Resurrecting Shakespeare using Node, Express, Twilio Add-Ons, IBM Watson and Love
Time to read: 3 minutes
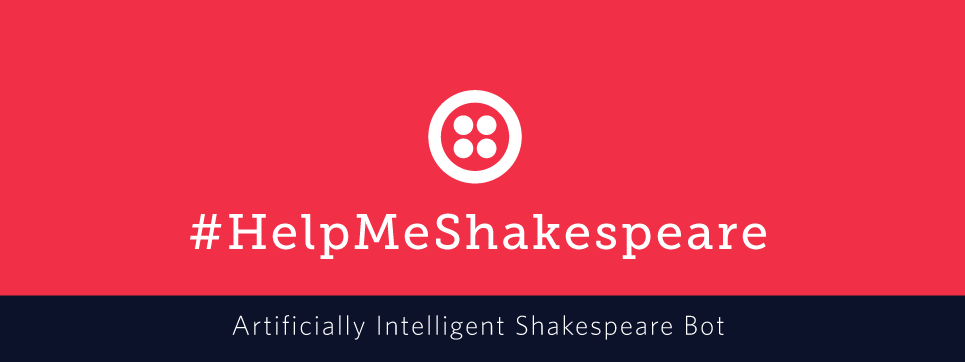
Valentine’s day isn’t my favorite holiday, mostly because I never seem to get my act together before the big day. But even though the holiday leaves me confused and clueless, the thought of adding a little more love into the world never does. So this year, instead of planning a wonderful night (like maybe I should have done?), I instead opened my laptop and decided to resurrect a 400 year-old poet who had a lot to say on the subject of love— Billy H. Shakespeare.
The thinking was simple, since I’m notoriously bad at writing romantic cards, maybe Shakespeare could do some of the heavy lifting for me. In short I wanted to create an artificially intelligent Shakespeare bot that would:
- Tell me if my romantic prose was good or awful
- Create a custom sonnet for me on demand
Worst-case scenario was that it would give me a good excuse to play with the Twilio-IBM Watson integration; best-case scenario was that it would save my Valentine’s day!
You can try out the finished product now by texting “Help Me Shakespeare!” to (501) 712-4613.
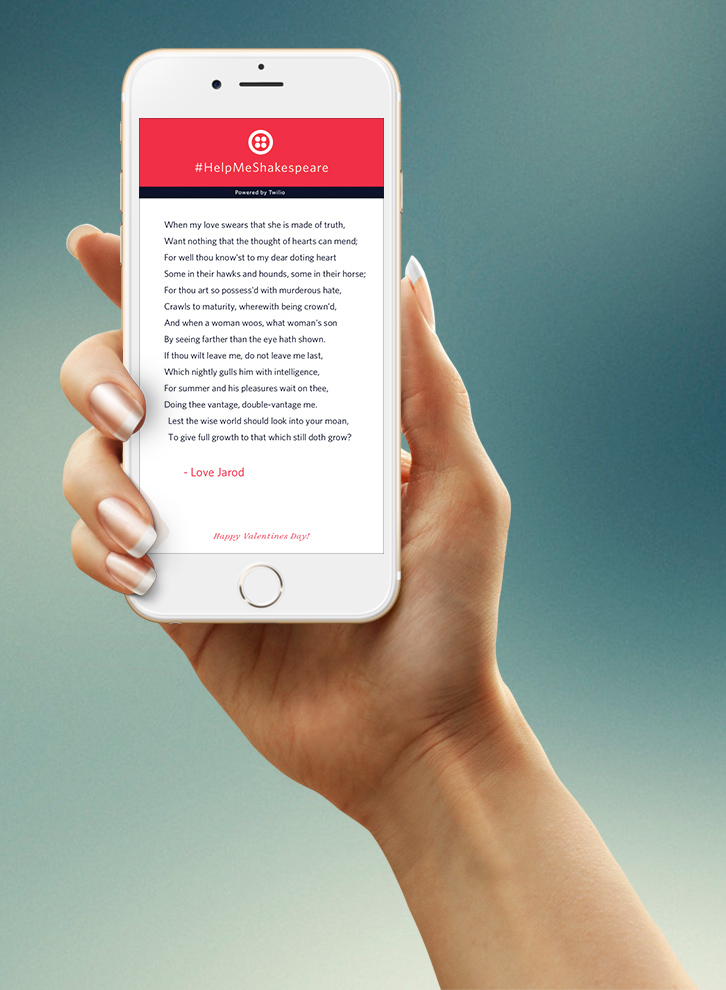
You can see the full code on github, but let’s walk through some of the challenges of resurrecting Shakespeare.
Writing a sonnet from scratch
The first custom piece we need to figure out is how to generate Shakespeare poetry. Luckily there is an awesome API called PoetryDB which allows us to search and filter by poet. Right in the documentation there was an example that searched for Shakespeare sonnets by filtering the results to only poems that were exactly 14 lines long.
But since these sonnets would make for fairly long text messages, which would be pretty hard to send to a special someone, we should try and generate an image of the poem. So to do that let’s use node-gd and generate a simple gif of our new rad poem.
Artificial Intelligence
To embed some artificial intelligence into this bot I needed to use Watson’s sentiment analysis to determine whether a phrase or sentence was positive or not. So let’s add the ability for our Shakespeare-bot to check the positivity of a message. Depending on whether or not it’s positive, we either send an uplifting message or we send a discouraging note with a new custom sonnet.
It turns out there is a fairly high correlation between a positive message and a generally nice message so it seems to work fairly consistently.
Notice that to grab the sentiment data in node, we first needed to JSON.parse() the string that was returned inside of req.body.AddOns we had to do this because even with body-parser
extended, express still does not create javascript objects for the nested objects in the Twilio response.
So let’s recap, we have an AI-driven bot that analyzes my romantic dexterity, generates custom Shakespeare poems and creates shareable images of those poems. Now all we need to put a bow on this is a user database, some routing in express and some logic to switch responses based on user input. To see how the code that handles all of this and more, go to github.
Otherwise enjoy your weekend, and if you have any questions about this app, how my valentine’s day ended up going, or you just want to dream up the next weird Twilio app– shoot me a note on twitter I’m always happy to jam.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.