How To Set Environment Variables
Time to read:
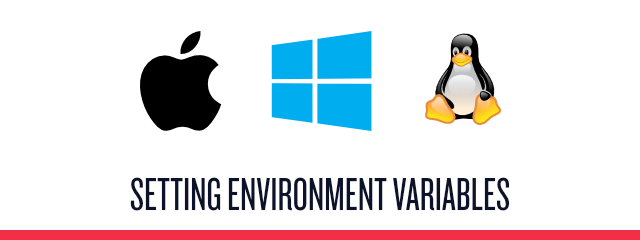
There are some things we just shouldn’t share with our code. These are often configuration values that depend on the environment such as debugging flags or access tokens for APIs like Twilio. Environment variables are a good solution and they are easy to consume in most programming languages.
Environment Variables????
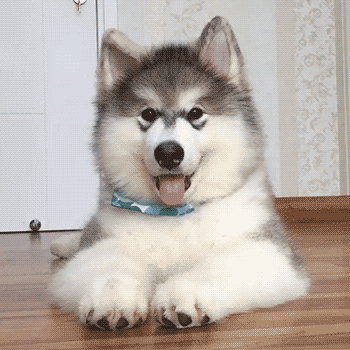
Environment variables, as the name suggests, are variables in your system that describe your environment. The most well known environment variable is probably PATH
which contains the paths to all folders that might contain executables. With PATH
, you can write just the name of an executable rather than the full path to it in your terminal since the shell will check the local directory as well as all directories specified in the PATH
variable for this executable.
Aside from ‘built-in’ variables you also have the opportunity to define our own environment variables. Since they are bound to our environment they are great for things such as API access tokens. You could have a variable set to one value on your development machine and another in your production environment without having if
-statements or special config files.
Twilio’s helper libraries for example, look for the TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
environment variables if you instantiate a client without the two values. This way you don’t have to worry about accidentally pushing sensitive credentials to a place such as GitHub.
Set Environment Variables on macOS and Linux Distributions
In order to set environment variables on macOS or any UNIX based operating system you first have to figure out which shell you are running. You can do that by running in your terminal the command:
The end of the output should indicate which shell you are running. The typical shell is the bash
shell which you will be using in this example. But the steps are similar for other shells like zsh
or fish
.
In order to set an environment variable, you need to use the export command in the following format:
Since this would only set this for the current session you need to add this command into a file that is executed for every session. For this, open the .bashrc file in your home directory with your favorite code editor. Then, add the following line somewhere in the file:
Replace <YOUR_ACCOUNT_SID>
with your actual Account SID from your Twilio Console. Save the file and open a new terminal instance to test if it worked by running:
