Build a cross-platform desktop application with Go and Wails
Time to read: 11 minutes
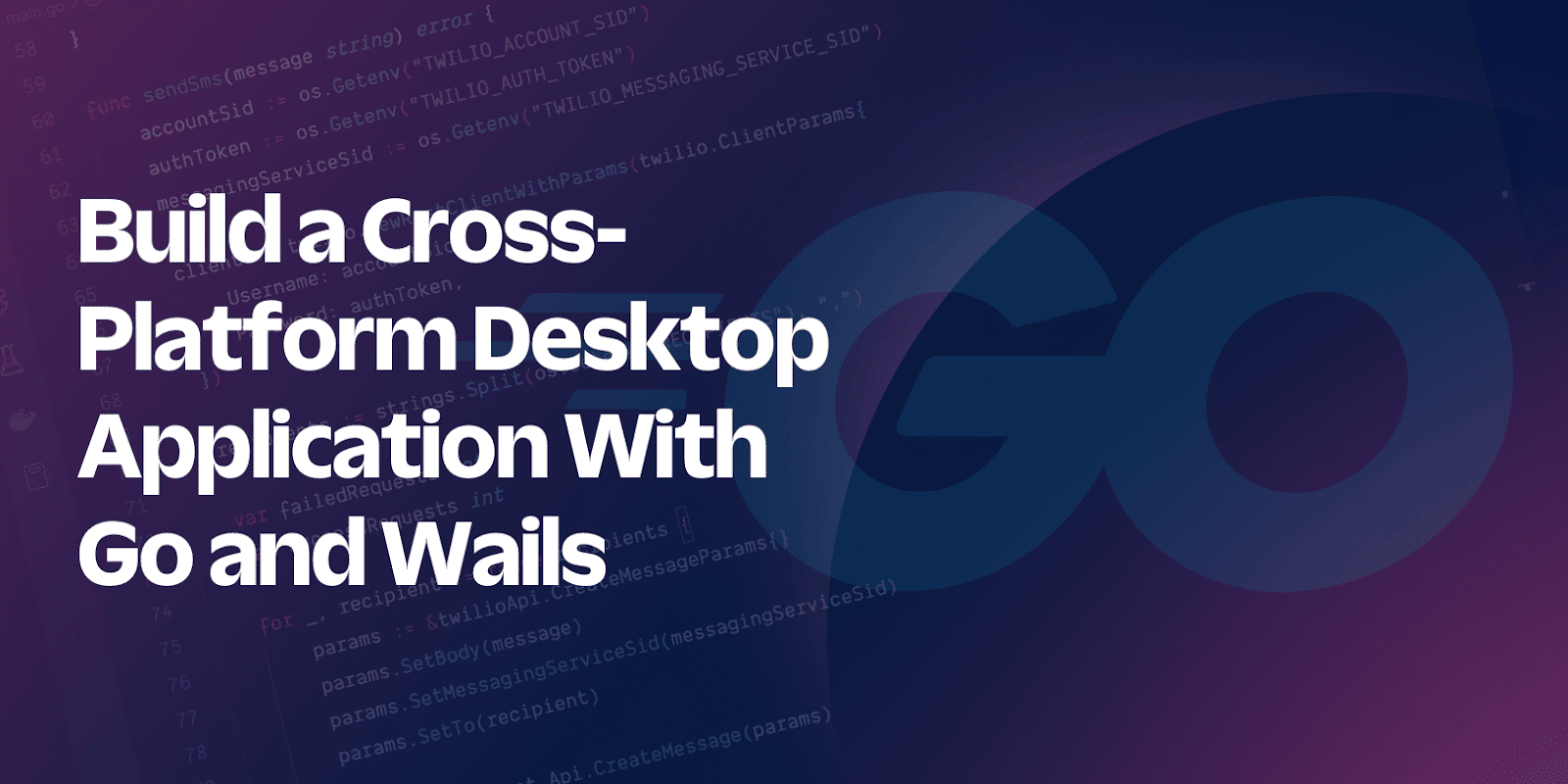
Electron was (and still is) a big hit for a number of reasons. First, its cross-platform functionality enables developers to support Linux, Windows, and macOS from a single codebase. On top of that, it has a lean learning curve for developers familiar with Javascript.
