Build phone verification in React Native with Twilio Verify
Time to read: 4 minutes
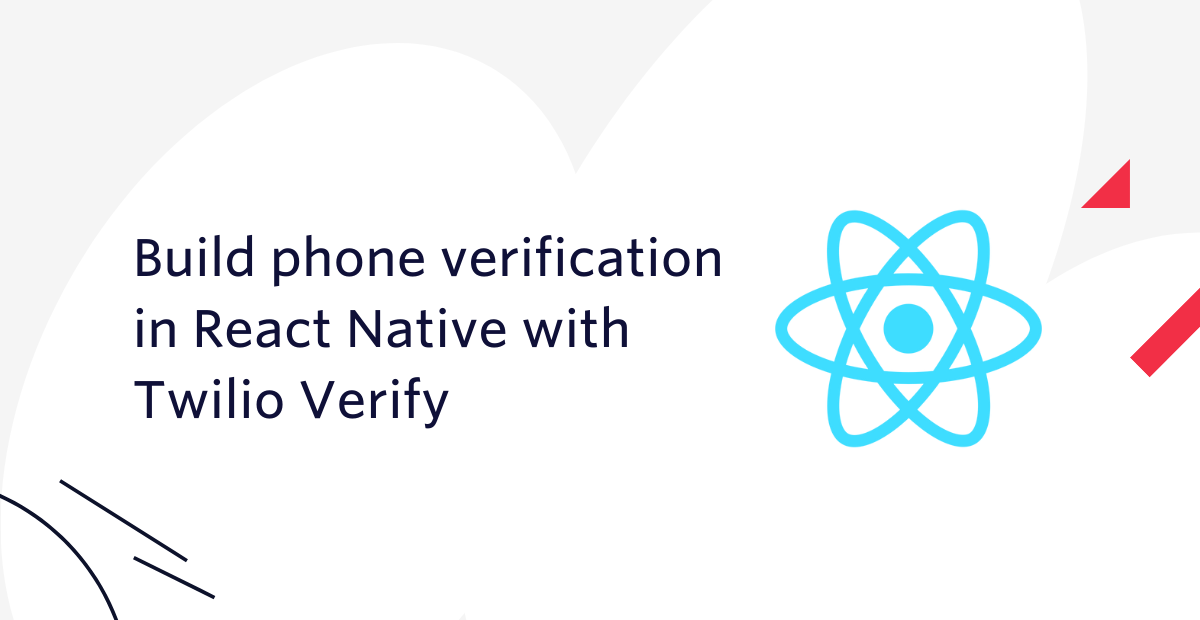
SMS verification is a great way to onboard your users, especially in a mobile app where they have access to their text messages. Phone verification can also help ensure unique users, decrease spam and fraud, or can be used for ongoing login verification. There are many options for verifying users, but SMS verification remains a popular choice for its ease of use.
This blog post will walk you through how to set up a React Native application from scratch and how to use Twilio's purpose built Verify API for SMS phone verification. If you want to skip ahead, you can find the completed code on my GitHub.
Prerequisites for building phone verification in React Native & Expo
Set up your React Native developer environment
We're using Expo CLI and Expo GO for this tutorial which is quick to set up and get running. You can learn more about the difference between using Expo CLI and the React Native CLI here. You can also find a version that uses the React Native CLI instead of Expo on my GitHub.
Follow the setup instructions in the React Native docs under the Expo CLI quickstart tab:
To test the project on a device, install Expo on an iOS or Android phone. You can also set up iOS or Android emulators on your computer. Check out the "React Native CLI" tab for more details on setting up emulators with Android Studio or XCode.
Set up your Twilio Verify backend
Sign up for or log in to your Twilio account and create a new Verify Service. This is what will allow you to send SMS verification messages.
Then head over to Twilio's Code Exchange and deploy the One Time Passcode (OTP) verification project. By default you'll have a nice web interface for testing verifications, but it also provides serverless functions hosted on Twilio for sending and checking OTPs that we will use from the mobile app. Make note of the base url for your deployed project, it will look something like this https://verify-1234-abcdef.twil.io
(you can also grab this later).
Create a new React Native application
Now that your environment is set up, create a new project called VerifySms
and choose the TypeScript template.

Then run the project with yarn start
. It will spin up Metro in your browser. If you installed Expo on your phone, scan the QR code (make sure you're on the same WiFi network) to bring up the app. You can also launch it in the emulator if you have that setup.
