Send PDF Invoices via WhatsApp using Laravel, PHP, Stripe, and the Twilio API for WhatsApp
Time to read: 4 minutes
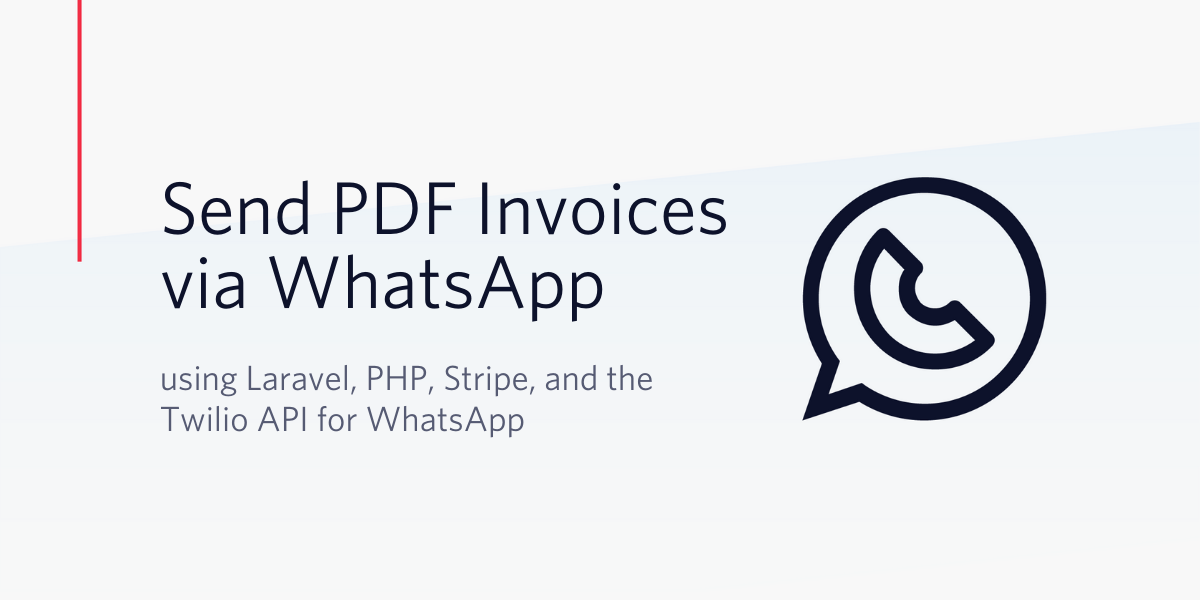
Stripe is a payment gateway that provides developer APIs to help you receive payments from your application/website.
The Twilio API for WhatsApp provides a platform that helps you send any type of business message via WhatsApp through a streamlined API.
In this tutorial, we will be exploring how to send payment invoices and notifications to our users via WhatsApp when they make payments on our website.
Pre-requisites
To follow along with this post, you will need the following:
- A Twilio account (Sign up for a free account now)
- Composer, Ngrok and Laravel CLI installed on your machine
- Knowledge of the Laravel framework
- A Stripe account
Getting Started
To get started, create a new Laravel application and assign the folder name.
Next, install our application dependencies using composer
. These dependencies include the Twilio SDK and the Stripe PHP library (to communicate with the Twilio and Stripe API respectively) as well as Laravel DomPDF (to generate PDFs from HTML/Blade templates).
The Stripe library uses a pair of publishable and secret keys (which can be retrieved from the Developer Section on your Stripe dashboard) for authentication. Similarly, the Twilio SDK uses your Account SID and Auth token (which can also be found on your Twilio console). Grab those credentials and append them to your .env
file.
Our application requires two tables in our database, products
and users
. Open the users migration file created by Laravel (YOUR-APP-NAME/database/migrations/2014_10_12_000000_create_users_table.php
) and change theup
method to the following:
Then, create a new migration for the products table with the command below:
And paste in the following in the newly created file.