While it has its downsides — with application size and memory consumption the most prominent — it has opened up a wealth of possibilities for creating cross-platform desktop apps.
However, since its release, a number of alternatives have come into the fray. This article explores one such alternative - Wails, a project which makes it possible to write desktop apps using Go and web technologies such as React and Vue. A major selling point for Wails is that it does not embed a browser, but instead uses the native rendering engine for the platform. This makes it a lightweight alternative to Electron.
To get familiar with Wails, you will build a GitHub desktop client which will interact with the GitHub API, providing the following features:
- View public repositories and Gists
- View private repositories and Gists for an authenticated user
- Create a new Gist for the authenticated user.
While the backend will be written in Go, React and Vite will be used for the frontend. The UI components will be created using Ant Design (AntD).
How it works
As mentioned earlier, Wails works by combining a backend written in Go with a frontend written using either a Javascript library/framework, or with Vanilla HTML and Javascript. Even though your functions and data types are declared on the backend, Wails makes it possible for them to be called on the frontend. What’s more, where a struct is declared on the backend, Wails is able to generate a TypeScript model for use on the frontend. The result of this is seamless communication between the frontend and backend. You can read more about how Wails works here.
Prerequisites
To follow this tutorial, you will need the following:
Getting started
Create a new Wails project by running the following command.
This scaffolds a new project using Go for the backend and React + Vite for the frontend. Once the scaffolding process is completed, navigate into the newly created folder and run the project, by running the commands below.
This will run the application as seen in the image below.
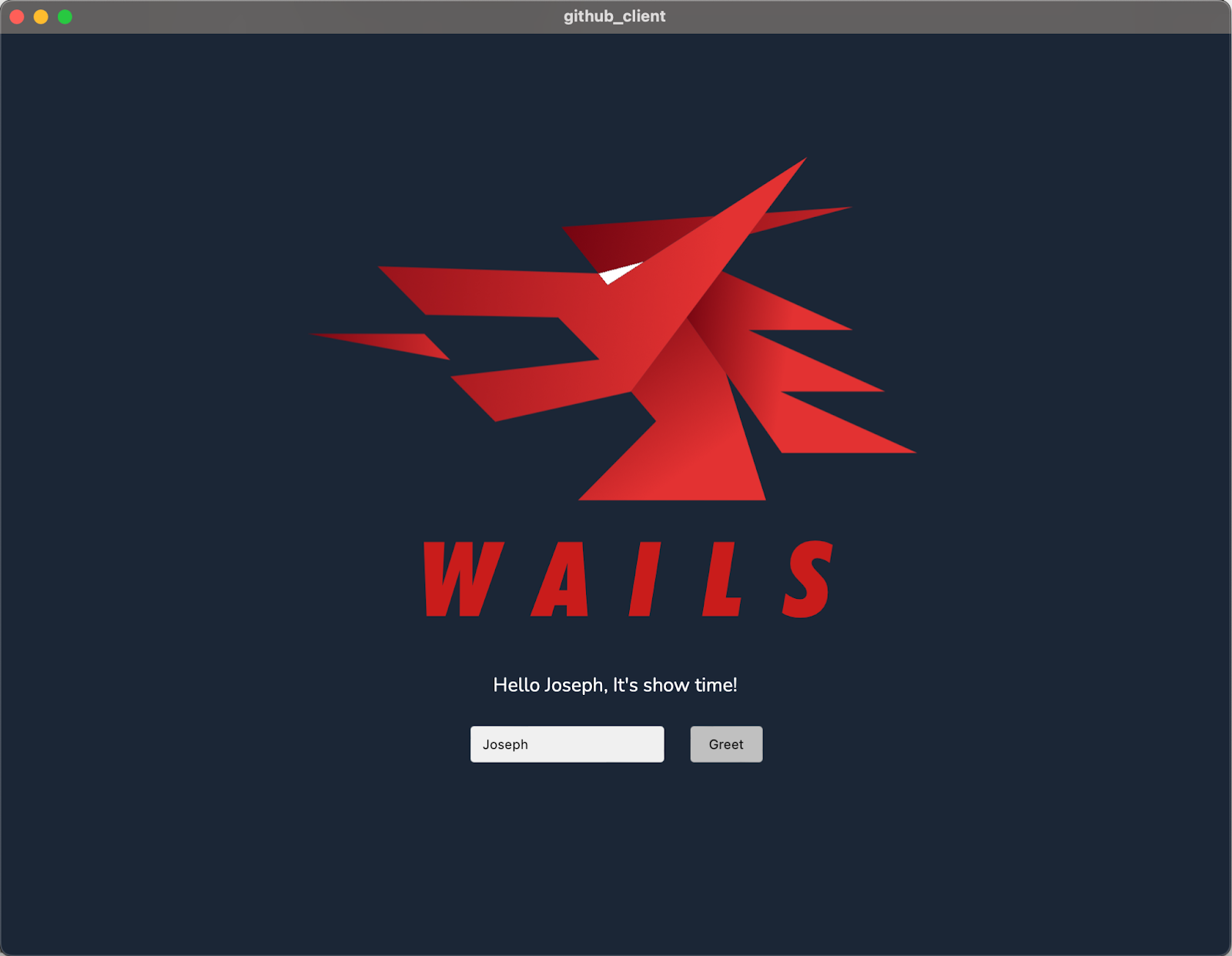
Close the application and open the project directory in your preferred editor or IDE, to get started with adding features to the application.
Build the backend
Add functionality for API requests
The first thing the app needs to have is the ability to send GET and POST requests to the GitHub API. In the root directory of the application, create a new file named api.go. In this file, add the following code.
The makeRequest()
function is used internally to make a request to a specified URL. In addition to specifying the URL, the request type, token, and payload are passed to the function. Using these, the request is prepared and sent with the API response returned by the function.