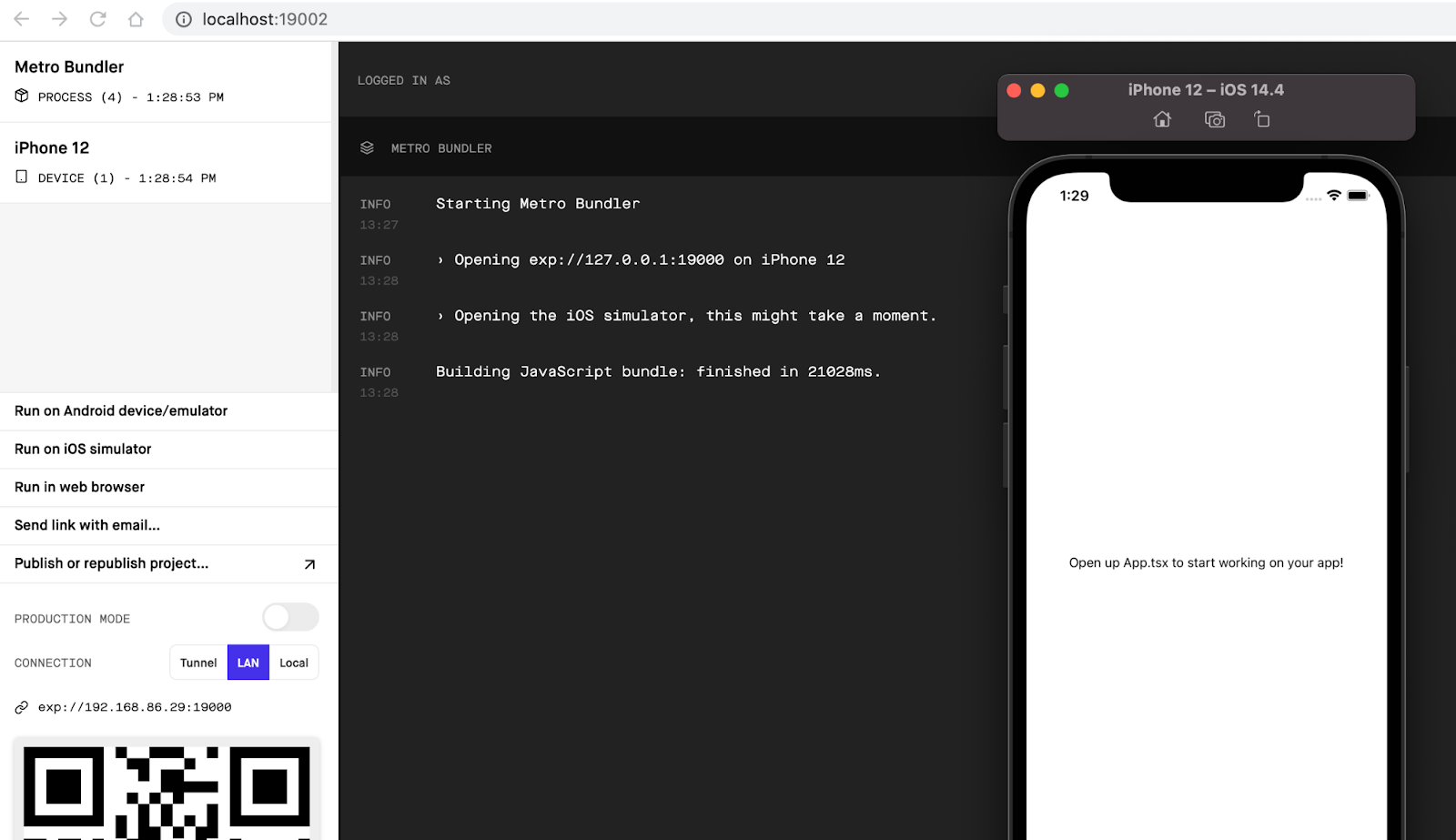
Set up page routing
I promise we'll get to the interesting code soon, but the project will be more manageable if we do some code organization up front.
Create a folder called screens
and add three files: PhoneNumber.tsx
, Otp.tsx
, and Gated.tsx
.
Install the React navigation library we'll be using with:
Then open up App.tsx
and replace the code with the following:
This code allows us to smoothly navigate between screens. You'll get an error if you try to run the code because we haven't created the PhoneNumber screen yet so we will do that next.
Add a phone number input screen for phone verification
We're going to use a library for the phone number input because it turns out there's a lot of intricacies to collecting phone numbers! Run the following command in your terminal to add the React Native phone number input package.