You should see the value that you stored in it.
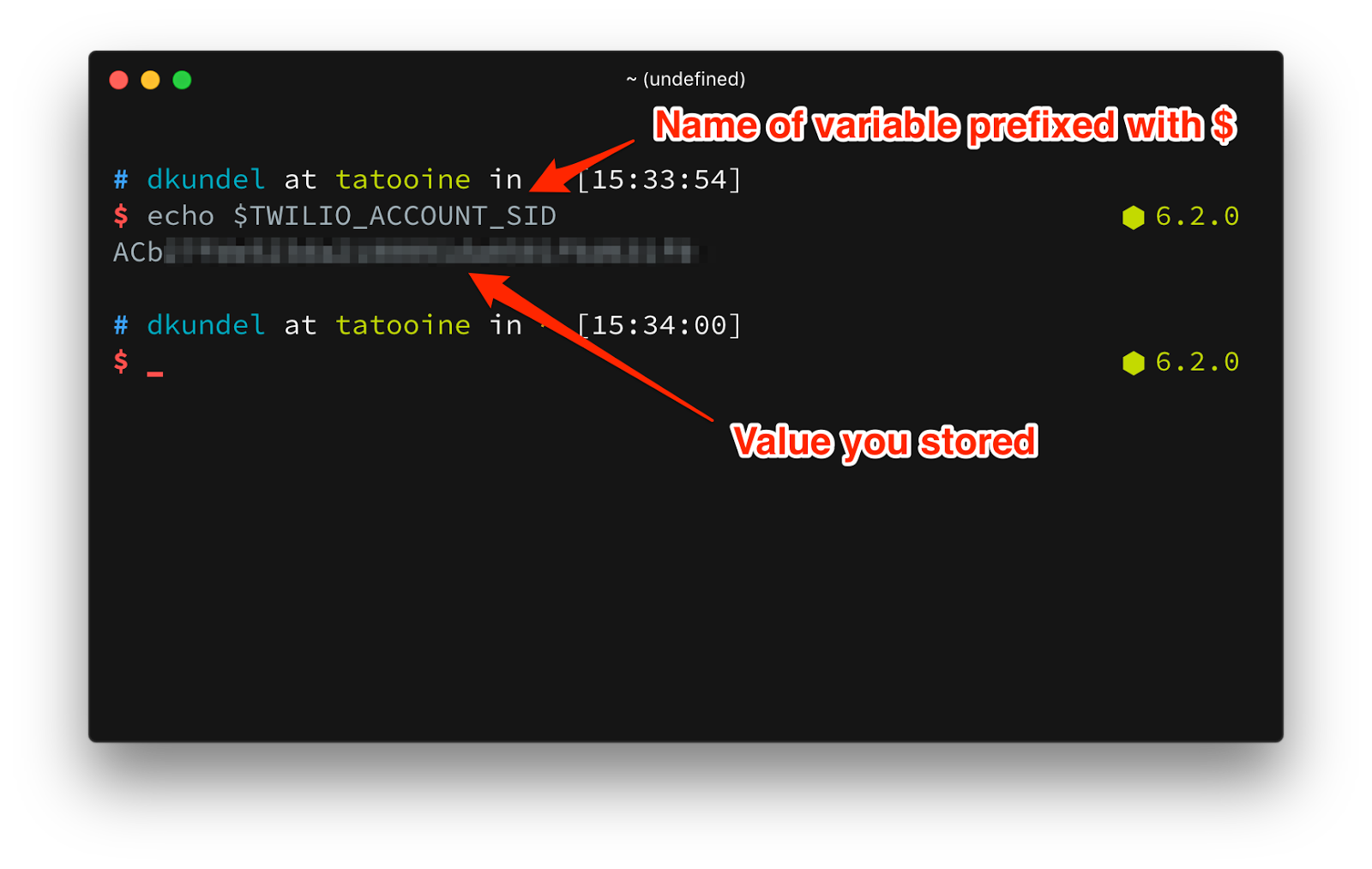
Windows Environment Variables
If you're using a Windows machine, you have a couple of ways to set environment variables. The most common methods are to use PowerShell, CMD, or the Graphical User Interface (GUI).
There are three different locations you can store environment variables:
- In the current process. The current process is most commonly the shell you are running, like PowerShell, CMD, Bash, etc. However, the current process could also be other applications including yours. Any child process started from the current process inherits that process' environment variables. The environment variables are removed when the process ends. This means you need to reconfigure the environment variables whenever you open a new shell.
This is also how environment variables are stored on macOS and Linux distributions. - In the user registry. The environment variable is available to all processes started by the user, and no other users can access those environment variables (without elevated privileges).
- In the machine registry. The environment variables are available to all users and processes, and setting environment variables for the machine requires elevated privileges.
In which location should you store the environment variables?
If you're running your application from a shell and don't want the environment variables to stick around, set the environment variables for the current process. If you do want the environment variables to stick around, store them in the user registry. If you want to configure environment variables for all users, not just for a specific user or yourself, then store them in the machine registry.
Set Environment Variables using PowerShell
While PowerShell is also supported on macOS and Linux distributions through PowerShell Core, Windows PowerShell is preinstalled on Windows and is the recommended shell for the Windows platform. The following cmdlets for configuring environment variables will work on Windows, macOS, and Linux.
The most common and easiest way to set environment variables in PowerShell is to use the $Env
variable, like this:
After $Env
, add a colon, followed by the environment variable's name, followed by the equals sign, followed by the value you want to use. This will set the environment variable for the current process, and will be inherited by any child process you start from this shell.