We need to seed both of the tables with sample data. Let’s generate Seeder classes for the tables we just created.
Next, we need to change the code in the app/database/seeds/UsersTableSeeder
class to the one below:
In the same way, let’s paste the following code into our app/database/seeds/ProductsTableSeeder
class.
Run the following commands to apply the database migrations and populate the tables with the seed data.
NOTE: You will need to update the .env
file with your database credentials before you run the migrations.
Since the User model class was generated when we created the application, we only need to create a single model for our products. This is achieved with Artisan’s make:model
command.
Setting up the Controller
For simplicity, we will let the auto-generated Controller file handle all of our application logic. For now, the controller only needs three methods:
- An
index
method that renders the home page. - A
checkout
method that renders the checkout page (which we will re-use later to send our users their invoices) and - A
charge
method to charge our users using the Stripe API.
Below is the controller file and the implementation of the methods listed above. Open up app/Http/Controllers/Controller.php
and replace the code with the content below:
Setting up the Views
Our sample application provides two views for our users to interact with our application. The home page (rendered by welcome.blade.php
) displays a list of products from which our users pick a product to buy. Upon selection, they are redirected to the checkout page (rendered by checkout.blade.php
) which allows the user to pay and complete their purchase. Since we are using the default welcome template, open resources/views/welcome.blade.php
and paste in the following:
Also, create our checkout template at resources/views/checkout.blade.php
and add the code below:
Now that our application’s logic and views are up, let’s inform the routes file of the changes we have made by adding the code below to routes/web.php
.
Sending our First WhatsApp Message via the Twilio SDK
To use the Twilio API for WhatsApp in production, we need a WhatsApp-approved account. However, for this tutorial we’ll only need the Twilio sandbox for us to test our application in a development environment. Grab your Twilio Sandbox phone number by following the instructions in the related Twilio Docs and append it to your .env
file.
Next, we modify the checkout
method of our Controller
class to send a WhatsApp message at the point of checkout. Replace the method with the code below:
NOTE: Make sure to replace the phone number placeholder with the E.164 format of your WhatsApp phone number i.e, both the “from” and “recipient” fields should be of the form “whatsapp:+14155238886”
Click the “Buy” button for a product from the home page and you should receive a message in the WhatsApp app on your mobile device just before the checkout page is rendered.
Attaching the Invoice as PDF
The Twilio API for WhatsApp allows us to attach media files to our WhatsApp message as long as the file sizes are less than 5MB and they are hosted on a publicly accessible URL. Create the invoice blade template at resources/views/invoice.blade.php
and add the following:
Additionally, add a mediaUrl
field to the parameters being passed to the Twilio client as shown below:
NOTE: You will need to create the invoices
folder in the public
folder. You will also need to update the user’s phone number (directly in the database) to your WhatsApp phone number in E.164 format.
The NGROK_URL
environment variable is the forwarding URL provided to us by ngrok when we start the tunnel. In a terminal window, start up the Laravel server (if it isn’t already running) and the ngrok tunnel with the following commands:
Next, copy the ngrok forwarding URL and append it to your .env
file .
Go ahead and click the “Buy” button for a product on the home page, if everything goes well, you should receive a WhatsApp message with the invoice PDF as an attachment on your WhatsApp phone number.
Conclusion
WhatsApp is one of the world’s most popular messaging applications and the Twilio API for WhatsApp gives you the ability to send conversational messages and notifications directly to your users on there. You can find the complete source code for this tutorial on Github and if you have an issue or a question, please feel free to create a new issue on the repository.
Michael Okoko is a student currently pursuing a degree in Computer Science and Mathematics at Obafemi Awolowo University, Nigeria. He loves open source and is mostly interested in Linux, Golang, PHP, and fantasy novels! You can reach him via:
Additional Reading
If you’d like to learn more about building with the Twilio API for WhatsApp, check out the following documentation:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.