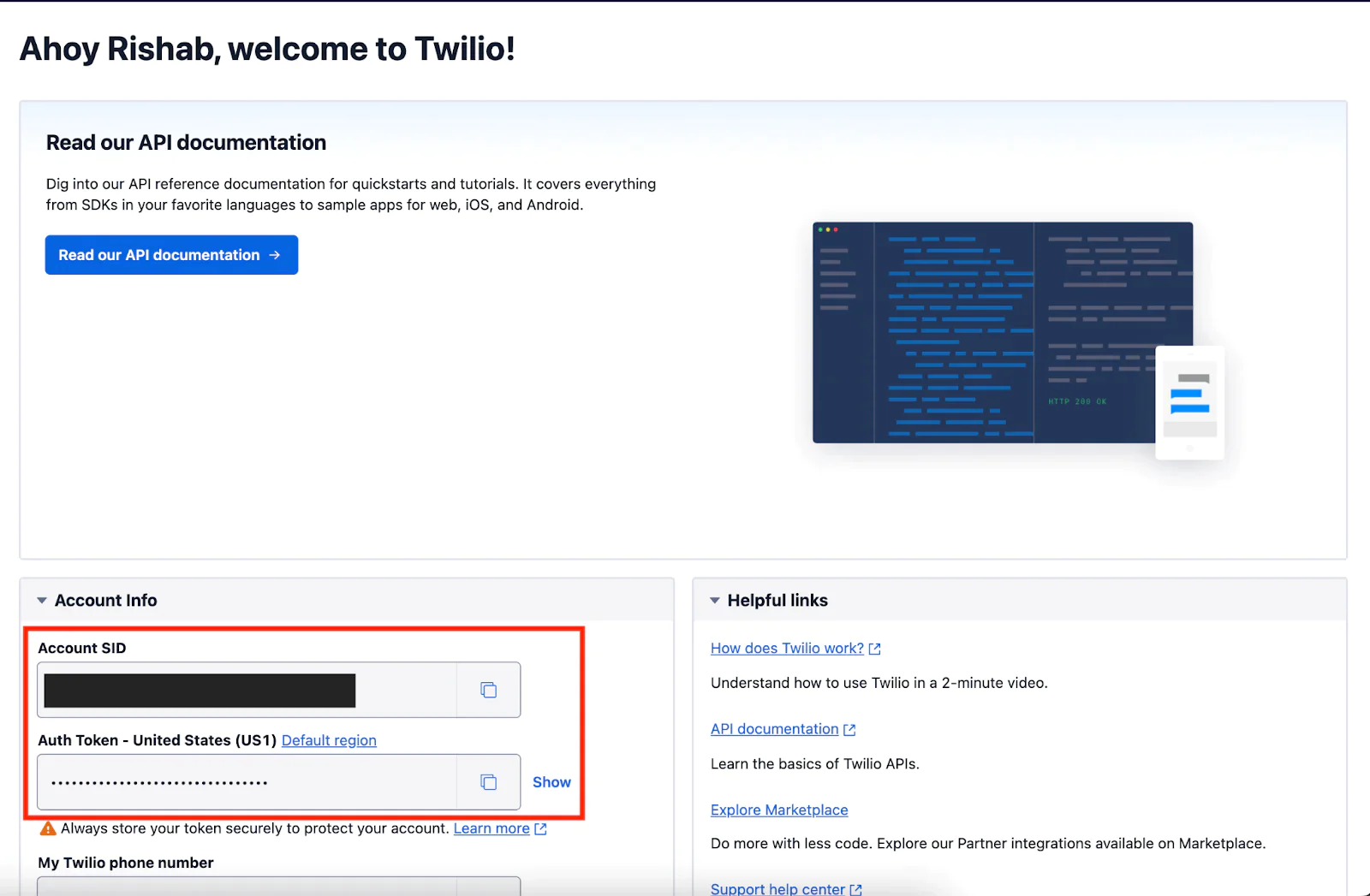
To send a message, you will need to buy a phone number—ideally from the region you are based in—to avoid roaming charges. To accomplish this, go to the Twilio Console and search for a number you’d like to buy.
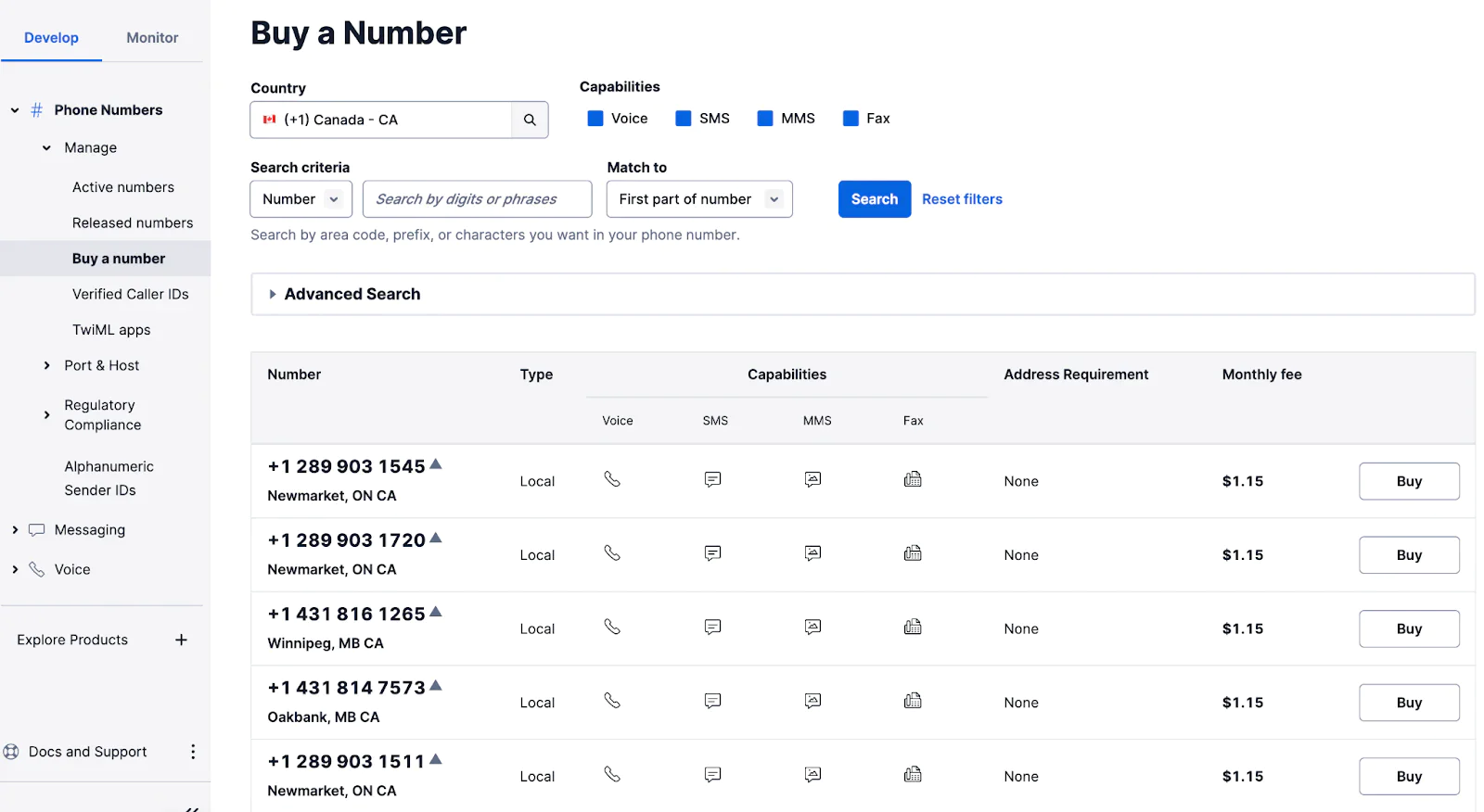
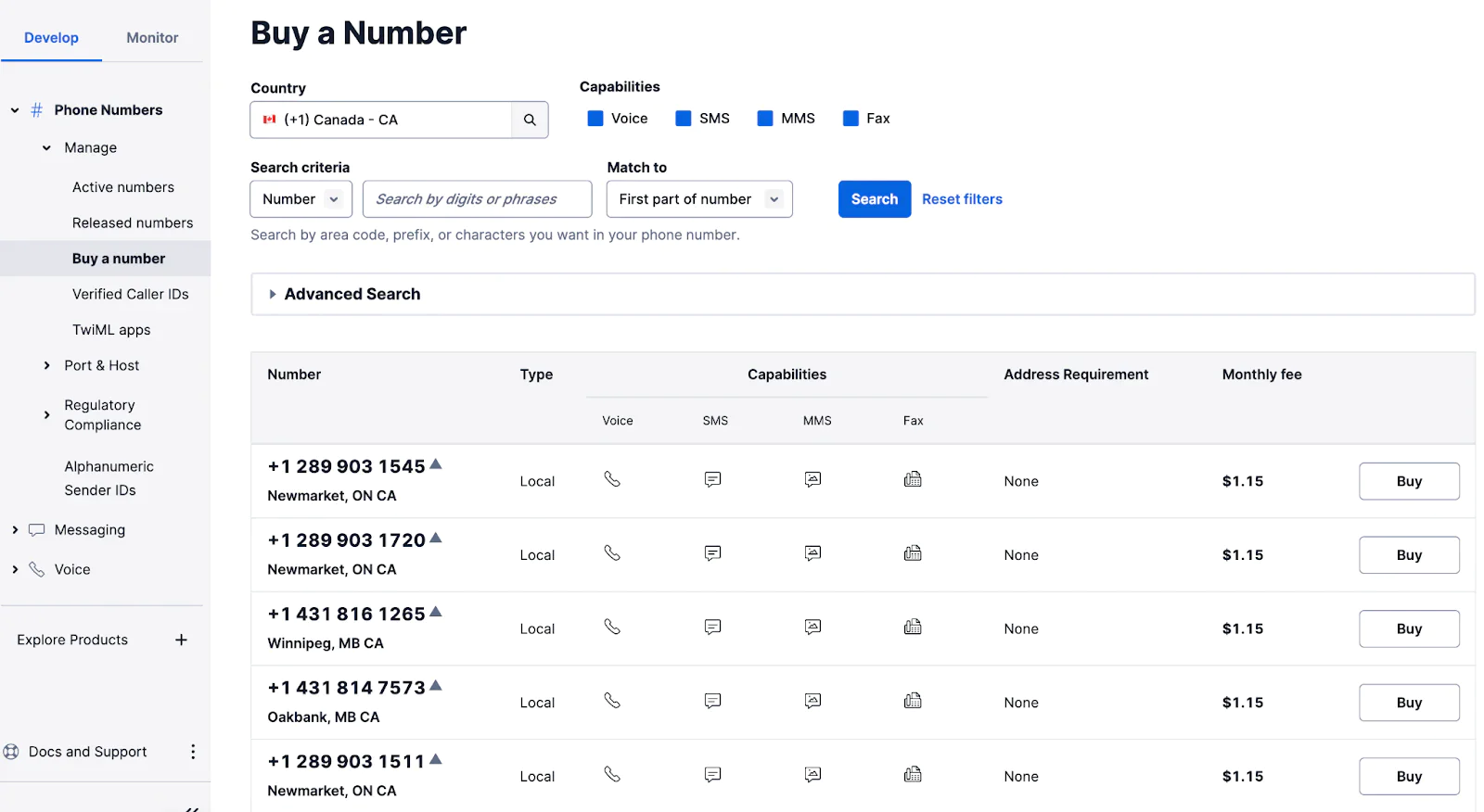
Now that we have the Account SID, Auth token and a Twilio phone number. Let’s update the local.settings.json
inside the MyTwilioProject folder:
Testing the Azure Function locally
