Getting Started With Twilio and Java Play Framework
Time to read:
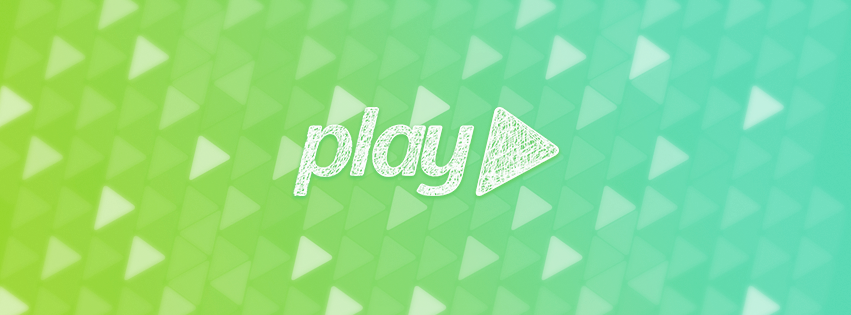
Version 2.3 of the Java Play Framework has recently been released with a move away from the ‘play’ command to the ‘activator’ command. TypeSafe UI has been bundled in as well as support for Java 8. The full announcement can be read here. It seems like a good time to take a look at using Twilio with Play Framework.
Here’s is what we are going to do:
We will then have a simple Play Framework app that can respond to both SMS and telephone calls using Twilio.
Playtime!
Let’s take a look at getting started with Play 2.3. Download the release, and unzip it to get started. You’ll want to add activator to your PATH and you’re good to go. There are instructions for doing this in UNIX/OS X/Windows on the Installing section of the Play Framework site.
Creating a new application is super easy. I’m using OS X, check the documentation for other platforms. At the command prompt type:
This will ask you what type of project you want to create:
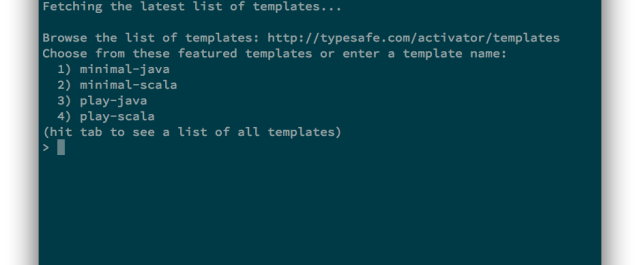
I selected play-java, (option 3) and named the project twilio-and-play.
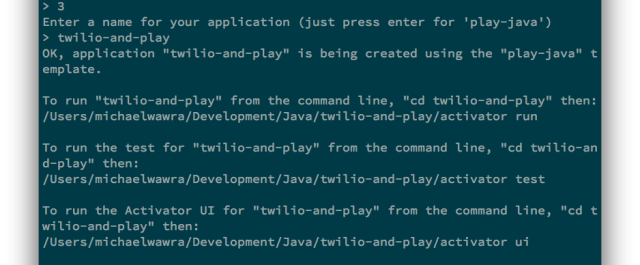
Now, we’re all ready to go. All we need to do is change into the twilio-and-play directory, and type:
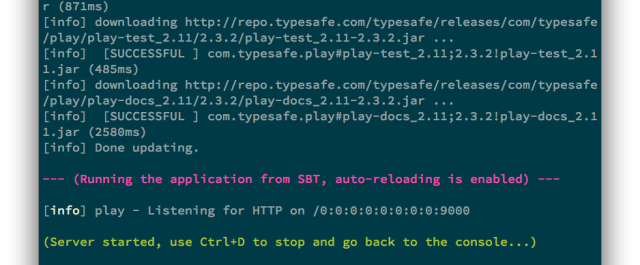
Note that Play has used my IPv6 loopback address, you can still use 127.0.0.1 or 0.0.0.0. But if you’d like to use your IPv6 loopback type [::] or [0:0:0:0:0:0:0:0] into your browser. You can specify port 9000 with [::]:9000 or [0:0:0:0:0:0:0:0]:9000. You should see the welcome screen for your new application.
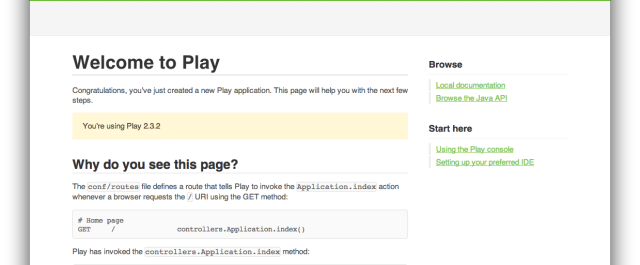
Clearly it is really easy to get started with Play Framework, so let’s find out how easy it is to get started with Twilio.
