Build a Book Recommendation SMS Bot
Time to read: 1 minute
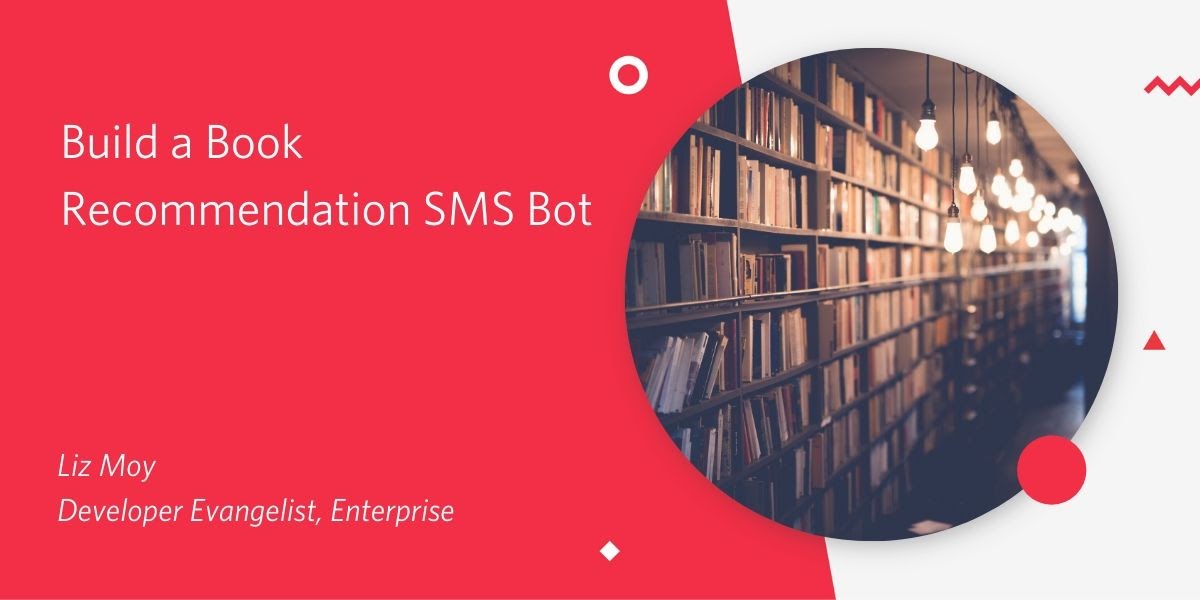
On June 14, publisher Amistad Books launched an initiative to encourage people to purchase any two books by Black writers between June 13th – June 20th.
In addition, artists such as Noname have launched book clubs and dialogue to support Black voices in written works from anti-racist texts, to poetry, to science fiction and fantasy.
To help folks decide on something to read, I built a simple book recommendation bot using Programmable SMS with Python and Airtable to store the book data. The result is a guided conversation that allows the texter to choose a genre they want to read, and responds with a title of a book and a link to a Black-owned bookstore in the U.S. that has the book for sale, either in print,e-book, or audio book.
Try it by sending a text to (409) 404-0403. You can also see the Python code below and an example of the Airtable base that I used.
It always amazes me how versatile a Programmable SMS or WhatsApp chatbot can be. If you’ve spun up anything cool with these tools lately, tell us about it in the comments or send me an email at lmoy [at] twilio [dot] com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.