To run the Azure Function locally, (yes it is possible, thanks to Azure Functions core tools) in the root directory of our project MyTwilioProject, run the following command:
And your function is now running locally.
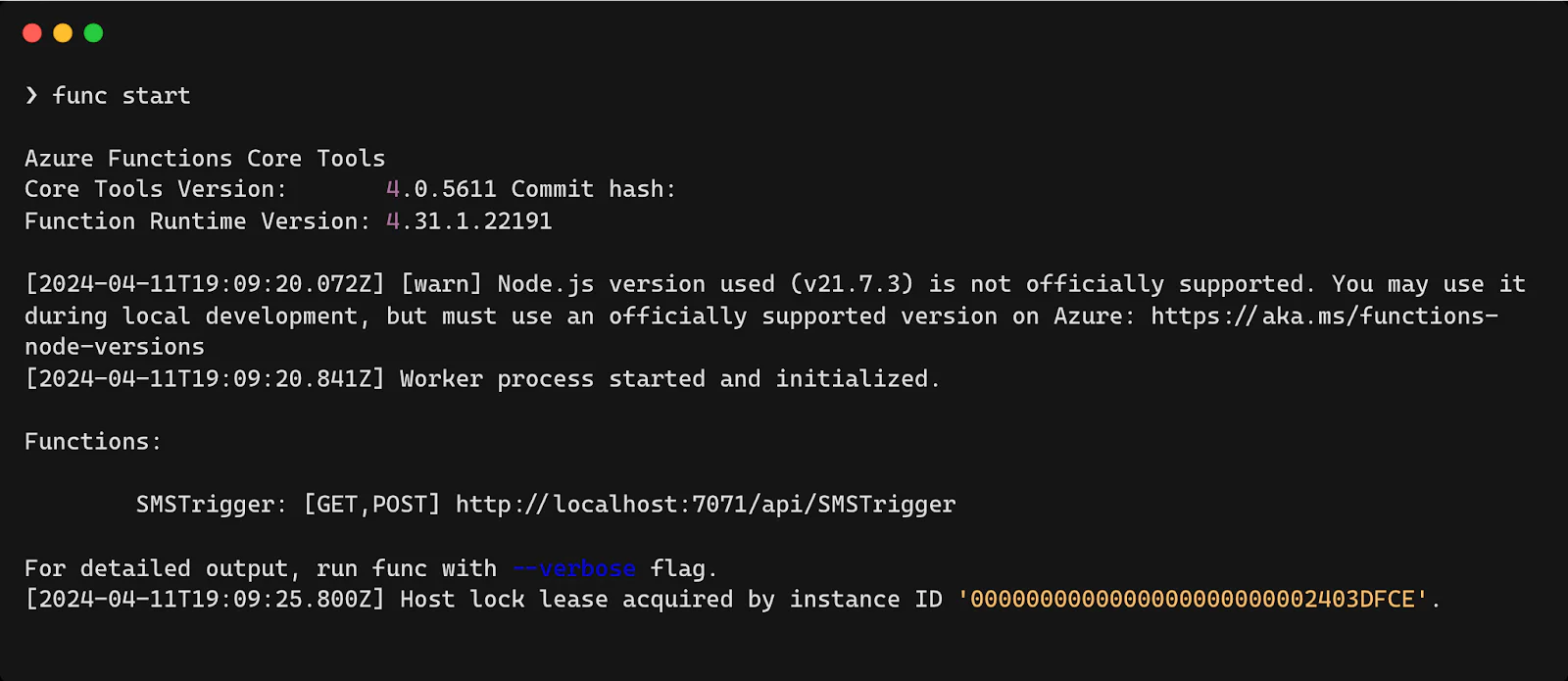
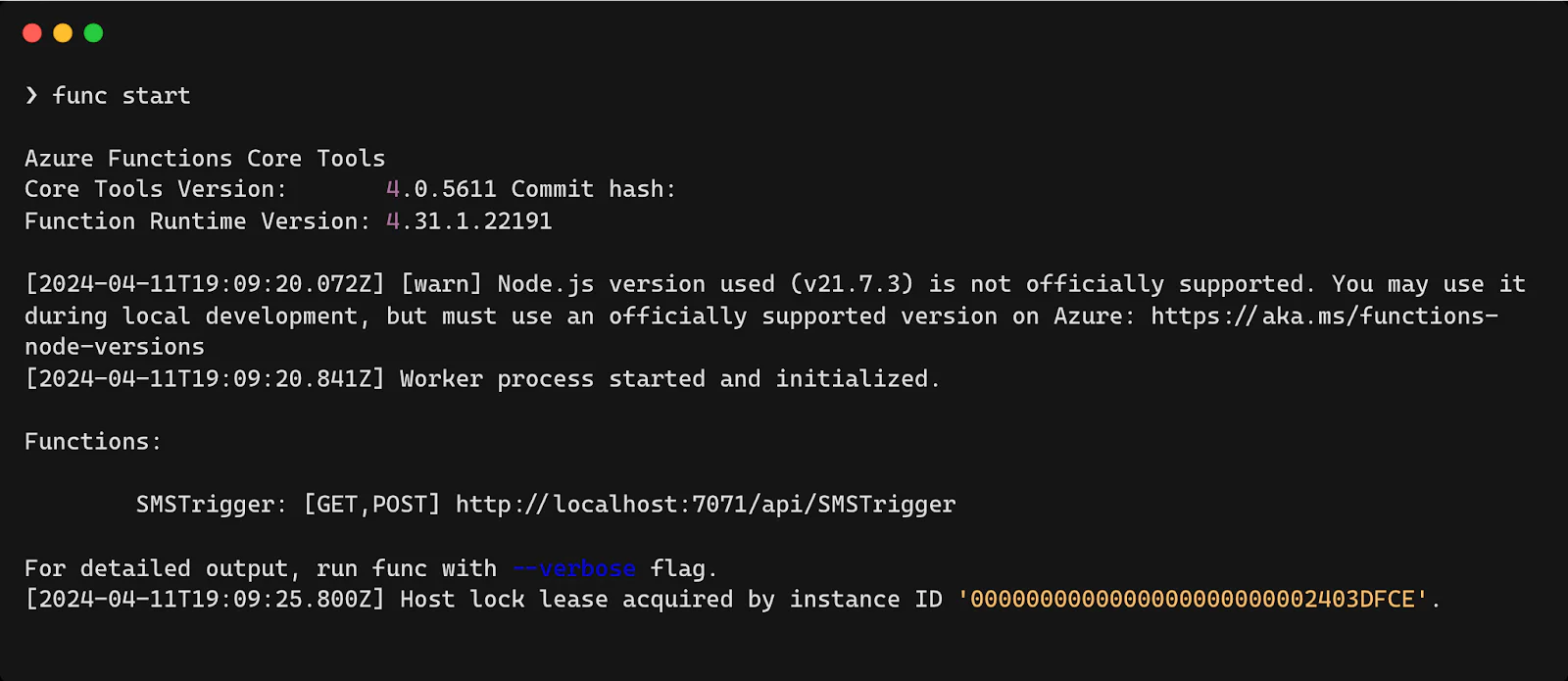
Now we can test our function by sending a POST request to the SMSTrigger URL - http://localhost:7071/api/SMSTrigger with the following query parameters:
- message
- number
You can use a tool like Postman or curl.