import Twilio *;
If you don’t already have a Twilio account, you can create one for free by going to https://twilio.com/try-twilio. Enter your name, email, and a password:
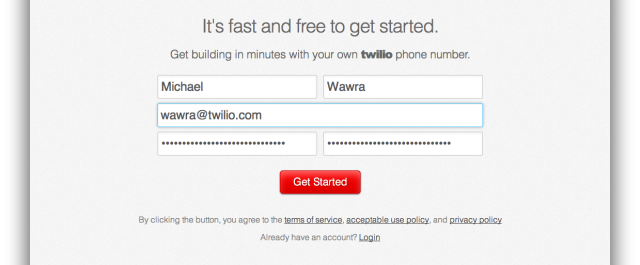
Click ‘Get Started’, and we’re nearly there. Twilio needs to check you’re not a robot using 2-factor authentication. This is a really common use-case for Twilio and my buddy Joel wrote about it in this blog post. All you need to do is enter your mobile number:
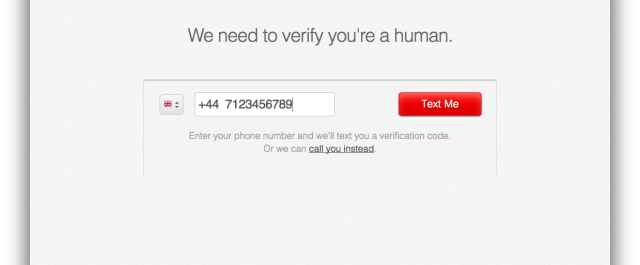
Click ‘Text Me’, and you’ll be sent a code via SMS. Enter the code:
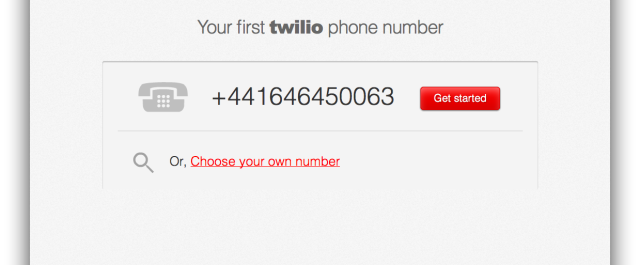
Click on ‘Get started’, and then click ‘Go to your account’ to get started right away. Welcome to your very own Twilio.com dashboard. The two bits of information (highlighted with arrows below) that are most important are your Account SID, and Auth Token. (Click the little padlock to reveal your Auth Token.) These are your username and password to a world a telephony goodness.
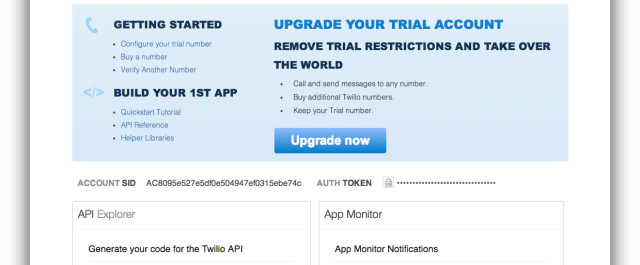
Play working? Check.
Twilio working? Check.
Play Together with Twilio
Play’s activator command has built most of the plumbing for our app, so we just need to include the Twilio dependency. At the time of writing, version 3.4.5 is the most recent release of the Twilio Java Library. We need to modify the Build.sbt file to include this dependency:
Next we need to run:
This will print out a long list of libraries and dependencies that are required. If you haven’t run it before, it make take a little while to install all the packages.
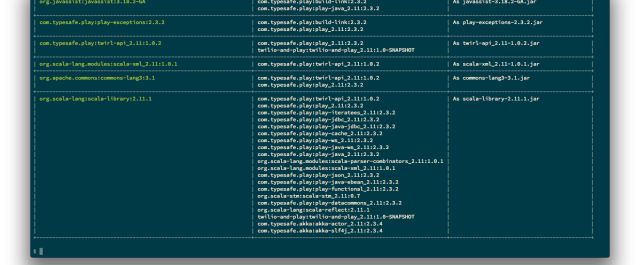
We now have play installed, the Twilio library installed, and all of our dependencies are loaded. It’s time to write some code.
Action!
Play Framework is based on the MVC model. To integrate Twilio we only really need to worry about the controller, as we won’t be storing any data or creating a UI. As a result we can write all of the new actions we need inside the Application Controller (app/controllers/Application.java).
The first thing to do is to import the Twilio Library:
Let’s start with SMS. When a person sends an SMS to our Twilio number, Twilio will send an HTTP GET or POST request to our application with information about the From and Body (as well as To and a few other fields). We need to respond to the HTTP request with some XML that we call TwiML. TwiML always starts with a root element, with a set of nested ‘verbs’ depending on your needs. In this instance, we want to respond with an SMS, so we’ll use the verb. We’ll do this in an action called ‘messages’ on the application controller:
Once we’ve added a new action to our controller, we need to add a URL route so that Play Framework knows to route request to it. We need to add the following line to conf/routes:
This means that whenever a HTTP POST requests is received with a path /messages, Play Framework will call our messages() method. We can run the app with:
You should now see you application up and running, in my case it was on port 9000.
