Authenticate Yii2 Users Using Twilio Verify
Time to read: 9 minutes
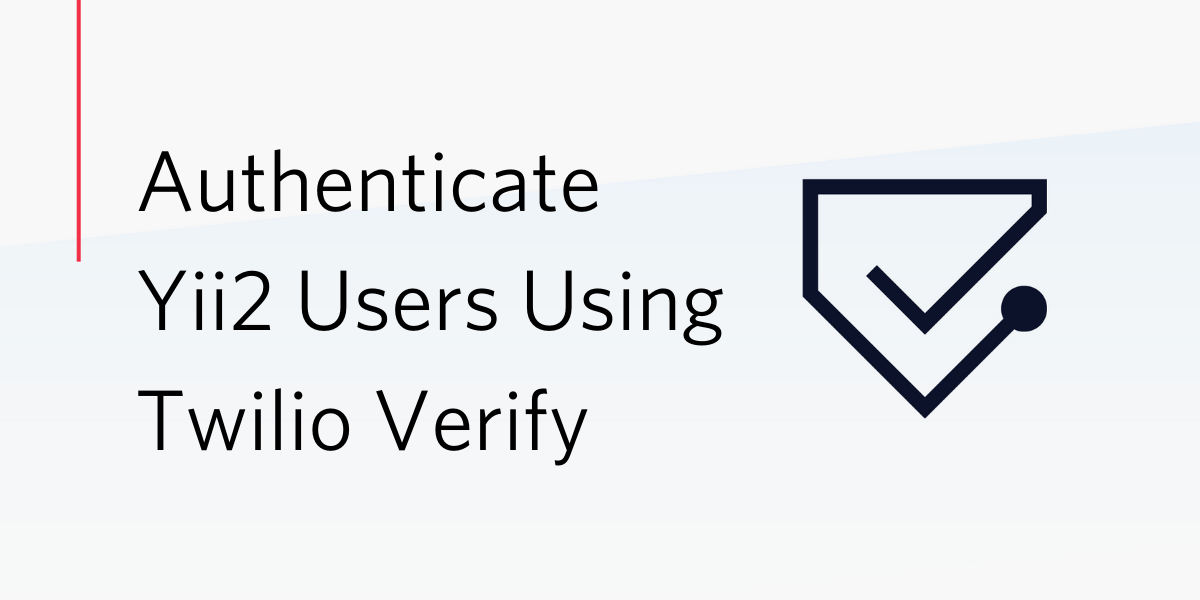
Registering users in your application is relatively easy enough. Ensuring that they provide valid phone numbers, however, can be a very difficult task.
Twilio's Verify API guarantees that your application’s database only contains users with verified phone numbers. So In this tutorial, I will show you how you can seamlessly capture and confirm user phone numbers during registration in a Yii2 application, by leveraging Twilio Verify.
Prerequisite
To get the most out of this tutorial, you will need the following:
- A basic understanding of PHP and version 2 of the Yii framework
- Composer globally installed on your computer
- A local MySQL database instance
- A Twilio account
Application Flow
During this tutorial, we will build a basic application using the Yii2 framework. It will support the ability to register users, and for users to login and view a simplistic user dashboard. During registration, a unique code will be sent to the user's phone, which they will need to use for verification purposes.
To avoid cluttering the main user table with details of users who are not willing to be verified or who enter an incorrect verification code, user details will be stored in a temporary user table. Once verified, user details will be moved to the main user table in the database.
This way, a clean-up can be performed on the database, deleting redundant user details from the temporary user table when necessary.
Creating the application
To begin, create a new Yii2 application, using Composer, by running the following command from the terminal.
This will create a Yii2 application using Yii's basic project template, sufficient enough for the tutorial, in the root of the folder where you ran the command from.
Note: other installation options are available which include creating an application with an Advance project template and building an application from scratch.
To verify that the application was created successfully, navigate into the yii-verify
folder and run the application, using the following commands.