Here is how the request would look like in Postman.
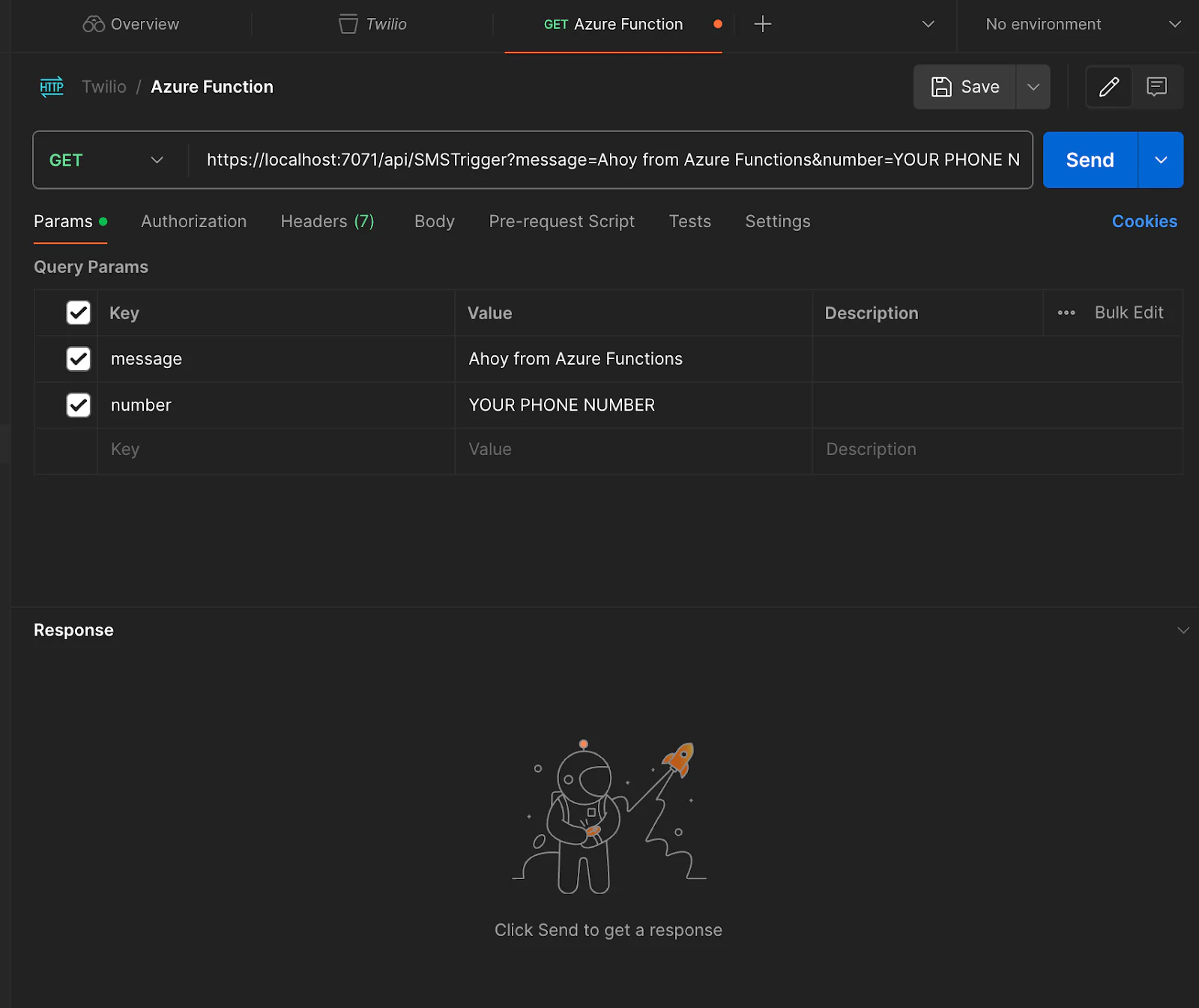
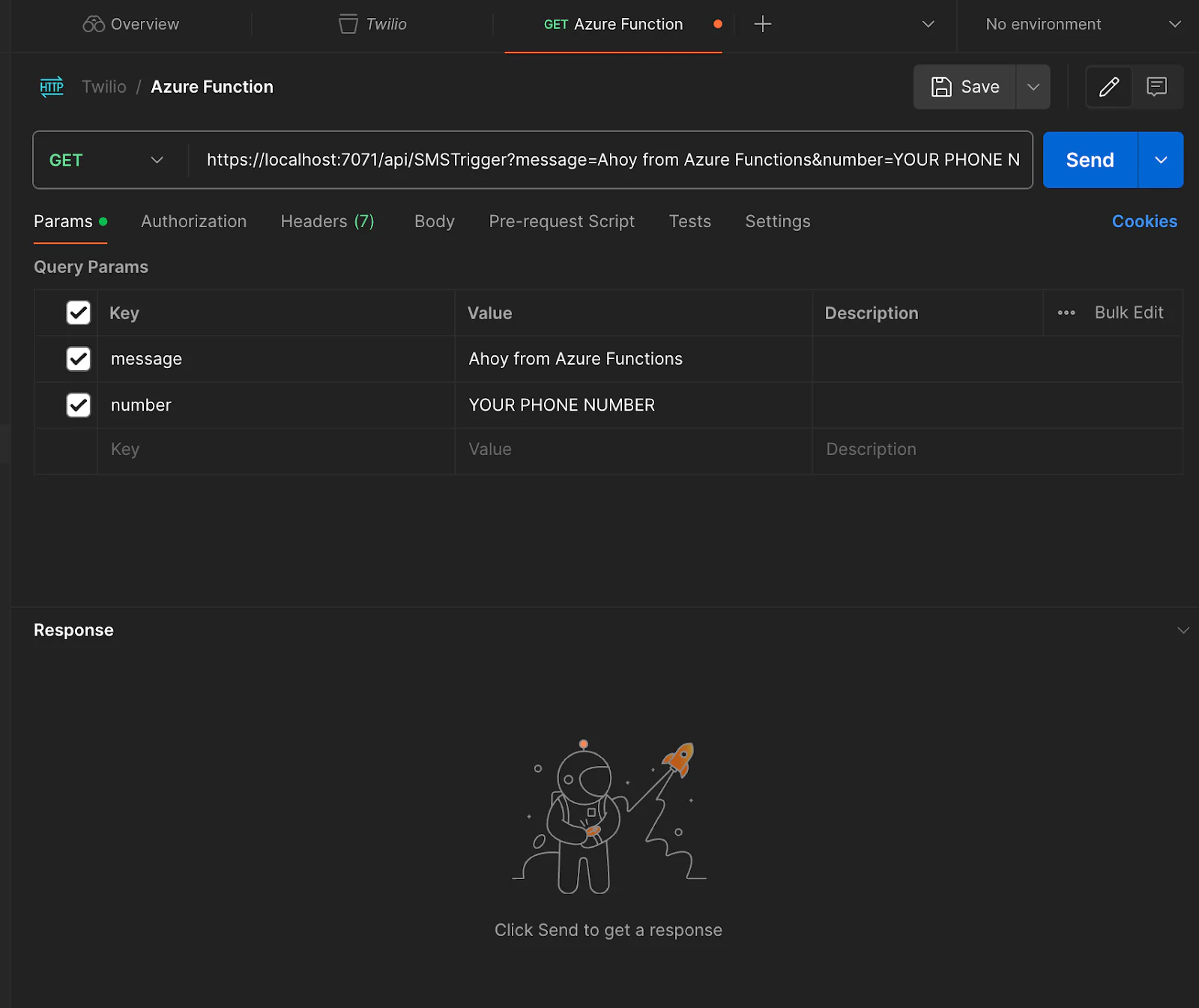
After you hit send, you will get a response “Message Sent”
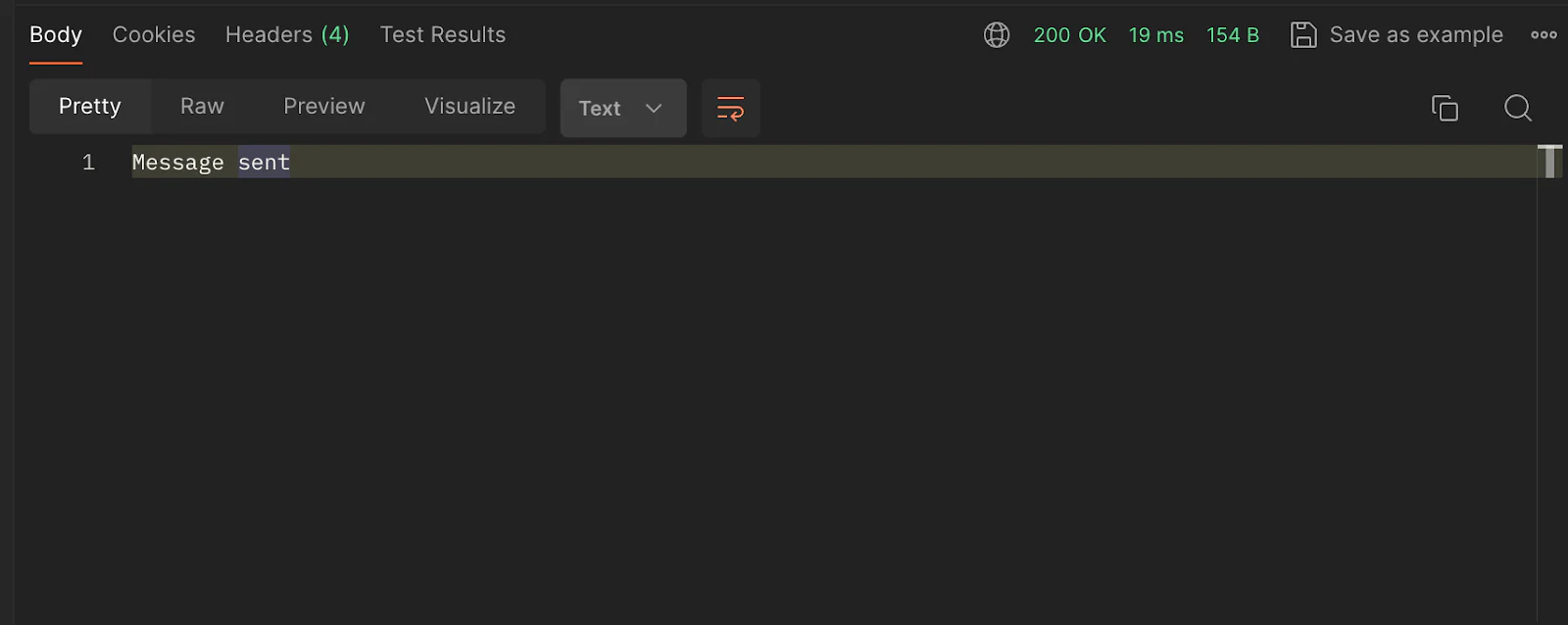
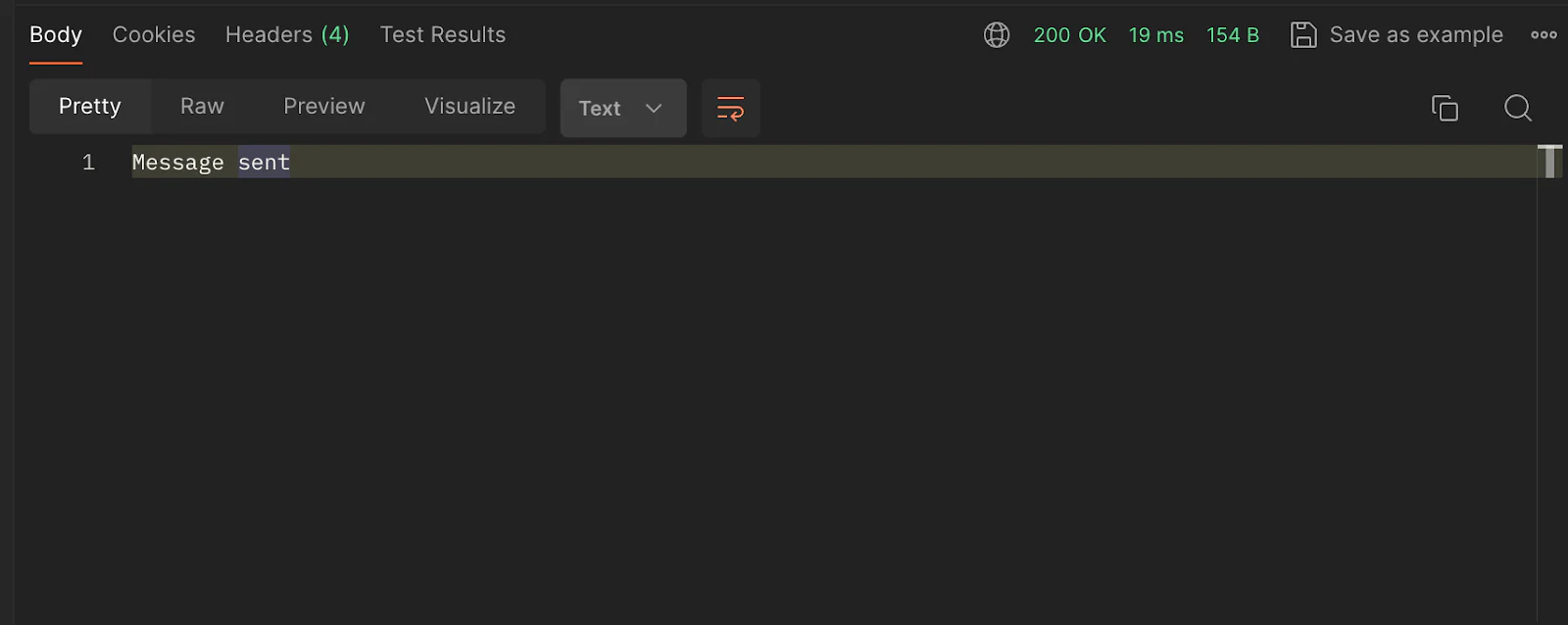
If you want to use curl:
And you will also see the output in terminal, where you have your Azure Function running locally. Similar to this one:
If you check your phone, you should have received a text message “Ahoy from Azure Functions”.
