Forward Voicemail Transcriptions to a Slack Channel in Laravel PHP with Twilio Voice
Time to read:
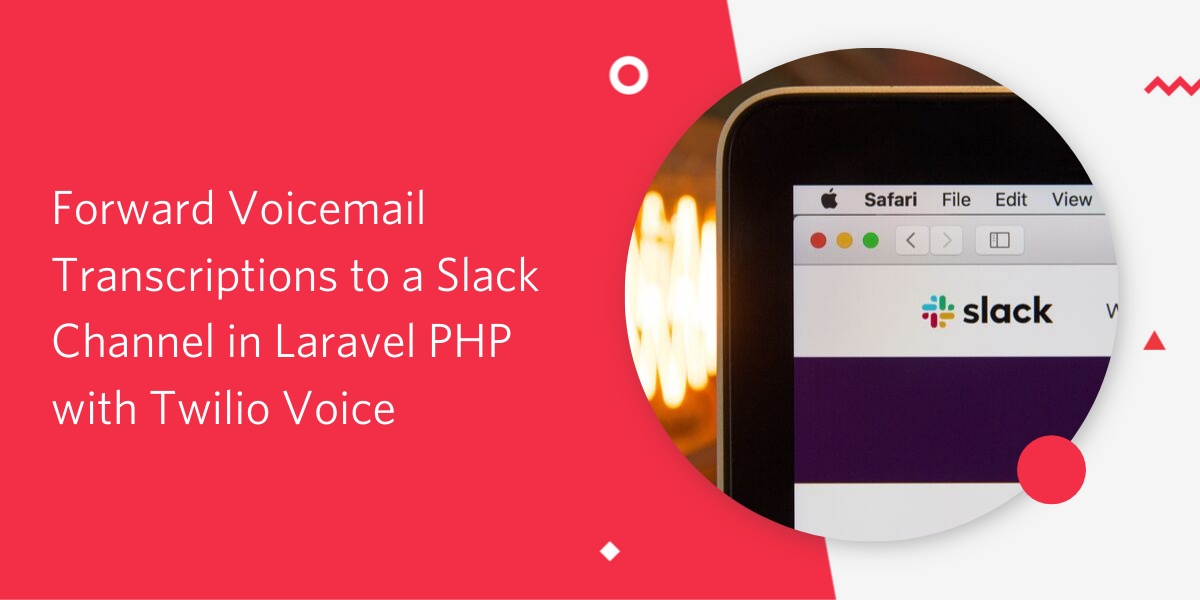
In a business environment, audio transcripts allow for easier collaboration as transcripts are easy to distribute and store. It also helps businesses to better understand their customers, which leads to improved customer experience. Lastly, it increases accessibility, particularly to people who are hearing-impaired and non-native speakers.
At a personal level, transcripts can help one to quickly discover actionable insights and improve accuracy as there is a written record that can be referenced.
Transcribing doesn’t have to be tedious. Using automated software and tools can expedite the process, thus saving you time to focus on other important things. One tool to expedite transcribing is Twilio’s Voice API.
In this tutorial, we are going to discuss how to transcribe voicemail and send the transcript to a Slack channel using Twilio.
Getting Started
To complete this tutorial, you will need the following:
Create a New Laravel Project
Create a new Laravel project by running the following command in your terminal:
Install Twilio PHP SDK
To get started, we first need to install the Twilio PHP SDK in our project using Composer. This will allow our application to easily connect to the Twilio API and programmatically record inbound calls as voicemails. In your terminal, run the command below:
Require Guzzle dependency
Guzzle is required to execute the HTTP API requests. To install Guzzle, navigate to the project directory and run the following command:
Create a Slack Incoming Webhook
An Incoming webhook enables you to share information from external sources with your slack workspace.
Log in to your Slack account and navigate to the create Slack app page. Enter the name of choice, choose a workspace, and click Create App.
You will be redirected to the settings page for the new app. On the page, select “Incoming Webhooks” and click “Activate Incoming Webhooks” to toggle and turn on the feature.
The page should refresh and display extra options once you enable the incoming webhook. Click the “Add New Webhook to Workspace” button. Select a channel that the app will post to, and then click Authorize to authorize your app.
You’ll be redirected back to the app settings page which should now have a new entry under the “Webhook URLs for Your Workspace” with a webhook URL.
Set Environment Variables
Go to Slack and retrieve the webhook URL we have just generated above and update the values in the .env
file:
Creating the Transcribe App
We will create a model and extend it with custom methods to save calls as a voicemail, receive the transcript, and forward the transcript to the Slack channel created earlier.
Next, we will create a controller to organize the route level logic and call the logic from the model.
Create the Transcribe Model
Run the command below to create the Transcribe
model:
Create the Transcribe Controller
Next, run the command below to create the TranscribeController
controller:
Create Logic to Save Voicemail, Create Transcript and Send to Slack
The first step is to create a function to generate TwiML for the inbound calls.