Note: if you have Docker installed, you can launch the application by running docker-compose up -d
. If you do, it will be available on port 8000.
By default, the application will be served on localhost on port 8080. Open the site in your browser (http://localhost:8080) where you should see the welcome page, as in the screenshot below.
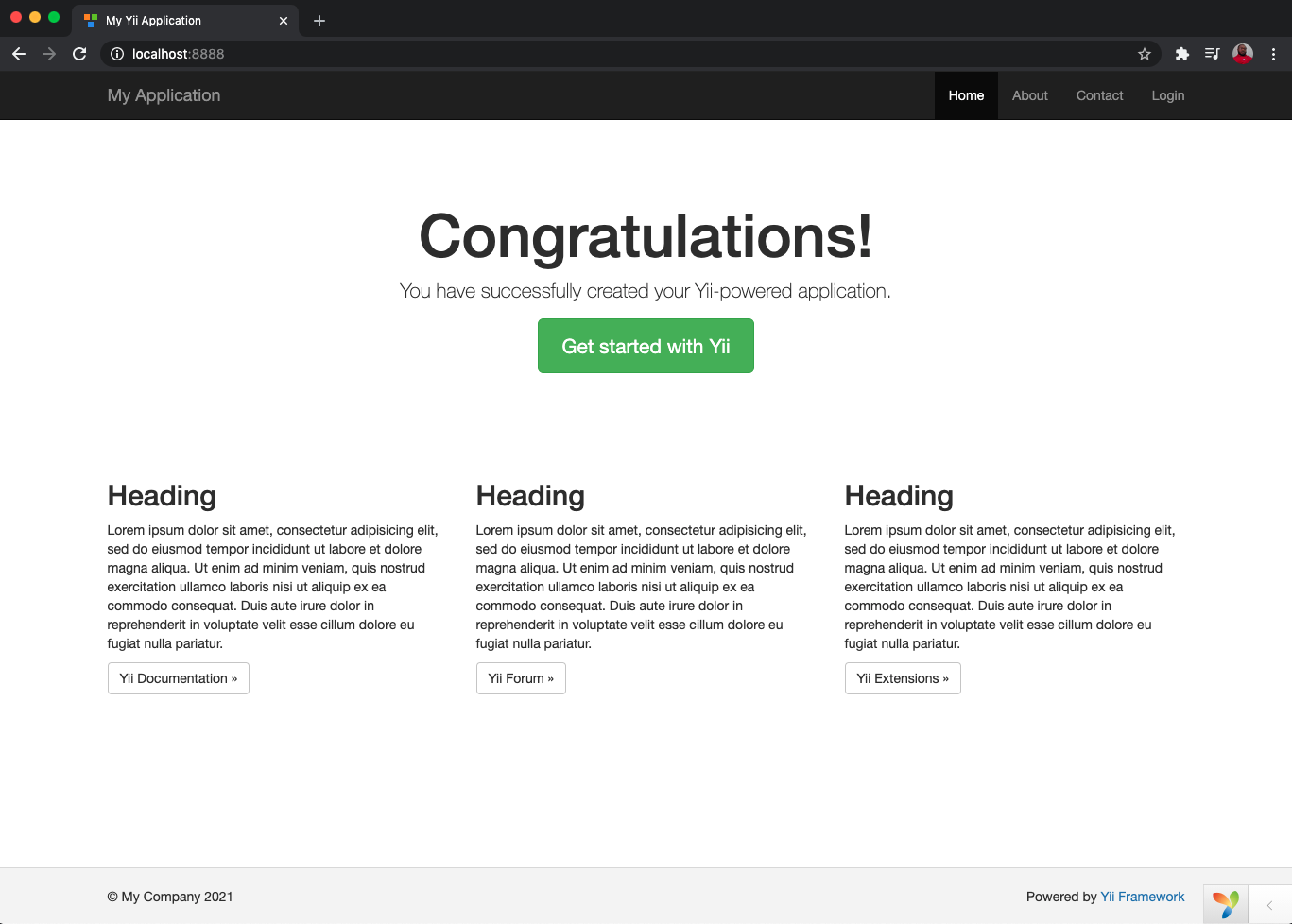
Configure the database connection
Next, you need to set up a connection to your local database instance using your preferred database management tool. Create a new database and name it as you wish. I named mine yii_verify
. Then, open config/db.php and update its content as shown below.