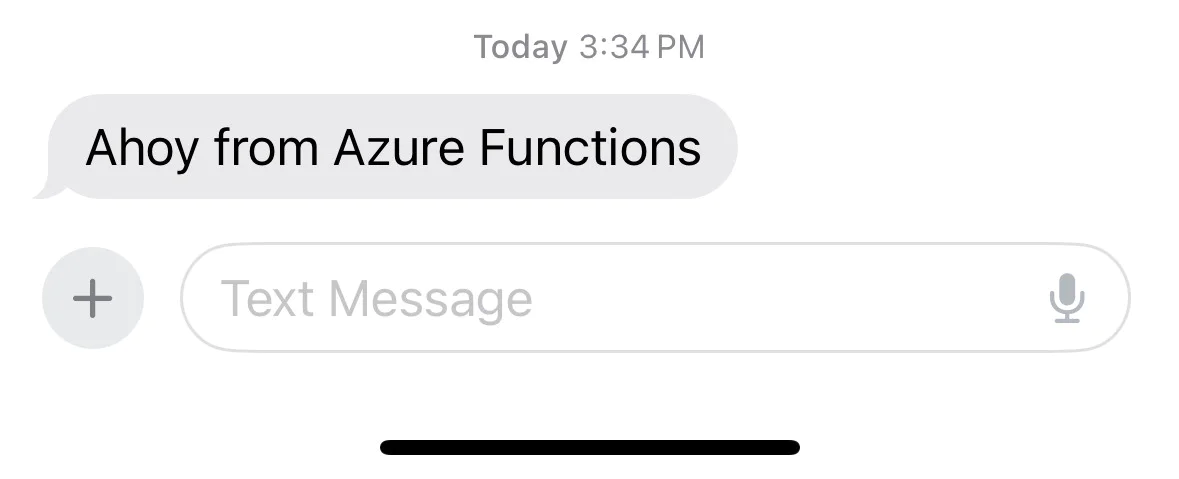
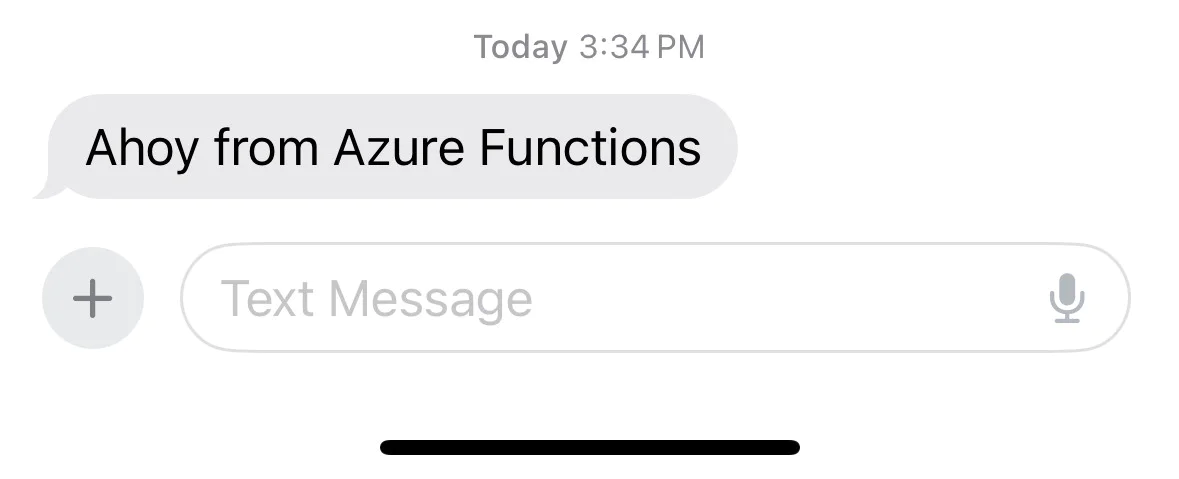
Deploying Azure Function to Azure
We have our Azure Function working locally, now it’s time to deploy it to the cloud. There are two ways you can do this, either via the Azure Portal or using the Azure CLI (either installed locally or accessed via Cloud Shell ).
I am going to show you how to deploy the Azure Function using the Azure CLI within Cloud Shell. Login into your Azure account and open the Cloud Shell, just select Try it from the upper right corner of a code block. You can also launch Cloud Shell in a separate browser tab by going to https://shell.azure.com.
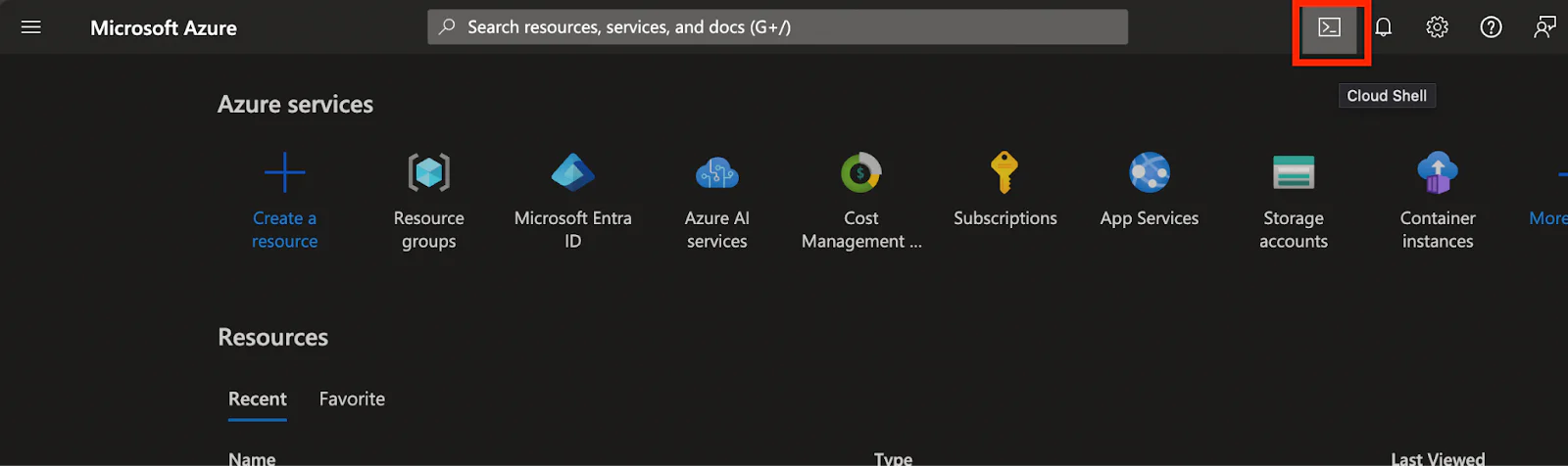
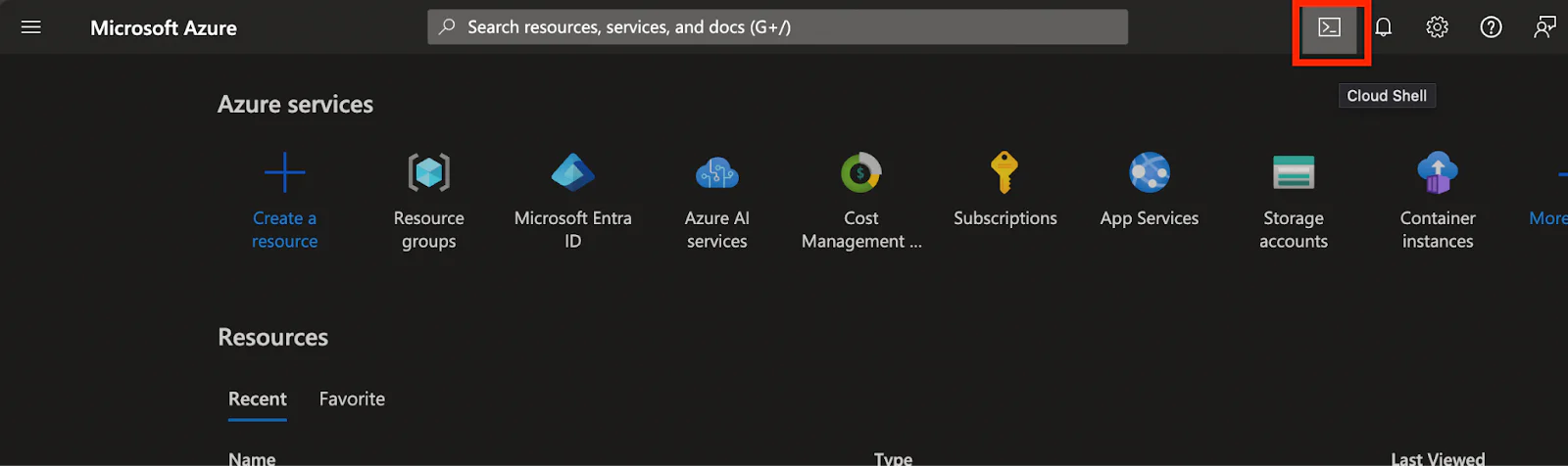
After the Cloud Shell is launched, create a new script twilio-azure-function.sh
and add the following code.
Let’s give our script the right permissions so that we can execute it.
Now, let’s run the script.
You will see that the resources will be created one by one.
Optionally you can install the Azure CLI locally and run the same script from your own machine.
If we check our Azure account now and look for the Resource Group, you will find all the resources that we just created.