The MakeGetRequest()
and MakePostRequest()
functions wrap around the makeRequest()
function to send GET and POST requests respectively.
Bind helper functions to the app
With the API functionality in place, you can declare some helper functions which will be bound to the frontend. This is done by adding receiver functions for the App
struct.
You can see an example of this at the end of app.go, where a receiver function named Greet()
is declared.
Now, add the following code to app.go.
Then, add "encoding/json" to the imports list at the top of the file, if your text editor or IDE doesn't do it for you automatically.
In addition to the existing code, it declares two new types: APIResponse
and Gist
. These will be used to model a response from the API and the structure of a Gist respectively. Next, it declares the receiver functions for the App
struct:
- The
GetPublicRepositories()
function retrieves a list of public repositories from the GitHub API via a GET request. Since this route does not require authentication, an empty string is passed as the token. - The
GetPublicGists()
function retrieves a list of public Gists from the GitHub API via a GET request. Authentication is also not required, hence an empty string is passed as the token. - The
GetRepositoriesForAuthenticatedUser()
function is used to get a list of the authenticated user’s private repositories. This function takes the token as a parameter. - The
GetGistsForAuthenticatedUser()
function is used to retrieve the authenticated user’s Gists. This function also takes a token as a parameter. - The
GetMoreInformationFromURL()
function is used to get more information on a repository. This information could be the commit history, list of contributors, or list of users who have starred the repository. It takes two parameters, the url to be called and the authentication token. For public repositories, the token will be an empty string. - The
GetGistContent()
function is used to get the content of a Gist. This function takes the URL for the Gist’s raw content and an authentication token (an empty string for public Gists). It returns a string corresponding to the content of the Gist. - The
CreateNewGist()
function is used to create a new Gist for the authenticated user. This function takes two parameters, the Gist to be created as well as the authentication token for the user.
Build the frontend
All the code for the frontend is stored in the frontend folder. But before writing any code, add the JavaScript dependencies using the following commands.