Create the database migration files
Next, create migrations for the two database tables that the application will use, user
and temporary_user
. Use the yii migrate/create
command to do so,by running the commands below, answering "yes" when prompted.
The commands above will create migration files within a new migrations directory. Migrations files are usually prefixed with the letter m
and the UTC date and time of when they were created, e.g., m210408_150000_create_user_table.php.
Edit the migration files
Next, modify the default content in the migration files generated above, starting with migrations/m<YYMMDD_HHMMSS>_create_user_table.php. Use the code below to replace the existing safeUp
function.
Then, update the safeUp()
function for migrations/m<YYMMDD_HHMMSS>_create_temporary_user_table.php to match the code below.
With the two migration files updated, run them to create the database tables, using the command below. Type yes
and press Enter
to run the migrations.
Create model classes
For proper manipulation of data and structured interaction with the tables in the database, we will create an Active Record class which can also be considered as a model for the temporary_user table.
By default, a Model has been created for the ser table during the installation of the application. Use the following command to create an Active Record class for the temporary_user table. When prompted, type "yes
" and press "Enter"
to continue.
The generated model class will be saved in the models directory, named TemporaryUser.php. Open it and replace the existing code with the code below.
Interestingly, the gii/model
command was able to discover the fields created in the temporary_user table and generated both the rules()
and attributeLabels
methods for the TemporaryUser
model. The change that we made was the findByUsername
method. This method retrieves the details of a particular user using the $username
parameter.
In the same vein, open models/User.php and replace the default content with the code below
Here, we made modifications by creating rules to specify the maximum string length for username
, password
, auth_key
and access_token
fields respectively. Also, we extended from the Active Record class, and created methods to retrieve the details of a particular user from the database.
Create the SignupForm Class
Similar to the models created in the preceding section, we will next create an Active Record based form also known as ActiveForm. This will be used to extract, represent, and validate the data entered by users.
At this stage of the tutorial, we only need a new form to represent the data requested from the user during the sign up stage. Create a new file, named SignupForm.php, within the models directory, and paste the code below into it.
Modify the SiteController to render the sign up Form
To render the signup form on the client side, we will pass an instance of the SignupForm
model using the ActiveForm widget to the view layer. Open controllers/SiteController.php and create an action to handle signups, by inserting the following code
Note: Don’t forget to import the SignupForm
use app\models\SignupForm
;
Next, uncomment and modify the urlManager
component in your application configuration, which is located in config/web.php. If it's not there, add the code below to the components
element of the $config
array.
This will enable a pretty URL format for all the routes within the application.
Create the view templates
Next, to render the signup form, create a new file, named signup.php, in the views/site folder. Then, open the newly created file and paste the following code into it.
If you run the app at this point, users will be able to sign up, by filling in and submitting the submit form (/signup
). After doing so, their details will be saved in the temporary user table. However, they won't, yet, be able to login.
In the next section, we will complete the signup process, by integrating SMS verification by using the Twilio Verify API. After doing so, during signup the user will receive a token to verify their authenticity which they will need to use.
Create a Twilio Service
To integrate the Twilio Verify API into the application, you need to have a Twilio Service.
Navigate to the Twilio Services dashboard and create a service, if you don't already have one. Give your service a friendly name, as you wish. I have named mine "yii-verify-service". Once you have created the service, copy the SERVICE SID
, which can be found on the Service's "General Settings" page. You can see an example below.