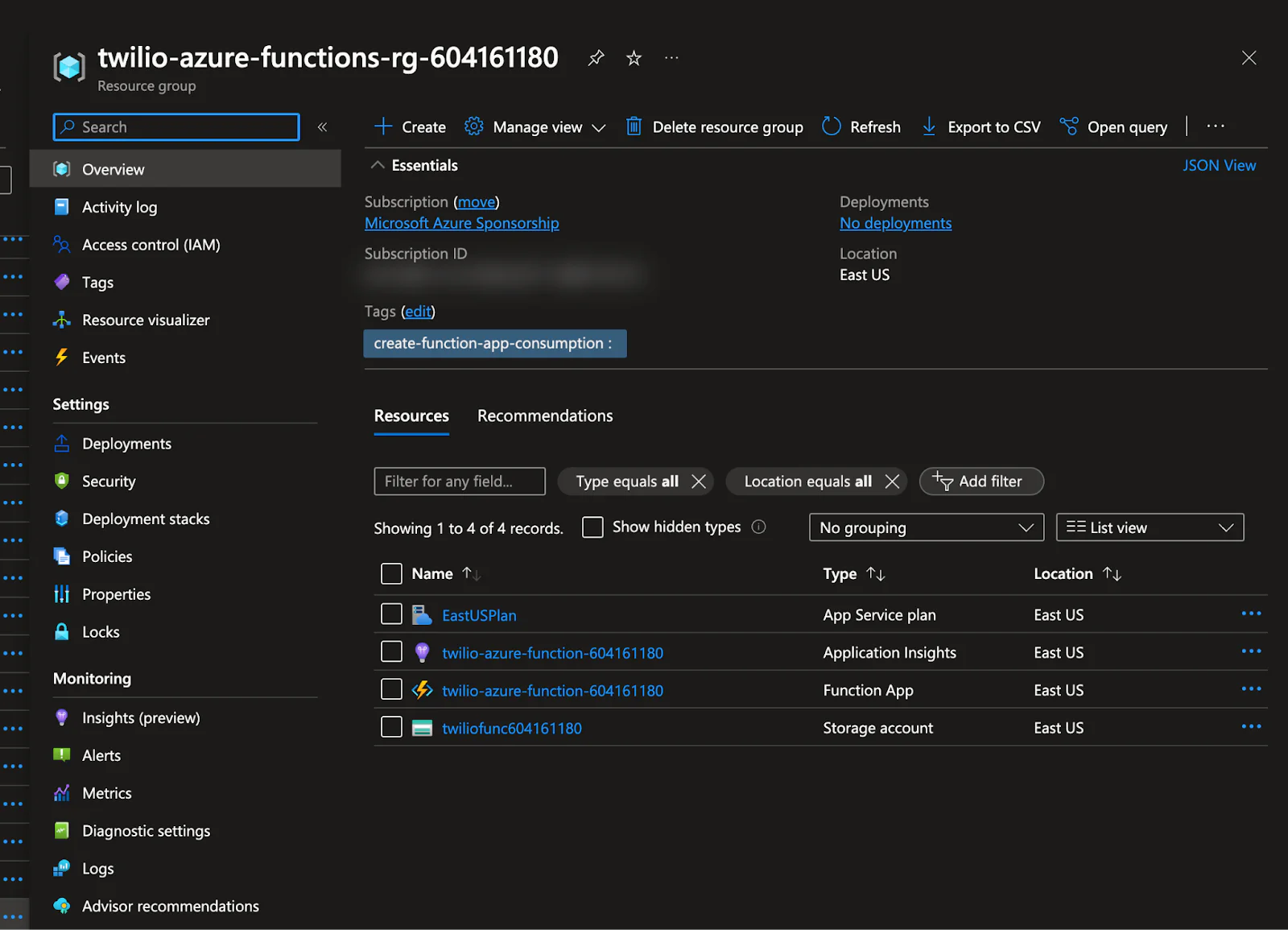
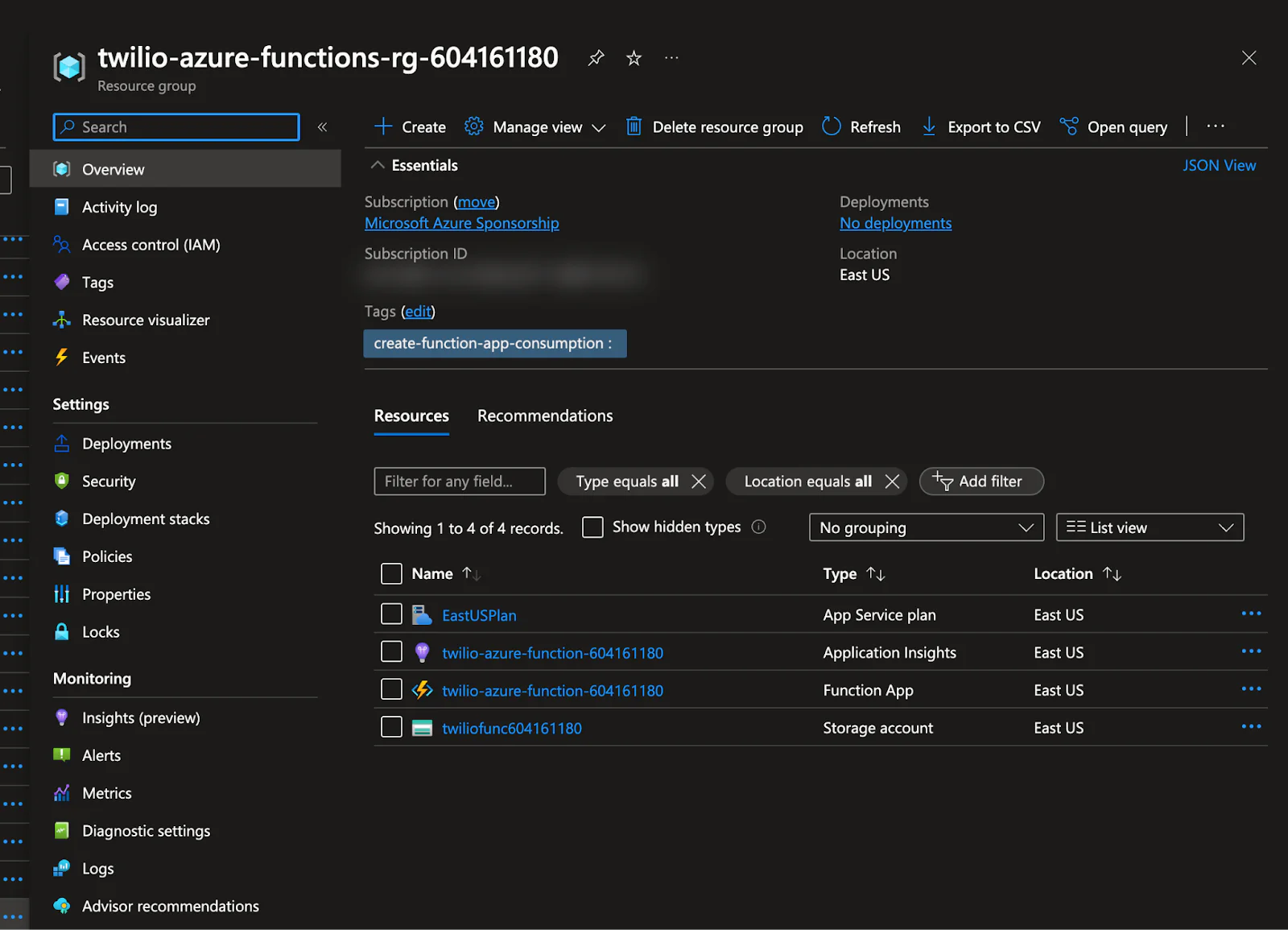
Now head over to your terminal where you have the local Azure Function running. Make sure you are in the MyTwilioProject
directory. If your local Azure Function is still running, you can stop it by CTRL + C
key combination. To deploy our local Azure Function, we will run the functionapp publish
command.
We are using the --publish-local-settings
option to automatically create app settings in your function app based on values in the local.settings.json file.
You can find your Function App Name from the Azure Portal.
