How to Send Email in Java using Twilio SendGrid
Time to read: 2 minutes
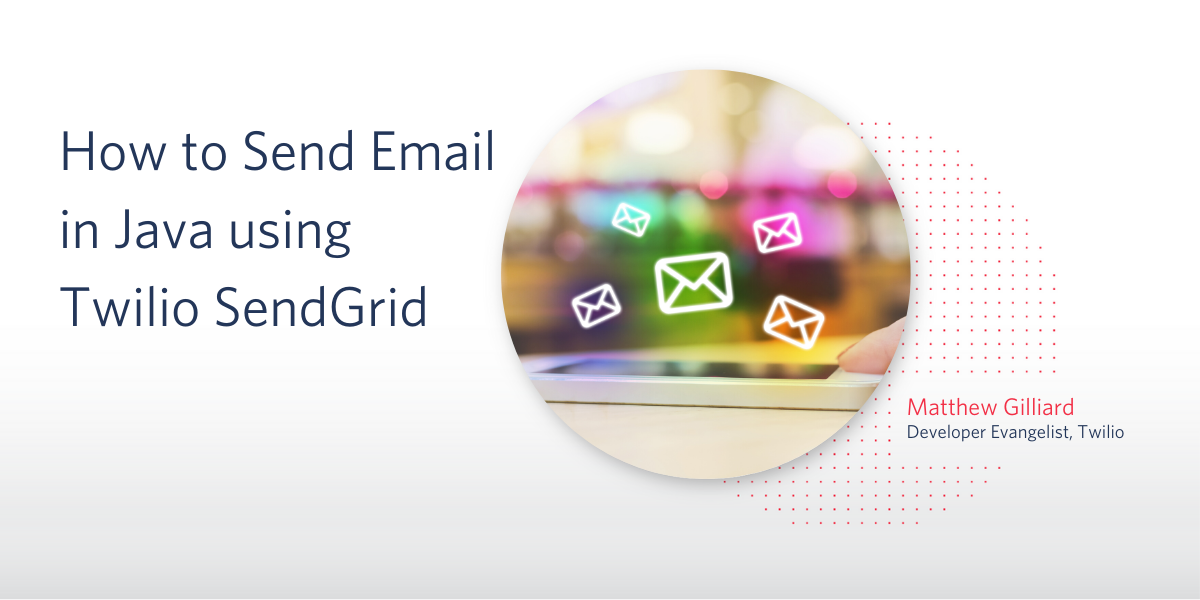
If you’re writing a Java app and you need to programmatically send some emails, the Twilio SendGrid API is just the thing you need.
You’ll need to sign up for a free Twilio SendGrid account and create an API key. Then you can get started with a few lines of code.
In this post I’ll go through each step to get you up and emailing in no time.
Starting Out
You’ll need:
- Java. I’m using JDK 11 but 8 is fine too.
- A free SendGrid account
- The SendGrid helper library for Java
The first thing you should do is to create a SendGrid account. The free tier will work just fine for this tutorial. Once you are signed up and logged in, create an API key. You can choose any name you like, but make sure to save the key before moving on.
