How to Make a Mysterious Phone Call with Twilio Voice and Java
Time to read: 5 minutes
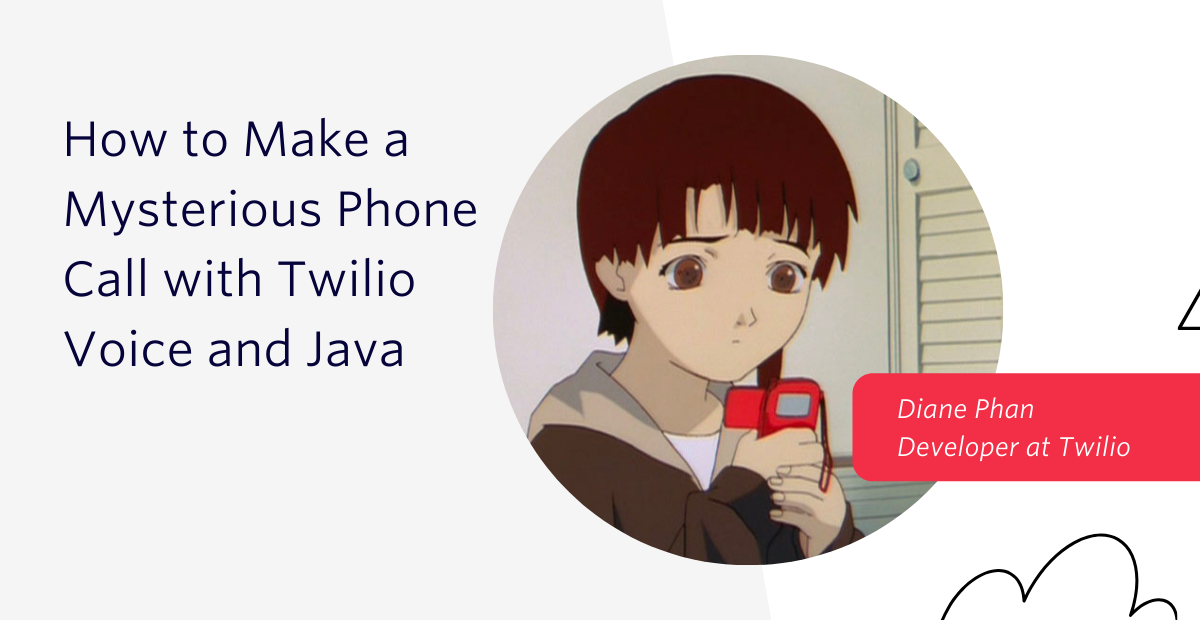
I'm sure all of us have made prank calls at some point in our lives or wanted to use phones to create a tricky puzzle.
With the help of Twilio and Java, you can surprise a friend with a mysterious phone call that plays your chosen sound file, without having to pick up your mobile device.
In this article, you'll write a couple of lines of Java code to make an outbound call that plays a chosen sound file to any phone number, right from the command line.
Tutorial requirements
- A free or paid Twilio account. If you are new to Twilio get your free account now! (If you sign up through this link, Twilio will give you $10 credit when you upgrade.)
- Some prior knowledge of Java or a willingness to learn.
- A smartphone with active service, to test the project
Configuration
Start off by creating a directory to store the files of the project. Using your favorite terminal, enter:
Next, you need to download the standalone Twilio Java helper library. The latest version of the jar files at the time of writing is twilio-8.9.0-with-dependencies.jar. Scroll down to find the directory named after the latest release version. Click on the directory to find and download the file and save it to your java_voice project folder.
Buy a Twilio phone number
If you haven't done so already, purchase a Twilio number to send the SMS. Log in to the Twilio Console, select Phone Numbers, and then click on the red plus sign to buy a Twilio number. Note that if you are using a free account you will be using your trial credit for this purchase.
In the Buy a Number screen you can select your country and check "Voice" in the capabilities field. Alternatively, you can check all the options in the Capabilities field to use the other features in the future. If you’d like to request a number from your region, you can enter your area code in the Number field.
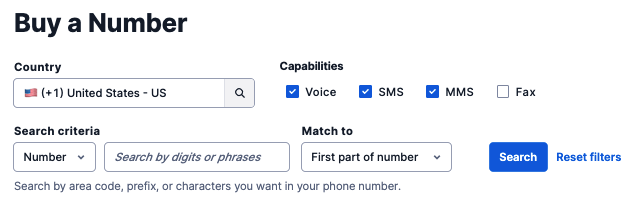
Click the Search button to see what numbers are available, and then click Buy for the number that you like from the results. After you confirm your purchase, click the Close button.
Create a publicly hosted MP3 file
In order to play your own audio file over the outbound call, the audio file must be exposed publicly to the web, so make sure that you are comfortable making the audio file public before continuing on with this project.