The dependencies are as follows:
- Ant Design - This helps designers/developers to build beautiful and flexible products with ease
- Ant-design icons - This gives you access to AntD’s SVG icon collection
- React-router - This will be used to implement client-side routing
- Prismjs - This will be used to implement syntax highlighting for the Gists
Next, create a folder in the frontend/src folder named components.
Add authentication
For authentication, the user will be required to provide a GitHub Personal Access Token. The token is included in the header of requests to endpoints requiring authentication. Create one if you don’t have one — however, you must set the following permissions for your token to be useful for this project.
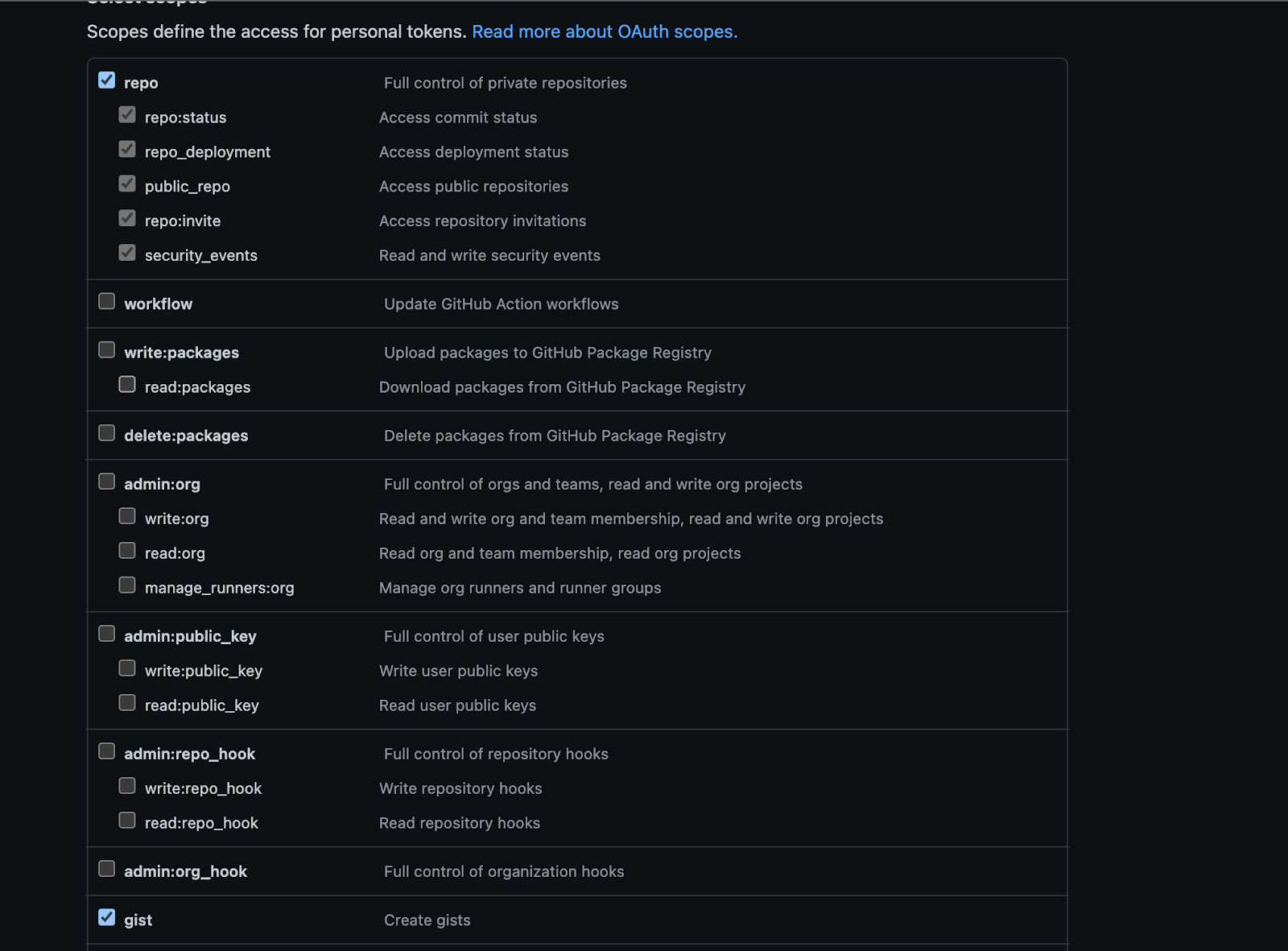
For this project, the React Context API will be used to store the token for an hour after which the user will have to re-authenticate by providing the token again.