The following two alternatives have the same result, but are less common:
To persist environment variables across shell instances, you can save the cmdlets into your profile script, or on Windows, you can save them in the user registry or machine registry like this:
The two commands above result in a no-op on macOS and Linux because there's no concept of User or Machine environment variables, only process environment variables. Consult the Microsoft documentation on PowerShell environment variables for more details.
Set Environment Variables from CMD
Cmd, otherwise known as cmd.exe and the Command Prompt also comes with all installations of Windows. You should probably use PowerShell, but if you can't, here's how you can set environment variables from cmd and batch files.
To set an environment variable you can use the set
command, like this:
This command will set the environment variable for the current process, and child processes will inherit the environment variables. However, when you close cmd the environment variables will be lost.
To persist the environment variables across processes, you can store them in the user and/or machine registry using the setx
command.
These commands have more capabilities which you can dig into by reading the set command and setx command docs.
Set Environment Variables using the Graphical User Interface
Setting environment variables in Windows using the GUI is hidden behind several layers of settings dialogs. To open the respective interface you first have to open the Windows Run prompt. Do so by pressing the Windows
and R
keys on your keyboard at the same time. Then, type sysdm.cpl
into the input field and hit Enter
or press Ok.
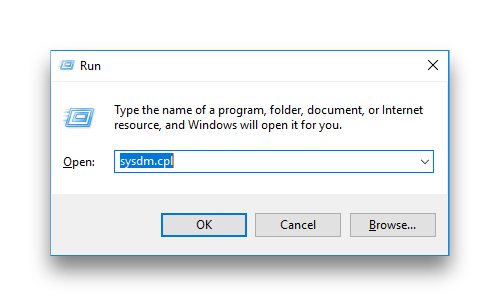
In the new window that opens, click on the Advanced tab and afterwards on the Environment Variables button in the bottom right of the window.
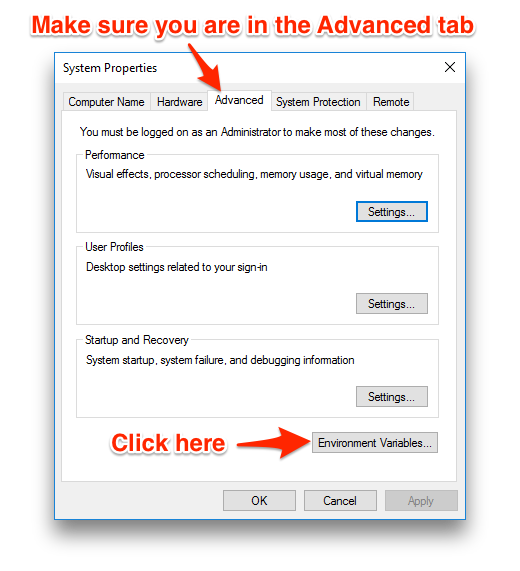
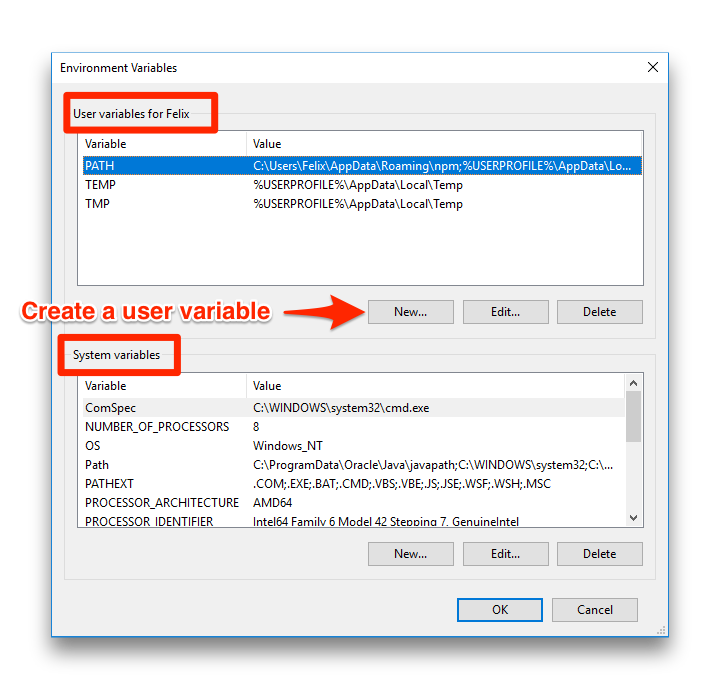
The window has two different sections. One is the list of environment variables that are specific to your user. This means they aren’t available to the other users. The other section contains the system-wide variables that are shared across all users.