In order for Twilio to access our application, we need to make it available on the internet. To do this, I like to use NGrok, which can provide a globally routable URL for an application running on localhost. My colleague Kevin Whinnery wrote a great post about how to get started.
Essentially, you need to install the NGrok executable, and type ‘ngrok 9000’ (assuming your app is on port 9000):
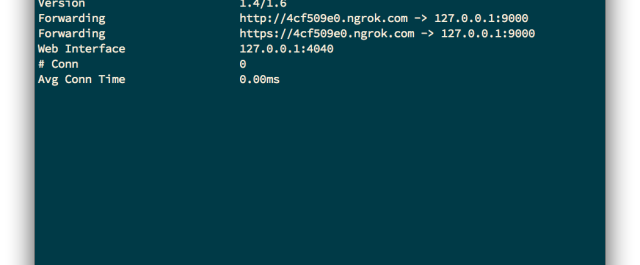
You can now specify this NGrok URL on your Twilio endpoint for messaging. Log into your account dashboard and set the URL using the NGrok URL and the /messages action route.
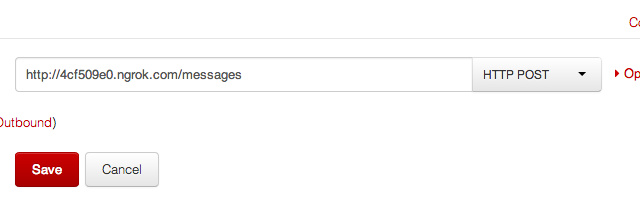
Click save, and reach for your phone. If we send an SMS to our Twilio number we should get a reply, powered by Twilio and Play Framework!
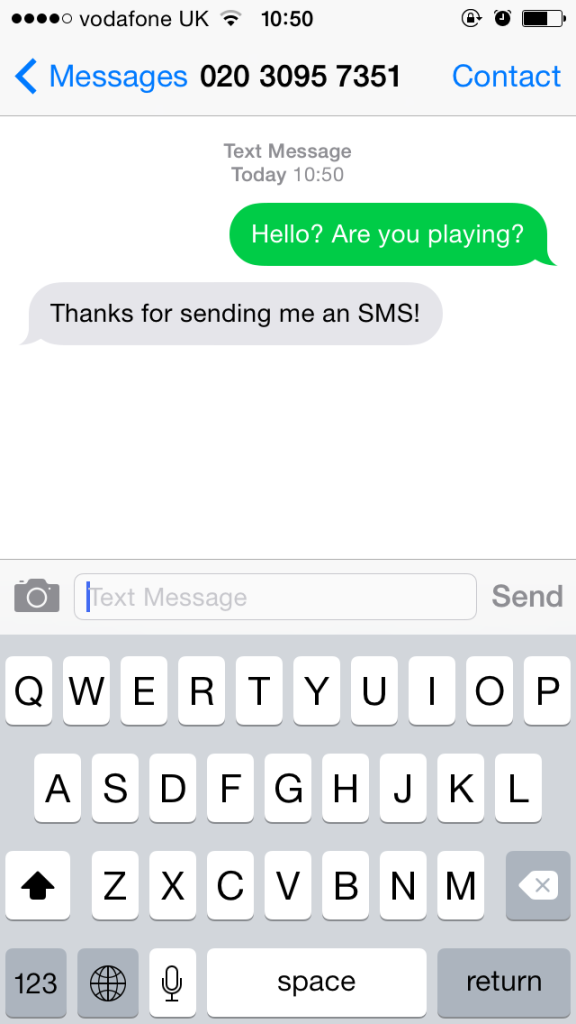
Let’s Talk About It
Well. That was easy.
Let’s teach our Play Framework application to talk. Wouldn’t it be great if I could phone my application and have it talk to me? Let’s create a new action in the Application controller called ‘calls’:
With that, we need to add another route for this action in conf/routes:

Now, we can just call our Twilio number. We don’t need to restart Play or NGrok which is nice. We’ll be connected to our application, and we should hear “Thanks for calling my Play Framework application” in a beautifully synthesized voice using the verb. We could also make an application that s MP3 and Wav files, or a host of other options with TwiML.
Play us out
In just a few minutes we’ve built a simple Java Play Framework application that can handle incoming phone calls and SMS messages using Twilio. I’d love to hear if you’ve built a Play Framework Twilio app, so feel free to drop me an email or a tweet and let me know what you made.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.