How to Send an SMS with Java
Time to read:
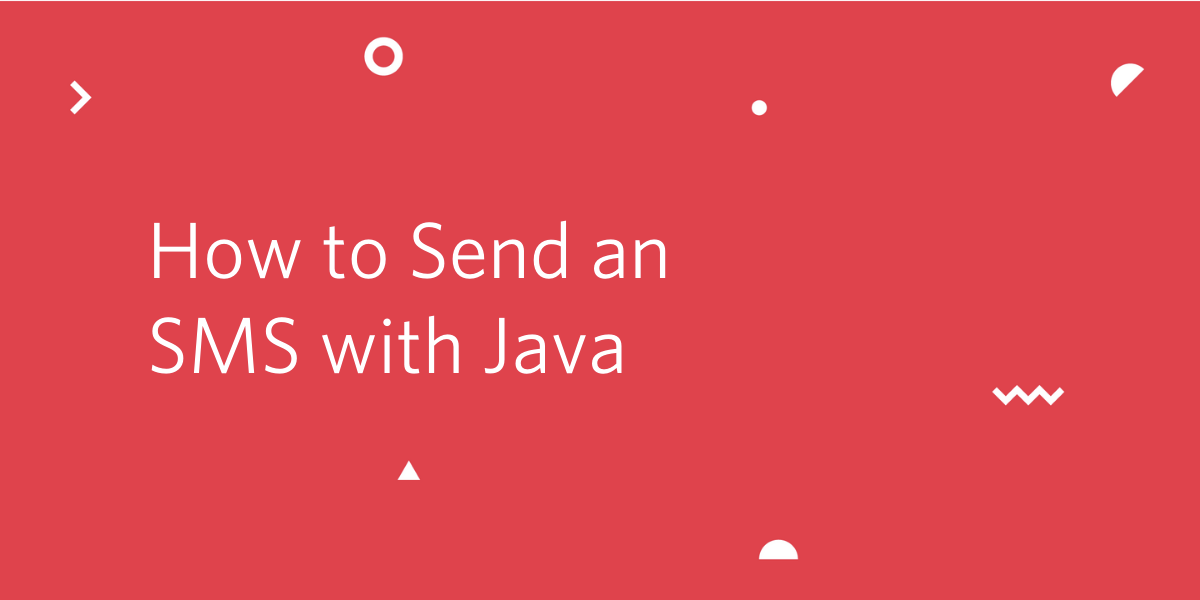
Twilio is all about powering communication and doing it conveniently and fast in any language.
With the help of Twilio and Java, you can deliver a quick message to someone without having to pick up your mobile device.
In this article, you'll be using your handy dandy command line and writing a couple of lines of Java code to send an SMS in an insanely fast manner. So why wait? Let's get started!
Tutorial requirements
- A free or paid Twilio account. If you are new to Twilio get your free account now! (If you sign up through this link, Twilio will give you $10 credit when you upgrade.)
- Some prior knowledge of Java or a willingness to learn.
- A smartphone with active service, to test the project
Configuration
We’ll start off by creating a directory to store the files of our project. Inside your favorite terminal, enter:
In this article, we will be downloading the standalone Twilio Java helper library. Download a pre-built jar file here. The latest version of the jar files during the time this article was published is twilio-8.8.0-with-dependencies.jar. Scroll down to download the file and save it to your java_sms project directory.
Buy a Twilio phone number
If you haven't done so already, purchase a Twilio number to send the SMS.
Log in to the Twilio Console, select Phone Numbers, and then click on the red plus sign to buy a Twilio number. Note that if you are using a free account you will be using your trial credit for this purchase.
In the Buy a Number screen you can select your country and check SMS in the capabilities field. If you’d like to request a number from your region, you can enter your area code in the Number field.