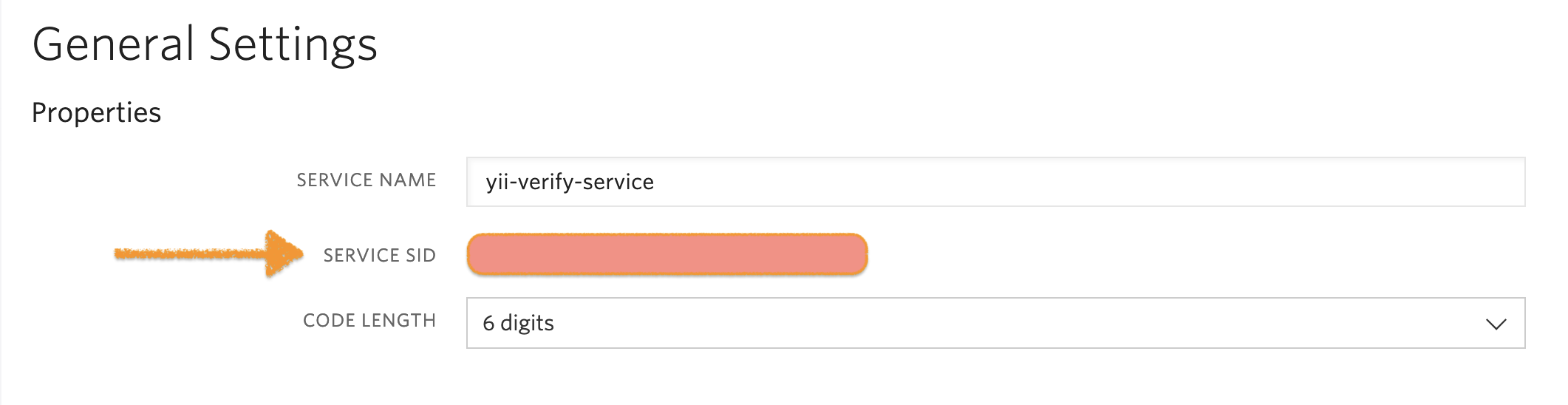
Ensure that you save the value of SERVICE SID
for the newly created service as it will be required later in the tutorial.
Note: While you're there, obtain your ACCOUNT SID and
AUTH TOKEN from the Twilio Console.
For the application and Twilio to communicate, you need to install Twilio PHP SDK. Run the command below to install it using Composer.
Once the installation is completed, create environment variables to hold the Twilio credentials. By default, Yii2 does not support the usage of a .env
file for storing environment variables.
So for this tutorial, we will improvise and define the required variables as constants. To do that, create a new file, named env.php, in the root directory of the application, and insert the following content into it.
Note: Please be sure to replace YOUR_TWILIO_ACCOUNT_SID
and YOUR_TWILIO_AUTH_TOKEN
with the appropriate credentials from the Twilio Console, and YOUR_TWILIO_SERVICE_SID
with the service id from the service which you created earlier.
Next, navigate to web/index.php and add the highlighted lines below to it to load env.php, which we have just created.
This is one of the simpler approaches to define, load, and make use of environment variables in Yii2 applications. You could also use a package such as phpdotenv.
Note: Don’t forget to exclude env.php from your version control system, for example, by adding it to .gitignore when using Git.
Now, we’ll create a service that allows us to handle SMS verification. At the root of the application, create a directory named service, then, inside that directory, create a file named TwilioSMSVerificationService.php. Open the file and paste the code below into it.
In the code above, we created the service with three private variables: the user’s phone number, the Twilio service ID, and a Client
object from the Twilio SDK, which are initialized within the class constructor. The third variable, Client
, will be used for making API requests to the Verify API.
Next we created two new functions:
sendVerificationToken
: This will be used to send a verification token to a phone number.isValidToken
: This will be used to verify the token provided by the user, by making a verification check to the Twilio Verify API and returning a boolean corresponding to whether the token is valid or not.