Replace the code in screens/PhoneNumber.tsx
with:
If you reload the app on your device you should see a phone number input field like this:
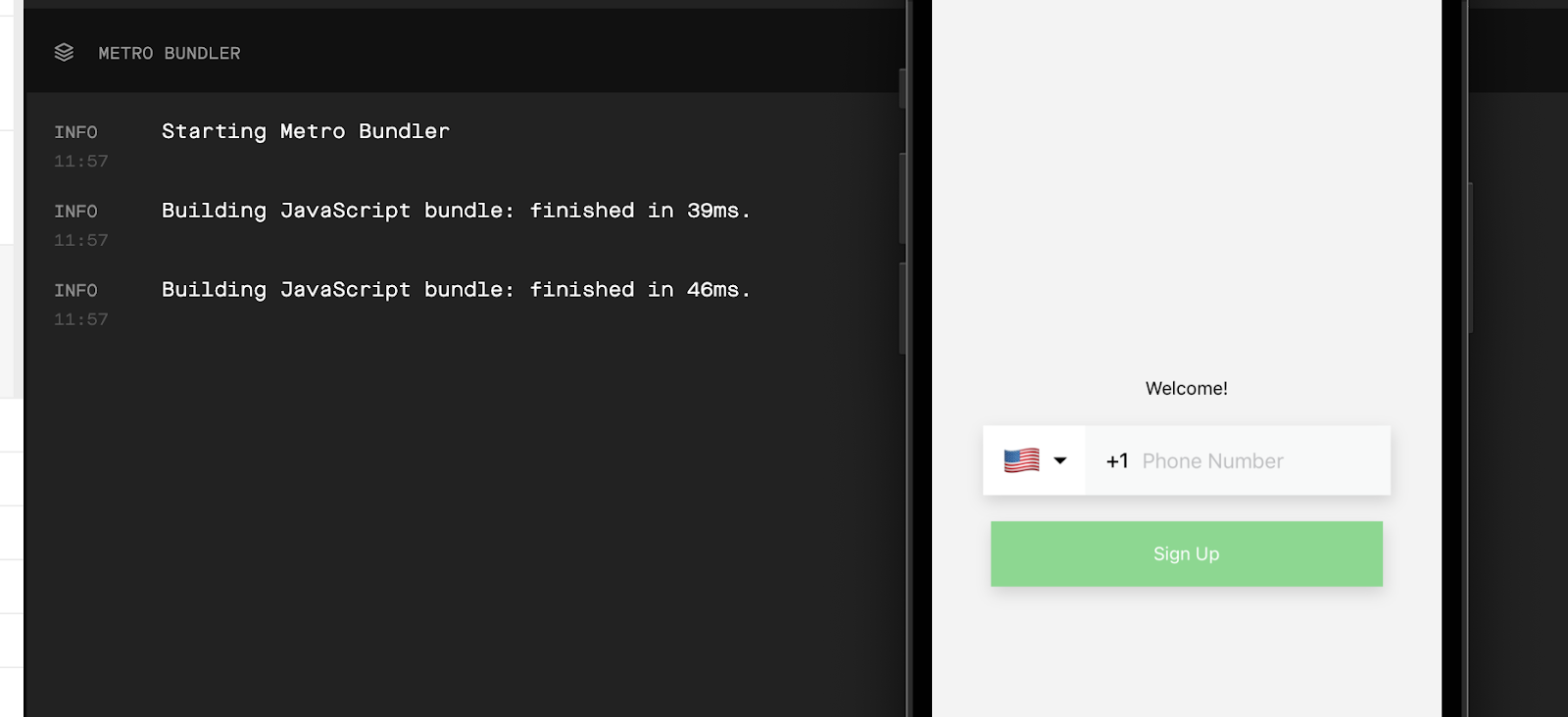
I've set the default country to the US, where I am, but you can customize that and other properties to your liking.
If you enter your phone number and hit "Sign Up" nothing will happen yet so let's build the SMS verification support now.
Grab your base URL from your deployed code exchange project, it will look something likehttps://verify-1234-abcdef.twil.io
. If you lost track of your URL you can find it by clicking on the verify
service in the Functions section of the Twilio Console.
Create a .env
file and add your BASE_URL as a variable:
Because React Native compiles to multiple platforms we need a way to manage environment variables. Install react-native-dotenv with the following command:
Then open up babel.config.js
and update it to:
Create a new folder called api
and add a file called verify.js
with the following code. This is what will actually send and check the SMS verifications using the Twilio Verify API.
Head back to PhoneNumbers.tsx
and import the sendSmsVerification function:
Then inside the <TouchableOpacity>
button where we have a #TODO to send an SMS we can call our function with the formattedValue
of the phone number which is the phone number input in E.164 format:
Pull up the application on your device, enter your phone number, and hit Sign Up. You should receive a text message with a OTP!