In the frontend/src/components folder, create a new folder named context. In that folder, create a new file named AuthModal.jsx and add the following code to it.
This component renders the authentication form. The form has a single field for the user to paste and save a token. The shouldShowModal
prop is used to conditionally render the form while the onSubmit
and onCancel
props are used to respond to the user’s action.
Next, in the context folder again, create a new file named AuthContext.jsx and add the following code to it.
There are two exports
in this file. The first is the useAuthContext
hook. This hook will be used to retrieve the token saved in Context
. The second is the AuthContextProvider
component. This component is responsible for rendering the authentication form (either on page load or when the token has “expired” after 1 hour).
It also renders an error page if the user clicks “Cancel” on the authentication form. This component takes a JSX element (named children
) as a prop and wraps it with a context provider — thus giving the child element access to the value of the token.
Add the Master-Detail layout
For displaying repositories and Gists, the master-detail layout will be used. A list of items will be rendered and clicking on one item will display more information on the selected item beside the list.
In the components folder, create a new file named ListItem.jsx and add the following code to it.
This component renders a single item in the list using the AntD Card component. The title of the card is provided as a component prop. In addition to the title, this component receives three other props:
- The
onSelect
prop is used to notify the parent item that the card has been clicked item
corresponds to the gist or repository which will be rendered on the cardselectedItem
is used by the component to determine if the rendered item was clicked by the user; in which case a light blue background is added to the card styling.
Next, create a new file named MasterDetail.jsx in the components folder and add the following code to it.
This component is responsible for rendering the list of items in one column and the details of the selected item in another column. The items to be rendered are provided as a prop to the component.
In addition to that, the getItemDescription()
prop is a function to get what will be displayed under the user avatar; this is the repository name or the Gist description.
The detailLayout()
prop is a function provided by the parent component which returns the JSX content for the detail section based on the provided item. This allows Gists and repositories to have entirely different layouts while using the same child component for rendering.
Add repository-related components
Next, in the components folder, create a new folder named Repository to hold components related to a repository. Then, create a new file named RepositoryDetails.jsx and add the following code to it.
Next, create the component for rendering public repositories. In the components/Repository folder, create a new file named PublicRepositories.jsx and add the following code to it.
This component makes the call to retrieve public repositories from the GitHub API. It does this using the GetPublicRepositories()
function declared in app.go which is automatically bound to the frontend by Wails.
Functions exported in this manner are asynchronous and return a Promise. Using the MasterDetail
and RepositoryDetails
components, the returned response is rendered accordingly.
Next, create another file named PrivateRepositories.jsx in the Repository folder and add the following code to it.
This component is very similar to the PublicRepositories
component but for two key things. First, this component will be wrapped with an AuthContextProvider
, which makes it possible to retrieve the saved token via the useAuthContext
hook. Second, it uses another bound function GetRepositoriesForAuthenticatedUser()
to get the repositories for the user whose token was provided.
Add Gist related components
Next, in the components folder, create a new folder named Gist to hold components related to a Gist. Then, in that new folder, create a new file named GistDetails.jsx and add the following code to it.
This component renders the code in the file(s) for a given Gist. Every Gist response comes with a files
key. This is an object containing all the files for the Gist. Each file object contains the URL to the file’s raw content and the language associated with the file. This component retrieves all the files using the GetGistContent()
function and renders them in a Carousel. Prism is used to render the code as would be found in an IDE.
Next, in the Gist folder, create a file named PublicGists.jsx and add the following code to it.
