How to confirm phone number ownership with Lookup Identity Match
Time to read: 3 minutes
There's a lot you can learn from a phone number. Lookup Identity Match uses phone numbers as an identity to provide a zero-knowledge match against authoritative data sources like mobile carrier records. This allows you to build real-time confirmation that you're interacting with a real person linked to the phone number provided.
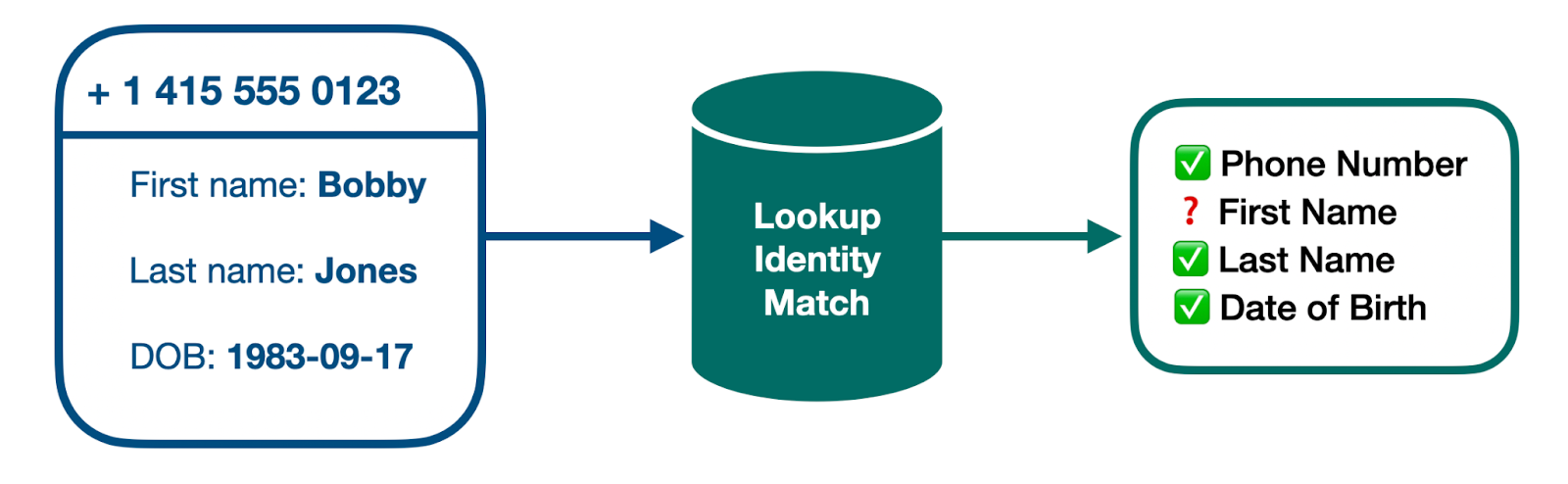
Use Identity Match to prevent fake accounts and improve trust with legitimate, unique users. Customers can use Identity Match at sign up, login, and even during account changes or checkout. Best of all, Identity Match happens seamlessly in the background, with lower costs and friction than alternatives like credit bureau and document verification used to satisfy Anti-Money Laundering (AML) rules.
This blog post will show you how to use Twilio Lookup Identity Match to confirm phone number ownership and reduce sign up fraud.
Here's a sneak peak at what the Identity Match request looks like. Learn more about accepted parameters and coverage in the documentation, plus how to test different outcomes with our "magic numbers" and special parameters.
Prerequisites for building with Lookup Identity Match
To code along with this post you'll need:
- A Twilio account. Sign up or sign in.
- [Outside US only] Submit this form for Identity Match approval (takes 2-4 weeks)
- Twilio CLI (I'm using v5.5.0)
- CLI Serverless plugin (run
twilio plugins:install @twilio-labs/plugin-serverless
)
This post uses the international telephone input Code Exchange project as a starting point, but you could use your own sign up form instead. The base project relies on the excellent intl-tel-input plugin for collecting phone numbers in E.164 format.