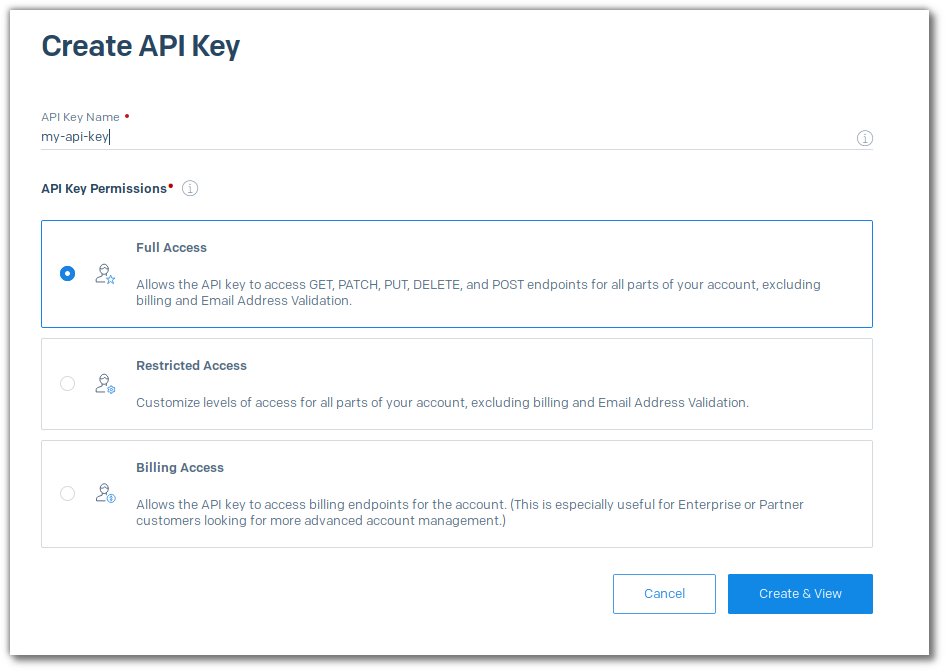
Choose “Create & View” then make sure to copy your key somewhere safe:
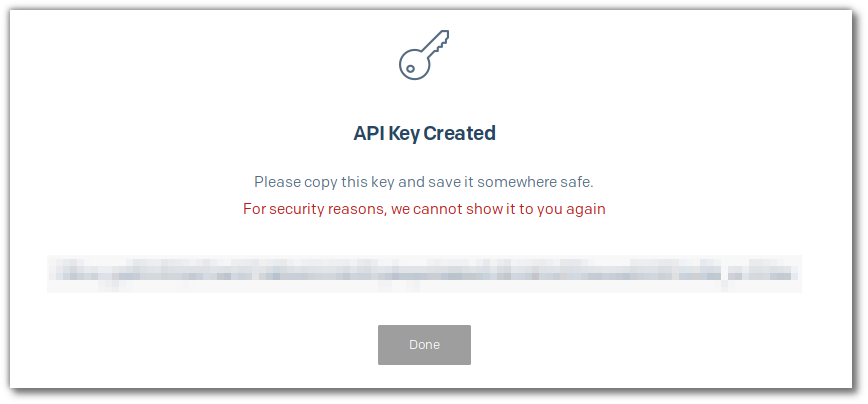
To avoid writing your API key in your Java code, it is best to store it as an environment variable. That way you can share your code without exposing an API key that would give access to your SendGrid account. IntelliJ and Eclipse can manage environment variables for you, or you can set them manually. For this post I’ll assume you have set an environment variable called SENDGRID_API_KEY
.
Sending an Email with Java
I strongly recommend using an IDE and a build tool for your Java projects. Eclipse and IntelliJ IDEA Community Edition are good free IDE choices, and Apache Maven and Gradle are both popular build tools. Whichever combination you choose you can use the IDE to create a new project. Add the SendGrid helper library to your project configuration:
Gradle config, add this in build.gradle
:
or Maven config, add this in pom.xml
:
Create a new class called SendGridEmailer
in your project with the following content:
Notice that on the highlighted line the code reads your API key from an environment variable, so be sure to set this in your IDE. If you forget to do this you will see an error: The provided authorization grant is invalid, expired, or revoked
.
Run the code in your IDE to send yourself an email, then check your inbox:
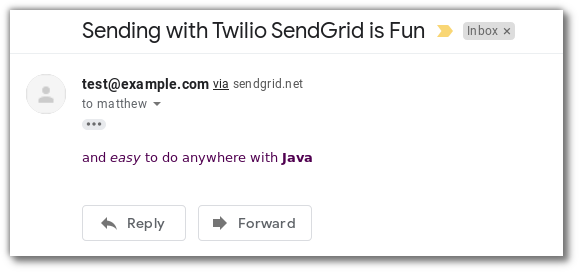
What next?
You just sent your first email using Java and SendGrid, but what else could you do? You can process and respond to incoming mail using SendGrid’s Inbound Parse Webhook, add attachments and personalizations, schedule mail or check the SendGrid API docs for lots more ideas.
I can’t wait to hear about what you build. Feel free to get in touch with your experiences, comments or questions.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.