How to Send a Message on WhatsApp With Java
Time to read: 3 minutes
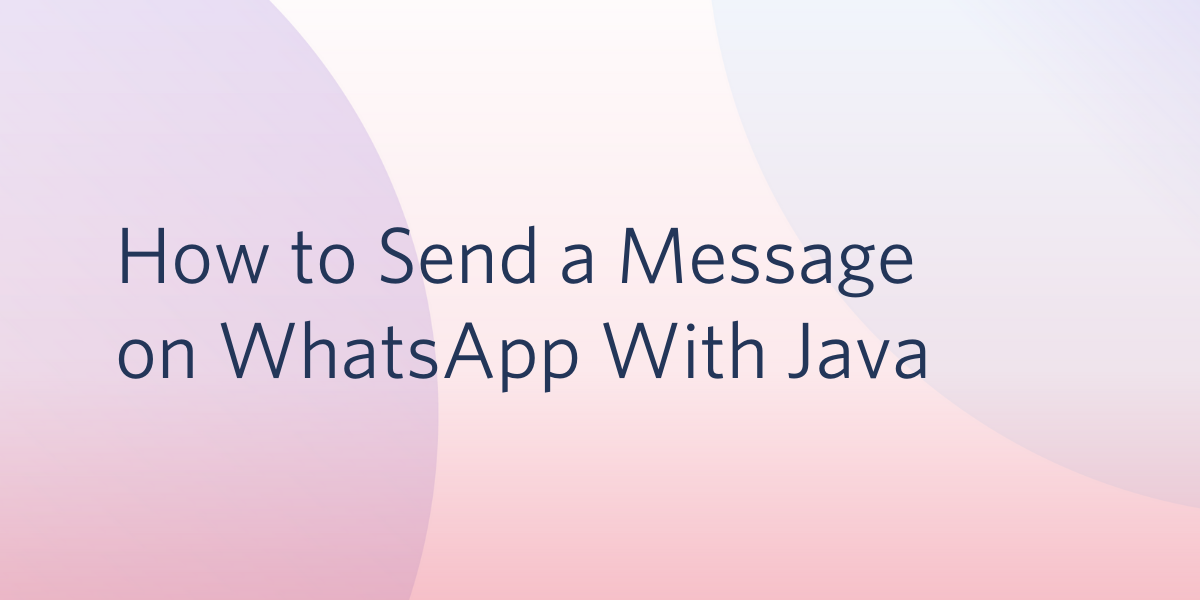
The WhatsApp Business API from Twilio is a powerful, yet easy to use service that allows you to communicate with your users on the popular messaging app.
With the help of Twilio and Java, you can deliver a quick message to someone over WhatsApp without having to pick up your mobile device.
In this article, you'll be using your handy dandy command line and writing a couple of lines of Java code to send a WhatsApp message in an insanely fast manner. So why wait? Let's get started!
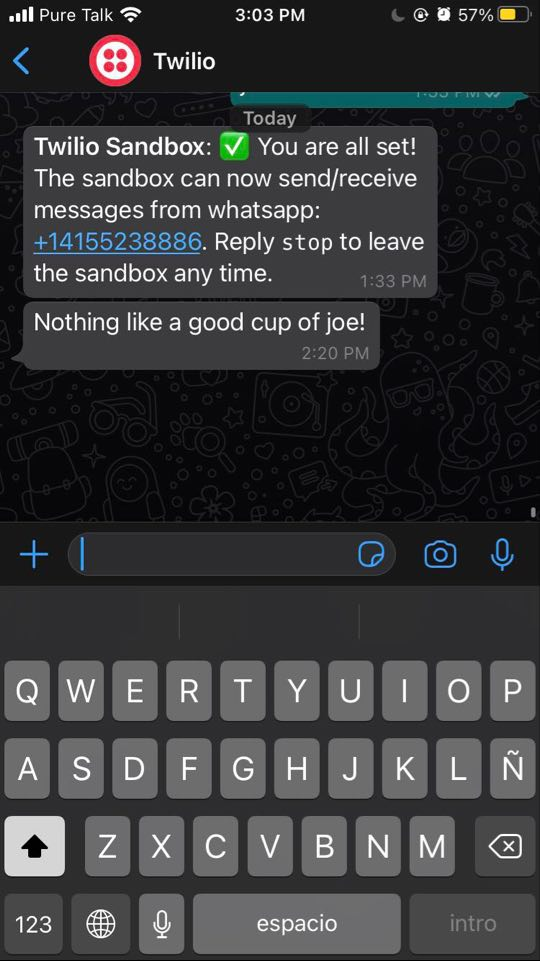
Tutorial requirements
- A free or paid Twilio account. If you are new to Twilio get your free account now! (If you sign up through this link, Twilio will give you $10 credit when you upgrade.)