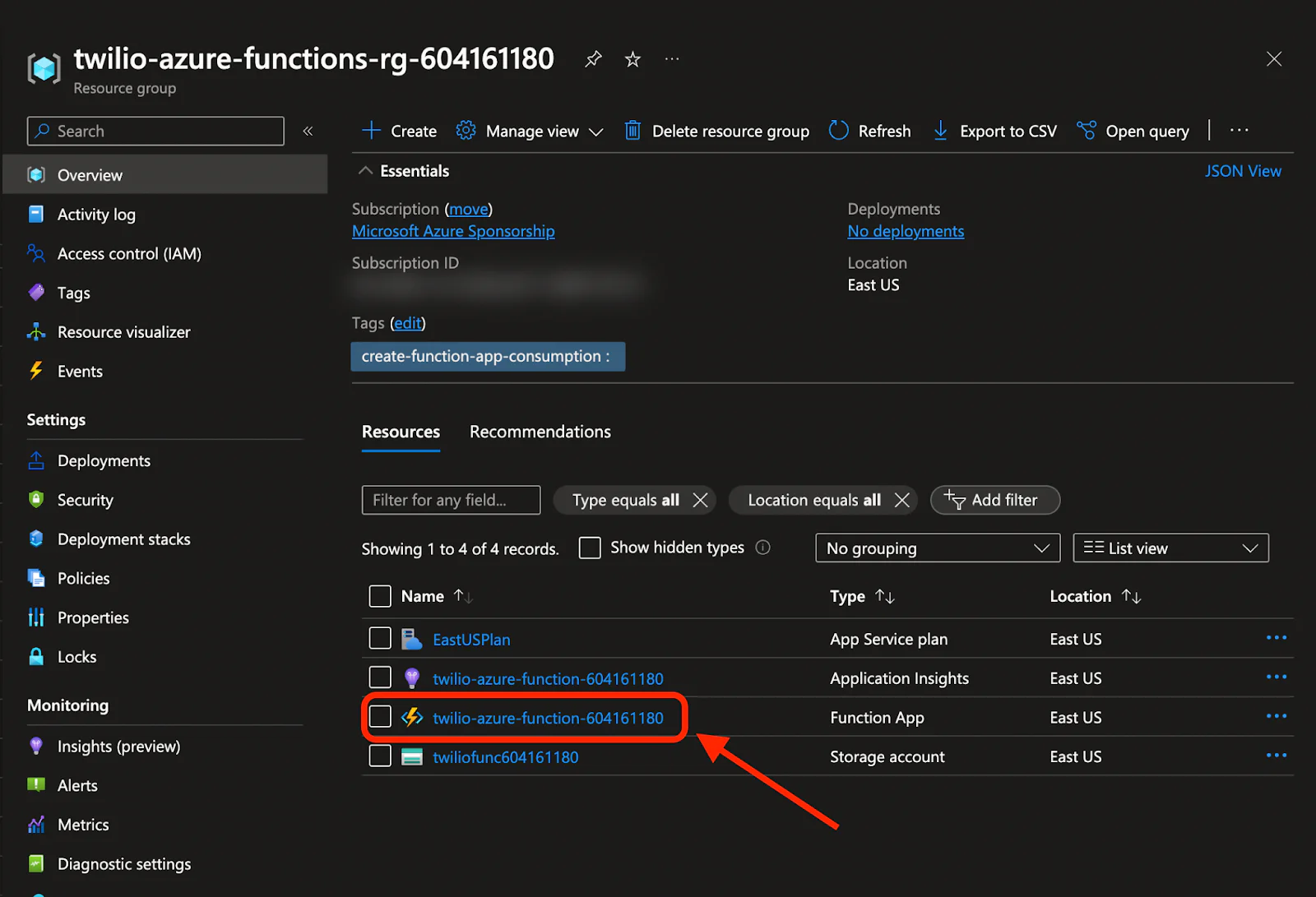
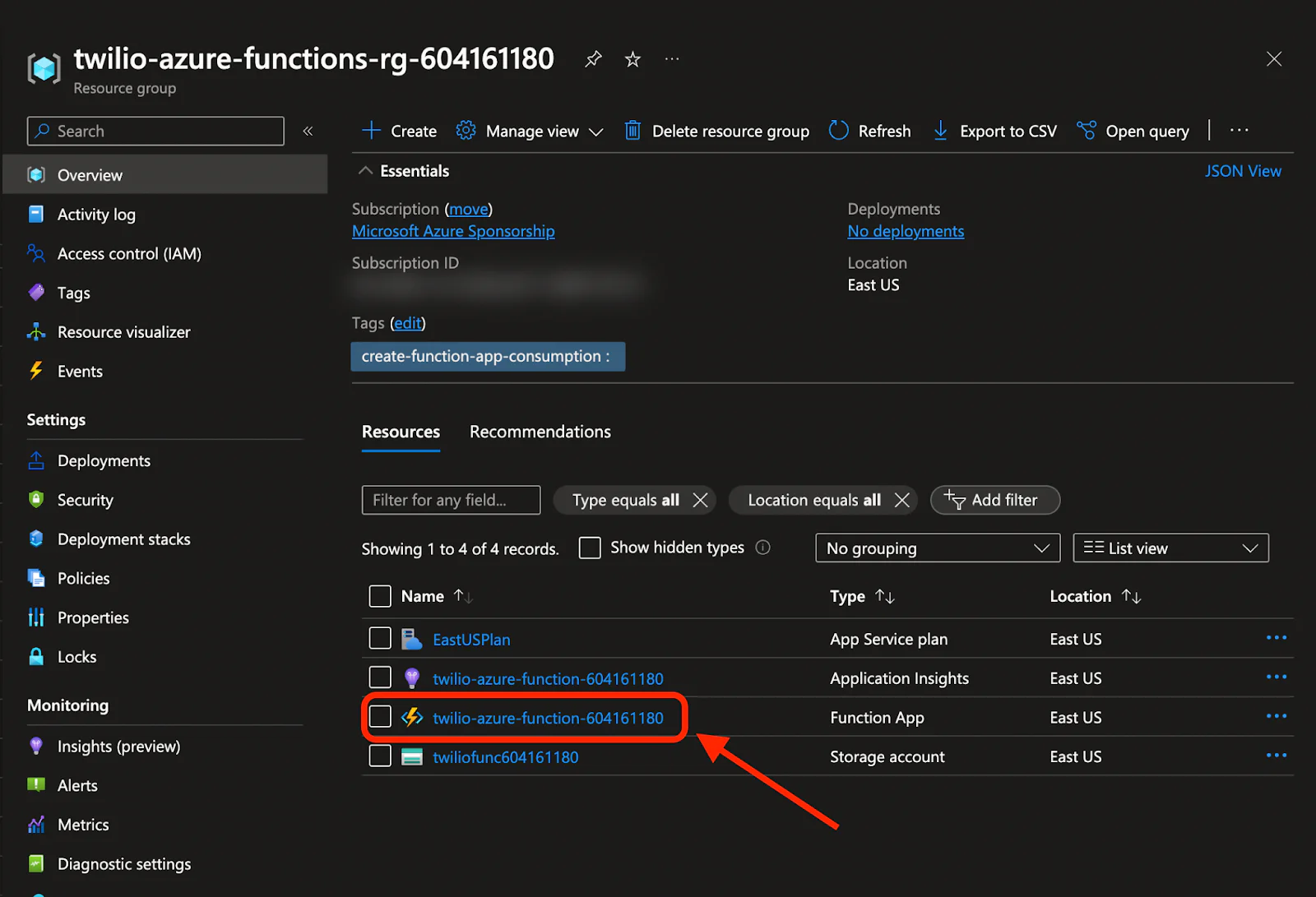
After you run the command, you might get a prompt if the local settings are different from the Azure Function settings. Type yes.
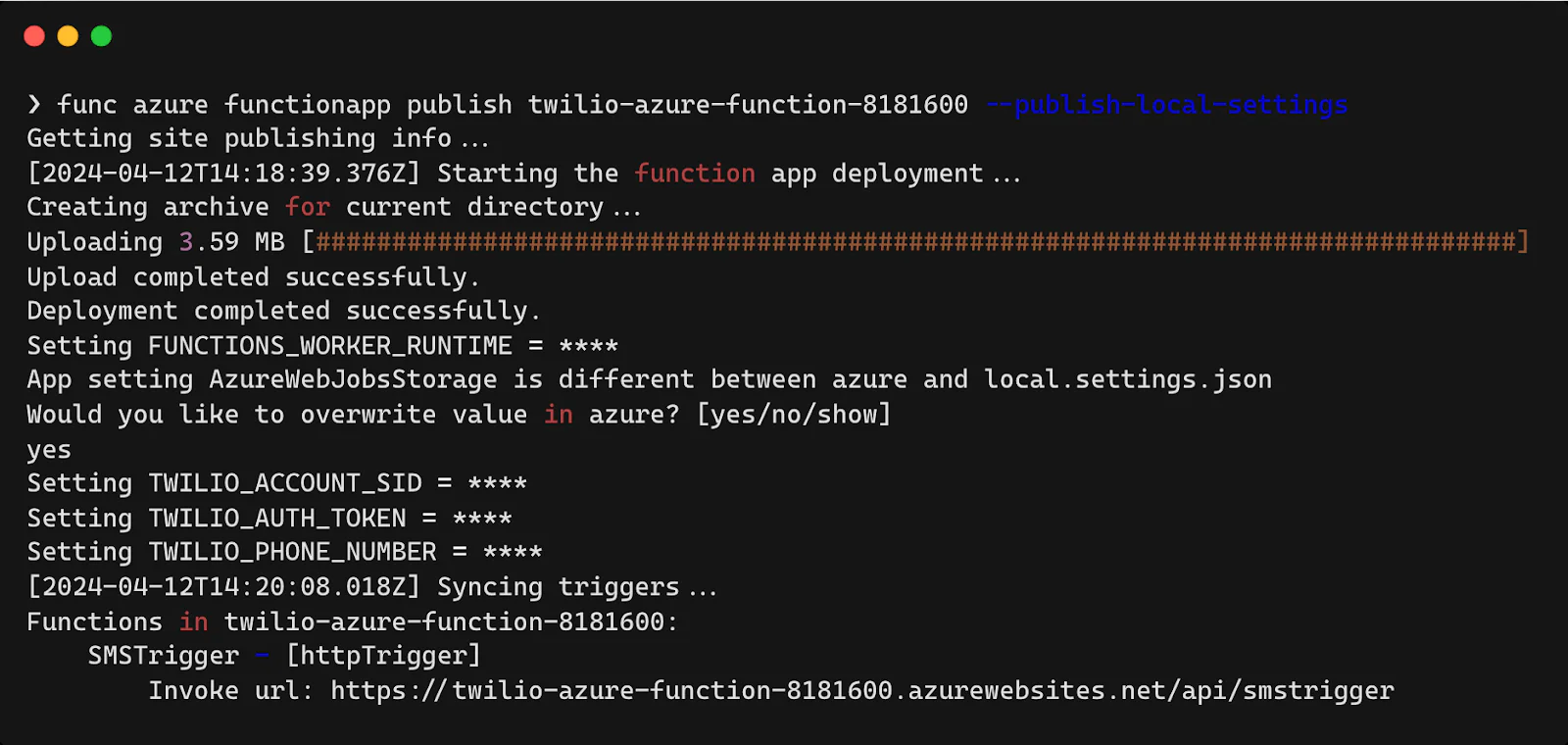
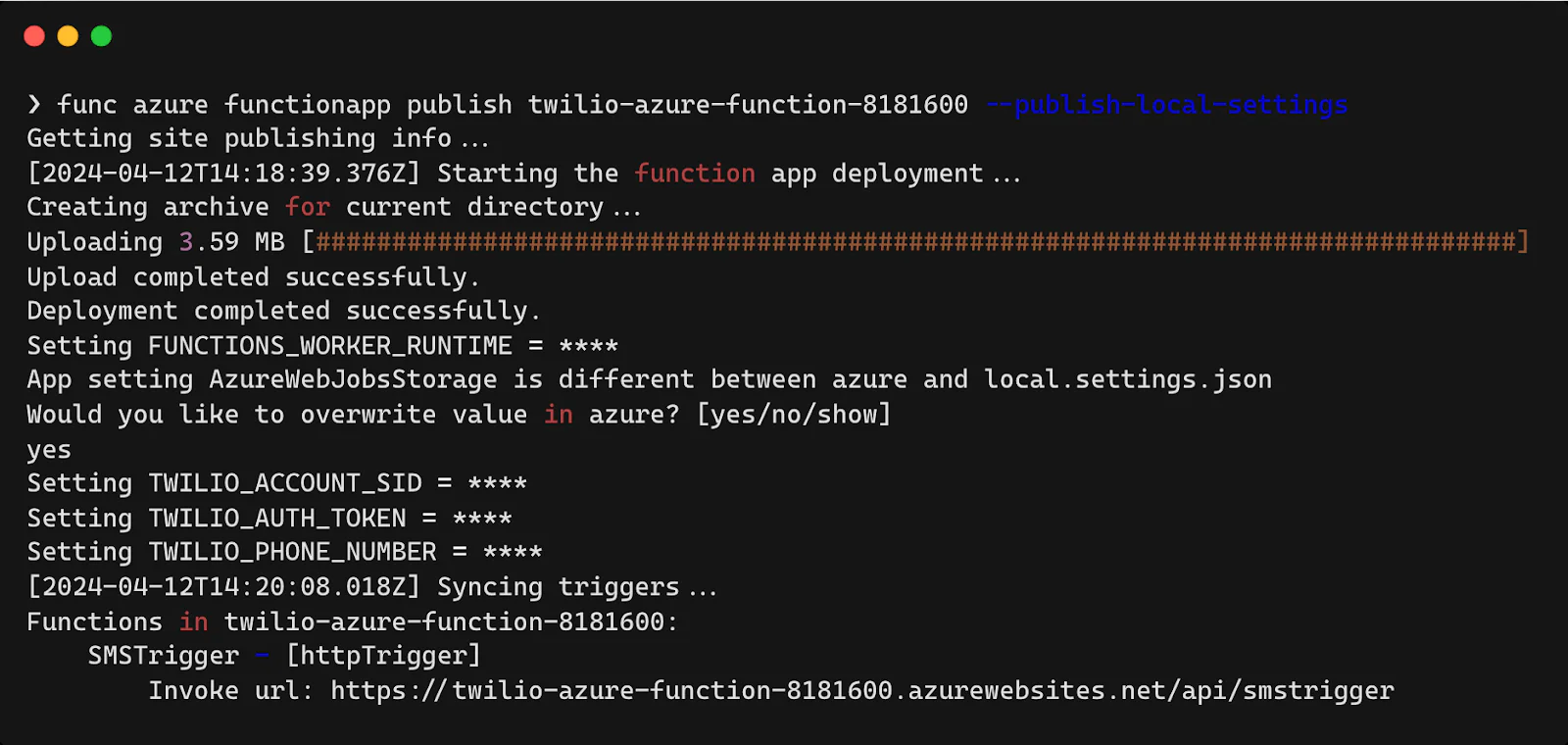
Voila! We have deployed our Azure Function to the cloud. The Invoke url
is our Azure Function HTTP Trigger, which now we can use to send SMS.
TING TING! We just received a text message from our Azure Function!
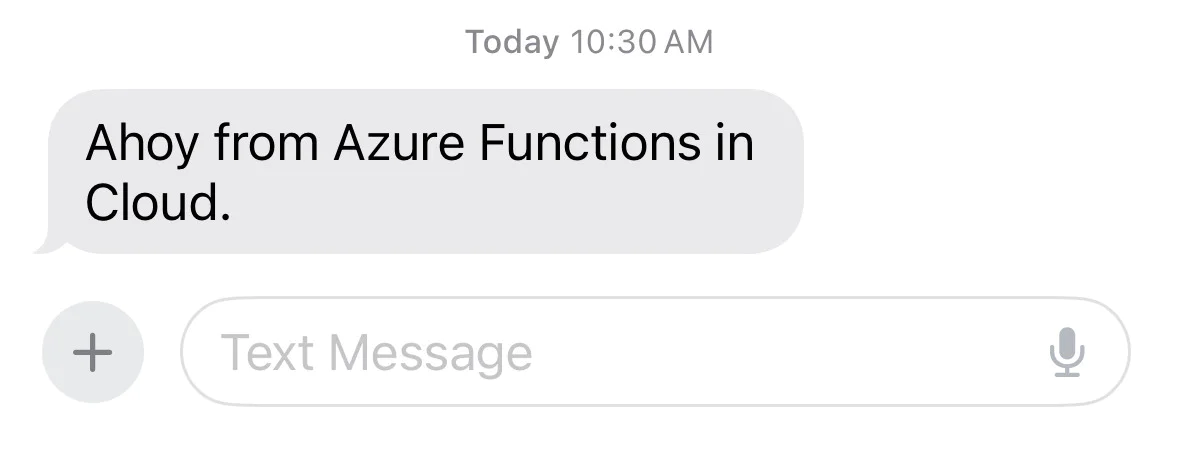
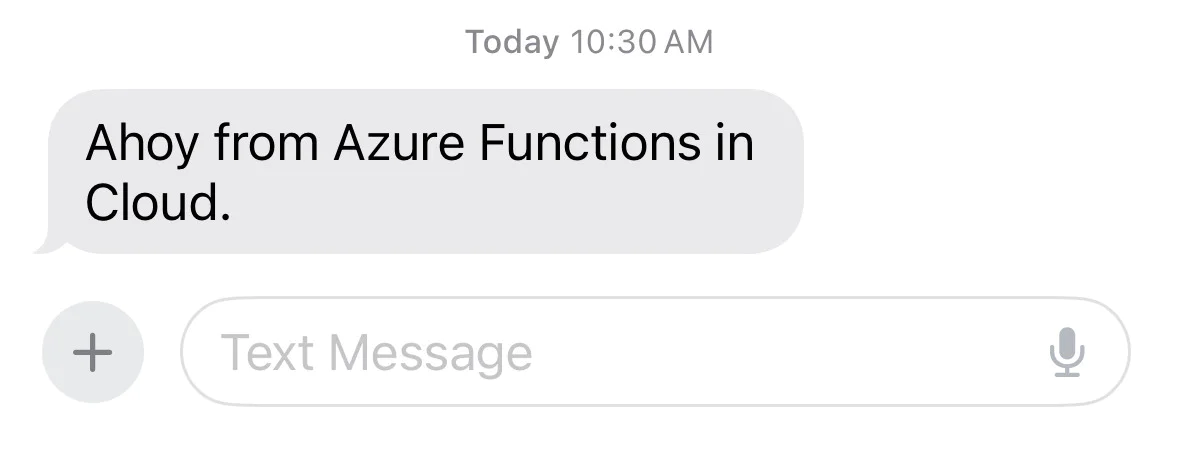
Conclusion
In conclusion, integrating Azure Functions with Twilio to send SMS messages using NodeJS offers a powerful, scalable, and cost-effective solution. This combination leverages the serverless cloud infrastructure of Azure and the seamless communication capabilities of Twilio, providing a reliable and efficient way to send SMS. With the simplicity of NodeJS and serverless architecture, developers can easily implement and maintain this solution without the need for extensive infrastructure management. This integration not only streamlines the process of sending SMS messages but also opens up possibilities for other innovative applications. I can't wait to see what you build!
Rishab Kumar is a Developer Evangelist at Twilio and a cloud enthusiast. Get in touch with Rishab on Twitter @rishabincloud and follow his personal blog on cloud, DevOps, and DevRel adventures at youtube.com/@rishabincloud.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.