Secondly, create logic to send the transcript to the Slack channel.
Open the file app/Transcribe.php
and add the code below:
NOTE: Remember to update 'transcribeCallback' attribute with the route in your application that will receive the request with the transcript details once it is available for access.
Next, let's create the functions in the controller to handle the routing logic.
Open the file app/Http/Controllers/TranscribeController.php
. Add the code below:
Create the Endpoints
At this stage we need to create routes; the Twilio webhook and the callback URL to send the transcript to the Slack channel.
Inside routes/api.php
, add these lines of code:
Create a Temporary Public URL using Ngrok
To test our app locally, we need to set up Ngrok. It assigns a local port a publicly accessible URL over the Internet. Once ngrok is set up, run the command below to expose our Laravel app running on port 8000 to the Internet.
Configure Twilio Phone Number Webhook
In the Number settings of your Twilio account, update the value for when “A call comes in” to NGROK_BASE_URL/response
.
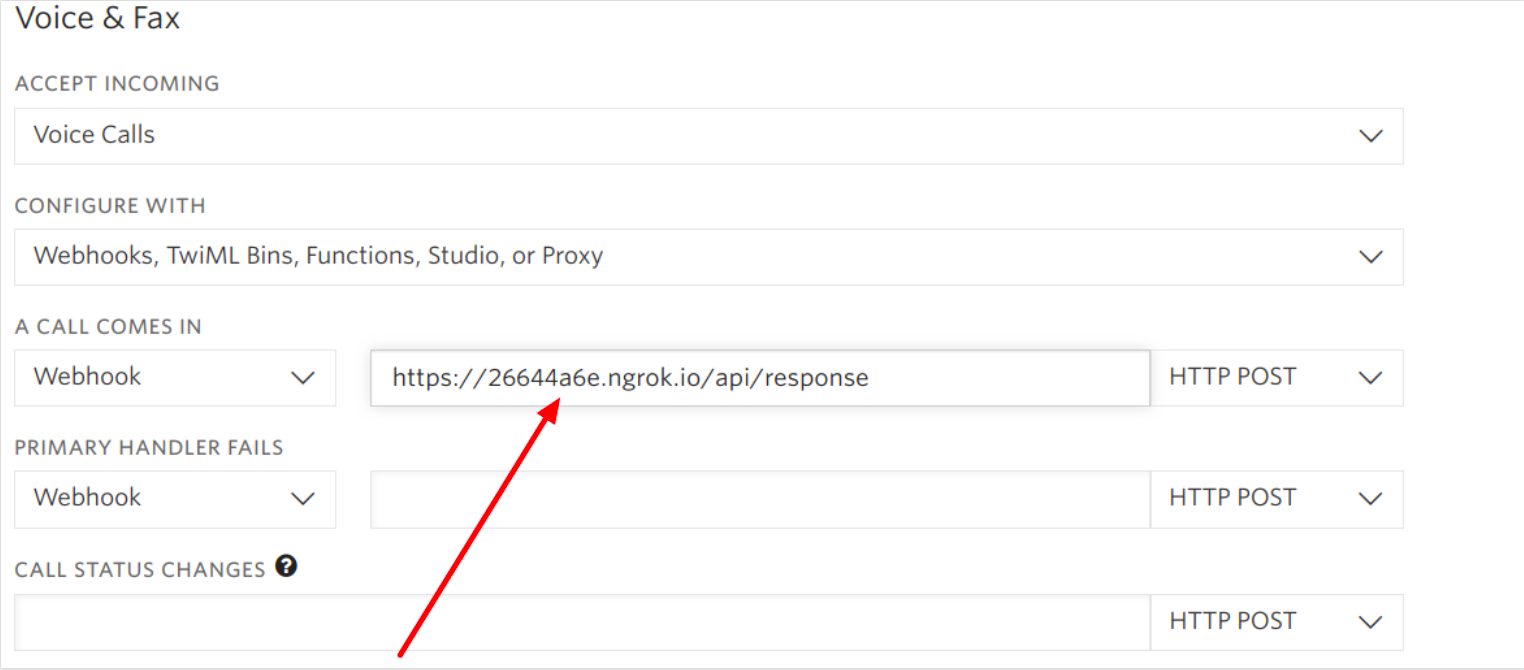
Testing
Finally, Let’s test the completed code. Call the Twilio phone number we have just configured above.
You should get the transcribed text in your slack channel as shown below.

Conclusion
External services like Google Speech to Text can improve the quality and accuracy of the transcripts. Also, Twilio provides add-ons to further customize your responses.You can check out the list of available Twilio Add-ons here.
The code is available on Github.
Have some ideas to better improve the app? Let me know. I can’t wait to see what you build.
Feel free to reach out to me:
- Twitter: @mikelidbary
- GitHub: mikelidbary
- Web: www.talwork.net
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.