Start by cloning the example phone number input using the Twilio CLI:
Add your Auth Token in the created .env
file within the identity-match folder. Test out the project by running twilio serverless:start
, you should be able to open http://localhost:3000/index.html and see a phone number input form.
Extend your sign up form with Identity Match
Identity match needs information about the phone number owner to match against. Parameters can be any combination of name, address, national ID or date of birth.
This example is going to use first and last name, so you need to start by making sure your form has fields to collect those separately:
Open assets/index.html and replace everything inside the <form>
tags with:
This adds a first name and last name input in addition to the phone number input.
Further down in index.html in the script section, add the following code under const data = …
to extract the name input values.
Then head over to functions/lookup.js to make use of the data and call the Lookup Identity Match API. The existing code already calls the basic Lookup API for formatting and validation, so you need to add the additional data parameters to tell the API to use the Identity Match package.
Inside the try
block but before const client
, create a data
object:
Then add data
as a parameter to the the existing Lookup fetch like so:
How to interpret the results of a Lookup Identity Match response
After calling the Lookup API, you need to decide what to do with the information in the response. Here's what the identity match portion of a sample response looks like:
Possible responses include exact_match
, no_match
, and no_data_available
. Name and address fields also support partial matches. Learn more about match scores in the documentation. The API will respond null
if you don't provide an input to match on.
This application is only looking at name matches and will consider the match a success if it's an exact match or high partial match (e.g. Kelly instead of Kelley – happens all the time).
Back in lookup.js, replace const success = …
with the following:
Then replace the .setBody
call inside the if (success)
block with:
Finally, make two small changes to display the response message in index.html. Replace the inside of the if (json.success) {
block with:
And since you're no longer only checking the phone number, update the error message in the else
block a few lines below:
Launch the application with twilio serverless:start
and test it out!
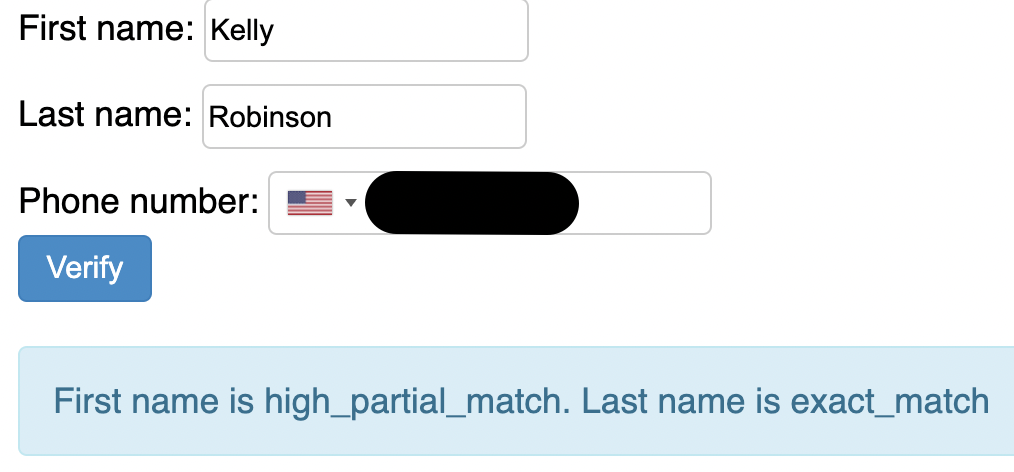
Next steps for identity verification
Try out different combinations with both real data and the test parameters to see the results.
You can extend your application with other Lookup packages like Line Type Intelligence, SIM swap detection and more. Check out our documentation to learn more about what's possible with the Lookup API.
Another way to prevent invalid phone numbers is to do phone verification - we also have an API for that! I can't wait to see what you build and secure.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.