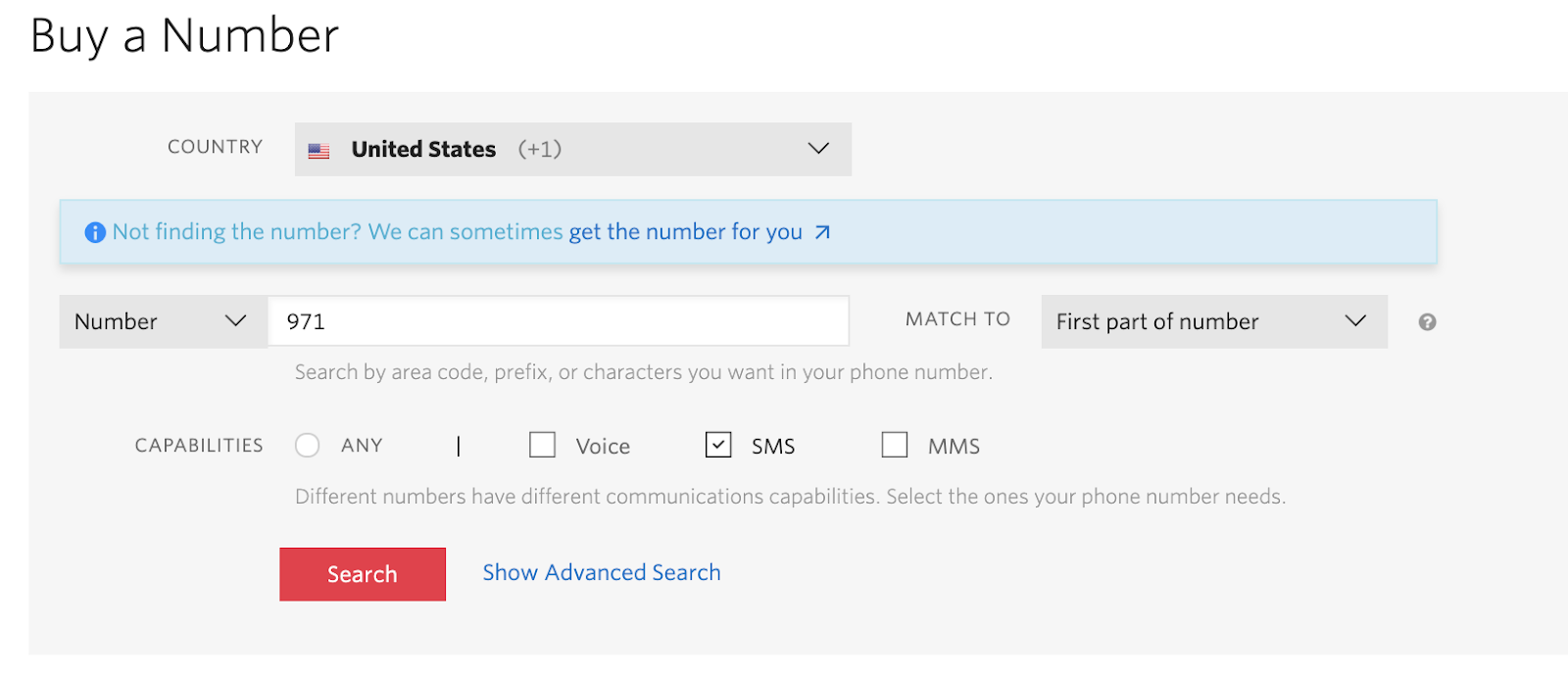
Click the Search button to see what numbers are available, and then click “Buy” for the number that you like from the results. After you confirm your purchase, click the Close button.
Send an SMS with Java
Create a file named SmsSender.java in your project directory and paste the following code:
Let's break down the code real quick.
It is best practice to protect your Twilio account credentials by setting environment variables.
You can find your account credentials on the Twilio Console.
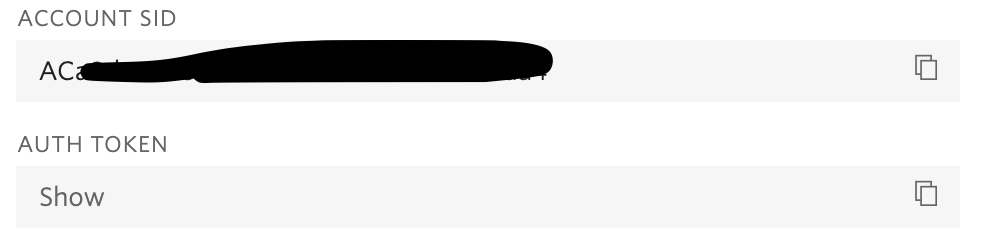
If you are using macOS or Linux, run the commands below to export your account credentials into a twilio.env file. Then, you will create a .gitignore file so that you do not accidentally commit your credentials to a public repository.
For Windows developers, you would have to replace export
with set
, as seen below:
Feel free to create a .env and .gitignore file as well for your convenience.
The SendSMS
class defines a void main
function where the Twilio client is initialized using the ACCOUNT_SID
and AUTH_TOKEN
variables set above. Replace <YOUR_PHONE_NUMBER>
and <YOUR_TWILIO_NUMBER>
with your actual numbers, using the E.164 format.
We create a new message
instance of the Message
resource as provided by the Twilio REST API in order to send an SMS to your personal phone number from your Twilio phone number. Make sure to replace those placeholders with the appropriate values.
After the message is sent, the message SID is printed on your terminal for your reference.
Save your file and compile the SendSMS
class by typing this command in your terminal:
If you are using macOS or Linux, run the successfully built Java class with the following command:
For Windows developers, use the following command to send the SMS from your terminal. Notice that the only difference is that the Windows command uses a semicolon instead:
In just a moment, you will see a ping from your personal phone with the SMS sent directly from your terminal!
What's next for sending SMS with Java?
Congratulations on writing a short Java file and using the command line to send a quick SMS to your phone! This was so wickedly quick, can you even believe it was possible?
There's so much more you can do with Twilio and code without even picking up your phone. Perhaps you can figure out how to reply to inbound SMS in Java as well.
Explore some other neat functions you can play around with in Java or test out Twilio's very own CLI:
Let me know about the projects you're building with Java and Twilio SMS by reaching out to me over email!
Diane Phan is a Developer Network editor on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.