- Some prior knowledge of Java or a willingness to learn.
- A smartphone with WhatsApp, to test the project.
The Twilio WhatsApp sandbox
Twilio provides a WhatsApp sandbox, where you can easily develop and test your application. Once your application is complete you can request production access for your Twilio phone number, which requires approval by WhatsApp.
In this section you are going to connect your smartphone to the sandbox. From your Twilio Console, select Messaging, then select Try it Out on the sidebar. Open the WhatsApp section. The WhatsApp sandbox page will show you the sandbox number assigned to your account, and a join code.
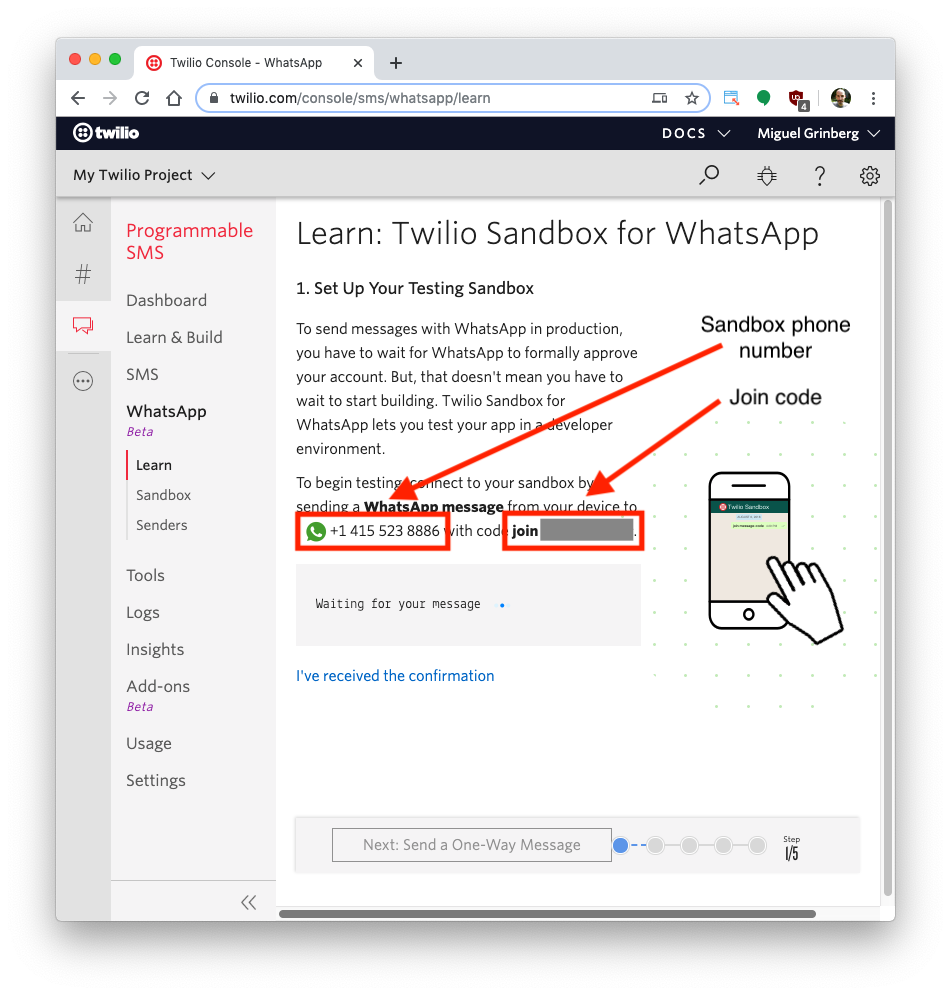
To enable the WhatsApp sandbox for your smartphone send a WhatsApp message with the given code to the number assigned to your account. The code is going to begin with the word "join", followed by a randomly generated two-word phrase.