Just as was done for the rendering of public repositories, the GetPublicGists()
function declared in app.go is used to retrieve public Gists from the Github API and pass them to the MasterDetail
component, along with the functions to get the Gist description and display more information on the Gist when selected.
Next, create a new file named PrivateGists.jsx in the Gist folder and add the following code to it.
This component will be wrapped with an AuthContextProvider
component, thus giving it access to the provided token. Using the token, an asynchronous call is made to the GitHub API via the GetGistsForAuthenticatedUser()
function. The results are then passed to the MasterDetail
component along with the other required props for appropriate rendering.
The last Gist related component to be built is the form to create a new Gist. To do this, create a new file named CreateGist.jsx in the Gist folder and add the following code to it.
The request to create a new Gist has three fields:
description
: Where provided, this will describe what the code in the Gist aims to achieve. This field is optional and is represented in the form by an input fieldpublic
: This is a required field and determines whether or not the Gist has public access. In the form you created, this is represented by a switch which is set to off by default. This means that unless otherwise specified by the user, the created Gist will be secret and only available to users who have its link.files
: This is another required field. It is an object and for each entry in the object, the key is the name of the file (with the extension included) and the value is the content of the file.
This is represented in the form you created as a dynamic list with each list item consisting of a text field for the file name and a text area for the file content. By clicking the Add File button, you have the ability to add multiple files. You also have the ability to delete a file. Note that you will be required to have at least one file and if you do not, an error message will be displayed.
When the form is properly filled out and submitted, the onFinish()
function is used to create an object conforming to the Gist
struct declared in app.go and a call is made to the CreateNewGist()
receiver function.
Because this component is wrapped with the AuthContextProvider
, the saved token can be retrieved and passed alongside the Gist as required by the function. Once a successful response is received, the app redirects to the list of Gists for the authenticated user.
Put the pieces together
Add navigation
With all the individual components in place, the next thing to add is navigation - a means by which the user can move around the application. To add this, create a new file in the components folder named NavBar.jsx and add the following code to it.
This component renders a navigation bar at the top of the window with two main items - Public Actions and Private Actions. Each item then has sub-items, which are links that will eventually render the component associated with the sub-item. With this in place, you can add routing to your application.
Add routing
In the frontend/src folder, create a new file named routes.jsx and add the following code to it.
Here, you specified the routes in the application as well as the component to be rendered for each path. In addition to that, you have wrapped the components which require the user to provide a token with the AuthContextProvider
component.
Next, open App.jsx and update the file's code to match the following.
Here, you have included the NavBar
component you declared earlier. You also declared an Outlet
component which is provided by react-router-dom
to render child route elements.
Finally update the code in main.jsx to match the following.
A HashRouter is the officially recommended approach for routing. This is created via the createHashRouter()
function. Using the routes
object you declared earlier, all router objects are passed to this component to render your app and enable the rest of the APIs. With this in place, your application will render the index page once it loads.
Test that the application works
You’ve successfully built your first app with Wails. Run the application again and take it for a spin by running the following command from the project's top-level folder.
By default, when the app loads, you will be greeted with a list of public repositories. Using the navigation menu, you can view public (and private) repositories and Gists by clicking the corresponding menu item.
When you select a menu item for a private repository or private Gist, a pop-up will be displayed asking for your GitHub token as shown below.
