Advanced Schedules for Twilio Studio
Time to read: 2 minutes
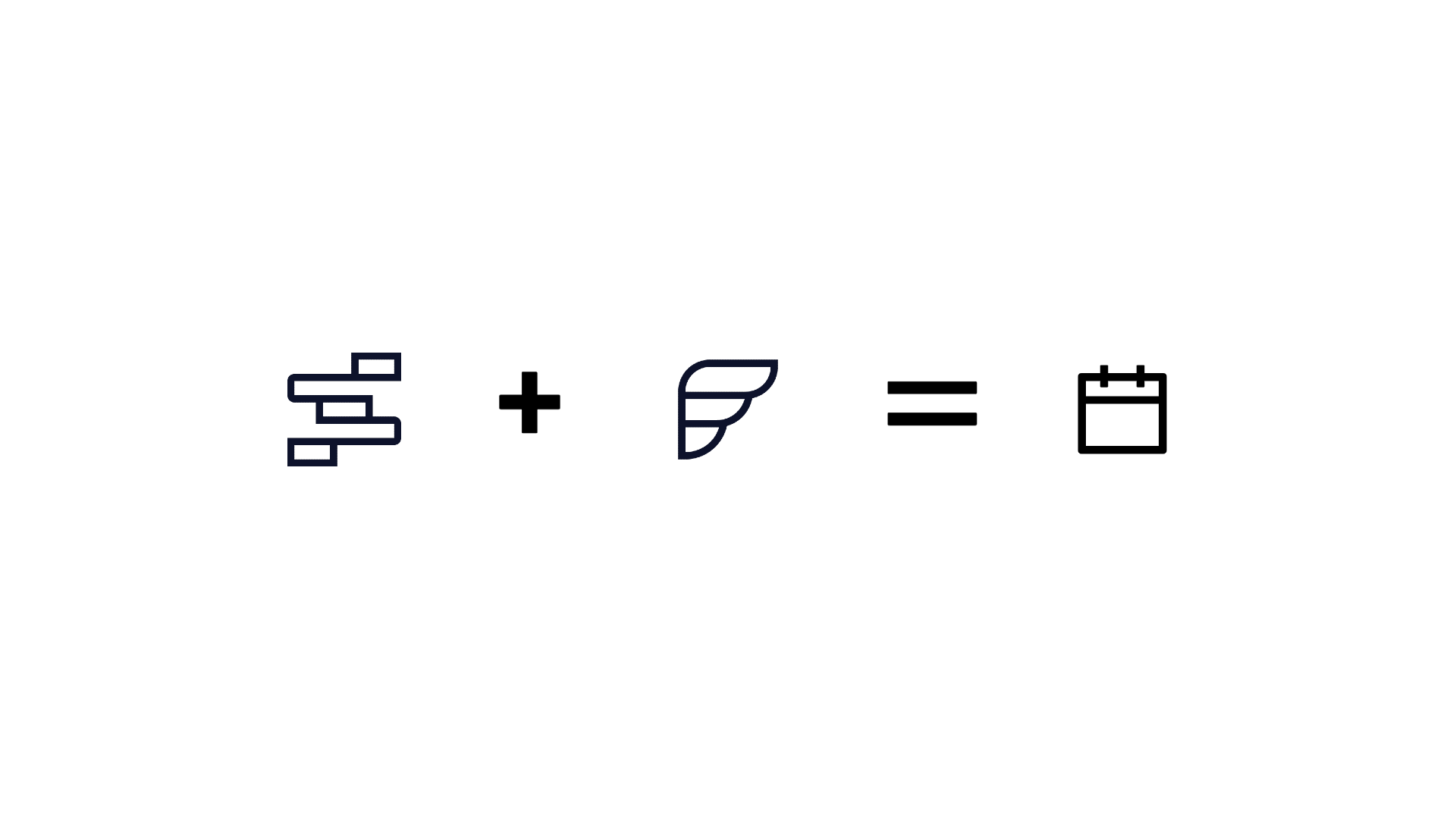
Recently, one of my customers asked me to design a scheduling function for them. Here were their requirements:
- Both Studio Flows and Web Chat had to use the same function
- The Function needed to handle holidays, as well as days with irregular hours of operation
- The customer wanted to play a special message in the IVR during the holiday season. The scheduling function has to be versatile enough to know if it's holiday season or not
- The customer will eventually operate in 12 different time zones, the function had to be market and time-zone aware
- Functions v2 must be used, so they can include it in their CI/CD pipeline
JSON file for the schedule
Here is a JSON file that I came up with that will meet my customer's requirements:
We have three keys: holidays
, partialDays
and regularHours
. On Holidays, the contact center is closed the entire day. On Partial Days, we have irregular hours, and we also include the Regular hours. The evaluation will be done from top to bottom, first we check for a holiday, then for a partial day, then finally the regular schedule.
