Send an SMS with Rust in 30 Seconds
Time to read: 3 minutes
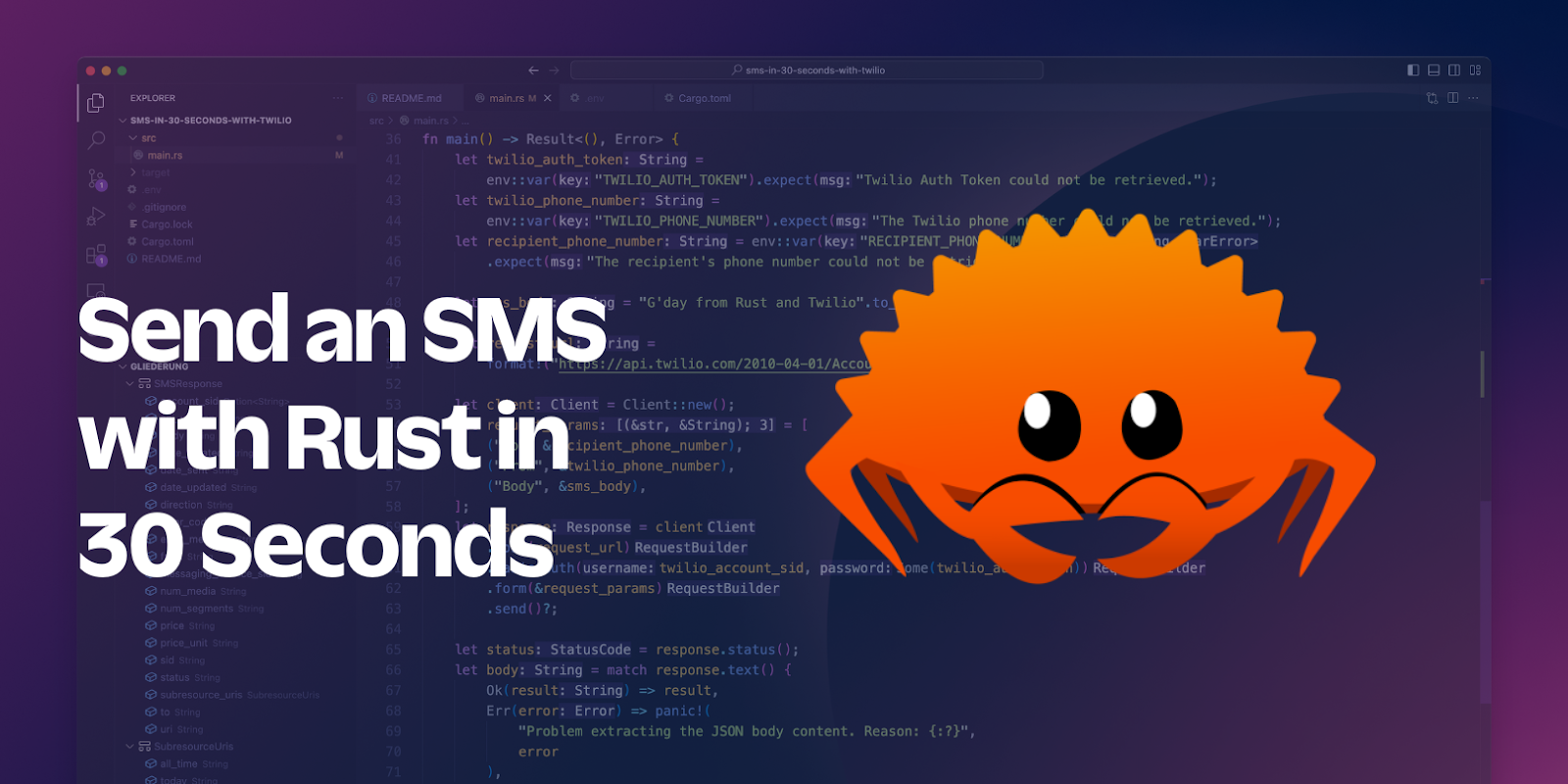
SMS is still one of the best ways to reach and engage with people around the world in 2023!
While it may seem like an old — even outdated — technology, nothing could be further from the truth. For example, according to WebTribunal SMS has an open rate of 98% and 23 billion SMS are sent every day.
So, if you want to integrate SMS into your Rust applications, then let me show you how with Twilio's Programmable Messaging API.
Prerequisites
You don't need a lot to follow along with this tutorial, just:
- A Twilio account (free or paid). If you are new to Twilio, click here to create a free account
- A mobile phone number that can receive SMS
- Rust
- Your favourite IDE or text editor for writing Rust code. I recommend Visual Studio Code
- Some prior experience with Rust would be beneficial
Generate a new Rust project
Start off by running the following commands to generate a new Rust project and change into the new project directory.
Add the required Crates
Then, open up the new sms-in-30-seconds directory on your preferred IDE and add the five required dependencies by updating the dependencies
section of Cargo.toml to match the following.
Here's what's going on:
- Reqwest: This package will simplify sending requests to Twilio's Programmable Messaging API
- rust-dotenv: This package helps keep secure information, such as API keys and tokens, out of code, by setting them as environment variables
- serde, serde_derive, and serde_json: These packages reduce the complexity of deserialising JSON responses from Twilio, so that they can be more easily used
Set the required environment variables
Now, create a new file called .env in the project's top-level directory, and paste the following code into it.
After that, retrieve your Twilio Auth Token, Account SID, and phone number from the Twilio Console Dashboard and insert them in place of the first three placeholders, respectively. Then, replace the fourth placeholder with the E.164-formatted phone number of the recipient.