Verify the SMS token using TwilioSMSVerificationService
Here, we will start by creating a form, named verifyUserFormBegin
, to enable a user to enter and verify the token sent to their phone number. Before creating the form, we will modify models/SignupForm.php to utilize TwilioSMSVerificationService
, and to store the current user’s details in the active session.
Open models/SignupForm.php and replace its content with the code below
The difference in this version of the class is that we instantiated the TwilioSMSVerificationService
with the formatted phone number of the user, which included the country code as the parameter.
Next, we created a new method, named storeDataInSession()
, to store the user data in the session. This makes it easier to track and monitor for verification purposes.
With those changes made, in the models folder, create a new file named VerifyUserForm.php. Open this file and paste the code below into it.
In the verifyUser()
method, we get the token provided by the user and call TwilioSMSVerificationService
to validate it. If the token is valid, we will retrieve the user’s details from the session and persist them in the user table.
Now, create an action in controllers/SiteController.php, named actionVerifyUser
, to utilize the newly created form. Open controllers/SiteController.php and paste the code below into it.
Note: Don’t forget to import the VerifyUserForm
use app\models\VerifyUserForm
;
To render the form, navigate to the views/site
folder and create a file named verifyUser.php
and paste the following code in it:
Redirect the user to the verify form
As created earlier, the actionSignup()
method in the SiteController
redirects the user to the login page. Modify the method to redirect to the verified user’s page instead, as shown in the snippet below, so that they can verify the SMS token that they will receive after submitting their details.
Create a protected dashboard view
This application will allow a verified and authenticated user to view the dashboard which, in real-life applications, would contain resources retrieved from the database for such users.
In controllers/SiteController.php add the code below, to render the dashboard view.
Now, create the view file, named dashboard.php, in the views/site folder and paste the following content in it:
Modify the main application layout view
The views/layouts/main.php file houses the structure on which the whole application was built and also contains the links in the navigation bar. Open this file and modify its content as shown below
Lastly, modify the login view to display the flash message added to the session whenever a user is verified. Open views/site/login.php and replace its content with the code below.
See it working
You've now created the application, so it's time to test it. Start by running the command below to launch the application.
Note: if you tested the signup process in the first version of the application, make sure to truncate the temporary_user table.
Then, navigate to the default URL at http://localhost:8080/ to view it. Click on Signup and provide the appropriate details.
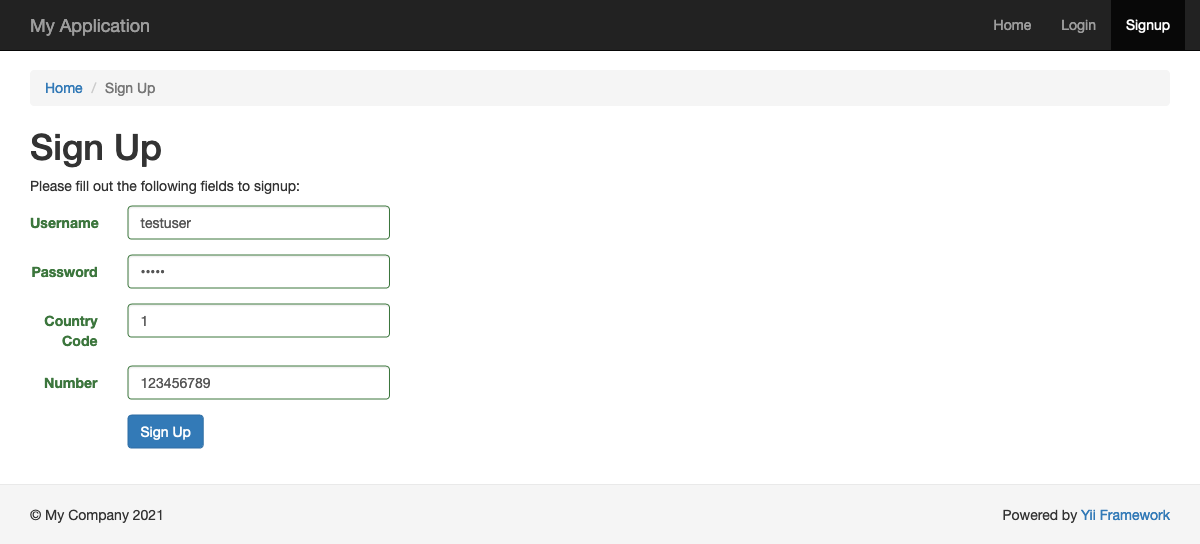
Then, provide the token sent to your phone number via SMS for verification in the next form.