Navigate to the Assets dashboard on the Twilio Console. Click on the blue Create Service button. A small pop up window will show up prompting you to give the service a name such as "mysteriousaudio" as seen in the image below:
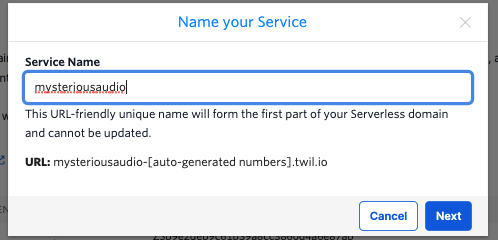
Click on the blue Next button to be shown the Twilio Assets dashboard. Then, click on the blue Add + button, near the top left-hand corner, and select Upload File as seen here:
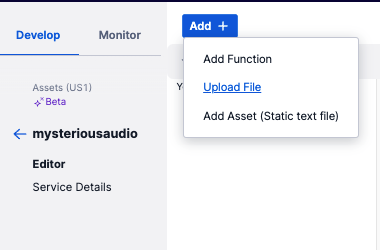
Locate and upload the MP3 file to be played during the outbound call. Click the blue Upload button. The name of the audio file will be the name of the endpoint when deployed onto a public URL.
After the upload is complete, click the Deploy All button near the bottom left-hand corner. Notice how there is a URL with the service name "mysteriousaudio" along with some randomly generated numbers to a twil.io
link above that button (mysteriousaudio-4190.twil.io
in the example below). This URL will deploy and host the uploaded MP3 file.
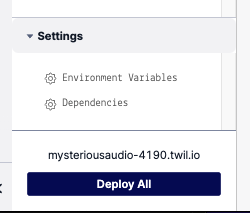
Once deployed, look for the Assets section on the dashboard. As mentioned above, the endpoint is named after the uploaded audio file.
Click on the three vertical dots on the assets endpoint and select Copy URL. Make sure that the endpoint asset is set to "Public' as well.
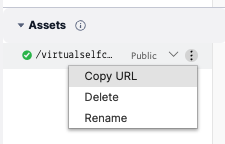
The URL will be used in the coding portion, however, you can enter this URL in a web browser to listen to your audio on the web.
The audio I used in this article is from Porter Robinson's side project Virtual Self's mysterious audio that was played when they dialed a phone number back in 2017. The lovely melody and transcription are very cryptic, but left fans excited for possibilities that may come next for this artist's music.
This audio file was recorded by SoundCloud user void and converted into an MP3 file for the project. Perhaps you can surprise someone with this phone call that will leave them feeling nostalgic, pleasantly amazed, or straight up confused.
Configure the environment variables
It is best practice to protect your Twilio account credentials by setting environment variables.
You can find your account credentials on the Twilio Console.
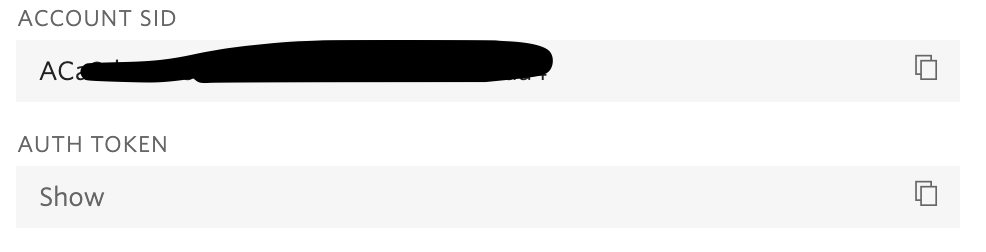
If you are using macOS or Linux, run the commands below to export your account credentials into a twilio.env file. Then, you will create a .gitignore file so that you do not accidentally commit your credentials to a public repository.
For Windows developers, you would have to replace export
with set
, as seen below:
Make an outbound phone call with Java
Create a file named MysteriousCaller.java in your project directory and paste the following code into it:
Let's break down the code real quick.
The MysteriousCaller
class defines a void main
function where the Twilio client is initialized using the ACCOUNT_SID
and AUTH_TOKEN
variables set above. Replace <YOUR_PHONE_NUMBER>
and <YOUR_TWILIO_NUMBER>
with your actual numbers, using the E.164 format.
It creates a new call
instance of the Call
resource as provided by the Twilio REST API, in order to make an outbound call to your personal phone number from your Twilio phone number.
After the call is made, the call SID is printed on your terminal for your reference.
Save your file and compile the MysteriousCaller
class by typing this command in your terminal:
If you are using macOS or Linux, run the successfully built Java class with the following command:
For Windows developers, use the following command to send the SMS from your terminal. Notice that the only difference is that the Windows command uses a semicolon instead:
In just a moment, you will hear your personal phone ring! Pick it up to hear the audio file you uploaded.
What's next for making outbound calls with Java?
Congratulations on writing a short Java file and using the command line to make a quick outbound call to a phone number! This was so wickedly quick, can you even believe it was possible?
There's so much more you can do with Twilio and code without even picking up your phone. Perhaps you can figure out how to handle incoming calls with Java and Twilio or surprise someone with a ghost writing bot written in Java instead. You can even send MMS from your command line wickedly fast to a provided phone number.
What audio files are you using in your phone calls? Let me know by reaching out to me over email!
Diane Phan is a software developer on the Developer Voices team. She loves to help beginner programmers get started on creative projects that involve fun pop culture references. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.