Add an OTP input screen for checking codes
We're also going to use a library for handling the OTP input. Add the React Native OTP input package with:
If you're using Android you also need to run the following (track the bug here):
Then open up Otp.tsx
and add the following code:
Learn more about how to customize the view in the docs. This code sets the pinCount
to 6, which is the Verify API's default length. If you change the OTP length in your Verify Service make sure to update that here too.
Head back over to PhoneNumbers.tsx
and update the sendSmsVerification callback to include the following navigation to the Otp screen:
In App.tsx
import the new Otp screen (and our placeholder for gated content) for navigation:
Add in the parameter types. We pass in a phoneNumber
to the OTP screen so we can use it as an identifier for checking the verification.
Register the new screens in the Stack Navigator:
Lastly, add the following code to screens/Gated.tsx
to give us a success view:
Test out your application again and you should be able to input and check the OTP code! Learn more about why you should let people edit their phone number in a phone verification flow in our developer best practices.
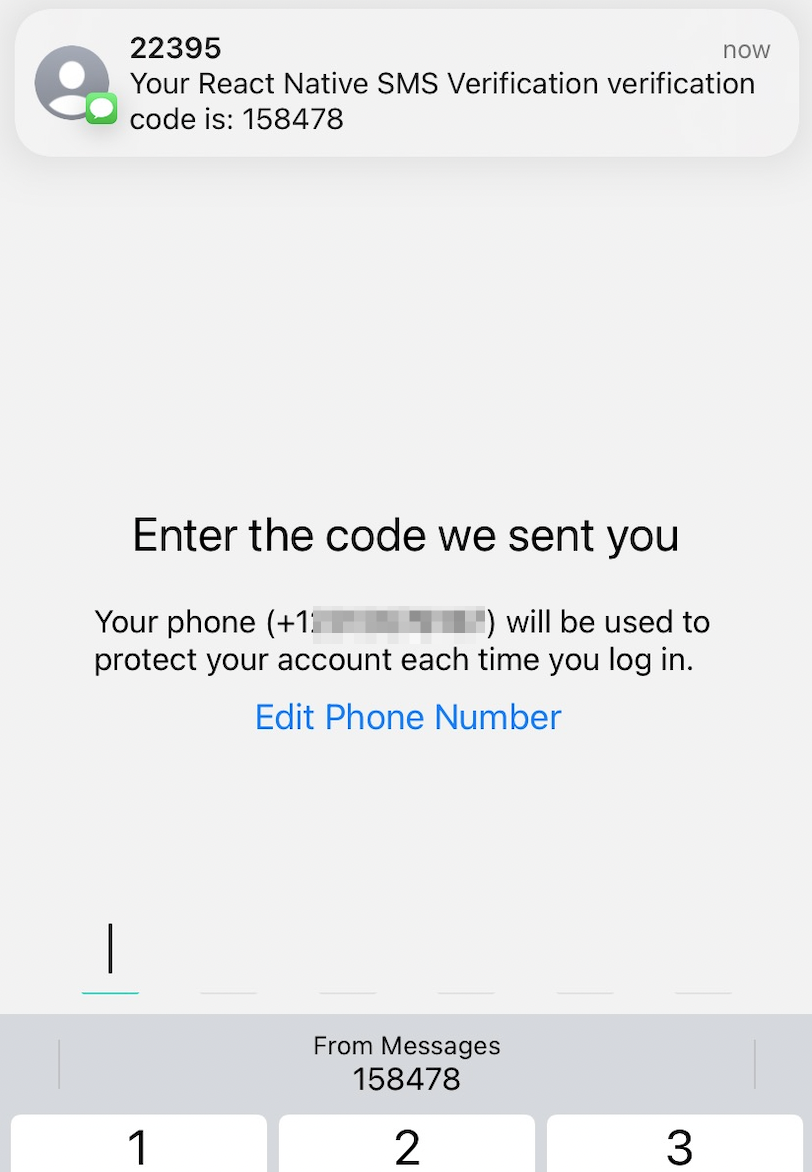
Next steps for building with Twilio Verify
Now that you have SMS verification set up, maybe you want to add additional channels like email, TOTP or Push authentication. Twilio Verify makes it easy to support multiple channels with a single API.
For more resources, check out the following guides, examples and tutorials:
- Silent authentication in React Native
- Verification and two-factor authentication best practices
- 5 reasons SMS 2FA isn't going away
- Verify TOTP Quickstart
I can't wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.