Shortly after you send the message you should receive a reply from Twilio indicating that your mobile number is connected to the sandbox and can start sending and receiving messages.
If you intend to test your application with additional smartphones, then you must repeat the sandbox registration process with each of them.
Configuration
We’ll start off by creating a directory to store the files of our project. Inside your favorite terminal, enter:
In this article, we will be downloading the standalone Twilio Java helper library. Download a pre-built jar file here. The latest version of the jar files during the time this article was published is twilio-8.9.0-with-dependencies.jar. Scroll down to download the file and save it to your java_whatsapp project directory.
Authenticate against Twilio services
We need to safely store some important credentials that will be used to authenticate against the Twilio service.
You can obtain the TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
credentials that apply to your Twilio account from the Twilio Console:
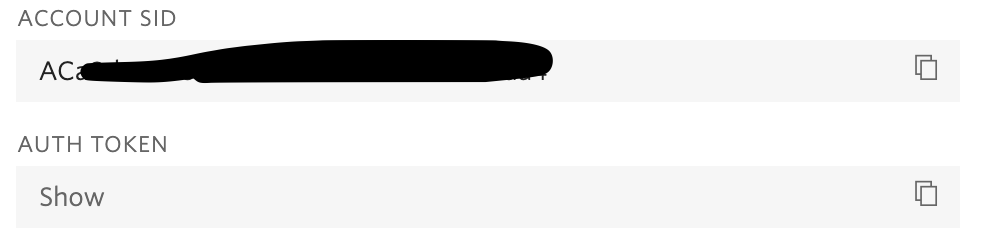
Once you have located the Account SID and Auth Token, we’ll be setting environment variables by typing the following commands into your terminal:
If you are following this tutorial on a Windows computer, replace export
with set
in the commands above.
Send a WhatsApp message with Twilio
Create a file named SendWhatsApp.java in your project directory and paste the following code:
The SendWhatsApp
class defines a void main
function where the Twilio client is initialized using the ACCOUNT_SID
and AUTH_TOKEN
variables set above. Replace <YOUR_WHATSAPP_PHONE_NUMBER>
with your actual number, using the E.164 format.
We create a new message
instance of the Message
resource as provided by the Twilio REST API in order to send a WhatsApp message to your personal phone number from your Twilio phone number. Make sure to replace this placeholder with the appropriate number.
After the message is sent, the message SID is printed on your terminal for your reference.
Save your file and compile the SendWhatsApp
class by typing this command in your terminal:
If you are using macOS or Linux, run the successfully built Java class with the following command:
For Windows developers, use the following command to send the SMS from your terminal. Notice that the only difference is that the Windows command uses a semicolon as a separator in the class path:
In just a moment, you will see a ping from your personal WhatsApp enabled phone with the message sent directly from your terminal!
What's next for sending WhatsApp messages with Java?
Congratulations on writing a short Java file and using the command line to send a quick WhatsApp message to your phone! This was so wickedly quick, can you even believe it was possible?
There's so much more you can do with Twilio and code without even picking up your phone. Perhaps you can figure out how to reply to inbound SMS in Java as well.
Explore some other neat functions you can play around with in Java or test out Twilio's very own CLI:
- How to send an MMS with Java
- How to send an SMS with Java
- How to make a voice call with Java and Twilio
Let me know about the projects you're building with Java and Twilio WhatsApp API by reaching out to me over email!
Diane Phan is a Developer Network editor on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.