SMS and MMS Notifications with Java and Servlets
Time to read: 2 minutes
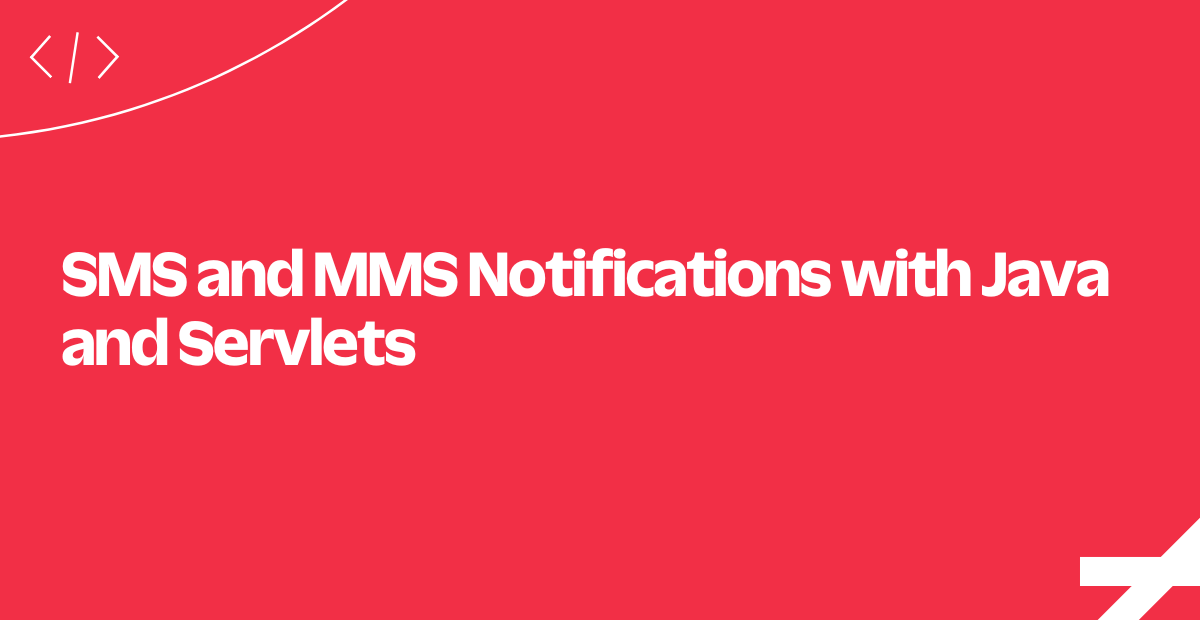
This Java Servlets tutorial will walk you through how you can automatically notify your system administrators if - well, when - something goes wrong on your server.
We'll dive in for a deeper look at all the wiring behind the scenes. Soon you'll see how easy it is to work notifications into your own application.
Sound good? Let's get started!
List Your Server Administrators
Here we create a list of administrators (and your choice of others) who should be notified if a server error occurs.
The only essential piece of data we need is a text-enabled phoneNumber
for each person you list.
Next at the plate: we'll take a look at how to set up the Twilio REST client.
Configuring the Twilio Client
To send a message we'll need to initialize the TwilioRestClient
(for details, see the Twilio Java Helper Library documentation).
We'll have to read a TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
from environment variables.
The values for your account SID and Auth Token will come from the Twilio console:
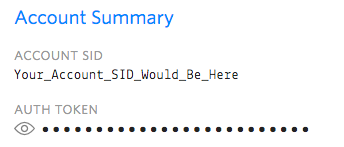
Next, let's look at how we're handling application exceptions.
Handling the Application's Exceptions
Using a Servlet Filter, we can capture any unhandled exceptions thrown by any other Servlets or Filters set up to handle a given URL.
And that's where we'll insert our logic to send out notifications to the server administrators.
Next up, let's look at how to craft a custom message upon spotting an exception.
Crafting a Custom Alert Message
Here we create an alert message to send out via text message.
Feel free to also include a picture with your notification message. Perhaps a screenshot of the application when the exception happened? Some meme from a chain email?
Next, let's look at loading the list of administrators.
Reading the Administrators from the JSON File
Next, we read the admins from our JSON file.
We're relying on the Gson Java library to convert our JSON text file into the Administrator
objects from our application.
And for our next trick, we'll show how the text messages themselves are sent. Carry on!
Sending a Text Message
There are the three parameters needed to send an SMS using the Twilio REST API: From
, To
, and Body
.
US and Canadian phone numbers can also send an image with the message. Other countries will have the picture url automatically shortened.
And that's it!
We've just implemented an automated server notification system that can send your administrators push alerts if anything goes wrong. Twilio makes it easy to incorporate these important - and useful - functions for your own use cases.
Carry on and we'll look at some other useful ways our customers have used Twilio.
Where to Next?
We've built a lot of sample applications with Twilio and Java, and we'd love to share them all. However, we'll limit our suggestions to just two:
Instantly collect structured data from your users with a survey conducted over a voice call or SMS text messages. That will help you quantify the impact of your support call handling, for starters.
If you're having issues with customers missing booked meetings, this tutorial is where you should head next. We look at sending automatic reminders as a meeting approaches.
Did this help?
Thanks for checking out this tutorial! Tweet to us @twilio to let us know what you thought or what you're building next!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.