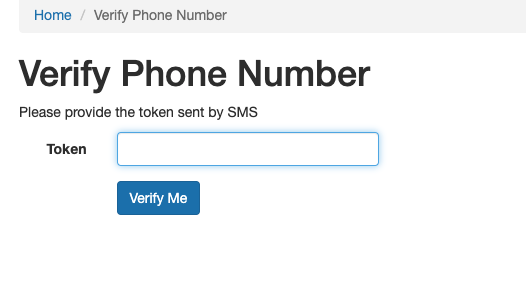
Once verified, you will be redirected to the login page with a flash message indicating that the phone number has been successfully verified.
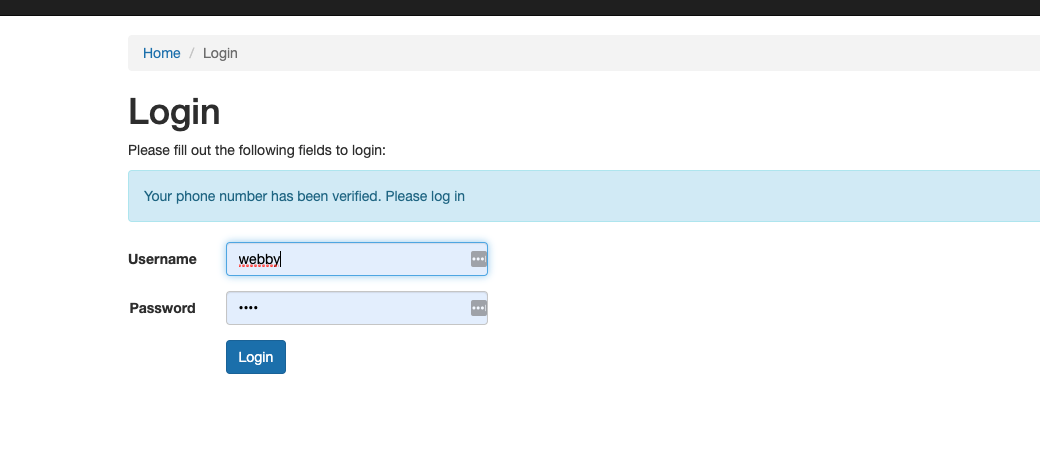
Conclusion
In this tutorial, you learned how to verify the phone number of, potential, new users of our Yii2-based application, by utilizing Twilio Verify API as an added layer of security. We built this application to ensure that only verified users with valid phone numbers can have their details moved to the main user table in our application database.
You can improve the application that we have built here, by adding more features as you deem fit. Find the complete source code on GitHub.
Oluyemi is a tech enthusiast with a background in Telecommunication Engineering. With a keen interest to solve day-to-day problems encountered by users, he ventured into programming and has since directed his problem-solving skills at building software for both web and mobile.
A full-stack software engineer with a passion for sharing knowledge, Oluyemi has published a good number of technical articles and content on several blogs on the internet. Being tech-savvy, his hobbies include trying out new programming languages and frameworks.
- Twitter: https://twitter.com/yemiwebby
- GitHub: https://github.com/yemiwebby
- Website: https://yemiwebby.com.ng/
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.