Write the code
Now, update src/main.rs to match the following code.
The code initialises three structs: the first two, collectively, hold successful responses, and the third holds error responses. Then, two functions are defined which deserialise responses into the correct structs.
Then, the main()
function loads the variables you defined in .env as environment variables and initiates four variables to hold the respective information. After that, it uses Reqwest
to send a POST request to Twilio's Programmable SMS API. The request will include POST data to set the recipient (To
), the sender (From
), and the SMS' body (Body
).
If the request fails the user is notified with a short message written to the terminal. Otherwise, the response's status code is used to determine whether the response was successful or not and deserializes it accordingly.
If the request was successful, a confirmation message, including the SMS body, is written to the terminal. Otherwise, the user is informed that the SMS was not able to be sent.
Test the application
With the code written, it's now time to test it by running the command below.
First, the dependencies will be downloaded and compiled. Then, src/main.rs will be compiled and executed. After the code's finished running, you should see an SMS similar to the screenshot below.
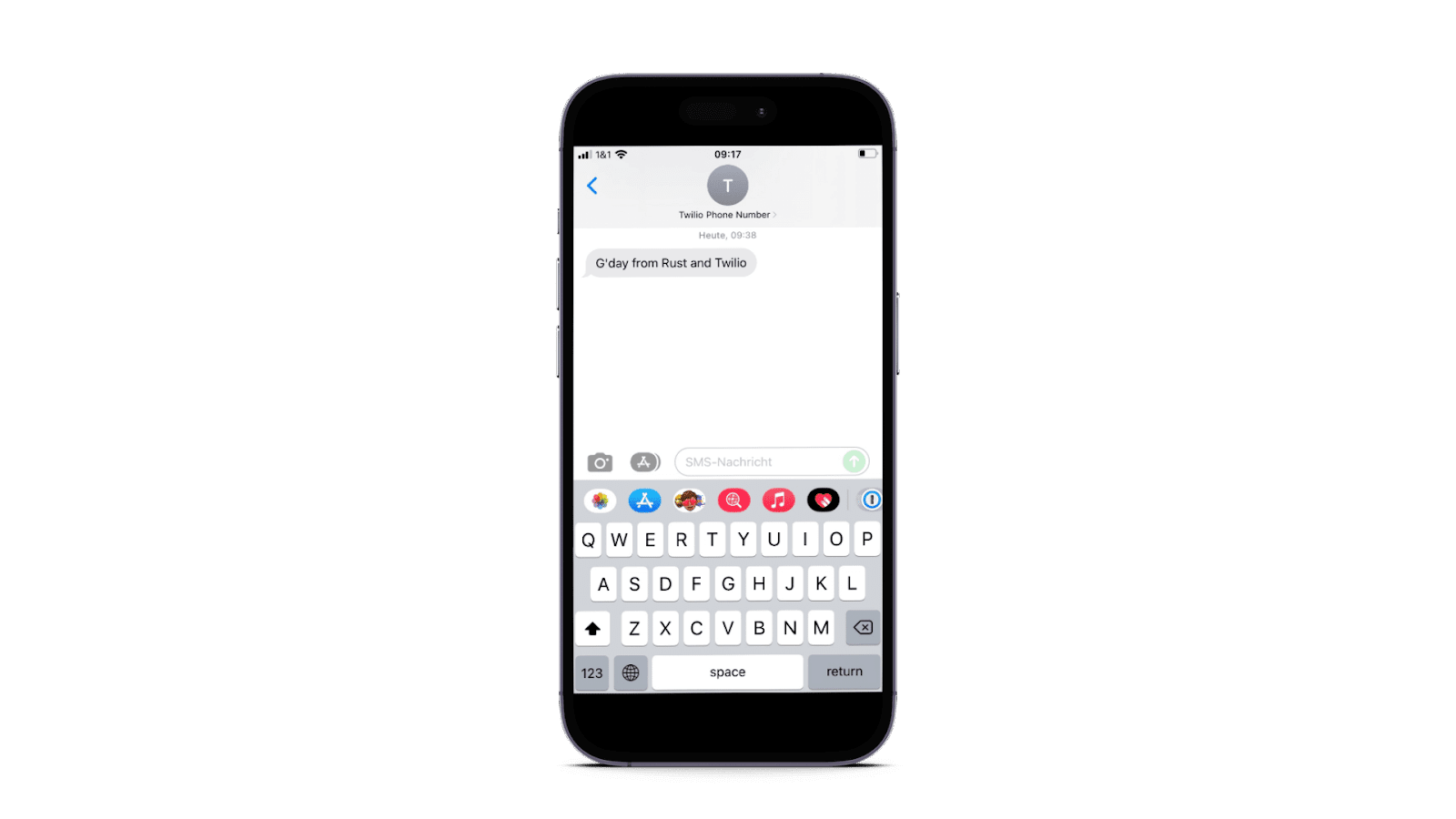
That's how to send an SMS in about 30 seconds with Rust
Well, perhaps a little longer than 30 seconds. While Twilio doesn't officially support Rust, yet, it doesn't take a lot of work to send SMS' with Rust, thanks to the simplicity of Twilio's Programmable Messaging API, and a few Rust Crates.
If you had any issues following along, grab a copy of the code from GitHub. Otherwise, I'd love to know how you'd improve the code.
Matthew Setter is a PHP/Go Editor in the Twilio Voices team and a PHP, Go, and Rust developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. When he's not writing PHP code, he's editing great PHP articles here at Twilio. You can find him at msetter[at]twilio.com, on LinkedIn, Twitter, and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.