The Function's Code
I created a Function v2 which will return the following response:
Tying the Studio Flow and Scheduling Function Together
How can you actually use this in a Studio flow or in Web Chat? Let's take a look at how all the components tie together. Here is a sample studio flow that utilizes our schedule:
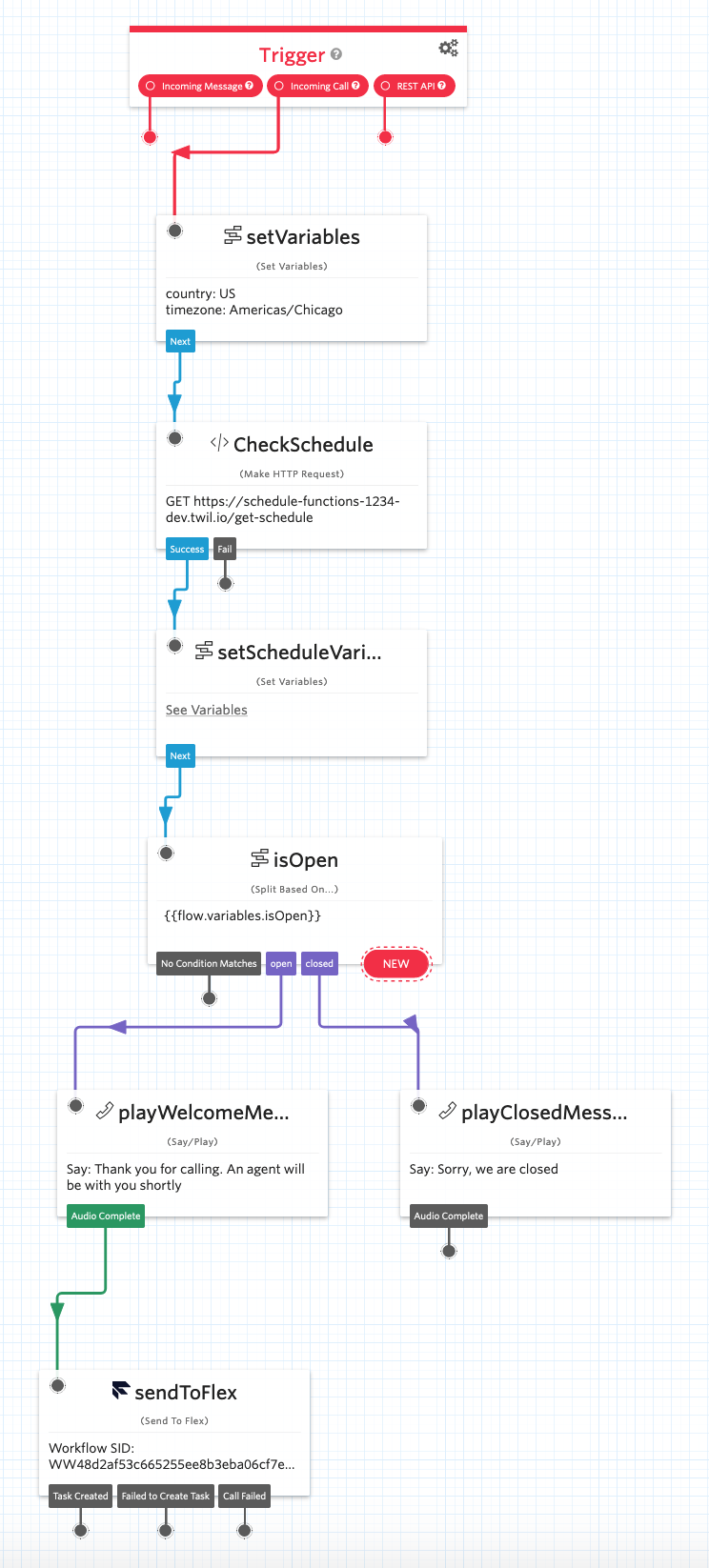
And here's the JSON for the Studio Flow above:
Taking the JSON File Further
The JSON file is a crucial component of this. It is critical that the formatting is correct, so one little mistake doesn't break the flow. To help ensure the integrity of the JSON, I wrote a schema file which can be used for validation:
That looks a little less scary. Here is a great tool I found for validating a JSON document against a schema: https://jsonschemalint.com/#!/version/draft-07/markup/json.
And that's it! With that, you now know how to set up advanced schedules for Studio. If you want to use this Function in a Web Chat, you simply make a fetch()
request before you render the Web Chat.
We can't wait to see what you build!
Want to try Studio? Sign up and get started here.
Lehel Gyeresi is a part of the Expert Services team and works as a Contact Center Solutions Consultant. You can reach him at lgyeresi [at] twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.