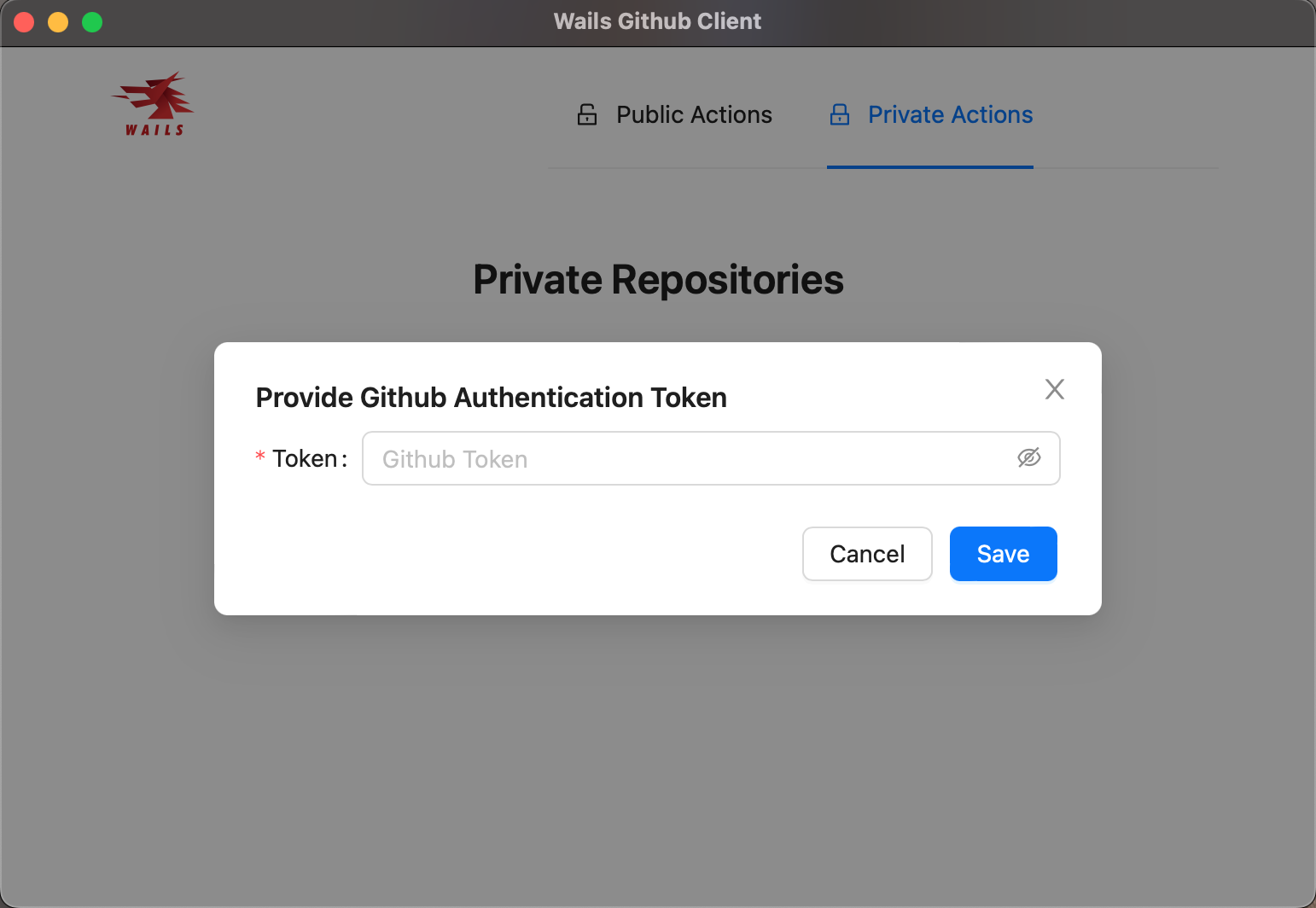
Paste your Personal Access Token (PAT) and click Save. Your repositories (or Gists as the case may be) will then be rendered. You will be able to navigate around the private section of the app without having to re-enter your token for a few minutes.
And that's how to build a cross-platform desktop application with Go and Wails
There’s still a lot you could do. For example, how would you handle ensuring that the token provided by the user is valid before trying to perform authenticated actions?
What other features do you think you can add to the application? Did you know that you could make further customizations to the app such as the width and height, or even start off in full-screen mode? Have a look at the Options documentation to see how you can further configure your app.
In case you get stuck at any point, feel free to access the codebase here.
I’m excited to see what more you come up with. Until next time ✌🏾
Joseph Udonsak is a software engineer with a passion for solving challenges – be it building applications, or conquering new frontiers on Candy Crush. When he’s not staring at his screens, he enjoys a cold beer and laughs with his family and friends.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.