Create a user specific variable by clicking the New button below the user-specific section. In the prompt you can now specify the name of your variable as well the value. Create a new variable with the name TWILIO_ACCOUNT_SID
and copy your Twilio Account SID from the Console. Press Ok in the prompt to create the variable, followed by Ok on the Environment Variables window. You are all set now.
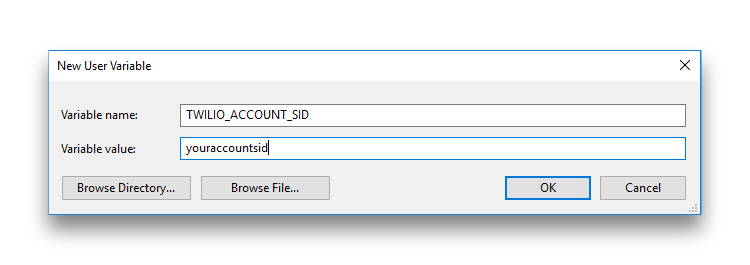
To test if it worked open the Command Prompt by pressing Windows
+ R
and typing cmd.exe
. If you have the Command Prompt open already, make sure to restart it to ensure your changes are applied. Inside the Command Prompt execute the following command:
This should print the value that you saved in the environment variable.
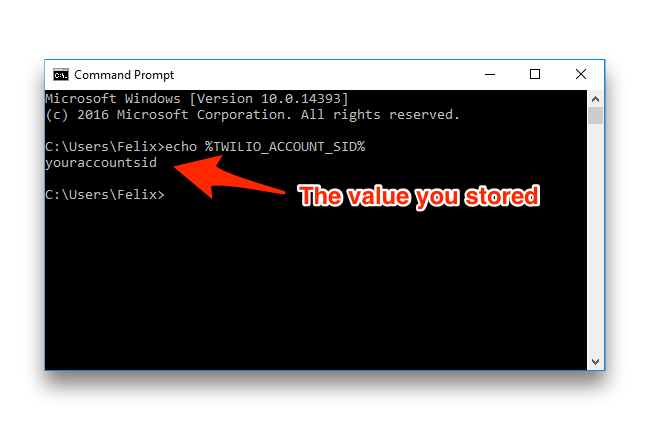
Use .env files
In some situations you only need an environment variable set for only a single project. In that case .env files are a great solution. They're files inside your project in which you specify environment variables and afterwards you use a library for your respective programming language to load the file which will dynamically define these variables.
There are libraries for most programming languages to load these files. Here are a few:
- Ruby
- Node.js
- Python
- C#, F#, VB.NET (dotnet-env or dotenv.net)
- Java (tutorial)
- PHP
Create a .env file in your project folder (typically at the root) and place the key value pairs in there. This can look like this:
Now all you need to do is consume the respective library and then you can use the environment variable. In Node.js, for example, the respective code would look like this:
Cloud Providers
Setting environment variables on your local development machine or in a VM is only half the work. What if you are hosting your application in a cloud environment such as Heroku, Azure, or AWS, or you've wrapped it in a Docker container? Luckily, all of these providers support ways to define environment variables.
If you can’t find your cloud host among this list it doesn’t necessarily mean there is no way to configure environment variables. Make sure to check their documentation for more on it.
Summary
That’s it! Now you can set environment variables which means that you can take all of your environment based configuration values out of your code and make your code more flexible and safe!
Be mindful that these values are still stored in plain text. If you are planning to store very sensitive values you should look into something like a secret storage solution such as Vault.
If you have any questions or would like to show me the awesome thing you are currently building, feel free to send me an email to: dkundel@twilio.com or contact me on Twitter @dkundel. I can’t wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.